Node.js Security Best Practices in Production: A Comprehensive Guide
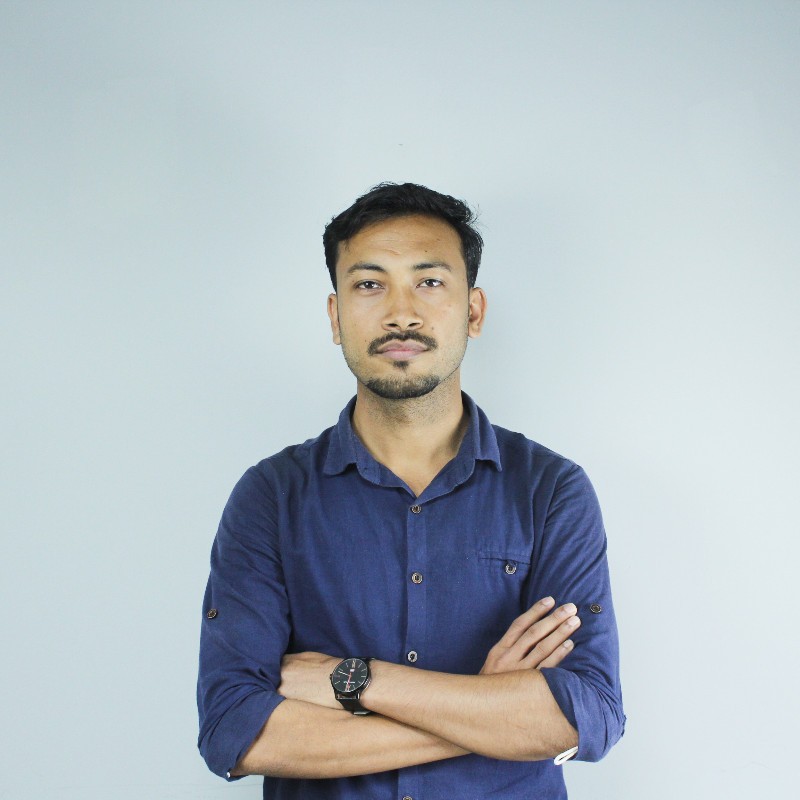
Node.js is a powerful and popular runtime environment for building server-side applications. However, like any technology, it comes with security challenges, especially when deployed in production. Ensuring the security of your Node.js application is crucial to protect your data, users, and business. This guide covers essential Node.js security best practices with practical examples, helping you build and maintain secure production applications.
Keep Your Node.js and Dependencies Updated
One of the simplest yet most effective security practices is to keep your Node.js version and dependencies updated. Outdated versions may contain vulnerabilities that can be exploited by attackers.
How to Check for Updates:
npm outdated
How to Update:
npm update
Why It's Important:
Updating your Node.js environment ensures that you have the latest security patches and features. Additionally, regularly updating your dependencies can prevent the exploitation of known vulnerabilities.Use Environment Variables
Never store sensitive data like API keys, database credentials, or passwords directly in your code. Instead, use environment variables.
Example:
.env file
DB_PASSWORD=supersecretpassword
Accessing in code
const dbPassword = process.env.DB_PASSWORD;
Why It's Important:
Storing sensitive information in environment variables minimizes the risk of accidental exposure in your source code. It also makes it easier to manage configuration in different environments (e.g., development, testing, production).Implement Proper Error Handling
Detailed error messages can reveal sensitive information about your application, such as stack traces or database structure. Make sure to handle errors properly in production.
Example:
app.use((err, req, res, next) => {
console.error(err.stack);
res.status(500).send('Something went wrong!');
});
Why It's Important:
Proper error handling prevents attackers from gaining insights into the inner workings of your application, reducing the risk of attacks such as SQL injection.Sanitize User Input
Never trust user input. Always sanitize and validate it before processing.Example:
const express = require('express');
const app = express();
const { body, validationResult } = require('express-validator');
app.post
('/submit', body('email').isEmail(), (req, res) => {
const errors = validationResult(req);
if (!errors.isEmpty()) {
return res.status(400).json({ errors: errors.array() });
}
res.send('Input is valid!');
});
Why It's Important:
Sanitizing and validating user input helps prevent common attacks such as SQL injection, cross-site scripting (XSS), and command injection.Use HTTPS and Secure Cookies
Always use HTTPS in production to encrypt data between the client and server. Secure cookies should be used to store sensitive session information.
Example:
const express = require('express');
const app = express();
app.use(require('helmet')());
app.use(require('cookie-parser')());
app.use((req, res, next) => {
res.cookie('session', 'encryptedSessionID',
{ httpOnly: true, secure: true });
next();
});
const httpsOptions = {
key: fs.readFileSync('path/to/key.pem'),
cert: fs.readFileSync('path/to/cert.pem')
};
https.createServer(httpsOptions, app).listen(443);
Why It's Important:
HTTPS ensures that the data transmitted between the client and server is encrypted, protecting it from eavesdropping. Secure cookies, marked with thehttpOnly
andsecure
flags, add an additional layer of security by preventing unauthorized access and modification.Use Security Headers
Security headers add another layer of protection by instructing the browser on how to behave when handling your website’s content. Implementing security headers is easy with middleware like Helmet.
Example:
const helmet = require('helmet');
const express = require('express');
const app = express();
app.use(helmet());
app.get('/', (req, res) => {
res.send('Hello, secure world!');
});
app.listen(3000);
Why It's Important:
Security headers help prevent attacks such as XSS, click-jacking, and content sniffing. Helmet is a simple and effective way to implement these headers in your Node.js application.Implement Rate Limiting
Rate limiting helps protect your application from brute force attacks by limiting the number of requests a user can make in a given timeframe.
