[Detailed Guide] Add and Delete Images in PowerPoint with Python

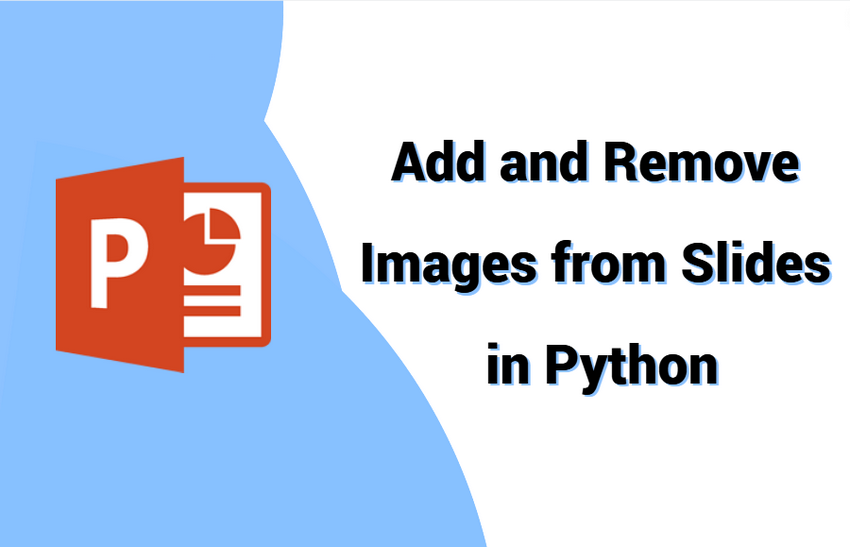
On many commercial and educational occasions, giving a presentation effectively shows your ideas and opinions. Whether preparing a sales pitch, an academic lecture, or a project update, you need to add or remove images from slides to keep your presentation engaging and up-to-date. This page will demonstrate how to add and delete images in PowerPont with Python, helping you maintain an attractive and updated presentation.
Prepare for Task
In today’s blog, to add and delete images in PowerPoint, we need to use Spire.Presentation for Python. It is a Python PowerPoint library that focuses on Office control. It contains almost all the features of Microsoft Office. By using it in Python, users can easily manage PowerPoint documents, including adding and removing images in PowerPoint slides.
You can install Spire.Presentation for Python from PyPI using the pip command below:
pip install Spire.Presentation
If you have already installed it and would like to upgrade it to the newest version, please use the following command:
pip install - upgrade Spire.Presenation
How to Add Images in PowerPoint Slides with Python
Although Spire.Presentation does not directly support adding images to PowerPoint documents, it provides an alternative solution. You can create a rectangle, set its size and position, and then embed a picture on the slide.
To give a better understanding of how Spire.Presentation imports a picture into a PowerPoint slide, here are the detailed steps for adding images in PowerPoint, and we have provided a code example for your reference.
Create a Presentation object and open a PowerPoint presentation using Presentation.LoadFromFile() method.
Get the slide to be operated with Presentation.Slides[] property.
Specify the file path of the image to be added.
Create a rectangle and set its position, and using Rectangle.FromLTRB() method to set the size.
Insert an image in a PowerPoint slide by calling Slide.Shapes.AppendEmbedImageByPath() method. Then set its fill type according to your demand.
Save the resulting file with Presentation.SaveToFile() method and release resources.
Here is a code example of adding an image to the fourth slide:
from spire.presentation.common import *
import math
from spire.presentation import *
# Create an object for the Presentation class
presentation = Presentation()
# Load a PowerPoint document from the disk
presentation.LoadFromFile("presentation.pptx")
# Get the fourth slide of the presentation
slide = presentation.Slides[3]
# Specify the image file path
imageFile = "pic1.jpg"
# Create a rectangle and specify the position and size
left = math.trunc(presentation.SlideSize.Size.Width / float(2)) - 430
rect1 = RectangleF.FromLTRB (left, 100, 450 + left, 350)
# Insert an image in a PowerPoint slide and set the fill type to none
image = slide.Shapes.AppendEmbedImageByPath (ShapeType.Rectangle, imageFile, rect1)
image.Line.FillType = FillFormatType.none
# Save the resulting presentation to the file
presentation.SaveToFile("AddImageToSlide.pptx", FileFormat.Pptx2010)
# Release the resources
presentation.Dispose()
How to Delete an Image from PowerPoint with Python
When dealing with PowerPoint presentations, deleting an image from slides is a common need. It helps you delete images that are wrong or don't go with the overall style of the document. It also helps keep images up-to-date.
To delete an image from slides in Python, the most essential step is looping through shapes on the slide and checking if the shapes are images. Retrieve the index of images on the slide, then remove specified images using the Slide.Shapes.RemoveAt() method.
