Setting Up a React Native App
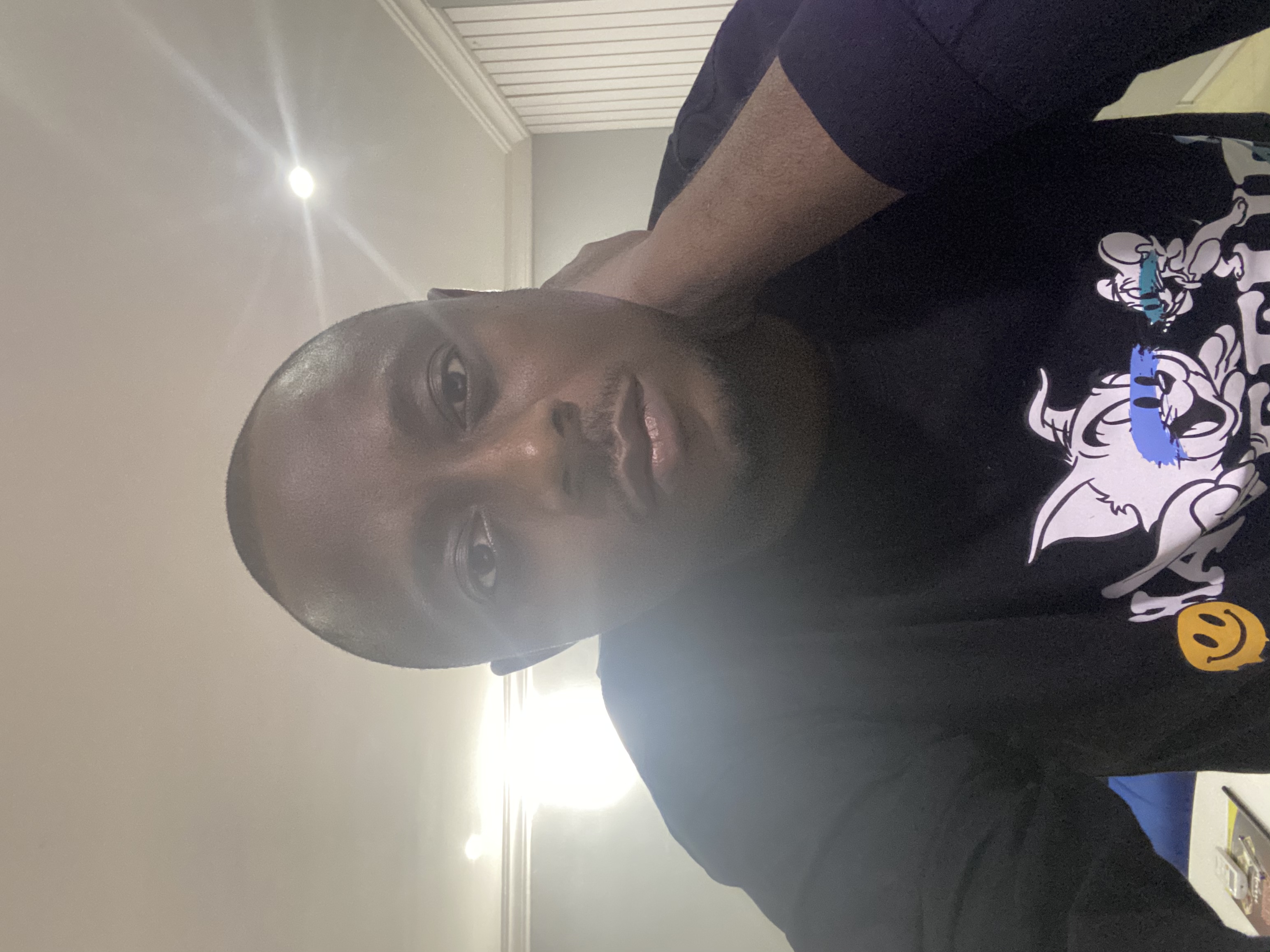
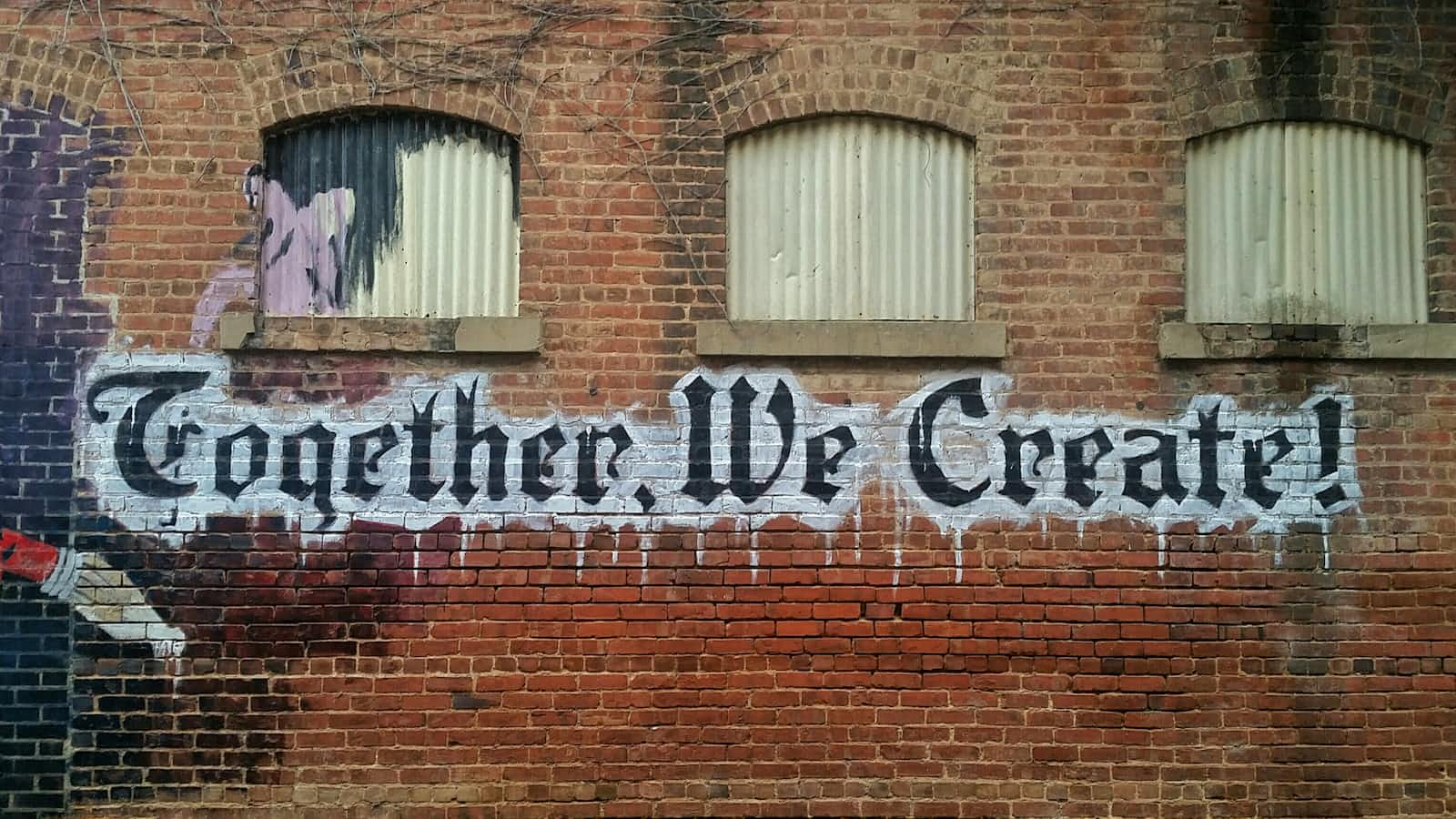
React Native is a popular framework for building mobile applications using JavaScript and React. Expo is a set of tools built around React Native that makes it easier to develop, build, and deploy React Native apps. In this blog post, we will walk through the steps to set up a simple "Hello World" application using React Native and Expo.
