Guide to Dynamic Routing in Next.js 14 Using App Router
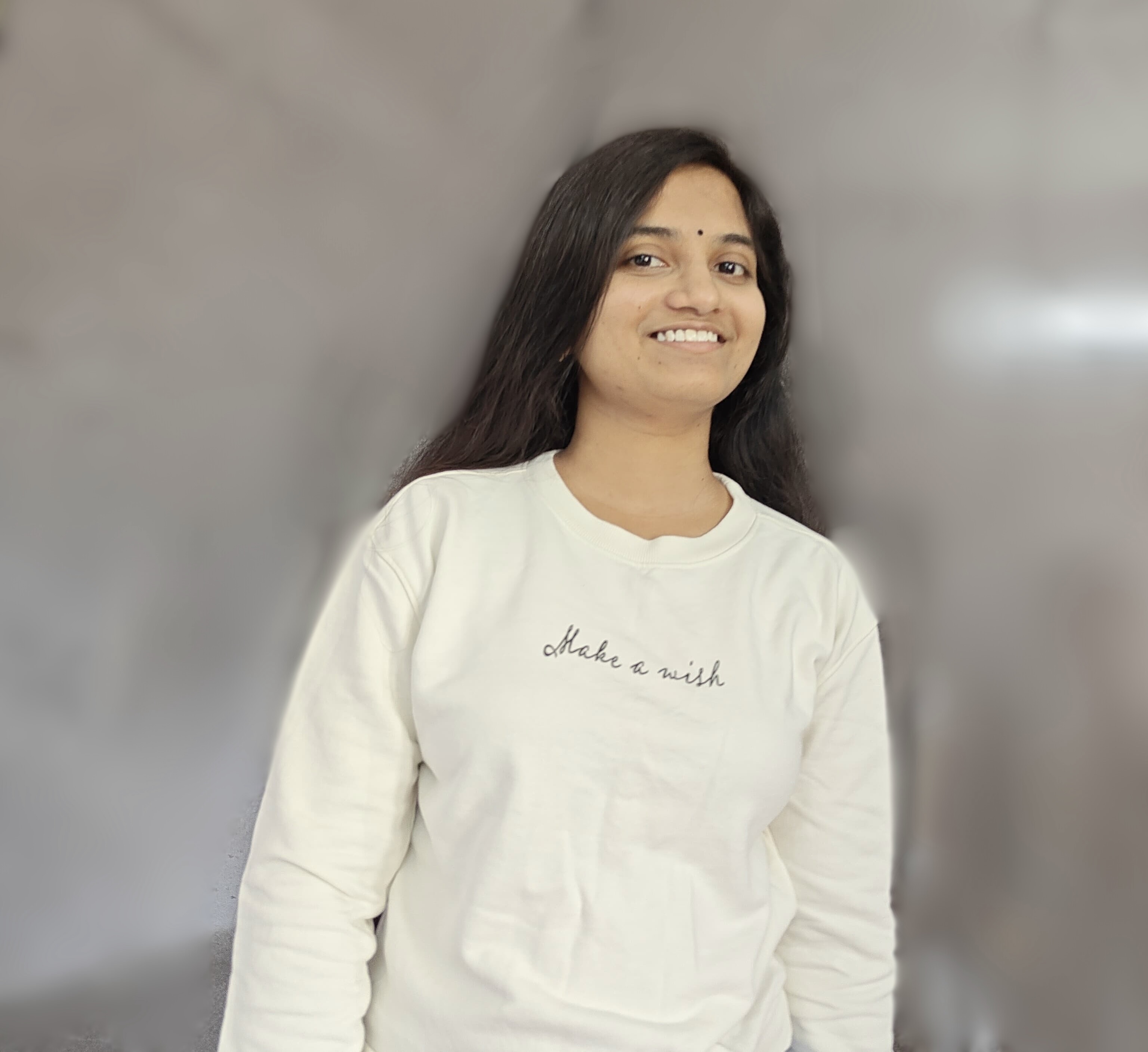
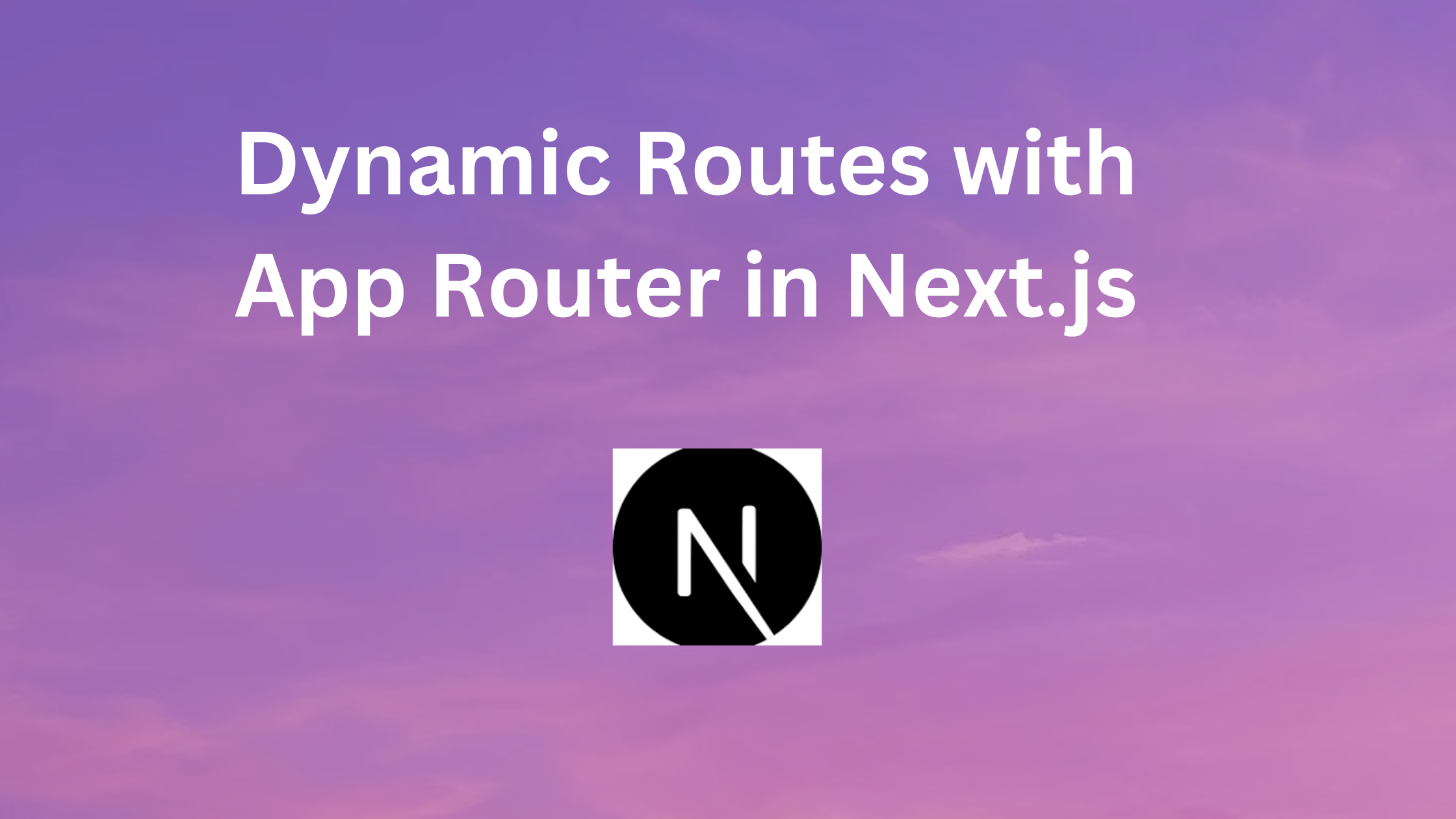
Let's dive into setting up and understanding dynamic routes in Next.js 14 using .tsx files. We'll go through the process of creating a blog application that uses dynamic routes for individual blog posts.
