Why You Need AWS Lambda for Your Applications
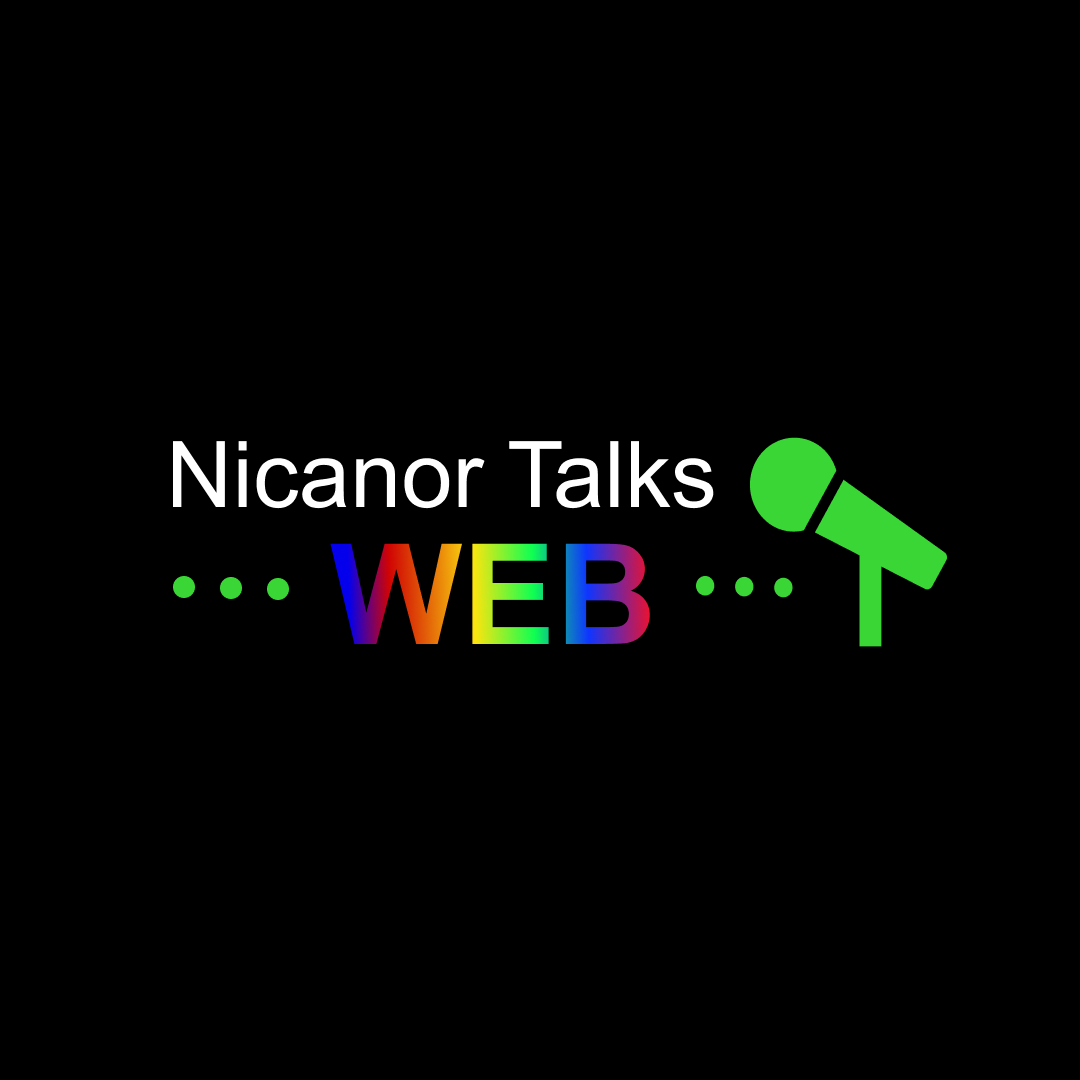
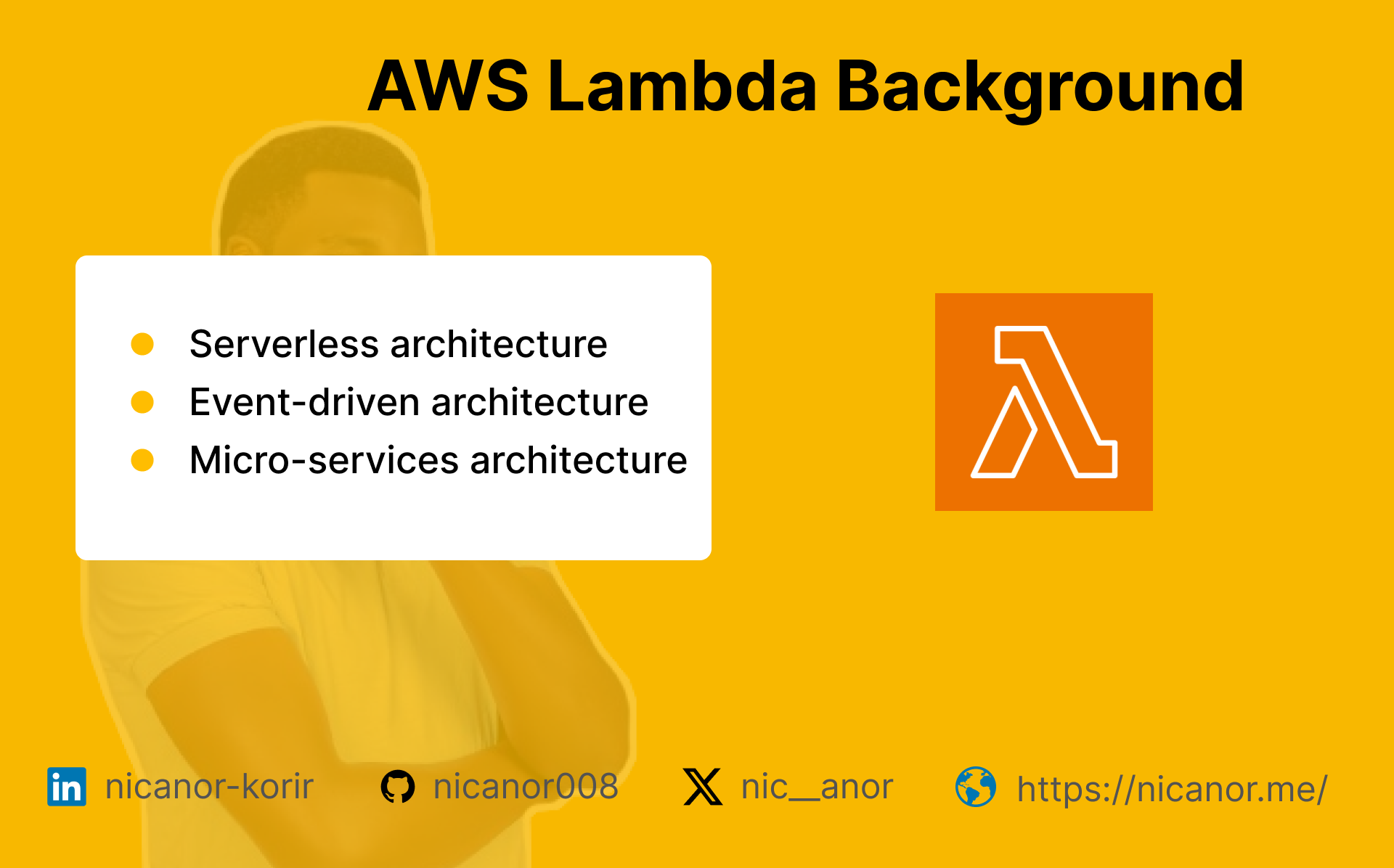
AWS Lambda is a serverless computing service that Amazon Web Services (AWS) provides. It allows you to run code without provisioning or managing servers. AWS Lambda executes your code only when needed and scales automatically, handling thousands of requests per second.
For in-depth, take a look at this article on RESTful Microservices with AWS Lambda, API Gateway and DynamoDB
The Theory Behind AWS Lambda
Serverless Architecture
The concept of serverless computing involves abstracting the server management away from developers. In a serverless architecture:
No Server Management: You don't need to worry about server provisioning, scaling, or maintenance.
Auto-scaling: The platform automatically scales up or down based on demand.
Pay-per-use: You only pay for the compute time your code uses, which can lead to significant cost savings.
Event-Driven Computing
AWS Lambda is designed for event-driven computing. It executes your code in response to various events, such as:
Changes to data in an Amazon S3 bucket
Updates to a DynamoDB table
HTTP requests via Amazon API Gateway
Scheduled events using Amazon CloudWatch Events
Microservices Architecture
Lambda functions fit perfectly into a microservices architecture, where applications are composed of small, independent services that communicate with each other. Each Lambda function can serve as an independent microservice, handling a specific piece of functionality.
