Anagram Problem in Elixir
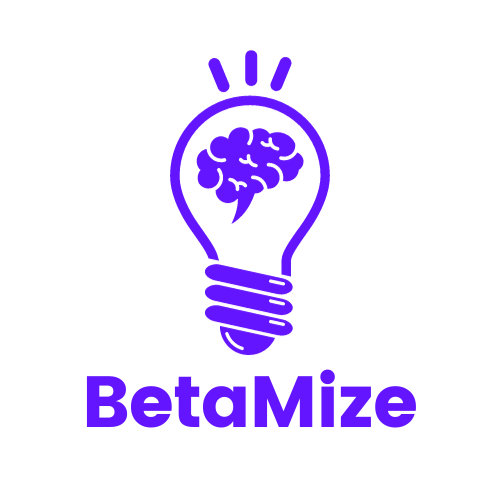
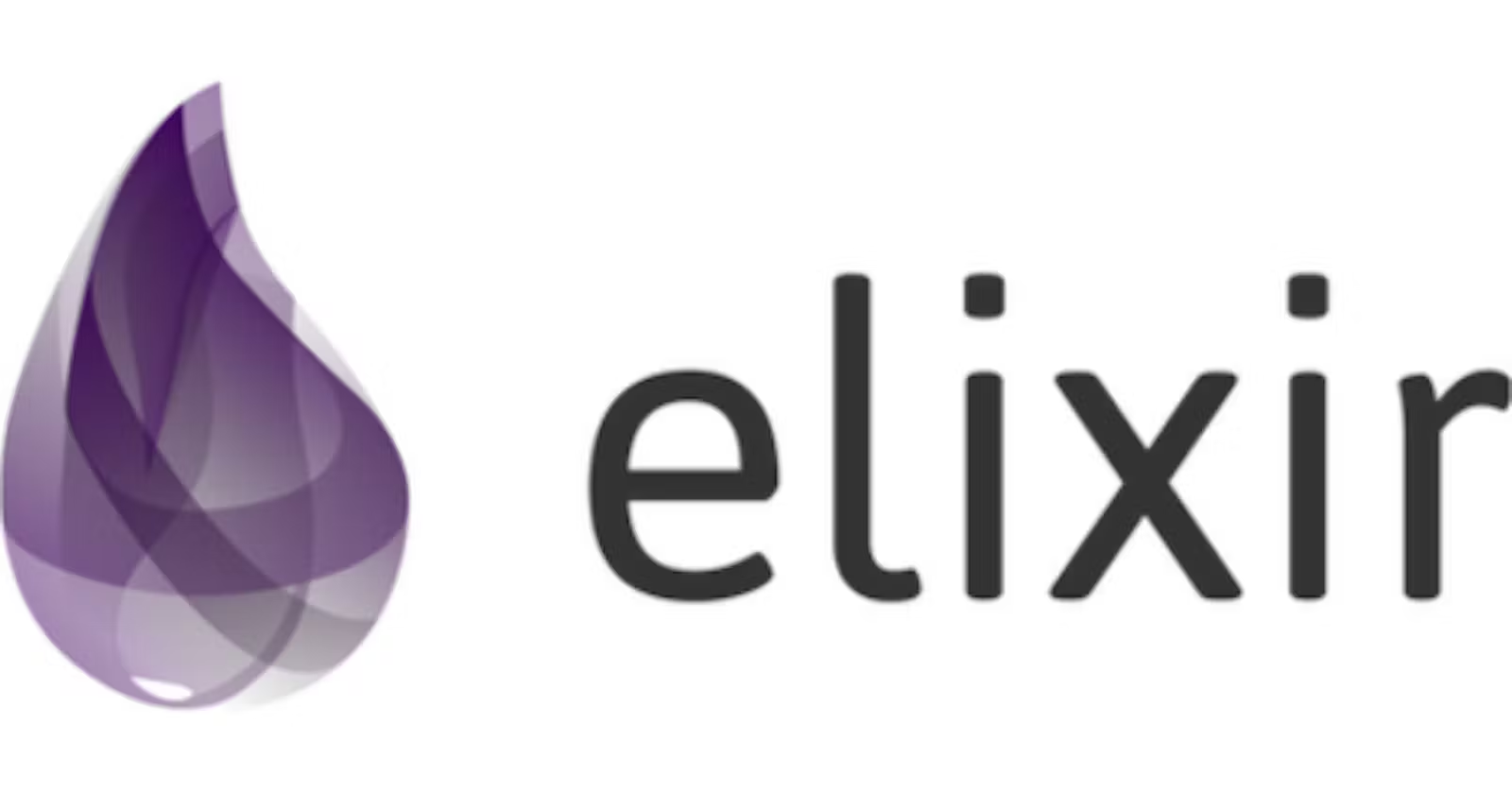
An anagram is a rearrangement of letters to form a new word. For example, "owns" is an anagram of "snow". An interesting twist is that a word is not considered its own anagram. In this article, we will explore how to solve the anagram problem using Elixir.
