π Mastering Middleware in Node.js and Express.js
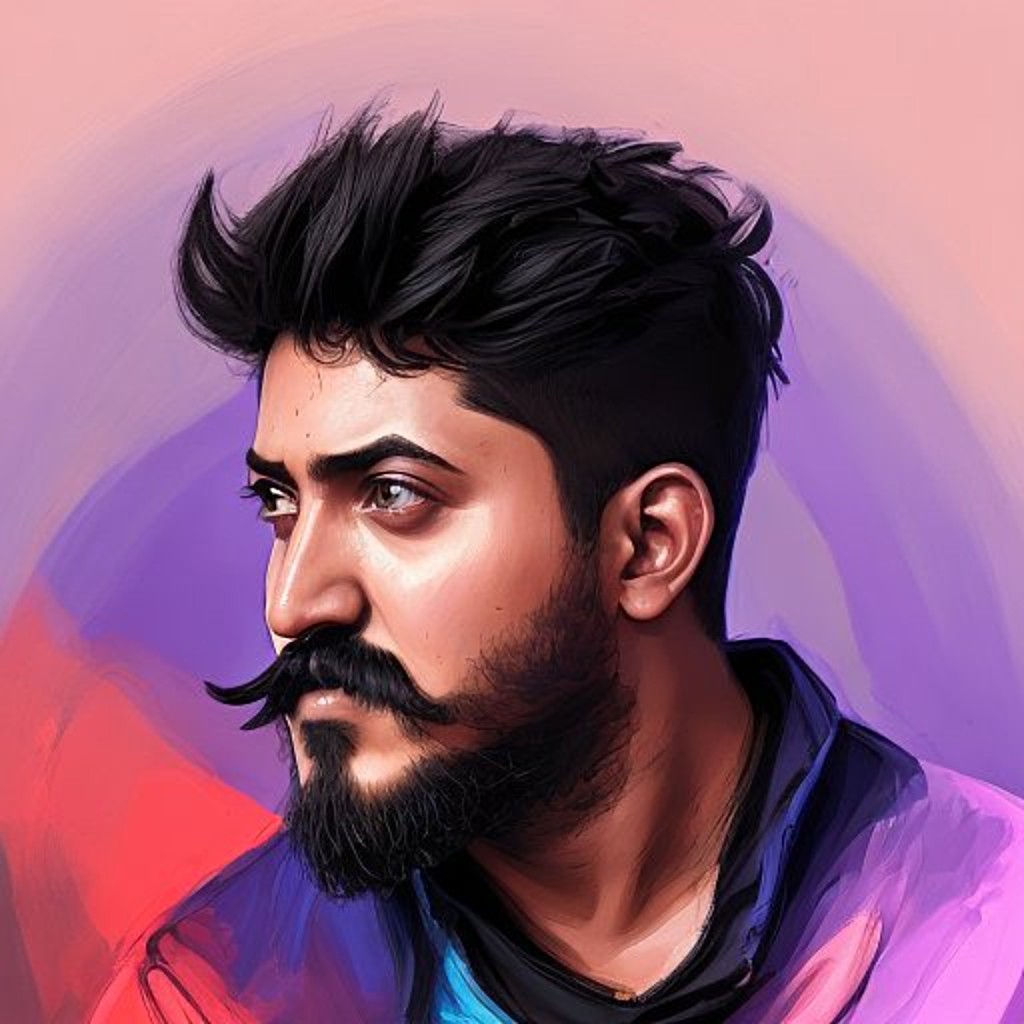
Today, we'll explore the essential concept of middleware in Node.js and Express.js. Middleware plays a crucial role in managing requests and responses in web applications, enabling you to build efficient, scalable, and maintainable systems. This guide will provide you with a comprehensive understanding of middleware and how to implement it effectively in your projects.
π What is Middleware?
Middleware functions are those magical pieces of code that execute during the lifecycle of a request to a server. They have access to the request object (req
), the response object (res
), and the next middleware function in the applicationβs request-response cycle. Middleware can:
Execute code
Modify the request and response objects
End the request-response cycle
Call the next middleware function in the stack
By the end of this post, you'll be equipped with the knowledge to utilize middleware in both simple and complex scenarios, enhancing your development skills.
π Setting Up Your Node.js Project
Let's start by setting up a basic Node.js project with Express.js.
Create a new directory for your project:
mkdir middleware-demo cd middleware-demo
Initialize a new Node.js project:
npm init -y
Install Express.js:
npm install express
Create a main application file
app.js
:const express = require('express'); const app = express(); // Start the server const PORT = process.env.PORT || 3000; app.listen(PORT, () => { console.log(`Server is running on port ${PORT}`); });
π Types of Middleware
1. Application-Level Middleware
Application-level middleware is bound to an instance of the Express app using the app.use()
or app.METHOD()
functions.
