Getting started with API Calls in Julia
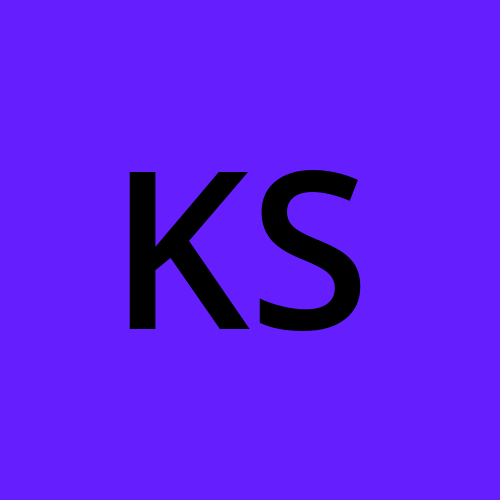
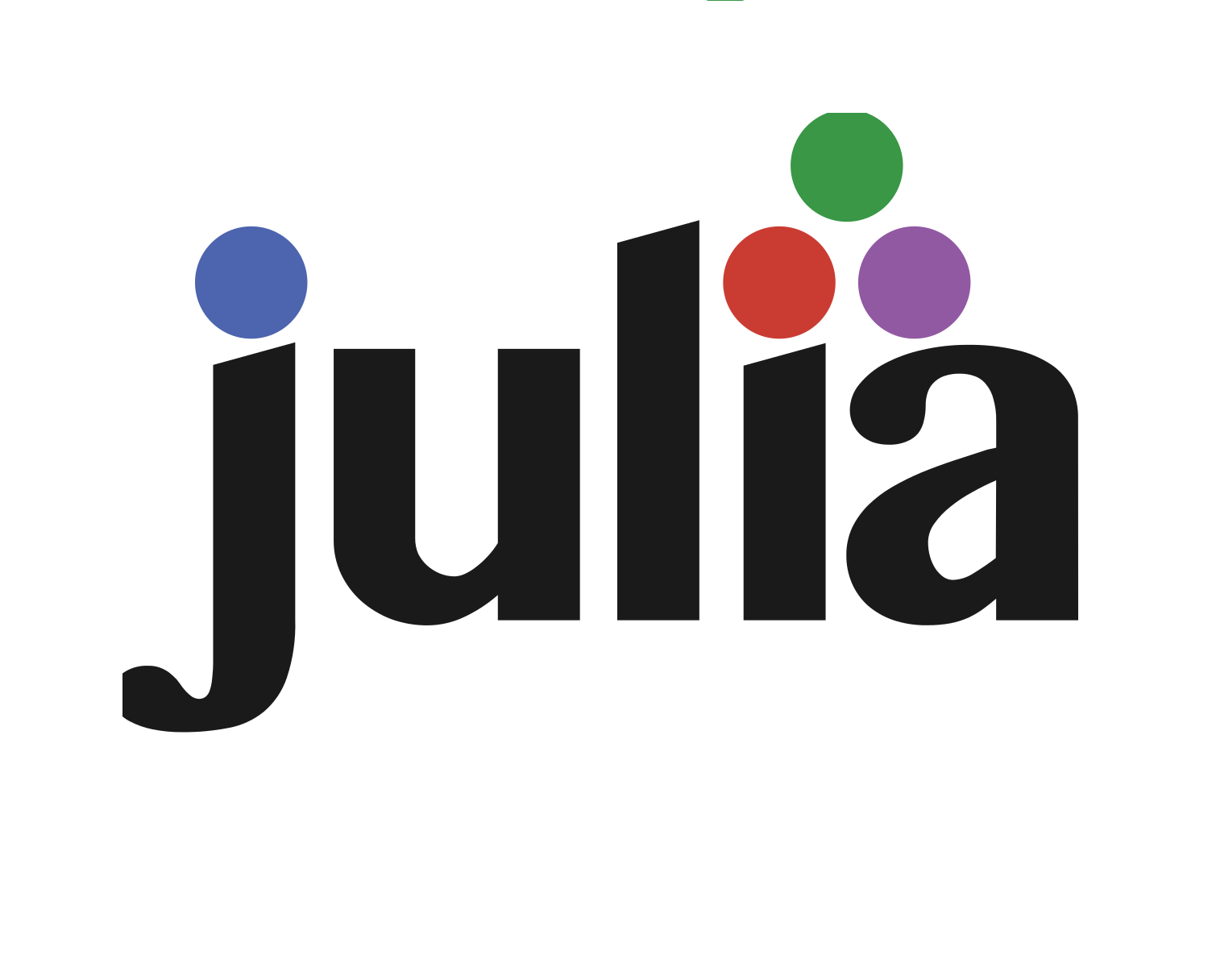
This program calls the get API which displays pokemon types w.r.t names using Julia
This article demonstrates how to make an HTTP GET request to an API, parse the response body as JSON, and extract relevant information from the parsed JSON object in Julia.