Problem Definition
Given a target word and a list of candidate words, we need to find the subset of candidates that are anagrams of the target word. The conditions are:
Lowercase and uppercase characters are equivalent, but the case of the letters should match the original case in the candidate set.
A word is not considered its own anagram.
Example
For example, given the target word "stone" and the candidates ["stone", "tones", "banana", "tons", "notes", "Seton"]
, the anagram set is ["tones", "notes", "Seton"]
.
Solution in Elixir
To solve this problem, we'll follow these steps:
Normalize the target word by sorting its characters.
Iterate over the list of candidate words.
Normalize each candidate word by sorting its characters.
Compare the normalized candidate word with the normalized target word.
Collect candidates that match and are not identical to the target word.
Here's how we can implement this solution in Elixir:
Step 1: Normalize the word
We normalize a word by converting it to lowercase and sorting its characters.
def normalize(word) do
word
|> String.downcase()
|> String.graphemes()
|> Enum.sort()
|> Enum.join()
end
Step 2: Find anagrams
We iterate over the list of candidates and collect those that match the normalized target word, excluding the target word itself.
defmodule Anagram do
def find_anagrams(target, candidates) do
normalized_target = normalize(target)
candidates
|> Enum.filter(fn candidate ->
candidate_normalized = normalize(candidate)
candidate_normalized == normalized_target and String.downcase(candidate) != String.downcase(target)
end)
end
defp normalize(word) do
word
|> String.downcase()
|> String.graphemes()
|> Enum.sort()
|> Enum.join()
end
end
Step 3: Testing the solution
Let's test our solution with the given example.
target = "stone"
candidates = ["stone", "tones", "banana", "tons", "notes", "Seton"]
anagrams = Anagram.find_anagrams(target, candidates)
IO.inspect(anagrams) # Output should be ["tones", "notes", "Seton"]
Explanation
Normalization: The
normalize/1
function converts the word to lowercase, splits it into characters, sorts them, and joins them back into a string. This helps in comparing two words irrespective of their original case or order of characters.Filtering Candidates: The
find_anagrams/2
function iterates over each candidate, normalizes it, and checks if it matches the normalized target word. It also ensures that the candidate is not the same as the target word by comparing their lowercase forms.
Conclusion
The anagram problem can be efficiently solved in Elixir by leveraging its powerful string manipulation and enumeration capabilities. The approach of normalizing words by sorting their characters simplifies the comparison process and ensures that we can accurately identify anagrams. This solution is both concise and effective, demonstrating the strengths of Elixir for text processing tasks.
If you're interested in more Elixir tutorials and real-time web development solutions, visit ElixirMasters for expert development services, developer hiring solutions, and consultancy. Elevate your project with the power of Elixir today!
Subscribe to my newsletter
Read articles from BetaMize directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
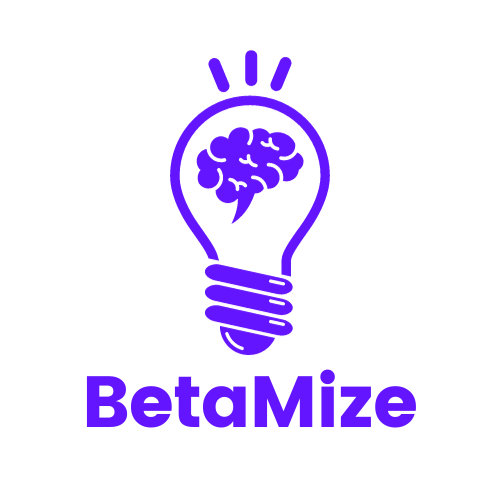