// Application-level middleware
app.use((req, res, next) => {
console.log(`${req.method} request for '${req.url}'`);
next(); // Pass control to the next middleware function
});
2. Router-Level Middleware
Router-level middleware works the same way as application-level middleware but is bound to an instance of express.Router()
.
const router = express.Router();
// Router-level middleware
router.use((req, res, next) => {
console.log('Router-Level Middleware');
next();
});
router.get('/', (req, res) => {
res.send('Home Page');
});
app.use('/', router);
3. Error-Handling Middleware
Error-handling middleware is defined with four arguments: (err, req, res, next)
. This middleware is used to handle errors in the application.
// Error-handling middleware
app.use((err, req, res, next) => {
console.error(err.stack);
res.status(500).send('Something broke!');
});
4. Built-In Middleware
Express has several built-in middleware functions that are included out-of-the-box.
// Built-in middleware to serve static files
app.use(express.static('public'));
// Built-in middleware to parse JSON data
app.use(express.json());
// Built-in middleware to parse URL-encoded data
app.use(express.urlencoded({ extended: true }));
5. Third-Party Middleware
Third-party middleware is created by the community and can be installed from npm. Popular examples include morgan
and cookie-parser
.
const morgan = require('morgan');
const cookieParser = require('cookie-parser');
// Third-party middleware for logging
app.use(morgan('dev'));
// Third-party middleware for parsing cookies
app.use(cookieParser());
π Chaining Middleware
You can chain multiple middleware functions together. This is a powerful way to compose complex request handling logic. Each middleware function should call next()
to pass control to the next middleware.
Example:
app.use((req, res, next) => {
console.log('First middleware');
next();
});
app.use((req, res, next) => {
console.log('Second middleware');
next();
});
app.get('/', (req, res) => {
res.send('Hello, World!');
});
When a request is made to the server, it will pass through each middleware in the order they are defined.
Chaining Middleware in Routes
You can also use multiple middleware functions in a single route. This is useful for handling different tasks sequentially.
const middleware1 = (req, res, next) => {
console.log('Middleware 1');
next();
};
const middleware2 = (req, res, next) => {
console.log('Middleware 2');
next();
};
app.get('/some-path', middleware1, middleware2, (req, res) => {
res.json({ message: 'Sample message' });
});
Output when accessing /some-path
:
Middleware 1
Middleware 2
Response:
{
"message": "Sample message"
}
π Real-World Example: User Authentication
Let's create a real-world example where we build a simple Express.js application for user authentication.
Step-by-Step Implementation
Set up the Express.js application:
const express = require('express'); const app = express(); const morgan = require('morgan'); const cookieParser = require('cookie-parser'); // Middleware to log requests app.use(morgan('dev')); // Middleware to parse JSON data app.use(express.json()); // Middleware to parse cookies app.use(cookieParser()); // Middleware to log request details const requestLogger = (req, res, next) => { console.log(`${req.method} request for '${req.url}'`); next(); }; app.use(requestLogger); // Middleware to authenticate users const authenticateUser = (req, res, next) => { const token = req.headers['authorization']; if (token === 'valid-token') { next(); // User is authenticated, proceed to the next middleware } else { res.status(401).json({ message: 'Unauthorized' }); } }; // Error-handling middleware const errorHandler = (err, req, res, next) => { console.error(err.stack); res.status(500).json({ message: 'Internal Server Error' }); }; // Route for user login (for simplicity, we just return a token) app.post('/login', (req, res) => { res.json({ token: 'valid-token' }); }); // Apply the authentication middleware to protected routes app.use('/protected', authenticateUser); // Protected route app.get('/protected/resource', (req, res) => { res.json({ message: 'This is a protected resource' }); }); // Apply the error handler middleware app.use(errorHandler); // Start the server const PORT = process.env.PORT || 3000; app.listen(PORT, () => { console.log(`Server is running on port ${PORT}`); });
Testing the Application
You can use tools like Postman or curl
to test your API.
Login Request:
curl -X POST http://localhost:3000/login
Response:
{ "token": "valid-token" }
Access Protected Resource (Unauthorized):
curl http://localhost:3000/protected/resource
Response:
{ "message": "Unauthorized" }
Access Protected Resource (Authorized):
curl -H "Authorization: valid-token" http://localhost:3000/protected/resource
Response:
{ "message": "This is a protected resource" }
π§βπ» Middleware in Pure Node.js
While Express.js simplifies middleware implementation, you can also create middleware-like functionality in pure Node.js applications. This involves manually handling the request and response objects.
