The JavaScript Launchpad: Start Your Coding Adventure
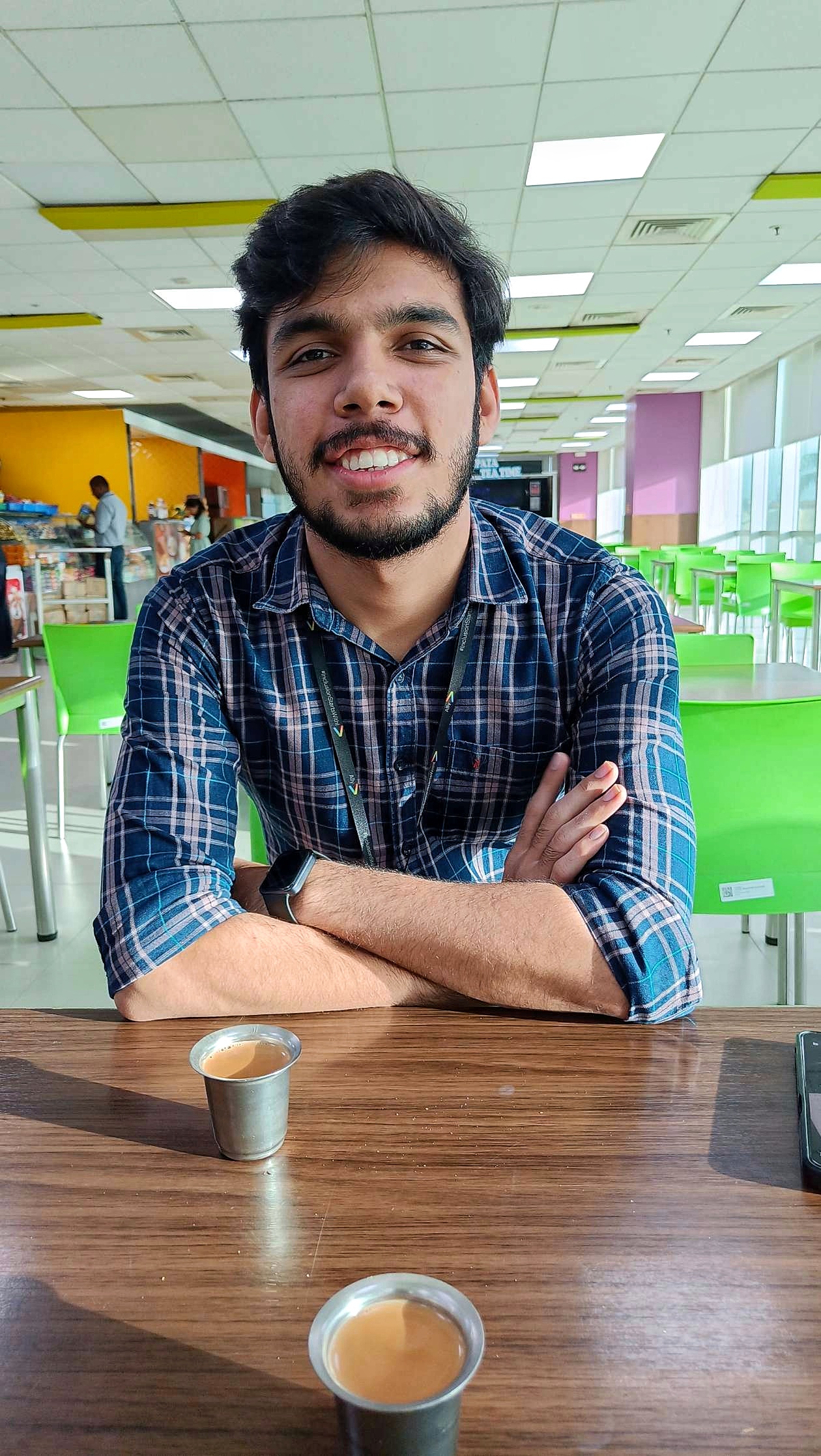
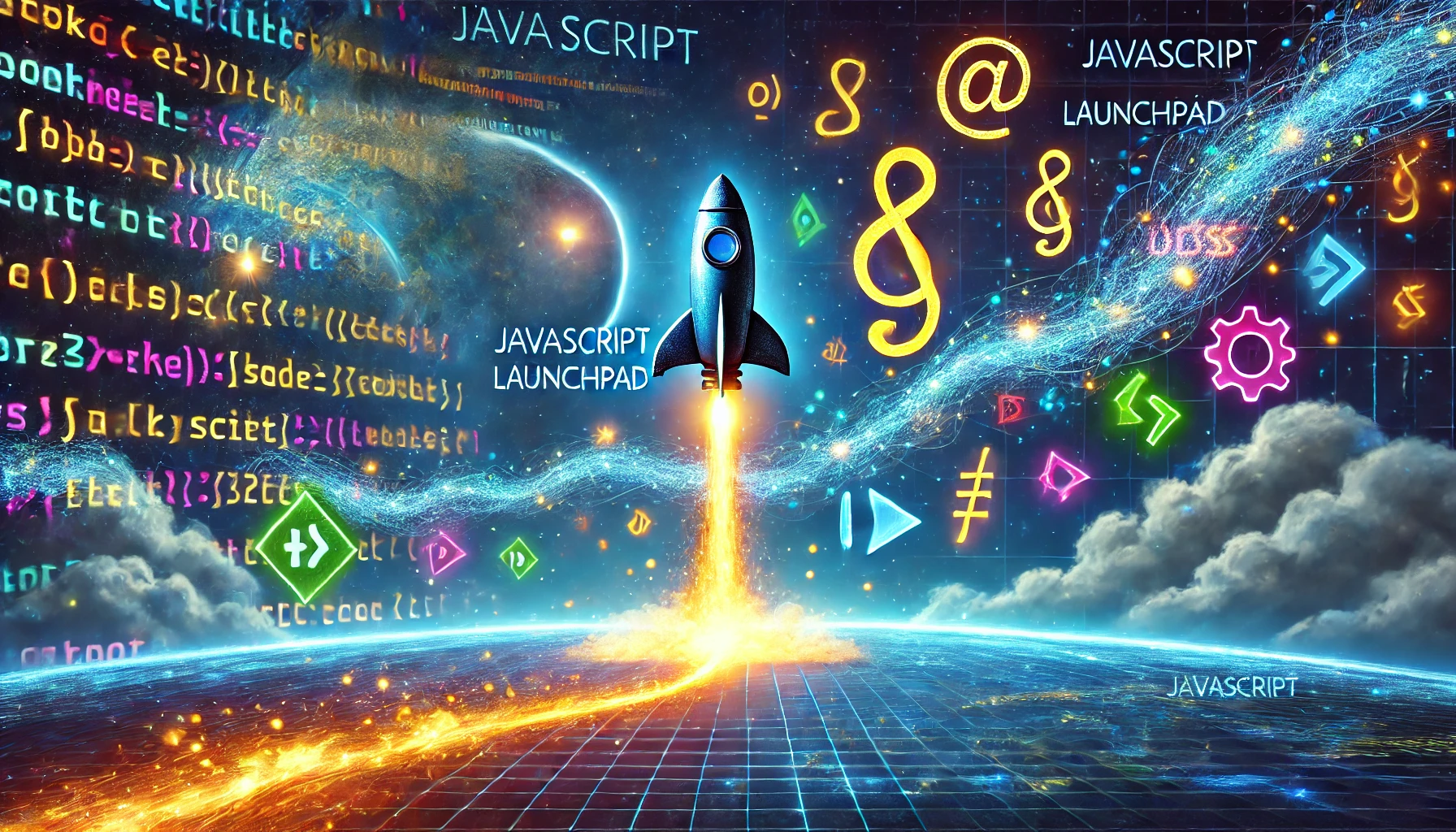
JavaScript has become a foundational element of contemporary web development. In this article, we'll explore the core principles and capabilities of JavaScript.
What is JavaScript?
JavaScript is a high-level, interpreted programming language, designed to bring web pages to life, transforming static HTML and CSS webpages into dynamic and interactive user experiences. Developed by Brendan Eich in 1995, JavaScript has evolved significantly. It is now used not only in web browsers but also in server-side development, mobile applications, and even hardware programming opening many possibilities for javascript developers.
Why JavaScript?
