A Deep Dive into Styled-Components
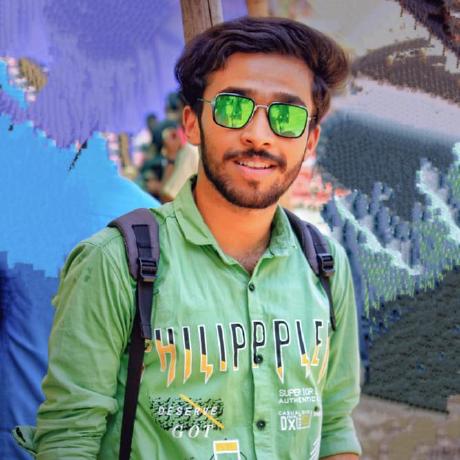
What is Styled-Components?
Styled-Components is a library that allows you to write CSS directly within your JavaScript files. It leverages tagged template literals to style your components, enabling a more dynamic and component-driven styling approach. This means you can style components based on their props, states, or any other JavaScript logic, making your styling more modular and reusable.
Why Use Styled-Components?
Here are some compelling reasons to consider using Styled-Components in your projects:
- Scoped Styles
One of the biggest challenges in styling applications is managing CSS conflicts. Styled-Components automatically scopes styles to the component, preventing any unintended side effects from global styles. This leads to cleaner and more maintainable code.
2. Dynamic Styling
Styled-Components allows you to apply styles dynamically based on props. For instance, you can change the color of a button based on whether it’s active or inactive, making your components more versatile.
const Button = styled.button`
background: ${props => (props.primary ? 'blue' : 'gray')};
color: white;
padding: 10px;
border: none;
border-radius: 5px;
`;
Theming Support
Styled-Components provides built-in support for theming, making it easy to implement design systems. You can define a theme and apply it globally, ensuring a consistent look and feel across your application.
const theme = {
primaryColor: 'blue',
secondaryColor: 'gray',
};
const ThemedButton = styled.button`
background: ${props => props.theme.primaryColor};
color: white;
`;
Easier Maintenance
By co-locating your styles with your components, Styled-Components makes it easier to maintain your code. When you need to change the styling, you know exactly where to look.
Getting Started with Styled-Components
Let’s walk through a simple example of using Styled-Components in a React application.
Step 1: Install Styled-Components
First, you need to install the library. You can do this using npm or yarn:
npm install styled-components
Step 2: Create a Styled Component
Now, let’s create a simple styled button component:
import styled from 'styled-components'; const StyledButton = styled.button` background: blue; color: white; padding: 10px 20px; border: none; border-radius: 5px; cursor: pointer; &:hover { background: darkblue; } `;
Step 3: Use the Styled Component
You can now use the
StyledButton
component in your application:import React from 'react'; import { ThemeProvider } from 'styled-components'; const theme = { primaryColor: 'blue', }; const App = () => { return ( <ThemeProvider theme={theme}> <StyledButton>Click Me!</StyledButton> </ThemeProvider> ); }; export default App;
Conclusion
Styled-Components offers a powerful way to style your React applications, providing scoped styles, dynamic styling capabilities, and excellent theming support. If you’re looking to enhance your styling approach and maintain cleaner code, give Styled-Components a try in your next project!
Subscribe to my newsletter
Read articles from Supratim Dey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
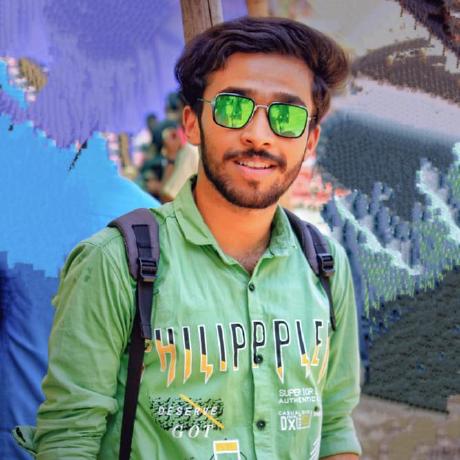