Effective Configuration Management with Chef
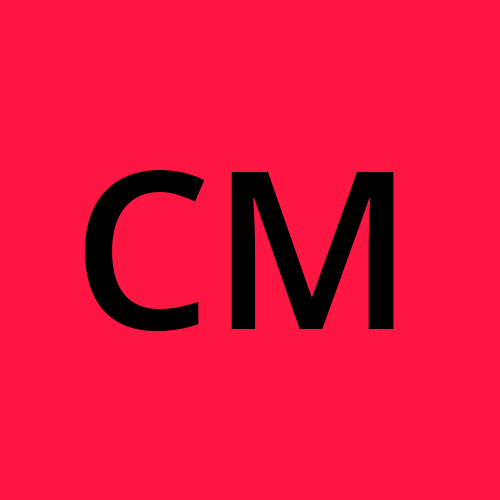
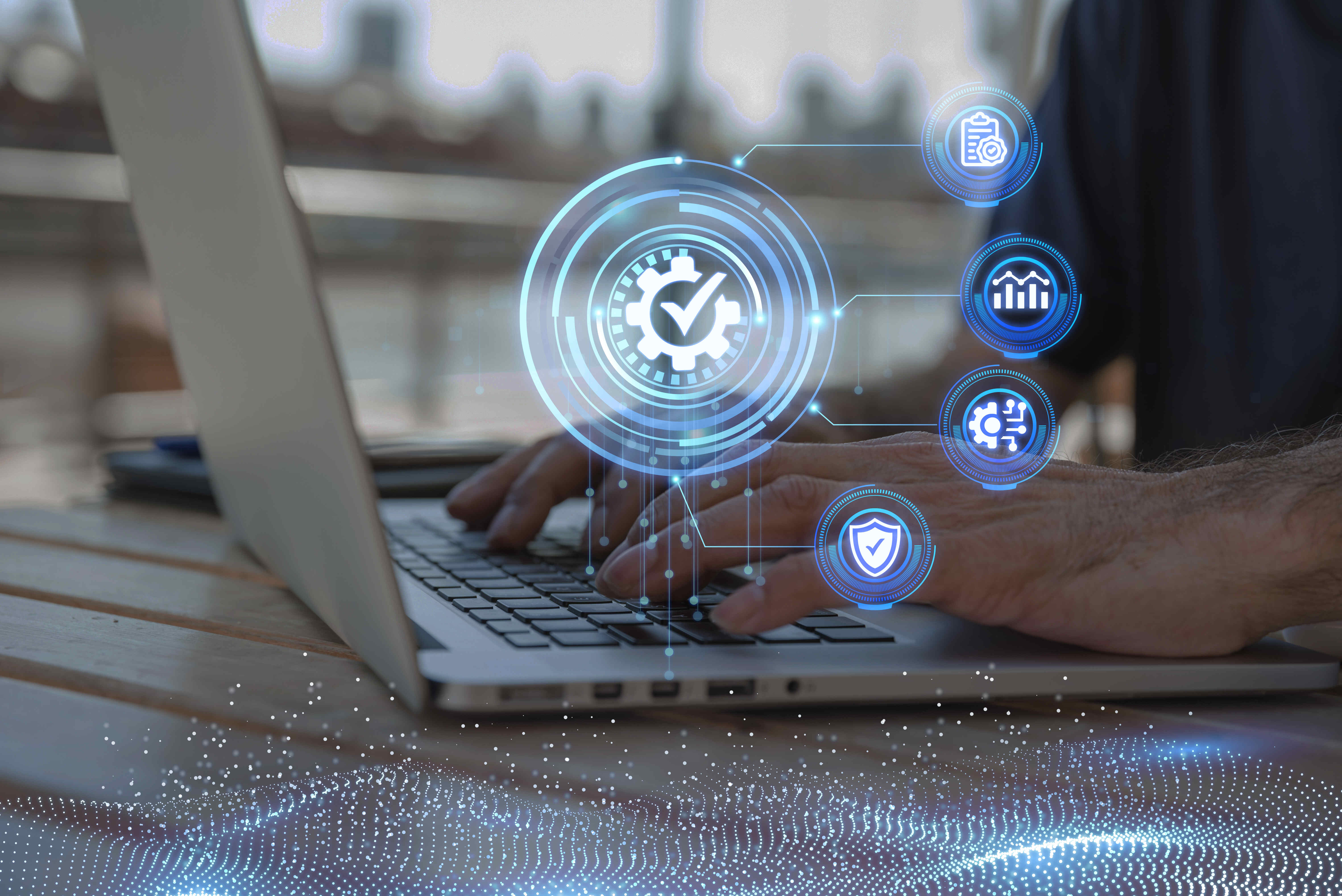
Introduction
Hey there! If you're diving into the world of DevOps, you've probably heard the buzz about configuration management. It's a game-changer for maintaining consistency across your systems. One tool that stands out in this arena is Chef. Today, I’ll walk you through how to use Chef for efficient configuration management in DevOps environments. We'll cover everything from getting started to integrating Chef with CI/CD pipelines. So, buckle up and let's get cooking!