JavaScript plays a crucial role in web development by allowing the creation of interactive and dynamic web pages. Originally Javascript was designed to run in browsers only using the browsers JS engine. However, after the introduction of Node.js, it became easy to use JavaScript for both client-side and server-side development. Along with Node.js, it has other libraries and frameworks like React and Angular.
Being so popular among the developers JavaScript has strong community support and extensive resources. JavaScript’s optimized performance ability to handle asynchronous programming and capacity to build cross-platform applications make it a valuable tool across various technological fields.
How to start using Javascript?
Javascript code is written inside the <script> tag of HTML. The most important thing is the placement of this <script> tag. There are three ways to include JavaScript in your web page:
In <head> tag:
Example:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Using JavaScript</title> <script> document.querySelector(".heading").innerHTML = "Hello World!"; </script> </head> <body> <h1 class="heading"></h1> </body> </html>
However, this method is not advised because we know that the HTML page is read from top to bottom. As a result, the script will load before the HTML content, and attempting to access any nodes from the DOM will result in an error similar to the one seen below.
Uncaught
TypeError: Cannot set properties of null (setting 'innerHTML')
In end of the <body> tag:
Example:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Using JavaScript</title> </head> <body> <h1 class="heading"></h1> <script> document.querySelector(".heading").innerHTML = "Hello World!"; </script> </body> </html>
By using this method, we can ensure that JavaScript doesn't run until the web page's body has finished loading.
In an external JavaScript file (.js file) include it in the body using the <script> tag. This method works well for lengthy scripts because the code will still be understandable and simple.
<!-- index.html --> <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Using JavaScript</title> </head> <body> <h1 class="heading"></h1> <!-- script --> <script src="index.js"></script> </body> </html> // external javaScript file // index.js document.querySelector(".heading").innerHTML = "Hello World!";
Core Concepts of JavaScript
To get started with JavaScript, you need to understand its core concepts:
Variables
Variables store data that can be used and manipulated throughout our code. JavaScript is loosely typed we don’t need to declare the data type of the variable. In JavaScript, you can declare variables using var, let, and const. And, some rules need to be followed while naming the variables:
Variable names must start with a letter (a to z to A to Z). underscore(_) or dollar($) sign.
After the first letter, we can use digits (0 to 9).
JavaScript variables are case sensitive i.e. const a, and const A; are different variables.
var name; let total; //declaration of variable total = 100; //initialization of variable const x = 30;
Types of variables:
Global variables: Global variables are those variables that can be accessed anywhere throughout a program. They are declared outside a function.
let result = 500; function printResult(){ //function declaration console.log(result); // global variable result accessible inside the function } printResult(); // function call // Output on console // 500
Local Variable: Local variables are the variables that are declared inside a block or function. They have local scope and cannot be used outside that block or function.
let result = 500; function printResult(){ //function declaration let x = 0; //local variable console.log(x); } console.log(x); //if we try to access the local variable outside the scope it will result in an error: // Uncaught ReferenceError: x is not defined
Data Types
Data types define what data can be stored in a variable and tell the interpreter how we intend to use the data. Understanding these data types and their behaviors is fundamental to effective programming in JavaScript.
JavaScript supports various data types which are classified into two categories:
Primitive Data Types:-
Non-Primitive Data Types:-
Operators in JavaScript
Operators in JavaScript are special symbols used to perform operations on operands. JavaScript supports several types of operators:
Arithmetic Operators:
Assignment Operators:
Comparison Operators:
Logical Operators:
Bitwise Operators:
Type Operators:
Control Statements In JavaScript
Control statements in JavaScript are used to control the flow of a program based on certain conditions. If the condition is met then only that block of code will be executed otherwise it will be skipped. Javascript provides two types of control statements:
Conditional Statements: These statements are used for decision-making. A decision is made based on some condition and according to that decision, code is executed. There are two types of conditional statements in Javascript.
