Converting Numbers to Words: Rupees and Paise in JavaScript
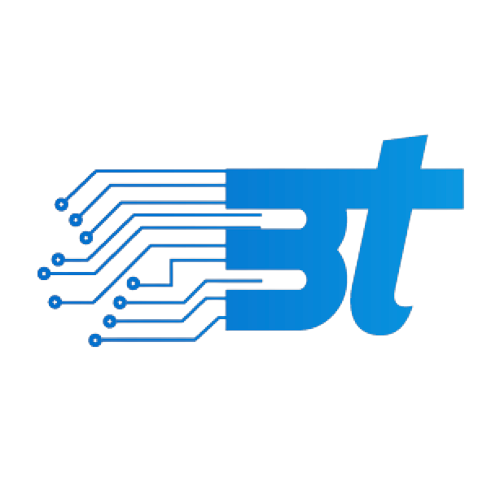
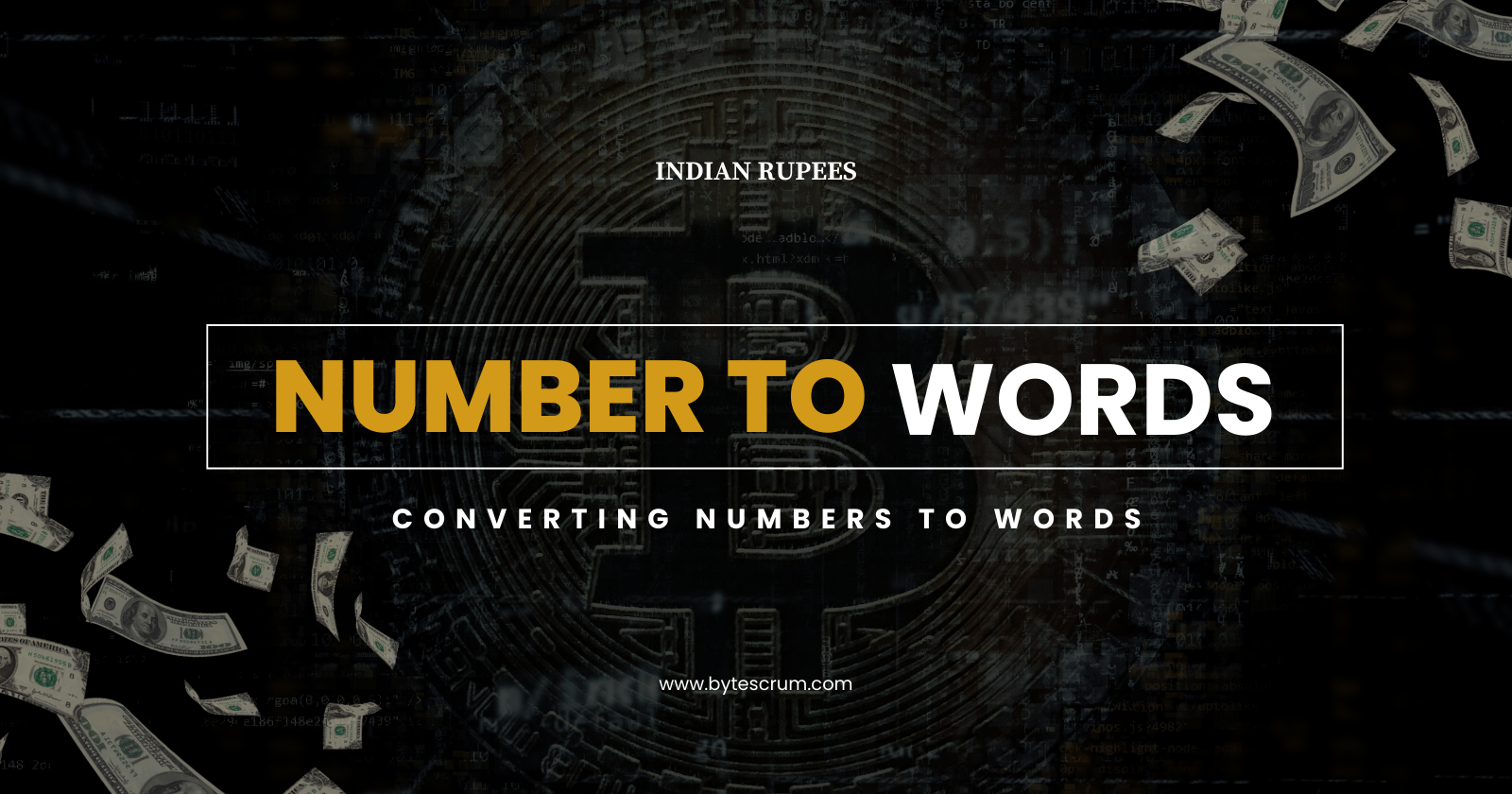
Working with currency in programming often requires converting numerical values into a human-readable format. One common task is to convert a number representing an amount of money into words, such as converting 101.25
into "one hundred one rupees and twenty-five paise." In this blog post, we will walk through a JavaScript function to accomplish this conversion.