Prerequisites
Before we start, make sure you have the following installed on your machine:
Node.js and npm: You can download and install them from nodejs.org.
Expo CLI: Install it globally using npm with the command:
npm install -g expo-cli
Step 1: Create a New React Native Project
First, we will create a new React Native project using Expo. Open your terminal and run the following command:
npx expo-cli init HelloWorldApp
You will be prompted to choose a template. Select the "blank" template. This will set up a new project with the necessary configuration.
Step 2: Navigate to Your Project Directory
Once the project is created, navigate to the project directory:
cd HelloWorldApp
Step 3: Open Your Project in a Code Editor
Open the project in your preferred code editor, such as Visual Studio Code. You can do this directly from the terminal if you have Visual Studio Code installed:
code .
Step 4: Modify the App.js
File
In the project directory, locate the App.js
file and open it. Replace its content with the following code to display "Hello World":
import React from 'react';
import { Text, View, StyleSheet } from 'react-native';
const App = () => {
return (
<View style={styles.container}>
<Text style={styles.text}>Hello World</Text>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: '#F5FCFF',
},
text: {
fontSize: 20,
textAlign: 'center',
margin: 10,
},
});
export default App;
Detailed Breakdown of the Code
Imports:
import React from 'react'; import { Text, View, StyleSheet } from 'react-native';
- Import necessary components from
react-native
.
- Import necessary components from
App Component:
const App = () => { return ( <View style={styles.container}> <Text style={styles.text}>Hello World</Text> </View> ); };
- Define the main component
App
which returns aView
containing aText
component displaying "Hello World".
- Define the main component
Styles:
const styles = StyleSheet.create({ container: { flex: 1, justifyContent: 'center', alignItems: 'center', backgroundColor: '#F5FCFF', }, text: { fontSize: 20, textAlign: 'center', margin: 10, }, });
- Create a
StyleSheet
to style theView
andText
components.
- Create a
Export:
export default App;
- Export the
App
component as the default export of the module.
- Export the
Step 5: Move the Project Directory to the Desktop
For easier access, you can move your project directory to the Desktop. Run the following command in your terminal:
mv ~/HelloWorldApp ~/Desktop/
Step 6: Run Your Project
Finally, to run your project, navigate to the project directory on your Desktop and start the Expo development server:
cd ~/Desktop/HelloWorldApp
npx expo start
This command will open the Expo DevTools in your web browser. From here, you can run your app on an Android emulator, iOS simulator, or a physical device using the Expo Go app. Simply scan the QR code provided by Expo DevTools with the Expo Go app on your device to see your "Hello World" application in action.
Conclusion
Congratulations! You have successfully set up and run a "Hello World" application using React Native and Expo. This simple example serves as a great starting point for exploring the powerful features of React Native and Expo.