If-else Statements: The most common type of conditional statement is the if-else statement. If the condition provided in the if statement inside () is true then the if block gets executed otherwise else block gets executed. Three forms of if-else statements can be there.
if statement:
let isLoggedIn = true; if (isLoggedIn) { console.log("You are LoggedIn."); }
If-else statement:
let isLoggedIn = true; if (isLoggedIn) { console.log("You are LoggedIn."); } else{ console.log("You need to Login."); }
If-else if statement:
let score = 85; if (score >= 90) { console.log("Grade: A"); } else if (score >= 80) { console.log("Grade: B"); } else if (score >= 70) { console.log("Grade: C");
Switch Statement: Switch statement is used to execute one block of code based on the value of the expression. We take different cases for the value of expressions and execute the one that matches and if no case matches then the default case is executed.
let dayNumber = 5; switch (dayNumber) { case 1: console.log("Monday"); break; case 2: console.log("Tuesday"); break; case 3: console.log("Wednesday"); break; case 4: console.log("Thursday"); break; case 5: console.log("Friday"); break; case 6: console.log("Saturday"); break; case 7: console.log("Sunday"); break; default: console.log("Invalid day number"); }
Iterative Statements: Iterative statements allow us to execute a block of code multiple times until a condition is satisfied. We also call them loops. Four types of loops are provided in JavaScript:
‘for’ loop: For loop is used to execute a block of code a specific number of times. For loop is used when we know in advance how many times we want to execute the block of code.
Syntax:
for (initialization; condition; increment/decrement) { // Code to execute }
Example:
for (let i = 0; i < 5; i++) { console.log(i); } // Output: 0 1 2 3 4
‘for…in’ loop: It iterates over the keys of an object.
Syntax:
for (let key in object) { // Code to execute }
Example:
const person = {name: "Abhishek", age: 24, country: "India"}; for (let key in person) { console.log(key + ": " + person[key]); } // Output: // name: Abhishek // age: 24 // city: India
‘while’ loop: A while loop runs a block of code as long as the specified condition is true. It's particularly useful when the number of iterations needed is unknown.
Syntax:
while (condition) { // Code to execute }
Example:
let i = 0; while (i < 5) { console.log(i); i++; } // Output: 0 1 2 3 4
‘do..while’ loop: Do while loops work similarly to while loops but they guarantee that the code will execute at least once even if the condition is false.
Syntax:
do { // Code to execute } while (condition);
Example:
let i = 5; do { console.log(i); i++; } while (i < 5); // Output: 5
Continue and Break: In JavaScript ‘continue’ and ‘break’ are control flow statements that are used to alter the flow of loops and switch statements.
Continue: the continue statement skips an iteration of loops. It’s useful when we want to skip certain conditions in a loop without ending the loop.
Example:
for (let i = 0; i < 10; i++) { if (i % 2 === 0) { continue; // Skip the current iteration and continue next one } console.log(i); } // Output: 1 3 5 7 9
In the example, we can see that each time the ‘i’ is divisible by 2 continue statement is called and we skip the loop.
Break: A break statement is used to exit the loops and switch statements. If we place the break statement after a certain condition then when that condition is met the break statement will end the execution of the loop or switch statement and jump to the code immediately after them.
Example:
for (let i = 0; i < 10; i++) { if (i === 5) { break; // Exit the loop when i is 5 } console.log(i); } // Output: 0 1 2 3 4
Functions in JavaScript
In JavaScript, functions are fundamental building blocks that allow us to encapsulate reusable code into a single unit. They consist series of statements that perform a specific operation and return a result. Functions help break down large programs into smaller, more manageable parts, making the code easier to understand and more efficient.
Syntax:
// function without parameters
function functionName() {
// Code to execute
}
// function with parameters
function functionName(parameters) {
// Code to execute
}
Let us see a simple example of a function in JavaScript.
function greet(name) { //function declaration
return "Hello, " + name + "!";
}
console.log(greet("Abhishek")); //function call
// Output: Hello, Abhishek!
Here, we have a function greet which takes a parameter name and returns a message with the name provided in the argument in the function call.
Parameters and Arguments in Function: We often might get confused between these two when we start working with function for the first time. Parameters are defined in the function declaration while arguments are the actual values passed to the function during the function call.
function introduce(name, age) {
return My name is ${name} and I am ${age} years old.;
}
console.log(introduce("Abhishek", 24));
// Output: My name is Abhishek and I am 24 years old.
Here, ‘name’ and ‘age’ are the parameters declared with the function declaration, and ‘Abhishek’ and ‘24’ are the arguments passed during the function call.