Getting Started with Chef
Overview of Chef
First things first, what exactly is Chef? Chef is a powerful automation platform that transforms infrastructure into code. It allows you to define your infrastructure as code using simple, reusable definitions, making it easy to manage and scale your systems. With Chef, you can automate the configuration, deployment, and management of your applications across your entire infrastructure.
Installation and Setup
Alright, let’s get our hands dirty. Installing Chef is pretty straightforward. Here’s a step-by-step guide to get you up and running:
1. Download the Chef Development Kit (ChefDK): Head over to the Chef website and download the ChefDK for your operating system.
Install ChefDK: Run the installer you just downloaded. For example, on macOS, you’d run:
sudo dpkg -i /path/to/chefdk.deb
2. Verify the Installation: Open your terminal and type:
chef -v
You should see the Chef version displayed, indicating a successful installation.
3. Set Up Your Chef Repository: Create a new Chef repository by running:
chef generate repo my_chef_repo
Navigate to your new repository:
cd my_chef_repo
There you have it! You’re now ready to start writing Chef cookbooks.
Writing Chef Cookbooks
Defining Configurations
Chef cookbooks are the heart and soul of your configuration management. They contain recipes, which are collections of resources that describe the desired state of your system. Here’s a simple example to illustrate:
1. Create a Cookbook: Generate a new cookbook:
chef generate cookbook my_cookbook
2. Write a Recipe: Open the recipes/default.rb file in your cookbook and define a basic configuration:
package 'apache2' do
action :install
end
service 'apache2' do
action [:enable, :start]
end
file '/var/www/html/index.html' do
content '<h1>Hello, world!</h1>'
action :create
end
3. Upload the Cookbook to the Chef Server: If you’re using Chef Server, upload your cookbook:
knife cookbook upload my_cookbook
Example Cookbook
To make things clearer, let’s look at a more detailed example. Imagine you want to set up a web server with a custom homepage:
1. Create a Cookbook:
chef generate cookbook web_server
2. Write the Recipe: Edit recipes/default.rb:
package 'nginx' do
action :install
end
service 'nginx' do
action [:enable, :start]
end
template '/usr/share/nginx/html/index.html' do
source 'index.html.erb'
mode '0644'
end
3. Create the Template: Add a new file templates/default/index.html.erb:
<html>
<body>
<h1>Welcome to my website!</h1>
<p>This page is managed by Chef.</p>
</body>
</html>
4. Upload and Apply the Cookbook:
knife cookbook upload web_server
knife bootstrap <node_ip> --run-list 'recipe[web_server]'
This cookbook installs Nginx, starts the service, and deploys a custom homepage. Simple, yet powerful!
