Day 13: Shell Scripting Challenge - Directory Backup with Rotation

Table of contents
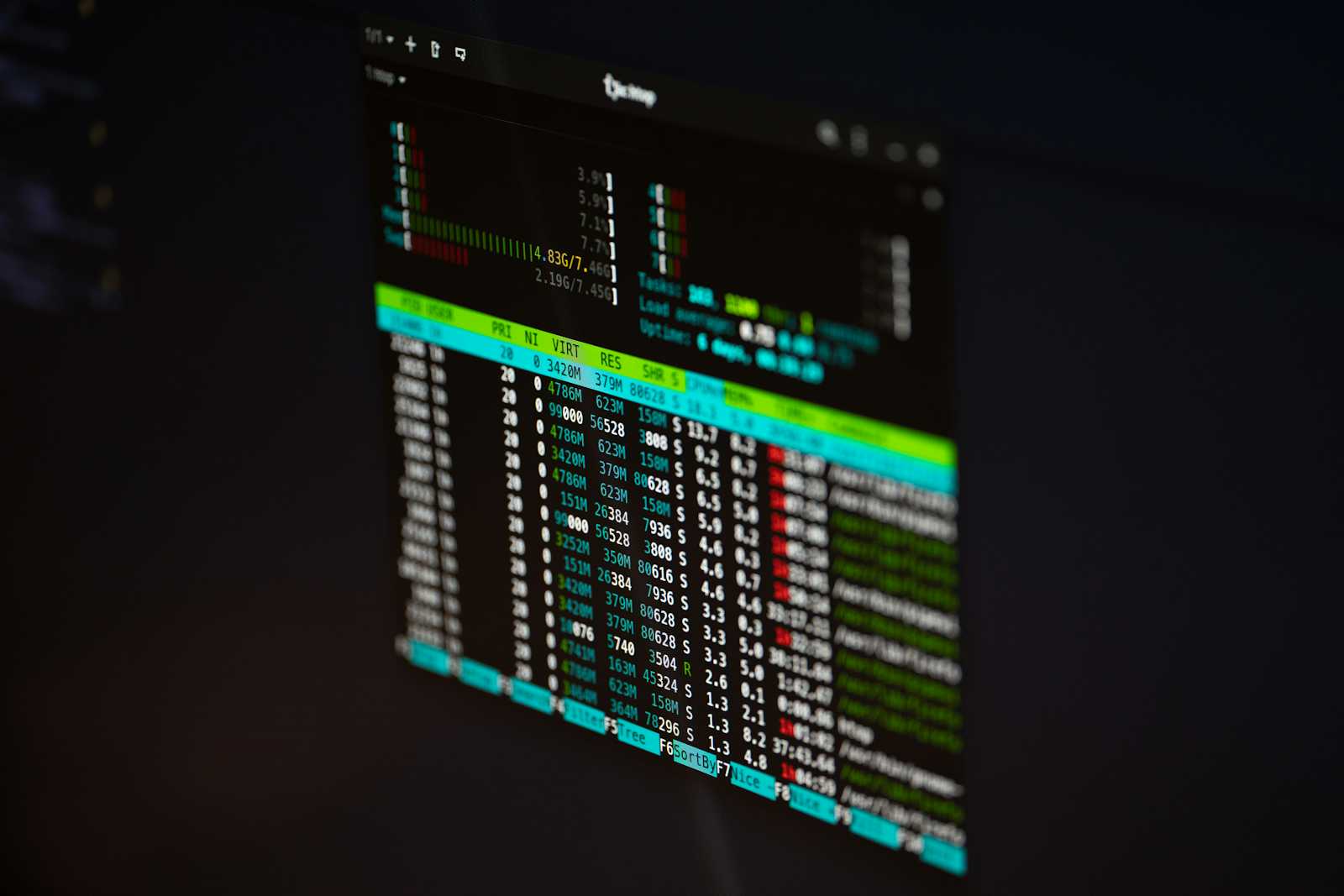
Welcome to Day 13 of DevOps adventurous journey. Today we will do a challenge - which is to perform some task using Shell Scripting so that we are more confident in using Shell Scripting in industry with real life scenarios.