Benefits of Using AWS Lambda
1. Cost Efficiency
With AWS Lambda, you only pay for the computing time of your functions. This pay-as-you-go model can lead to significant cost savings, especially for applications with variable workloads.
2. Scalability
AWS Lambda automatically scales your applications in response to incoming requests. Whether you have a few requests per day or thousands per second, Lambda adjusts the compute resources accordingly.
3. Reduced Operational Overhead
By eliminating the need to manage servers, AWS Lambda reduces operational overhead. Developers can focus on writing code rather than dealing with server maintenance, patching, and scaling.
4. Faster Time to Market
With AWS Lambda, you can quickly deploy and update your applications. The serverless architecture allows for rapid development and iteration, enabling faster time to market.
Use Cases for AWS Lambda
1. Real-Time File Processing
Lets say you have an application that needs to process images uploaded by users in real time. The processing involves resizing the images and generating thumbnails.
A reasonable solution would be to:
Use Amazon S3 to store the uploaded images.
Trigger an AWS Lambda function whenever a new image is uploaded to the S3 bucket.
The Lambda function processes the image, resizes it, and stores the thumbnails back in the S3 bucket.
and this way you would benefit from:
Automatic scaling to handle any number of uploads.
Pay only for the compute time used during image processing.
No need to manage or scale servers.
2. Data Transformation and ETL
Let's say you need to perform Extract, Transform, and Load (ETL) operations on data from various sources before storing it in a data warehouse.
A solution would be to use AWS Lambda to create ETL functions that extract data from different sources, transform it according to your business logic, and load it into Amazon Redshift or another data warehouse.
3. Building RESTful APIs
This is very common occurrences for most of developers. let's say you want to build a scalable RESTful API for your application without managing the underlying infrastructure.
You could:
Use Amazon API Gateway to expose your RESTful endpoints.
Implement the API logic using AWS Lambda functions.
This way helps:
Auto-scaling API endpoints.
Pay only for the API requests and Lambda compute time.
Simplified API management with API Gateway.
4. Scheduled Tasks and Automation
Scenario: You need to run scheduled tasks, such as cleaning up old data from a database or sending out periodic reports.
Solution:
- Use Amazon CloudWatch Events to trigger AWS Lambda functions on a schedule (e.g., daily, hourly).
Benefits:
Automated task execution without managing servers.
Scalability to handle variable workloads.
Cost efficiency by paying only for the execution time.
Example: Building a Serverless Image Processing Pipeline
Let's walk through a practical example of building a serverless image processing pipeline using AWS Lambda.
Step 1: Setting Up S3 Buckets
Create an S3 bucket for uploads:
Go to the AWS Management Console.
Navigate to S3 and create a new bucket named
image-uploads
.
Create an S3 bucket for processed images:
- Create another bucket named
processed-images
.
- Create another bucket named
Step 2: Creating the Lambda Function
Create a new Lambda function:
Go to the AWS Lambda console.
Click on "Create function."
Choose "Author from scratch."
Provide a function name (e.g.,
ImageProcessor
) and selectPython
as the runtime.
Write the Lambda function code:
- Use the following code to process the images:
const AWS = require('aws-sdk');
const Jimp = require('jimp'); // Using Jimp for image processing
const s3 = new AWS.S3();
exports.handler = async (event, context) => {
try {
const bucket = event.Records[0].s3.bucket.name;
const key = event.Records[0].s3.object.key;
// Download the image from S3
const response = await s3.getObject({ Bucket: bucket, Key: key }).promise();
const image = await Jimp.read(response.Body);
// Process the image (e.g., resize)
await image.resize(128, 128);
const buffer = new Buffer();
await image.quality(80).write(buffer); // Setting JPEG quality at 80%
// Upload the processed image to the processed-images bucket
await s3.putObject({ Bucket: 'processed-images', Key: key, Body: buffer, ContentType: 'image/jpeg' }).promise();
return {
statusCode: 200,
body: JSON.stringify('Image processed successfully!'),
};
} catch (error) {
console.error(error);
return {
statusCode: 500,
body: JSON.stringify('Error processing image'),
};
}
};
Step 3: Setting Up S3 Event Notifications
Configure event notifications for the uploads bucket:
Go to the
image-uploads
bucket in the S3 console.Click on "Properties" and then "Event notifications."
Add a new event notification for
ObjectCreated
events and set the destination to theImageProcessor
Lambda function.
Step 4: Testing the Setup
Upload an image to the
image-uploads
bucket:- Go to the S3 console, select the
image-uploads
bucket, and upload an image.
- Go to the S3 console, select the
Verify the processed image:
- Check the
processed-images
bucket to see if the resized image appears.