File Structure Overview: We'll design a project where each blog post has a unique ID, and you can access each post via a dynamic route. Hereโs the structure we'll follow:
/app
/blog
[id]
page.tsx
page.tsx
layout.tsx
page.tsx
Blog Page (/app/blog/page.tsx
)
This page lists all blog posts.
// /app/blog/page.tsx
import Link from 'next/link';
const BlogPage = () => {
const posts = [
{ id: '1', title: 'First Post' },
{ id: '2', title: 'Second Post' },
];
return (
<div>
<h1>Blog</h1>
<ul>
{posts.map((post) => (
<li key={post.id}>
<Link href={`/blog/${post.id}`}>{post.title}</Link>
</li>
))}
</ul>
</div>
);
};
export default BlogPage;
Explanation:
This page fetches or defines a list of blog posts.
Each post is displayed with a link to its individual page using a dynamic route (
/blog/${
post.id
}
).http://localhost:3000/blog
Dynamic Blog Post Page (/app/blog/[id]/page.tsx
)
This page handles individual blog posts using a dynamic route.
"use client";
// /app/blog/[id]/page.tsx
import { useParams } from "next/navigation";
const BlogPostPage = () => {
const params = useParams();
const { id } = params;
return (
<div>
<h1>Blog Post {id}</h1>
<p>Content for blog post {id} goes here...</p>
</div>
);
};
export default BlogPostPage;
Explanation
We import
useParams
fromnext/navigation
The
useParams
hook provides access to the dynamic route parameters.We extract the
id
parameter from theparams
object.
We use the id
parameter to display content specific to the blog postDetailed Explanation
http://localhost:3000/blog/2
Dynamic Routing Mechanism
- File-based Routing:
Next.js uses the file and directory structure within the
pages
orapp
directory to define routes.Files named with square brackets (e.g.,
[id].tsx
) are treated as dynamic routes, where the part inside the brackets is used as a variable.
- Creating Dynamic Routes:
When a file named
[id].tsx
is placed in thepages
orapp
directory, it creates a dynamic route that matches URLs with that pattern.For example, a file located at
/app/blog/[id]/page.tsx
will match URLs like/blog/1
,/blog/2
, etc., where1
and2
are dynamic values.
- Accessing Dynamic Parameters:
The
useparams
hook from Next.js provides access to the current route parameters.The
params
object contains key-value pairs representing the dynamic parts of the URL. In this case,params.id
would contain the value1
when accessing/blog/1
.
Benefits of Dynamic Routing
Flexibility
Dynamic routes allow you to create pages that can handle a variety of content based on URL parameters.
This is useful for applications with many similar pages differentiated by a unique identifier (e.g., product pages, user profiles, etc.)
Scalability:
- As your application grows, you can easily add new routes and handle dynamic content without changing the routing logic.
Dynamic routes reduce the need for creating multiple static pages for each piece of content.
Maintainability:
- Using dynamic routes keeps your codebase clean and organized by leveraging Next.js's file-based routing system.
It allows you to focus on the logic for handling content rather than managing a complex routing configuration.