Managing Nodes with Chef
Node Management
Managing nodes in Chef involves defining which configurations (recipes) should be applied to which nodes. Here are some best practices:
1. Use Roles: Roles allow you to group configurations. For example, you can create a role for web servers:
{
"name": "web_server",
"run_list": [
"recipe[web_server]"
]
}
2. Use Environments: Environments help manage configurations across different stages like development, testing, and production. Define environments in JSON files:
{
"name": "production",
"description": "Production environment",
"cookbook_versions": {
"web_server": "= 1.0.0"
}
}
3. Automate Node Configuration: Use the knife command to assign roles and environments to nodes:
knife node run_list add <node_name> 'role[web_server]'
knife environment from file production.json
knife node environment set <node_name> production
Chef Server vs. Chef Solo
When it comes to deploying Chef, you have two main options: Chef Server and Chef Solo. Let’s break them down:
Chef Server: A central server that stores cookbooks, roles, environments, and more. It’s great for larger infrastructures with multiple nodes.
Chef Solo: A lightweight alternative that doesn’t require a central server. Ideal for smaller setups or development environments.
Here’s a quick comparison table:
Feature | Chef Server | Chef Solo |
Centralized Storage | Yes | No |
Scalability | High | Limited |
Use Cases | Large infrastructures | Small setups, development |
Complexity | Higher | Lower |
Example Code Snippets and Charts
Here are a few additional snippets to give you a head start on various tasks:
Creating a User with Chef
user 'deploy' do
comment 'Deployment User'
uid '1234'
home '/home/deploy'
shell '/bin/bash'
password 'password_hash'
end
Managing a Service with Chef
service 'nginx' do
supports status: true, restart: true, reload: true
action [:enable, :start]
end
Sample Table
To summarize some of the features and tools we've discussed, here's a handy table:
Feature | Description | Tool/Command |
Install ChefDK | Install Chef Development Kit | chef-dk |
Create Cookbook | Generate a new Chef cookbook | chef generate cookbook <name> |
Upload Cookbook | Upload cookbook to Chef Server | knife cookbook upload <name> |
Bootstrap Node | Configure a new node with Chef | knife bootstrap <node_ip> |
CI/CD Pipeline | Integrate Chef with Jenkins/GitLab CI | knife, .gitlab-ci.yml |
Manage Env | Define and manage multiple environments | knife environment |
Sample Chart
To visualize the workflow of integrating Chef with a CI/CD pipeline, here's a simple chart:
plaintext |
This flow ensures that your configurations are tested and deployed consistently, enhancing reliability and speed.
Integrating Chef with CI/CD Pipelines
CI/CD Integration
Integrating Chef with your CI/CD pipeline automates the deployment process, ensuring consistency and speed. Let’s see how to do this with Jenkins:
1. Install Jenkins: Follow the installation guide on the Jenkins website.
2. Install Chef Plugin: Go to Jenkins > Manage Jenkins > Manage Plugins and install the Chef plugin.
3. Configure Jenkins Job: Create a new Jenkins job and configure it to use Chef: Source Code Management: Connect to your repository.
Build Steps: Add a step to execute Chef commands:
knife cookbook upload my_cookbook
knife bootstrap <node_ip> --run-list 'recipe[my_cookbook]'
Automate Testing: Add a post-build step to run tests, ensuring everything works as expected:
chef exec rspec
Example Setup
To give you a practical example, let’s integrate Chef with GitLab CI:
1. Create .gitlab-ci.yml: Define your CI/CD pipeline in this file:
stages:
- build
- test
- deploy
build:
stage: build
script:
- chef exec berks install
test:
stage: test
script:
- chef exec rspec
deploy:
stage: deploy
script:
- knife cookbook upload my_cookbook
- knife bootstrap <node_ip> --run-list 'recipe[my_cookbook]'
2. Commit and Push: Commit your changes and push them to your GitLab repository. Your pipeline will automatically trigger, building, testing, and deploying your Chef configurations.
Advanced Chef Features
Using Chef with Multiple Environments
Managing configurations across different environments can be tricky, but Chef makes it easier. Here’s how:
1. Define Environments: Create environment files in JSON format:
{
"name": "staging",
"description": "Staging environment",
"cookbook_versions": {
"web_server": "= 1.0.0"
}
}
2. Assign Nodes to Environments: Use the knife command to assign nodes:
knife node environment set <node_name> staging
3. Use Environment-specific Cookbooks: Define different configurations for each environment within your cookbooks.
Chef Automate
For those who want to take their automation to the next level, Chef Automate is the way to go. It provides end-to-end visibility, compliance, and automated workflows. Here’s a quick look at its features:
Visibility: Real-time insights into your infrastructure.