Subscribe to my newsletter
Read articles from Ogunuyo Ogheneruemu B directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
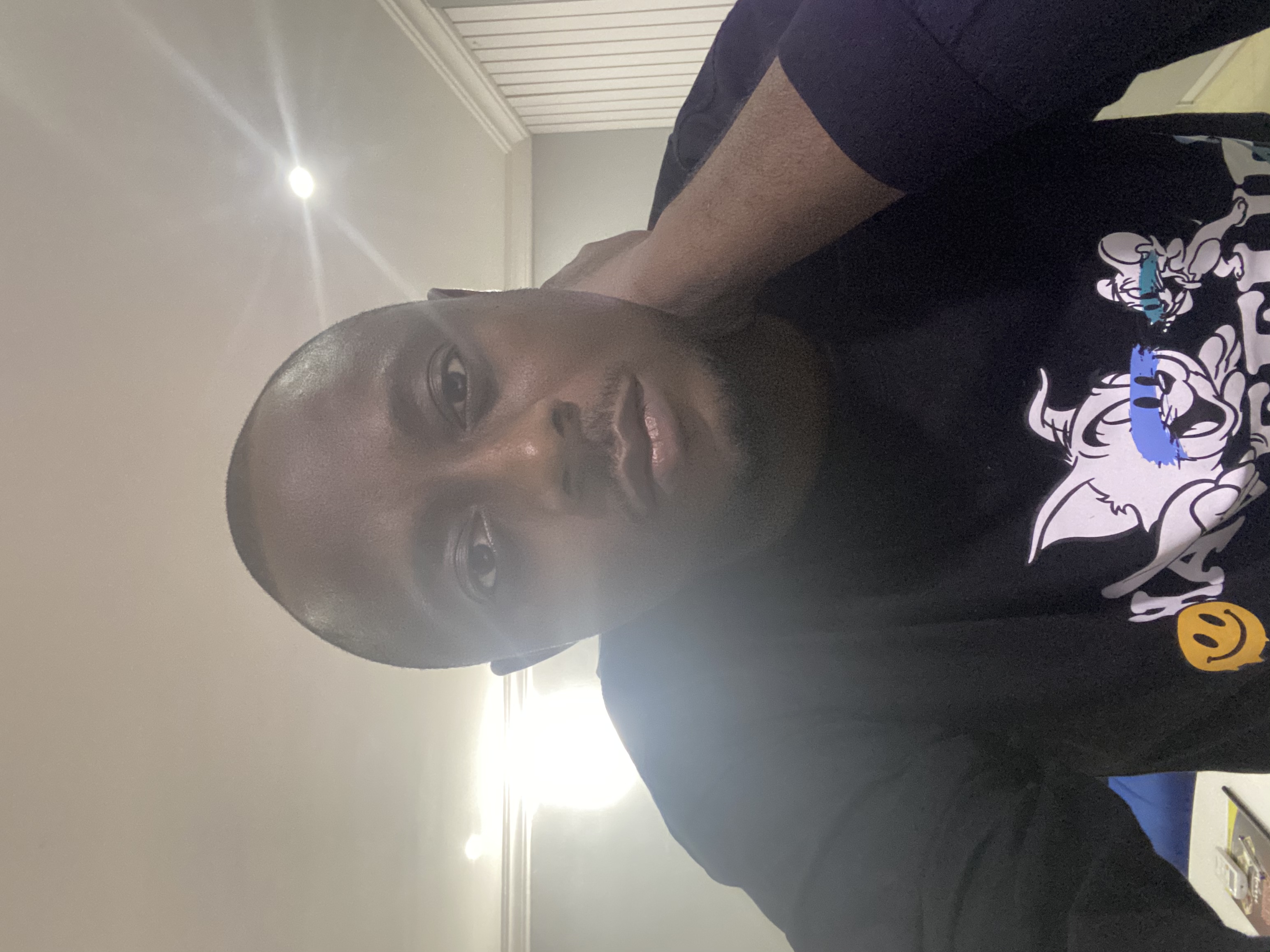
Ogunuyo Ogheneruemu B
Ogunuyo Ogheneruemu B
I'm Ogunuyo Ogheneruemu Brown, a senior software developer. I specialize in DApp apps, fintech solutions, nursing web apps, fitness platforms, and e-commerce systems. Throughout my career, I've delivered successful projects, showcasing strong technical skills and problem-solving abilities. I create secure and user-friendly fintech innovations. Outside work, I enjoy coding, swimming, and playing football. I'm an avid reader and fitness enthusiast. Music inspires me. I'm committed to continuous growth and creating impactful software solutions. Let's connect and collaborate to make a lasting impact in software development.