Integrating bpmn-js-token-simulation & bpmn-js-properties-panel with Angular
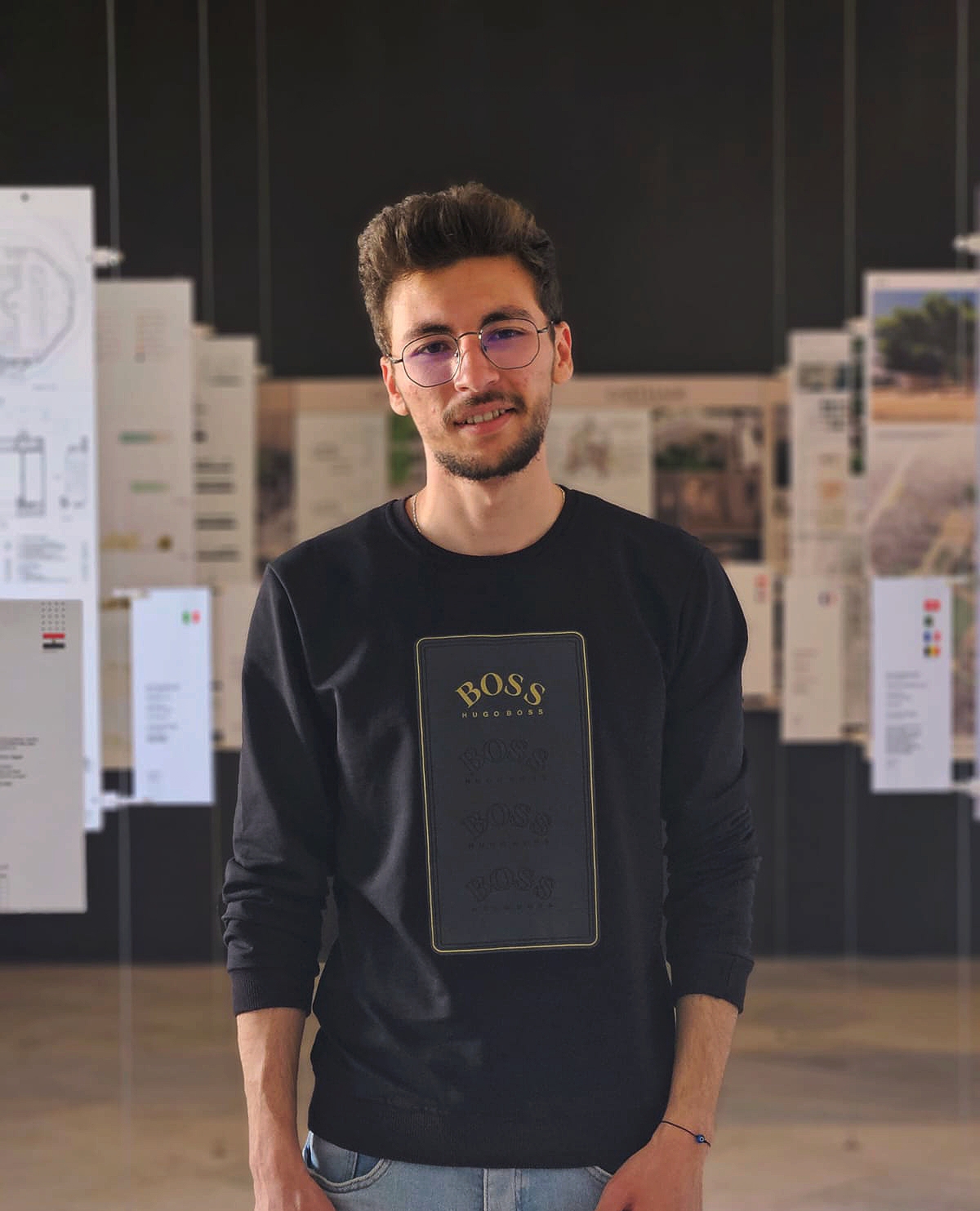
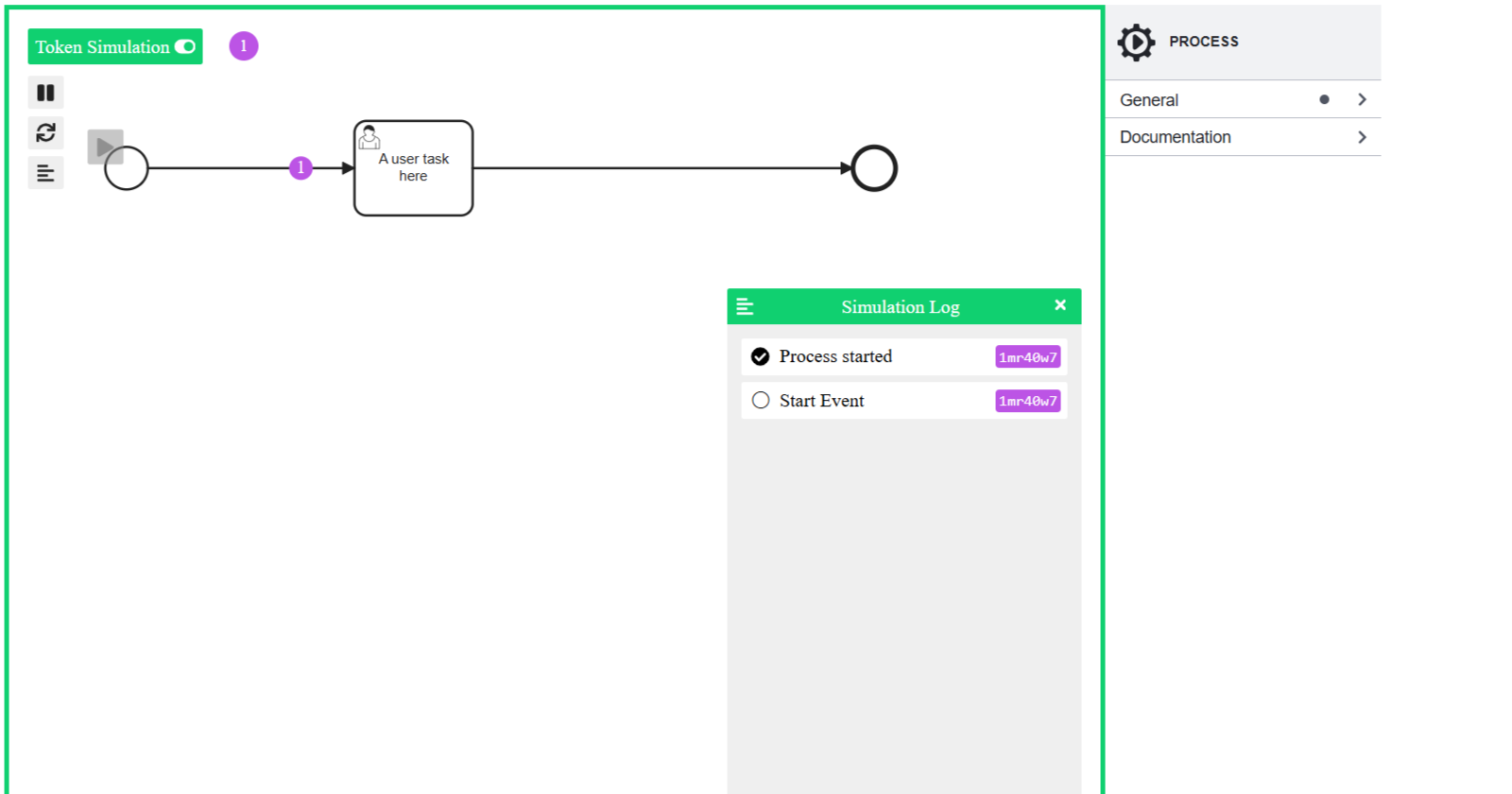
After setting up our Angular project, integrating bpmn-js and setting a Modeler, we will dive deeper and add additional modules to our modeler. For those who missed the previous steps, click on the link below to check out the full blog : Integrating bpmn-js with Angular : step-by-step guide.
You can check what changes we are making and features we are adding to our modeler by adding bpmn-js-token-simulation and bpmn-js-properties-panel.