I hope you enjoyed the blog. If you liked the content, please share and give it a like. Happy coding! ๐
Subscribe to my newsletter
Read articles from Sruthi Palle directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
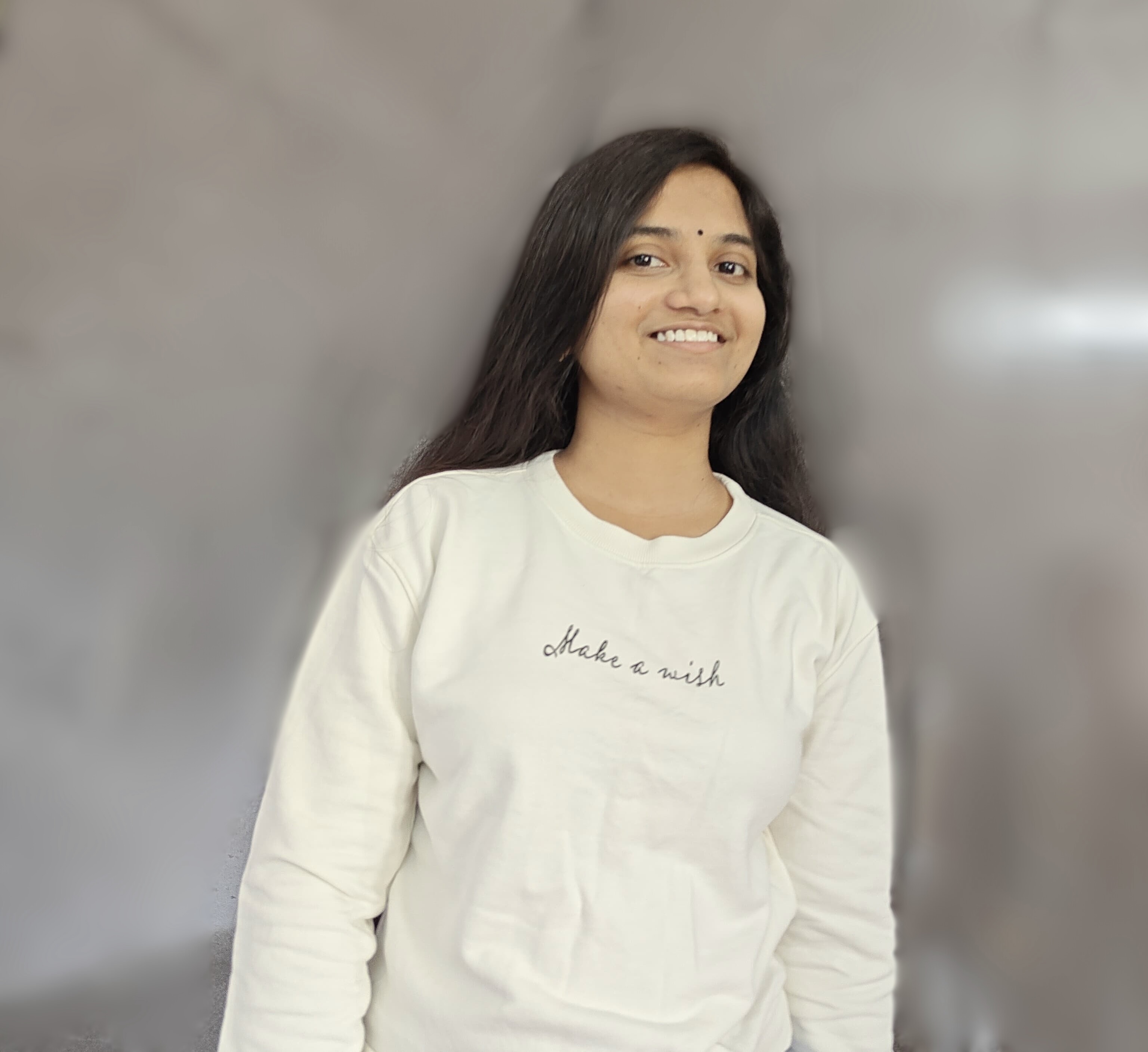
Sruthi Palle
Sruthi Palle
๐ I'm Sruthi , a Full Stack developer with around 3 years of experience, currently working at HTC Global services as a Full Stack developer. My expertise lies in frontend development, where I've dived into various frameworks and technologies like Html, Css, JavaScript, ReactJs, Redux, NextJs, Typescript, NodeJS,Expressjs, Mongodb, Material Ui, TailwindCSS, Ant-Design, RESTAPI's, SVN, Git, Postman, AWS Beyond my professional endeavors, I'm deeply passionate about continuous self-improvement. I firmly believe in the power of growth, both in the professional and personal spheres. I'm also committed to giving back to the tech community. You can find valuable blog content.