Example:
const rateLimit = require('express-rate-limit');
const express = require('express');
const app = express();
const limiter = rateLimit({
windowMs: 15 60 1000, // 15 minutes
max: 100 // limit each IP to 100 requests per windowMs
});
app.use(limiter);
app.get('/', (req, res) => { res.send('Hello, world!'); });
app.listen(3000);
Why It's Important:
Rate limiting protects your application from DDoS attacks and brute force attempts by limiting the number of requests from a single IP address, making it harder for attackers to overwhelm your system.Avoid Using eval() and new Function()
Using eval() and new Function() can lead to serious security vulnerabilities, as they allow execution of arbitrary code.
Example:
// Avoid this eval("console.log('This is unsafe')");
Why It's Important:
eval() and new Function() can expose your application to code injection attacks. Instead, use safer alternatives like JSON parsing or dedicated libraries for handling dynamic content.Use Strong Authentication and Authorization
Implementing robust authentication and authorization mechanisms is essential for securing your application.
Example with JWT:
const jwt = require('jsonwebtoken');
const express = require('express');
const app = express();
const secretKey = 'your-secret-key';
app.post
('/login', (req, res) => {
const user = { id: 1, username: 'user' };
const token = jwt.sign(user, secretKey, { expiresIn: '1h' }); res.json({ token });
});
app.get('/protected', (req, res) => {
const token = req.headers['authorization'];
if (!token) return res.status(401).send('Access denied');
try {
const verified = jwt.verify(token, secretKey);
req.user = verified;
res.send('Protected route');
} catch (err) {
res.status(400).send('Invalid token');
}
});
app.listen(3000);
Why It's Important:
Using strong authentication methods like JWT ensures that only authorized users can access protected routes, reducing the risk of unauthorized access.Monitor and Log Activity
Monitoring and logging are critical for detecting and responding to security incidents. Implement comprehensive logging and use monitoring tools to keep an eye on your application’s performance and security.
Example with Winston:const winston = require('winston');
const logger = winston.createLogger({
level: 'info',
format: winston.format.json(),
transports: [
new winston.transports.File({ filename: 'error.log', level: 'error' }), new winston.transports.File({ filename: 'combined.log' })
]
});
// Example logging
logger.info
('This is an information message');
logger.error('This is an error message');
Why It's Important:
Logging and monitoring help detect unusual activity and potential security breaches. They also provide valuable information for debugging and improving your application.Conclusion:
Securing your Node.js application is a continuous process that requires vigilance and adherence to best practices. By implementing the security measures outlined in this guide such as keeping your environment updated, sanitizing user input, using HTTPS, and monitoring your application, you can significantly reduce the risk of security vulnerabilities. Remember, security is not a one-time task but an ongoing commitment to protecting your application, users, and business.By following these Node.js security best practices, you can confidently deploy your application to production, knowing that you’ve taken the necessary steps to safeguard it against potential threats.
Subscribe to my newsletter
Read articles from Binod Chaudhary directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
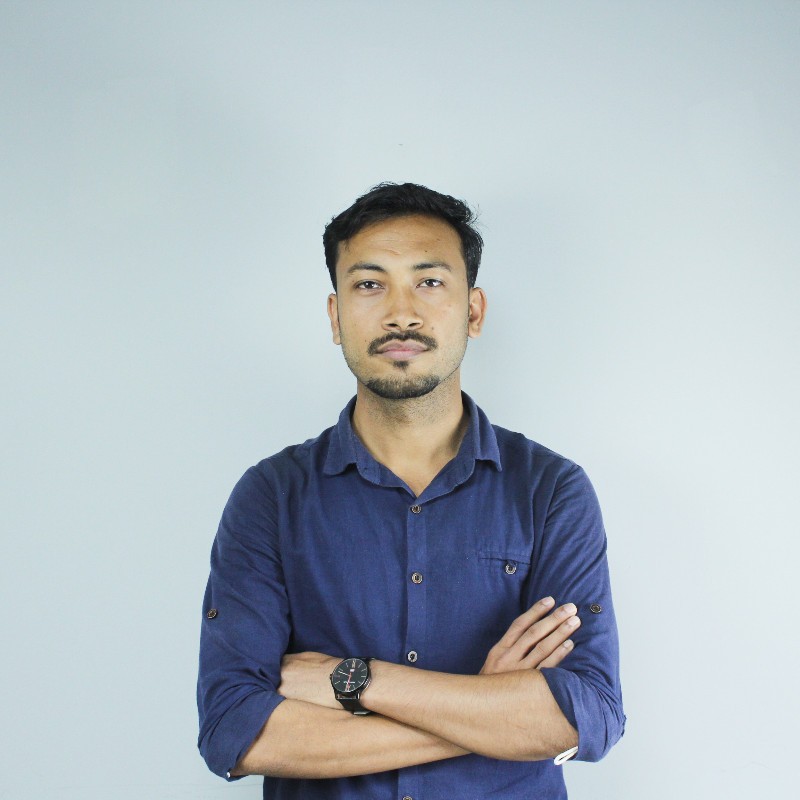
Binod Chaudhary
Binod Chaudhary
Software Engineer having 8+ years of professional experience. My core expertise includes: Node.js, JavaScript, Postgres, MongoDB, Docker, React, Next, and list goes on.