1-Integrating bpmn-js-token-simulation
Now we are ready to start ! First you need to install the library using the following command :
npm install bpmn-js-token-simulation
After installing the library we need to import the TokenSimulationModule in our bpmn-modeler component :
import TokenSimulationModule from 'bpmn-js-token-simulation';
You may encounter an error : Could not find a declaration file for module 'bpmn-js-token-simulation'. This occurs because bpmn-js-token-simulation package may not come with its own TypeScript type declarations (.d.ts
files), which TypeScript relies on for type checking.
To resolve this issue we need to go to tsconfig.json file, "compilerOptions" and change the property "strict" to false.
"strict": false,
This works because it tells TypeScript to relax some of its checks, including the requirement for type declarations. The "skipLibCheck": true skips checking the types of existing declarations, but it doesn't help if the declarations are missing entirely which is our case.
Now our library is well imported ! let's add additional modules to our Modeler :
constructor() {
this.bpmnJS = new Modeler({
container: this.bpmnModelerRef?.nativeElement,
additionalModules: [
TokenSimulationModule,
],
})
}
The final step is to add the corresponding css to the angular.json file.
Go to angular.json
and look for "architect", then "build" and edit the "styles in the "options". You need to add some lines so it looks like this:
"styles": [
"src/styles.css",
"node_modules/bpmn-js/dist/assets/bpmn-font/css/bpmn.css",
"node_modules/bpmn-js/dist/assets/diagram-js.css",
"node_modules/bpmn-js-token-simulation/assets/css/bpmn-js-token-simulation.css"
],
Now save and run the following command in your terminal :
ng serve
Congratulations ! You can now simulate tokens in your browser on bpmn diagrams ! You should be able to start the simulation by visiting this url http://localhost:4200
and clicking on the corresponding button.
2-Integrating bpmn-js-properties-panel
Let's start by installing the required dependencies. Add the properties panel together with @bpmn-io/properties-panel.
npm install --save bpmn-js-properties-panel @bpmn-io/properties-panel
In our case, the bpmn-js-properties-panel
package provides the logic and functionality for the properties panel, while the @bpmn-io/properties-panel
package handles the styling. Separating the styling into a different package provides more flexibility to users who might want to customize or replace the default styles without altering the core functionality.
The next step is to import the necessary modules from the installed packages. :
import {
BpmnPropertiesPanelModule,
BpmnPropertiesProviderModule
} from 'bpmn-js-properties-panel';
Let's add the propertiesRef
: This reference is for the container where the properties panel will be rendered. We simply need to add :
@ViewChild('propertiesRef', { static: true }) private propertiesRef: ElementRef | undefined;
We also need to add the additional modules to our bpmnJS :
constructor() {
this.bpmnJS = new Modeler({
container: this.bpmnModelerRef?.nativeElement,
additionalModules: [
TokenSimulationModule,
BpmnPropertiesPanelModule,
BpmnPropertiesProviderModule,
],
propertiesPanel: {
parent: this.propertiesRef
},
})
}
Next we have to Set Up the HTML Template to include the new reference :
<div class="container">
<div #bpmnModelerRef class="bpmnModeler-container"></div>
<div #propertiesRef class="properties-container"></div>
</div>
And the final step is to attach the properties panel to the properties container after the content is initialized.
ngAfterContentInit(): void {
this.bpmnJS.attachTo(this.bpmnModelerRef.nativeElement);
const propertiesPanel = this.bpmnJS.get('propertiesPanel') as any;
propertiesPanel.attachTo(this.propertiesRef.nativeElement);
this.importDiagram(this.xml);
}
"If you're wondering why we used 'as any', here's why :
And our component is ready ! If you run your application you can see the properties panel !
To achieve the standard appearance for the properties panel, we need to include the corresponding CSS file. This can be done by adding the following lines to the styles
array in your angular.json
file:
"styles": [
"src/styles.css",
"node_modules/bpmn-js/dist/assets/bpmn-font/css/bpmn.css",
"node_modules/bpmn-js/dist/assets/diagram-js.css",
"node_modules/bpmn-js-token-simulation/assets/css/bpmn-js-token-simulation.css",
"node_modules/@bpmn-io/properties-panel/dist/assets/properties-panel.css"
],
Congratulations ! You can notice the changes on the properties panel and get the final results as expected !
