Optimizing Web Performance in NextJs: Beyond Lazy Loading and Code Splitting ๐
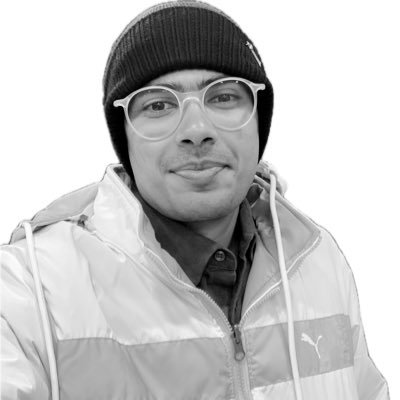
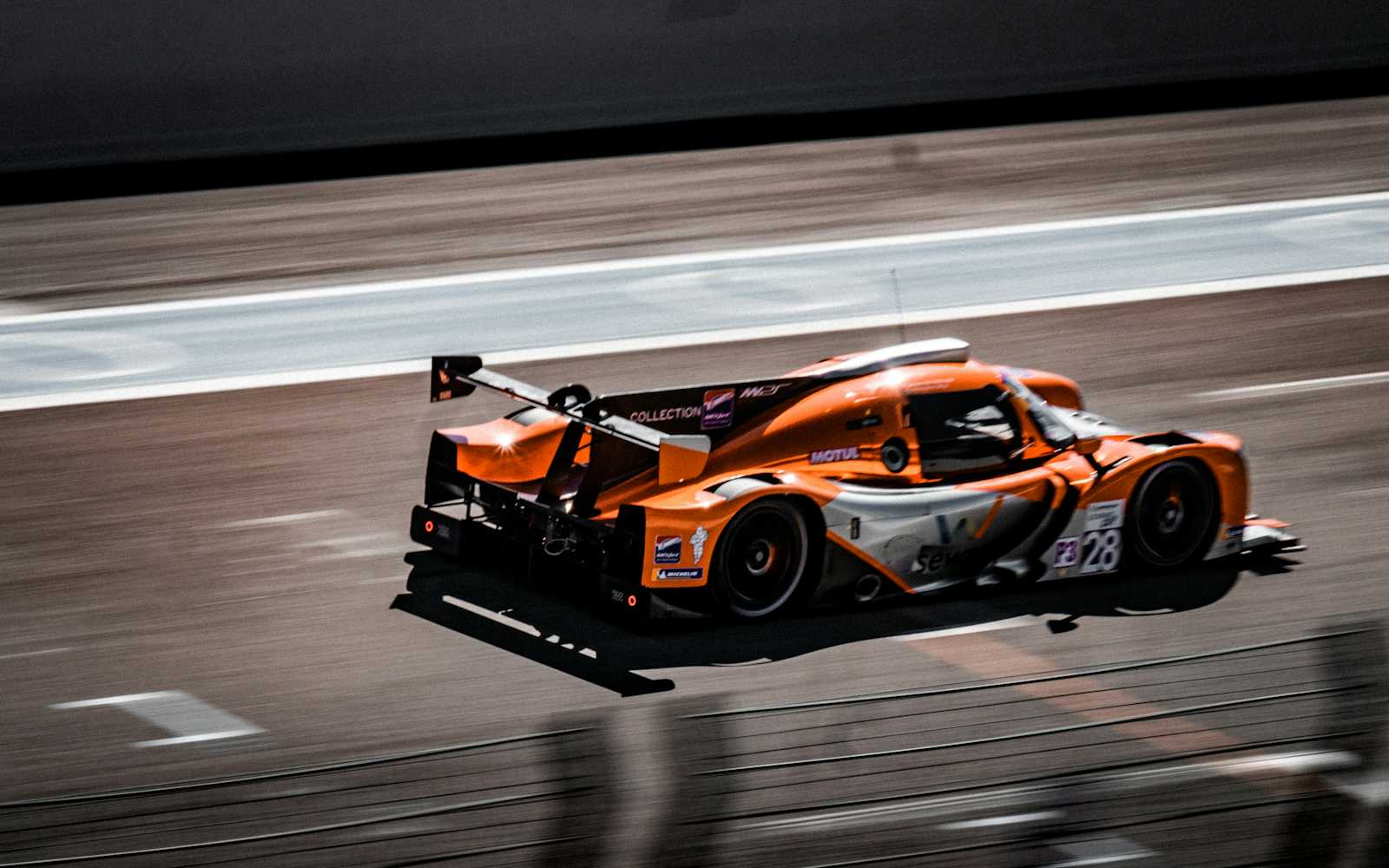
In the world of web development, optimizing performance is crucial for delivering a fast and smooth user experience. While lazy loading and code splitting are popular techniques for improving web application performance, there are more advanced strategies you can employ. In this blog post, we'll explore caching strategies, prefetching, and rendering optimizations to take your web performance to the next level. We'll use Next.js code samples to illustrate these concepts.