Challenge
Our today's challenge is as follows:
Create a bash script that takes a directory path as a command-line argument and performs a backup of the directory. The script should create timestamped backup folders and copy all the files from the specified directory into the backup folder.
Additionally, the script should implement a rotation mechanism to keep only the last 3 backups. This means that if there are more than 3 backup folders, the oldest backup folders should be removed to ensure only the most recent backups are retained.
Script Breakdown
Hereβs the bash script to accomplish this:
#!/bin/bash
#This function will display how to use shell script while we run it.
function display_usage {
echo "Usage: $0 <path to the directory whose backup needs to be done>"
}
#This condition is used to check if valid arguments are being provided by user or not.
if [ $# -eq 0 ] || [ ! -d "$1" ]; then
echo "Please enter a valid argument"
display_usage
exit 1
fi
#Take source directory as argument from user
source_dir=$1
#Create backup function
function create_backup {
local time_stamp=$(date +"%Y%m%d_%H%M%S") #This is the timestamp which is modified
#Format in which backup file should be created
local backup_file="${source_dir}/backup_${time_stamp}.tar.gz"
#Zipping the file to occupy less space
tar -czf $backup_file $source_dir
#Checking if the above tar command was successful
if [ $? -eq 0 ]; then
echo "Backup created successfully"
else
echo "Backup not created"
exit 1
fi
}
#Create a function to keep only last 3 backups
function perform_rotation {
#List all backups
local backups=($(ls -t "${source_dir}/backup_"*))
echo "Current Backups: ${backups[@]}"
#Condition to check if backups are more than 3
if [ "${#backups[@]}" -gt 3 ]; then
#Listing backups and slicing them to get all backups except latest 3
local backups_to_remove=("${backups[@]:3}")
#Loop to remove all backups except the latest 3 backups
for backup in "${backups_to_remove[@]}"; do
rm -rf "$backup"
#To check if remove command ran successfully
if [ $? -eq 0 ]; then
echo "Removed old backup: $backup"
else
echo "Failed to remove old backup: $backup"
fi
done
fi
}
#Calling both the functions
create_backup
perform_rotation
How It Works
Input Validation: The script checks if a valid number of arguments are provided and if it is a directory path.
Timestamp Creation: A timestamp is generated for the backup folder name, ensuring each backup is uniquely identifiable.
Backup Creation: A new backup file is created each time with the timestamp, and that file is zipped as well to optimize space utilization.
Backup Rotation: The script lists all backup directories, sorts them by date, and removes the oldest ones if there are more than three.
Benefits
Automated backups : Saves time and reduces the risk of human error in manual backups. You can also schedule backups to run at specific intervals using cron jobs.
Efficient Storage Management: With rotation, old backups are automatically deleted or archived after a certain period. This keeps the storage usage under control and ensures you donβt run out of space.
Disaster Recovery: In case of data loss, corruption, or other disasters, you can quickly restore from the latest or an earlier backup, minimizing downtime and data loss.
Conclusion π
Implementing a backup with rotation using a shell script is a powerful, cost-effective, and customizable solution for managing your data. By automating the backup process and incorporating a rotation mechanism, you ensure that your data is consistently protected while efficiently managing storage space. This approach not only simplifies disaster recovery but also empowers you with full control over your backup strategy.
Subscribe to my newsletter
Read articles from Tanmaya Arora directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Tanmaya Arora
Tanmaya Arora
Hey there! π π Welcome to my DevOps journey! π I'm Tanmaya Arora, an enthusiastic DevOps Engineer. Currently, on a learning adventure, I'm here to share my journey and Blogs about DevOps. I believe in fostering a culture of resilience, transparency, and shared responsibility. Embracing agility and flexibility, in this adventure let's grow together in this vibrant DevOps space. Join me in transforming software delivery through collaboration, innovation, and excellence! ππ§π‘ π Connect with me for friendly chats, group discussions, shared experiences and learning moments.