Getting Started
Julia installation is straightforward, whether using precompiled binaries or compiling from source. Download and install Julia by following the instructions at https://julialang.org/downloads/.
You can get started with Julia with their documentation
Install Packages
First, import two packages - HTTP
and JSON3
- that provides the functionality to make HTTP requests and parse JSON data, respectively.
Open the Julia command line, also known as the REPL (read-eval-print-loop)
To import packages we enter the following command
using Pkg
We use the Pkg.add()
function to install the HTTP
and JSON3
packages.
Pkg.add("HTTP")
Pkg.add("JSON3")
After installing the required packages, we then import the HTTP
and JSON3
packages using the using
keyword. This is necessary to use the functions and types provided by these packages in the code.
using HTTP
using JSON3
function GetCall(url)
try
response = HTTP.get(url)
return String(response.body)
catch e
return "Error occurred : $e"
end
end
Next, we define a function GetCall
that takes a URL as an argument and uses the HTTP
package's get()
function to send an HTTP GET request to the specified URL. The function returns the response body as a string.
print("Enter pokemon name ? \n\n")
pokemonName=readline()
Next, we prompt the user to input the name of a Pokemon by Julia readline()
function.
url="https://pokeapi.co/api/v2/pokemon/"*lowercase(pokemonName)
response = GetCall(url)
The program then constructs a URL by concatenating the user input with a base URL. The asterisk operator *
is used for concatenation in Julia. The GetCall
function is called with this URL as an argument, and the response body is stored in a variable called response
.
The response body is a JSON string that needs to be parsed into a Julia object to be used. This is done using the JSON3
package's read()
function, which takes a JSON string as input and returns a Julia object.
jobj = JSON3.read(response)
In this case, the parsed JSON object is stored in a variable called jobj
.The jobj
object contains information about the Pokemon, including its type(s), which is stored in an array called types
.
types=jobj.types
resultArray=[];
for i in types
push!(resultArray, i.type.name)
end
The program then creates an empty array called resultArray
, loops through each element in the types
array, and extracts the type name of each element, which is stored in the type.name
property. The program uses the push!()
function to add each type name to the resultArray
.
Note that the push!()
is a built-in function in Julia that is used to add an element to the end of an array. It modifies the original array resultArray
and returns the modified array as well. This means that resultArray
is updated with each iteration of the loop, and the final value of resultArray
after the loop completes will contain all the elements that were added using push!()
.
Finally, the program prints the elements of resultArray
as a comma-separated string, enclosed in square brackets using the println()
, repr()
and join()
function.
println()
is a function used to print output to the console with a newline character at the end.repr()
function, a built-in function that returns a string representation of an object in a form that can be evaluated to recreate the object.The
join()
function takes an array of strings and concatenates them into a single string, with a specified separator between each element.
println('[', join(repr.(resultArray),", "), ']')
Here, we are using these functions to print out the contents of an array called resultArray
as a comma-separated list within square brackets. Here's how it works:
repr.(resultArray)
creates a new array of strings by applying therepr()
function to each element ofresultArray
. The.
operator is used to indicate that this is a broadcast operation, meaning thatrepr()
is applied element-wise to the array.join(repr.(resultArray),", ")
concatenates the resulting strings with commas and spaces between them. For example, ifresultArray
contains the strings"fire"
,"water"
, and"grass"
, the resulting string would be"fire, water, grass"
.Finally, the
println()
function is used to print the concatenated string with square brackets around it. The entire expression is enclosed in parentheses to ensure that thejoin()
operation is performed before the string is printed.
Conclusion
Overall, this is a simple example of how to make an HTTP GET request to an API, parse the response body as JSON, and extract relevant information from the parsed JSON object using Julia. This functionality can be used to retrieve and process data from a wide variety of web APIs.
Subscribe to my newsletter
Read articles from Kanza Syed directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
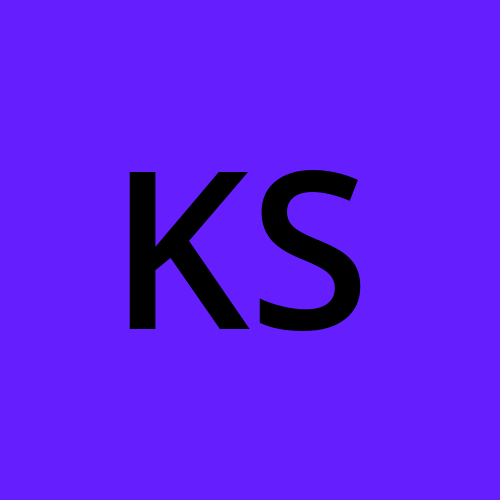
Kanza Syed
Kanza Syed
A learner :-)