Step-by-Step Solution
1. Splitting the Number
First, we need to separate the rupees and paise from the input number. This can be done using the split
method, which divides the number into two parts: the whole number (rupees) and the decimal part (paise).
2. Number to Words Conversion
We'll use two helper functions: one to convert numbers to words for the rupees part and another to convert the paise part.
3. Putting It All Together
Finally, we'll combine the two parts and handle the special cases where there might be no rupees or no paise.
Code Implementation
Here’s the complete JavaScript code to achieve this:
const ones = [
"",
"one",
"two",
"three",
"four",
"five",
"six",
"seven",
"eight",
"nine",
];
const teens = [
"eleven",
"twelve",
"thirteen",
"fourteen",
"fifteen",
"sixteen",
"seventeen",
"eighteen",
"nineteen",
];
const tens = [
"",
"ten",
"twenty",
"thirty",
"forty",
"fifty",
"sixty",
"seventy",
"eighty",
"ninety",
];
const thousands = ["", "thousand", "lakh", "crore"];
function numberToWords(num) {
if (num === "0") return "zero";
let numStr = num.toString();
let word = "";
let n = numStr.length;
if (n > 9) return "overflow"; // number out of bounds
// Pad the number with leading zeros for easier parsing
numStr = numStr.padStart(9, "0");
// Split the number into groups of two
let crore = numStr.slice(0, 2);
let lakh = numStr.slice(2, 4);
let thousand = numStr.slice(4, 6);
let hundred = numStr[6];
let ten = numStr.slice(7);
if (parseInt(crore) > 0) {
word += `${convertTwoDigits(crore)} crore `;
}
if (parseInt(lakh) > 0) {
word += `${convertTwoDigits(lakh)} lakh `;
}
if (parseInt(thousand) > 0) {
word += `${convertTwoDigits(thousand)} thousand `;
}
if (parseInt(hundred) > 0) {
word += `${ones[hundred]} hundred `;
}
if (parseInt(ten) > 0) {
word += convertTwoDigits(ten);
}
return word.trim();
}
function convertTwoDigits(num) {
num = parseInt(num, 10);
if (num < 10) return ones[num];
if (num > 10 && num < 20) return teens[num - 11];
let unit = num % 10;
let ten = Math.floor(num / 10);
return `${tens[ten]} ${ones[unit]}`.trim();
}
function convertRupeesPaise(amount) {
let [rupees, paise] = amount.toString().split(".");
let rupeesInWords = numberToWords(parseInt(rupees));
let paiseInWords = paise ? convertTwoDigits(paise.padEnd(2, "0")) : "";
let result = "";
if (rupeesInWords) {
result += `${rupeesInWords} rupees`;
}
if (paiseInWords) {
result += ` and ${paiseInWords} paise`;
}
return result.trim();
}
console.log(convertRupeesPaise("101.25"));
Explanation
ones
,teens
,tens
: These arrays hold the word equivalents for single digits, teens, and tens respectively.thousands
: This array is used to handle thousands, lakhs, and crores.
convertToWords
Function
This function converts a numeric string into words by:
Iterating through each digit of the number.
Appending the corresponding word for each digit.
Handling special cases for teens and tens.
convertRupeesPaise
Function
This function:
Splits the input amount into rupees and paise.
Converts each part into words using the
convertToWords
function.Combines the rupees and paise parts into a final string.
Checkout this repo which has basic UI of this app.
Conclusion
Happy coding!
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
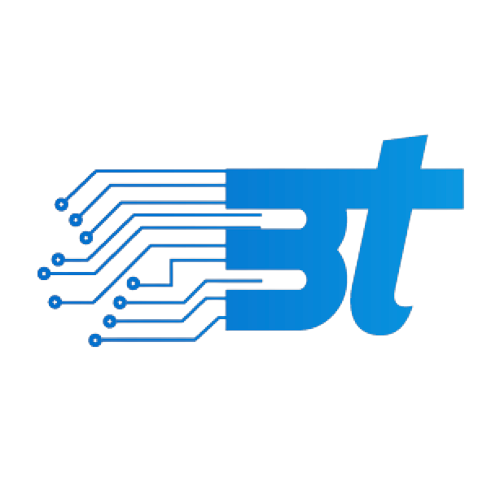
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.