The part below shows how to delete an image from PowerPoint slides:
Create an object for the Presentation class and read the target document from a file using Presentation.LoadFromFile() method.
Create a list to store indexes of images.
Get a slide with Presentation.Slides[] property and iterate through shapes on the slide.
Check if shapes are pictures; if yes, record the index of those pictures in the list.
Delete an image from PowerPoint using Slide.Shapes.RemoveAt() method.
Store the document with Presentation.SaveToFile() method and release resources.
Here is a code example of removing the first and third images from the fifth slide:
from spire.presentation.common import *
from spire.presentation import *
# Create a Presentation object
ppt = Presentation()
# Load the PowerPoint document from the disk
ppt.LoadFromFile("presentation.pptx")
# Create a list to keep track of image indexes to delete
indexes = []
# Get the fifth slide
slide = ppt.Slides[4]
# Iterate through shapes on the slide
image_index = 0
for i in range(slide.Shapes.Count - 1, -1, -1):
# Check if shapes are pictures
if isinstance(slide.Shapes[i], SlidePicture):
image_index += 1
# Record indexes of the first and third images
if image_index in (1, 3):
indexes.append(i)
# Remove the first and third images from the slide
for index in indexes:
slide.Shapes.RemoveAt(index)
# Save to file
ppt.SaveToFile("Removepic_1st3rd.pptx", FileFormat.Pptx2013)
# Release the resources
ppt.Dispose()
How to Remove All Images from a PowerPoint Slide in Python
The page has covered how to remove specified images from a slide above, but there are some occasions when you need to delete all the images from a slide. Whether you are reformating a PowerPoint document or preparing a template for reuse, deleting each image from a slide can be a crucial step. In this section, we’ll guide you through the process of using Python to remove all images from a PowerPoint slide.
Instructions to remove all images from a slide:
InstaInstantiate a Presentation object and use Presentation.LoadFromFile() method to load a PowerPoint presentation.
Retrieve a slide with Presentation.Slides[] property and loop through shapes on the slide.
Check if these shapes are images; if yes, remove these images by calling Shapes.RemoveAt() method.
Save the modified presentation to the disk using Presentation.SaveToFile() method and release resources.
Here is a code example of removing each image from the 5th slide:
from spire.presentation.common import *
from spire.presentation import *
# Create a Presentation object
ppt = Presentation()
# Load the PowerPoint document to be modified from the disk
ppt.LoadFromFile("presentation.pptx")
# Get the fifth slide
slide = ppt.Slides[4]
# Loop through shapes on the slide
for i in range(slide.Shapes.Count - 1, -1, -1):
# Check if those shapes are images
if isinstance(slide.Shapes[i], SlidePicture):
# Remove pictures on the fifth slide
slide.Shapes.RemoveAt(i)
# Save to file
ppt.SaveToFile("Removepic_Slide.pptx", FileFormat.Pptx2013)
# Release the resources
ppt.Dispose()
How to Remove Images from All Slides in PoweiPoint with Python
After learning how to remove all images from a single slide, you might need to extend this action across an entire PowerPoint presentation. The steps to remove images from all slides in PowerPoint are similar to the steps to delete images from a single slide. However, the former requires an additional loop to iterate through all slides before removing the images from each slide.
But don’t worry, this section will walk you through how to remove images from all slides in PowerPoint, providing a guide and a code example.
Steps to remove images from PowerPoint slides in Python:
Create an object for the Presentation class and use Presentation.LoadFromFile() method to open a presentation.
Loop through all slides and all shapes on each slide.
Check if these shapes are images, then remove images from all slides in PowerPoint using Shapes.RemoveAt() method.
Save the resulting document to the disk with Presentation.SaveToFile() method and release the resources.
from spire.presentation.common import *
from spire.presentation import *
# Create a Presentation object
ppt = Presentation()
# Load the PowerPoint document to be modified from the disk
ppt.LoadFromFile("presentation.pptx")
# Loop through all slides
for slide_index in range(len(ppt.Slides)):
slide = ppt.Slides[slide_index]
# Loop through shapes on the slide
for i in range(slide.Shapes.Count - 1, -1, -1):
# Check if those shapes are images
if isinstance(slide.Shapes[i], SlidePicture):
# Remove pictures on the slide
slide.Shapes.RemoveAt(i)
# Save to file
ppt.SaveToFile("Removepic_AllSlide.pptx", FileFormat.Pptx2013)
# Release the resources
ppt.Dispose()
The Conclusion
This article provides a comprehensive guide on how to add images in PowerPoint, how to delete an image from PowerPoint slides, remove all images from a slide, and remove images from all slides in PowerPoint. Each instruction includes detailed steps and a code example for reference, helping you understand how to accomplish these tasks. We hope you find it useful!
Subscribe to my newsletter
Read articles from Casie Liu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