Conclusion
JavaScript is a powerful and versatile language that plays a crucial role in modern web development. Its ability to create dynamic and interactive web applications, along with a thriving ecosystem and community, makes it an essential skill for any developer. Whether you're building the next big web application or simply adding interactivity to a static site, JavaScript is the tool that will help you achieve your goals. Embrace JavaScript, explore its features, and start creating amazing web experiences today!
Subscribe to my newsletter
Read articles from Abhishek Sadhwani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
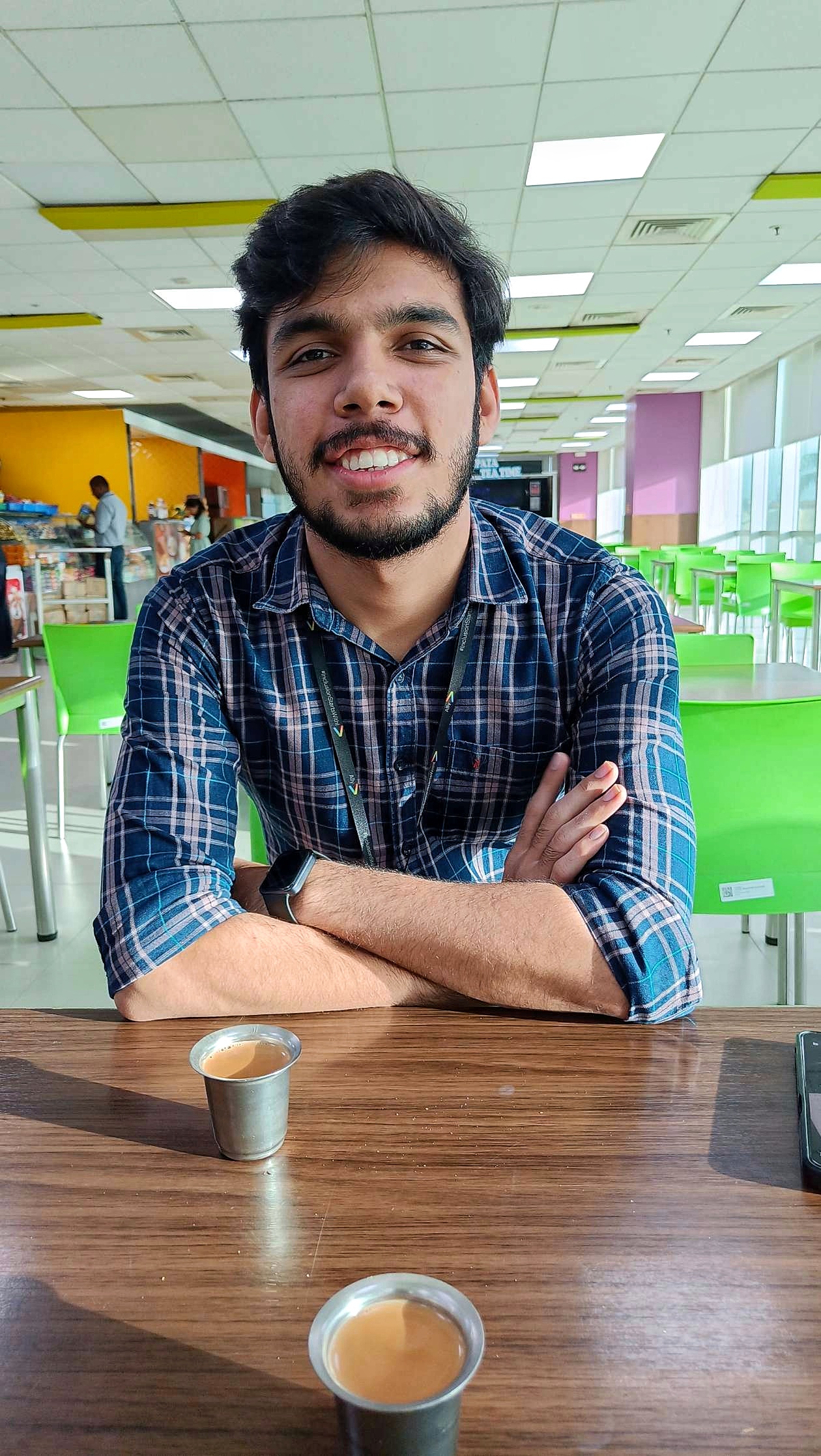