3-Conclusion
By following the steps outlined in this guide, you can successfully integrate the bpmn-js-token-simulation
and bpmn-js-properties-panel
modules into your Angular application. If you encounter any issues or have questions, feel free to leave a comment below. Happy coding !
Subscribe to my newsletter
Read articles from Hatem Siala directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
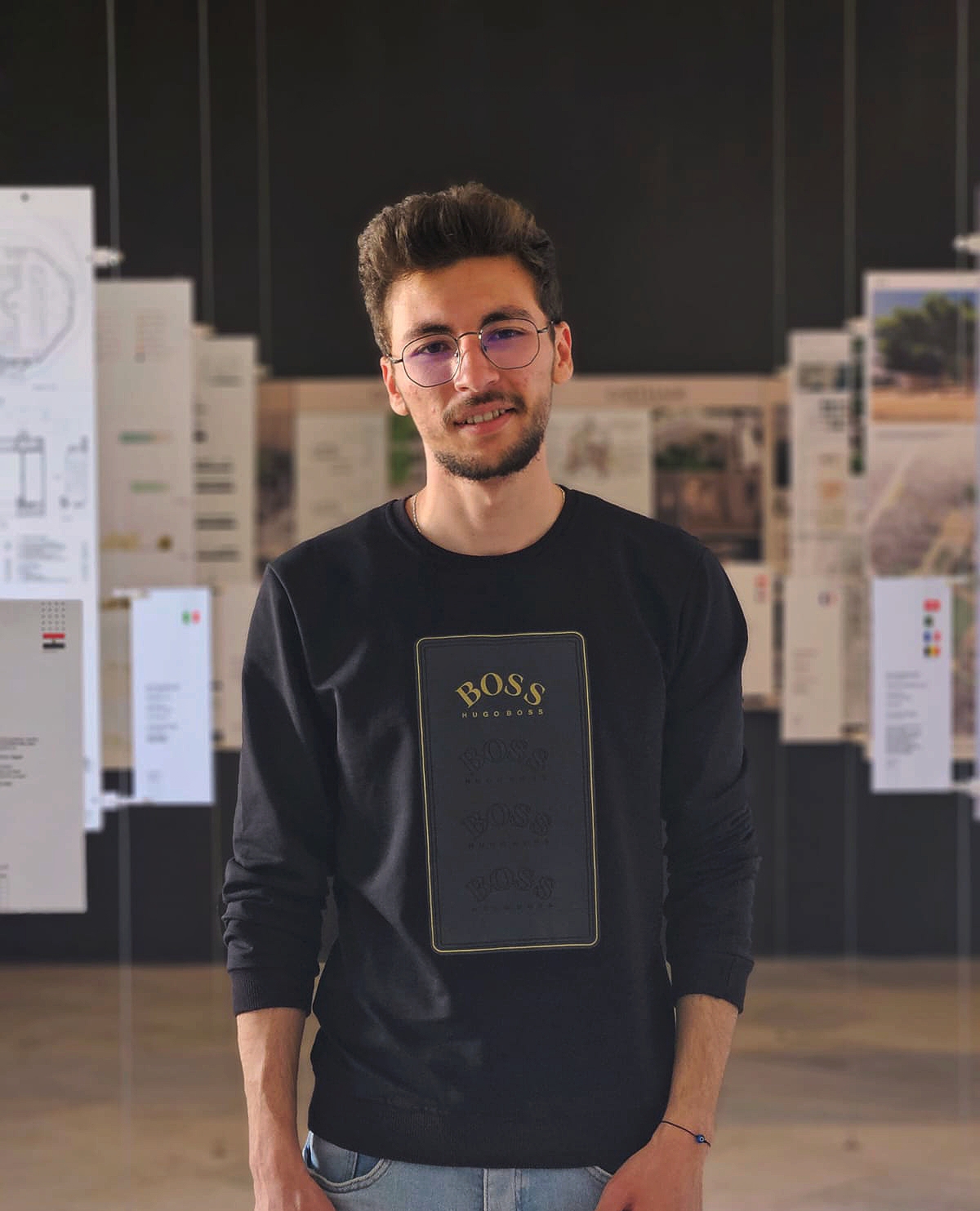