Compliance: Ensure your systems meet regulatory requirements.
Automated Workflows: Streamline your operations with automated pipelines.
The Role of DevOps Consultations in Chef Implementations
Enhancing Chef Implementations
You might wonder, "Can I get more out of Chef?" The answer is yes! Professional DevOps consultations can help you optimize your Chef usage, ensuring you get the best out of your configurations. A DevOps service provider company can offer tailored solutions, from initial setup to ongoing management, helping you avoid common pitfalls and achieve your goals faster.
Case Study: Example of a Company Benefiting from DevOps Consultations
Let me share a real-life example to show how impactful DevOps consultations can be. Imagine a growing e-commerce startup struggling with inconsistent configurations and deployment failures. They decided to bring in a DevOps consulting company to help streamline their processes.
The consultants started by evaluating their existing infrastructure and identifying pain points. They introduced Chef to automate their configurations and integrated it with their CI/CD pipeline using Jenkins. The result? Deployment times were slashed by 50%, and system reliability improved dramatically. The company could now roll out updates faster and with greater confidence, directly contributing to their rapid growth.
Final Thoughts
Summary of Key Points Discussed
Alright, let's recap what we've covered today. We started with a brief introduction to configuration management and the pivotal role Chef plays in DevOps environments. From there, we explored how to get started with Chef, including installation, setup, and writing your first cookbooks. We dove into managing nodes, comparing Chef Server and Chef Solo, and integrating Chef with CI/CD pipelines.
We also touched on advanced features like managing multiple environments and using Chef Automate for enhanced automation and reporting. Finally, we discussed the value of DevOps consultations and how they can optimize your Chef implementations.
I hope this guide has been helpful for you. Using Chef for configuration management in DevOps environments can make a world of difference.
Frequently Asked Questions (FAQs)
1. What is Chef and how does it work?
Chef is a powerful configuration management tool used to automate the setup and management of infrastructure. It uses a master-agent architecture where the Chef server communicates with Chef clients to apply configurations, known as cookbooks, across different nodes.
2. What are Chef cookbooks and recipes?
Cookbooks in Chef are collections of configurations, policies, and resources. They contain recipes, which are specific configurations written in Ruby that describe how particular parts of your infrastructure should be configured, such as installing software or setting system parameters.
3. How does Chef handle security?
Chef uses tools like Chef Vault to securely store and manage sensitive data like passwords and SSL keys. It also supports compliance and security audits through Chef InSpec, which can enforce policies and remediate any misconfigurations identified during audits.
4. What are the different versions of Chef available?
Chef offers several versions to suit different needs: Hosted Chef, which is a cloud-based service; Chef Server, which you manage yourself; and Private Chef, an enterprise version for internal infrastructure. Each provides different levels of control and management capabilities.
5. How does Chef integrate with CI/CD pipelines?
Chef can be integrated into CI/CD pipelines using tools like Jenkins or GitLab CI. This integration ensures that infrastructure changes are tested and deployed automatically alongside application code, maintaining consistency across development, testing, and production environments.
Subscribe to my newsletter
Read articles from Charles Mason directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
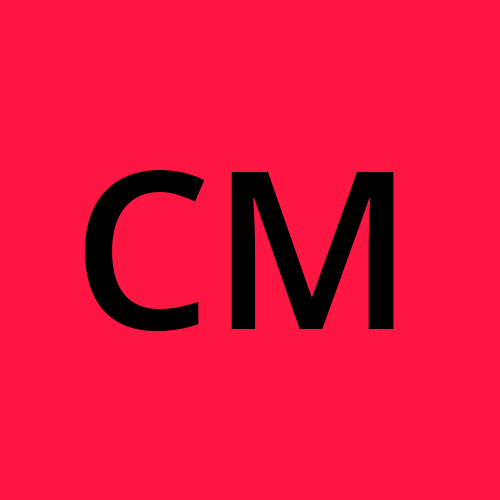