Caching Strategies ๐๏ธ
Caching is a powerful technique to reduce server load and speed up the delivery of content to users. By storing copies of resources locally, you can avoid unnecessary network requests and significantly improve load times.
Example: Implementing Caching in Next.js ๐ป
Next.js provides built-in support for caching through its API routes and static file serving. Here's how you can implement basic caching:
// app/api/data/route.js
import { NextResponse } from 'next/server';
export async function GET() {
const data = await fetch('https://api.example.com/data');
const jsonData = await data.json();
const response = NextResponse.json(jsonData);
response.headers.set('Cache-Control', 'public, s-maxage=86400, stale-while-revalidate=59');
return response;
}
Key Takeaways:
Cache-Control Header: Use the
Cache-Control
header to specify caching policies.s-maxage: Sets the max age for shared caches (e.g., CDNs).
stale-while-revalidate: Allows serving stale content while revalidating in the background.
Prefetching ๐๏ธ
Prefetching involves loading resources in advance so that they are readily available when needed. This technique can significantly reduce perceived latency and improve the overall user experience.
Example: Link Prefetching in Next.js ๐
Next.js makes it easy to prefetch pages with its built-in Link
component:
// app/page.js
import Link from 'next/link';
export default function HomePage() {
return (
<div>
<h1>Welcome to My App</h1>
<Link href="/about" prefetch={true}>
<a>About Us</a>
</Link>
</div>
);
}
Key Takeaways:
Link Prefetching: Automatically prefetch linked pages in the background.
Reduced Latency: Improves navigation speed by preloading resources.
Rendering Optimizations ๐ผ๏ธ
Rendering optimizations involve techniques to improve how content is rendered on the client side, reducing the time it takes for users to see meaningful content.
Example: Static Site Generation (SSG) in Next.js โก๏ธ
Static Site Generation (SSG) allows you to generate HTML at build time, which can be served quickly without needing to render on the server for every request.
// app/about/page.js
export const revalidate = 60; // Revalidate every 60 seconds
export async function getStaticProps() {
const res = await fetch('https://api.example.com/about');
const data = await res.json();
return {
props: {
data,
},
};
}
export default function AboutPage({ data }) {
return (
<div>
<h1>About Us</h1>
<p>{data.description}</p>
</div>
);
}
Key Takeaways:
Static Site Generation: Pre-renders pages at build time for fast delivery.
Revalidation: Periodically revalidate static content to keep it up-to-date.
Combining Techniques for Optimal Performance ๐ก
By combining caching, prefetching, and rendering optimizations, you can achieve significant performance improvements. Here's an example of how you might integrate all these techniques in a Next.js project:
// app/api/data/route.js
import { NextResponse } from 'next/server';
export async function GET() {
const data = await fetch('https://api.example.com/data');
const jsonData = await data.json();
const response = NextResponse.json(jsonData);
response.headers.set('Cache-Control', 'public, s-maxage=86400, stale-while-revalidate=59');
return response;
}
// app/page.js
import Link from 'next/link';
import { getStaticProps as getHomePageData } from './home';
export const revalidate = 60; // Revalidate every 60 seconds
export async function getStaticProps() {
return getHomePageData();
}
export default function HomePage({ data }) {
return (
<div>
<h1>Welcome to My App</h1>
<p>{data.message}</p>
<Link href="/about" prefetch={true}>
<a>About Us</a>
</Link>
</div>
);
}
// app/about/page.js
export const revalidate = 60; // Revalidate every 60 seconds
export async function getStaticProps() {
const res = await fetch('https://api.example.com/about');
const data = await res.json();
return {
props: {
data,
},
};
}
export default function AboutPage({ data }) {
return (
<div>
<h1>About Us</h1>
<p>{data.description}</p>
</div>
);
}
Optimizing web performance involves more than just lazy loading and code splitting. By implementing advanced techniques like caching, prefetching, and rendering optimizations, you can create a faster and more efficient web application. Next.js provides powerful tools and built-in features to help you achieve these optimizations with ease.
Happy coding! ๐
Feel free to ask questions or share your own performance optimization tips in the comments below! ๐ฌ
Subscribe to my newsletter
Read articles from Vivek directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
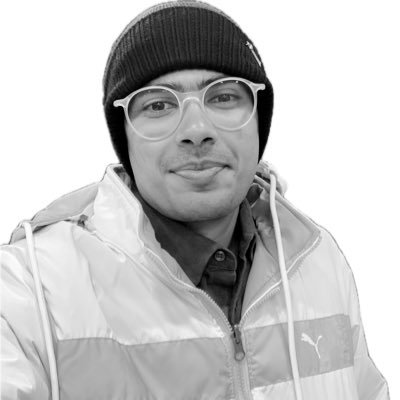
Vivek
Vivek
Curious Full Stack Developer wanting to try hands on โจ๏ธ new technologies and frameworks. More leaning towards React these days - Next, Blitz, Remix ๐จ๐ปโ๐ป