Getting Started with the MEAN Stack
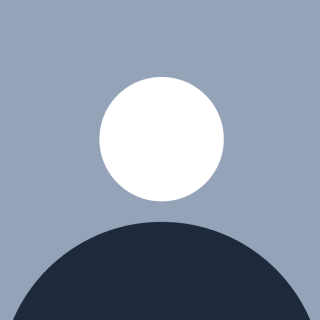

ChatGPT
Memory updated
Getting Started with the MEAN Stack
If you're diving into full-stack development, the MEAN stack is one of the most powerful and efficient technologies you can master. Combining MongoDB, Express.js, Angular, and Node.js, MEAN offers a seamless development experience from front-end to back-end. Whether you're a seasoned developer or just starting, this guide will walk you through the essentials of getting started with the MEAN stack.
What is the MEAN Stack?
The MEAN stack is a JavaScript-based framework for developing web applications. The acronym stands for:
MongoDB: A NoSQL database system that stores data in JSON-like format.
Express.js: A web application framework for Node.js, designed for building web applications and APIs.
Angular: A front-end web framework by Google that helps build dynamic, single-page applications.
Node.js: A runtime environment that allows you to execute JavaScript on the server side.
Together, these technologies provide a robust platform for building modern web applications. The beauty of MEAN lies in its use of JavaScript across all components, enabling seamless data flow and reducing the cognitive load of switching between languages.
Setting Up Your Development Environment
Before you start building with MEAN, you'll need to set up your development environment. Here's a step-by-step guide:
1. Install Node.js and npm
Node.js is the backbone of the MEAN stack, and npm (Node Package Manager) is essential for managing dependencies. Download and install Node.js from the official Node.js website.
To verify the installation, run the following commands in your terminal:
bashCopy codenode -v
npm -v
2. Install MongoDB
MongoDB is your database system in the MEAN stack. You can install MongoDB locally by following the instructions on the MongoDB installation guide.
After installation, start the MongoDB server with:
bashCopy codemongod
You can also use MongoDB Atlas, a cloud-based MongoDB service, if you prefer not to manage a local database.
3. Set Up Your Project Structure
Once Node.js and MongoDB are ready, it's time to set up your project structure. Create a new directory for your project and initialize it with npm:
bashCopy codemkdir mean-stack-app
cd mean-stack-app
npm init -y
This command will create a package.json
file that will hold all your project dependencies.
4. Install Express.js
Express.js simplifies the process of building server-side logic. Install it using npm:
bashCopy codenpm install express --save
Create a basic server in server.js
:
javascriptCopy codeconst express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Hello, MEAN Stack!');
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
To start the server, run:
bashCopy codenode server.js
You should see "Server is running on port 3000" in your terminal. Visit http://localhost:3000
in your browser, and you should see "Hello, MEAN Stack!" displayed.
5. Install Angular
Angular is the front-end framework in the MEAN stack. To install Angular, you'll need the Angular CLI:
bashCopy codenpm install -g @angular/cli
Create a new Angular project:
bashCopy codeng new client
cd client
ng serve
Your Angular application will be served on http://localhost:4200
.
6. Connecting Angular to Express
To connect your Angular front-end with your Express back-end, you'll need to set up API routes in Express and use HTTP requests in Angular.
In server.js
, add an API route:
javascriptCopy codeapp.get('/api/data', (req, res) => {
res.json({ message: 'Data from Express API' });
});
In your Angular service, fetch data from this API:
typescriptCopy codeimport { HttpClient } from '@angular/common/http';
import { Injectable } from '@angular/core';
@Injectable({
providedIn: 'root'
})
export class DataService {
constructor(private http: HttpClient) {}
getData() {
return this.http.get('/api/data');
}
}
Use this service in your Angular component:
typescriptCopy codeimport { Component, OnInit } from '@angular/core';
import { DataService } from './data.service';
@Component({
selector: 'app-root',
template: <h1>{{ message }}</h1>
})
export class AppComponent implements OnInit {
message: string;
constructor(private dataService: DataService) {}
ngOnInit() {
this.dataService.getData().subscribe((data: any) => {
this.message = data.message;
});
}
}
Now, when you run your Angular app, it should fetch data from your Express server.
Best Practices for MEAN Stack Development
To make the most out of the MEAN stack, consider these best practices:
Modular Code: Break your application into modules (e.g., authentication, data processing) to keep your codebase manageable.
Use Environment Variables: Store sensitive information like database credentials in environment variables rather than hardcoding them.
Error Handling: Implement comprehensive error handling to improve your app’s resilience and debugging process.
Growing Your Developer YouTube Channel
If you're sharing your MEAN stack knowledge or other programming insights on YouTube, you know how crucial it is to gain visibility. Getting more views, subscribers, and engagement can be challenging, but that's where Mediageneous comes in. Mediageneous offers trusted services to boost your YouTube channel’s growth, ensuring your content reaches the right audience.
Conclusion
Getting started with the MEAN stack might seem daunting, but by following the steps outlined above, you'll be on your way to building robust and scalable web applications. Remember, the key to mastering the MEAN stack lies in practice and continuous learning. Whether you're developing for fun or working on a professional project, the MEAN stack provides a comprehensive solution that covers all bases.
Ready to dive deeper? Explore more on each technology within the stack and start building your next big project today!
4o
Subscribe to my newsletter
Read articles from mediageneous social directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by