- Check the
AWS Lambda offers a powerful and flexible way to build scalable, cost-efficient applications without managing servers. By leveraging Lambda's serverless architecture, you can focus on writing code and implementing business logic while AWS handles the infrastructure. Whether you're processing images, building APIs, or automating tasks, AWS Lambda provides the tools you need to build modern, event-driven applications.
Subscribe to my newsletter
Read articles from Nicanor Talks Web directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
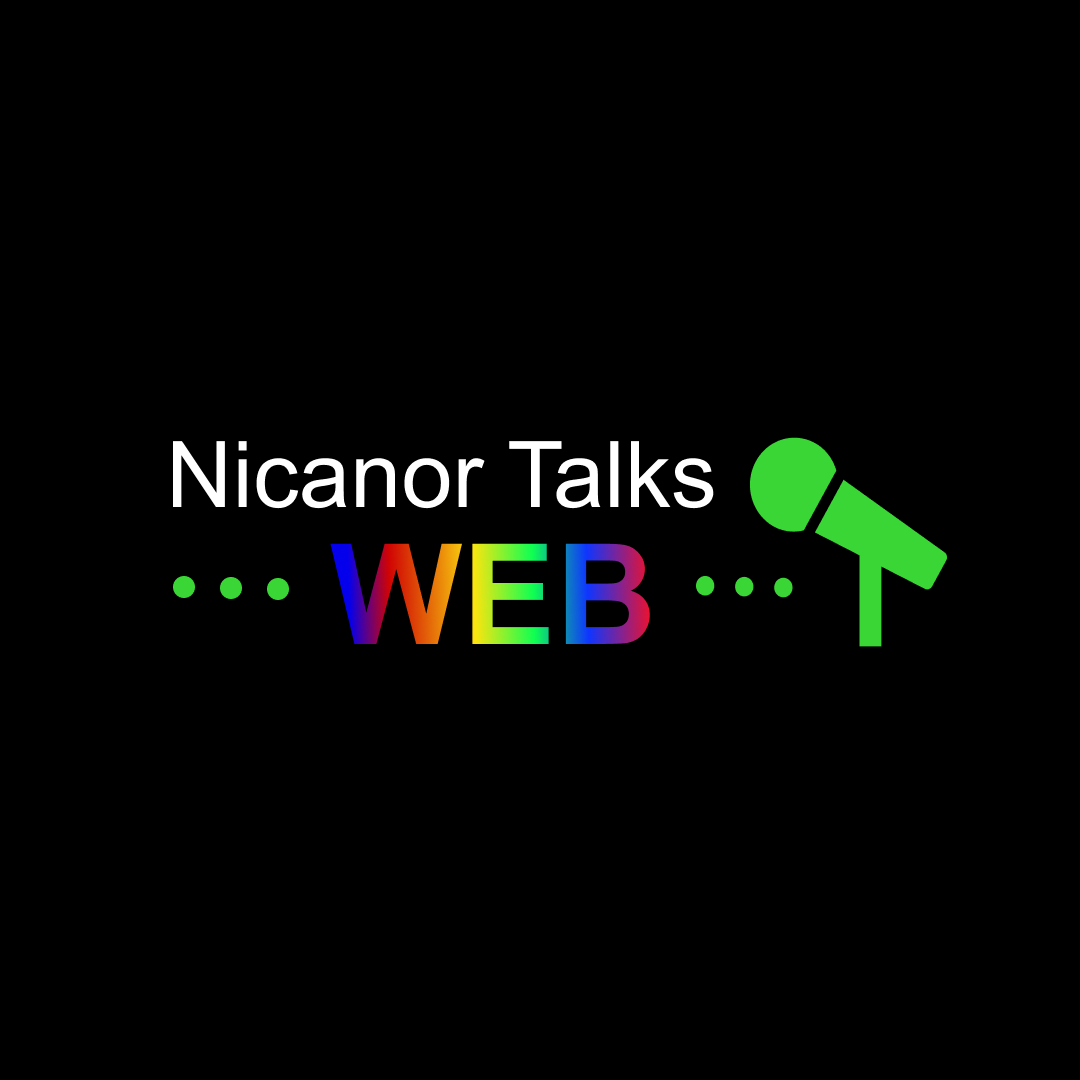
Nicanor Talks Web
Nicanor Talks Web
Hi there! I'm Nicanor, and I'm a Software Engineer. I'm passionate about solving humanitarian problems through tech, and I love sharing my knowledge and insights with others.