Here's a basic example of middleware in pure Node.js:
const http = require('http');
// Simple middleware function
const loggerMiddleware = (req, res, next) => {
console.log(`${req.method} request for '${req.url}'`);
next();
};
// Function to handle requests
const requestHandler = (req, res) => {
// Chain middleware functions
const middlewares = [loggerMiddleware];
// Execute middleware functions sequentially
const next = () => {
if (middlewares.length > 0) {
const middleware = middlewares.shift();
middleware(req, res, next);
} else {
// Final request handler
res.end('Hello, World!');
}
};
next();
};
// Create and start the server
const server = http.createServer(requestHandler);
server.listen(3000, () => {
console.log('Server is running on port 3000');
});
π Conclusion
Middleware is a powerful feature in Node.js and Express.js that helps you manage the flow of requests and responses in your application. By understanding and utilizing different types of middleware, you can build more robust and maintainable web applications. Keep experimenting with different middleware functions and see how they can simplify your development process.
Happy coding! πβ¨
Subscribe to my newsletter
Read articles from Jobin Mathew directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
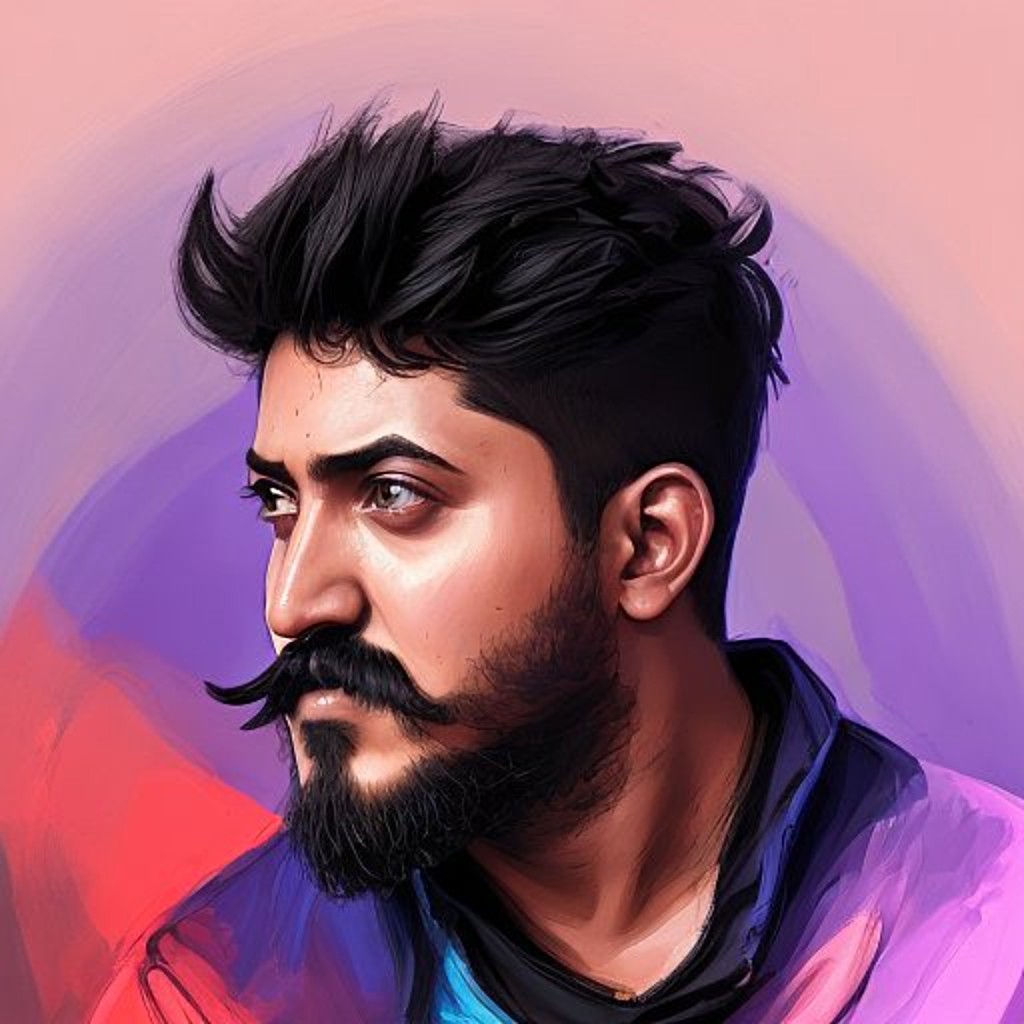
Jobin Mathew
Jobin Mathew
Hey there! I'm Jobin Mathew, a passionate software developer with a love for Node.js, AWS, SQL, and NoSQL databases. When I'm not working on exciting projects, you can find me exploring the latest in tech or sharing my coding adventures on my blog. Join me as I decode the world of software development, one line of code at a time!