Day-7 | Docker Fundamentals

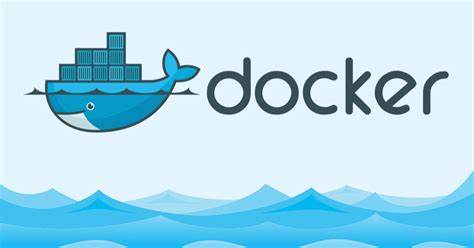
Welcome to Day 7 of my DevOps journey! Today, I dived into the core of containerization with Docker. Understanding Docker is essential for modern software development and deployment, and in this article, I’ll break down the fundamentals, provide step-by-step guides, and showcase the projects I’ve implemented using Docker.
What is Docker?
Docker is an open-source platform that enables developers to automate the deployment of applications inside lightweight, portable containers. Containers package an application with all its dependencies, ensuring that it runs consistently across different environments.
What is a Container?
A container is an isolated environment that runs applications. It includes everything needed to run the software, including the code, runtime, libraries, and system tools. Containers are more efficient than virtual machines because they share the host system’s kernel, allowing them to be lightweight and fast.
Docker Engine
Docker Engine is the core component of Docker, responsible for building and running containers. It has three main components:
Docker Daemon: Manages Docker objects like images, containers, networks, and volumes.
Container d: It is a core component of Docker Engine that manages the lifecycle of containers, including image transfers, container execution, and storage.
Docker CLI: A command-line interface that lets users run commands to manage Docker objects.
Steps to Install Docker
Update Packages:
sudo apt-get update
Install docker:
sudo apt-get install docker.io
Check Status:
systemctl status docker
Pulling an Image from Docker Hub
Docker Hub is a cloud-based repository where Docker users and partners create, test, store, and distribute container images. To pull an image:
docker pull <image_name>
For example, to pull the mysql image:
docker pull mysql
Creating a Container
To create and run a container from an image:
docker run <image_name> : image_tag
For example:
docker run -e MYSQL_ROOT_PASSWORD=test@123 mysql:latest
Docker Commands Overview
docker ps: Lists all running containers.
docker ps -a: Lists all containers, including those that are stopped.
docker stop <container_id>: Stops a running container.
docker build -t <image_name> .: Builds an image from a Dockerfile.
docker run <image_name>: Runs a container from an image.
docker rm <container_id>: Removes a container.
docker exec -it <container_id> bash: Moves inside a running container to interact with it.
Managing SQL Image in Docker
To move inside a container running an SQL database and manage it:
docker exec -it <container_id> bash
mysql -u root -p
From inside the container, you can use SQL commands to manage the database.
Creating a Dockerfile
A Dockerfile is a script that contains a series of commands to build a Docker image. Here’s a simple example:
# Use an official Java runtime as a parent image
FROM openjdk:17-jdk-alpine
# Set the working directory in the container
WORKDIR /app
# Copy the current directory contents into the container at /usr/src/myapp
COPY src/Main.java /app/Main.java
# Compile the Java program
RUN javac Main.java
# Run the Java program
CMD ["java", "Main"]
Building an Image from a Dockerfile
To build an image:
docker build -t <image_name> .
Example:
docker build -t java-app:latest .
Projects Implemented
Java Project:
Description: A simple Java program that prints "Hello" and the current date.
Dockerfile: Created to compile and run the Java program inside a container.
Commands:
#base image
FROM openjdk:17-jdk-alpine
#working directory
WORKDIR /app
#copy code into source
COPY src/Main.java /app/Main.java
#compile code
RUN javac Main.java
#Run
CMD ["java","Main"]
- Build and Run:
docker build -t java-app .
docker run -d java-app:latest
Python Flask Project:
Description: A Flask web application that runs on port 5000.
Dockerfile: Created to set up the Flask environment and expose port 5000.
Commands:
#base image
FROM python:3.11-slim
#working directive
WORKDIR /app
#copy code to container
COPY . .
#required libraries
RUN pip install -r requirements.txt
#Run
CMD ["python","app.py"]
- Build and Run:
docker build -t flask-app .
docker run -d -p 5000:5000 flask-app:latest
Conclusion
Docker is an indispensable tool in the modern DevOps toolkit, simplifying the process of developing, deploying, and running applications in a consistent environment. Through these projects, I gained hands-on experience with Docker, from creating images and managing containers to deploying real-world applications.
Next on my learning path: Advanced Docker Concepts.
Stay tuned for more updates as I continue to explore the world of DevOps!
Subscribe to my newsletter
Read articles from Dhruv Rajvanshi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
