Securing User Passwords in Django: An Interactive Dive into create_user, create, and set_password

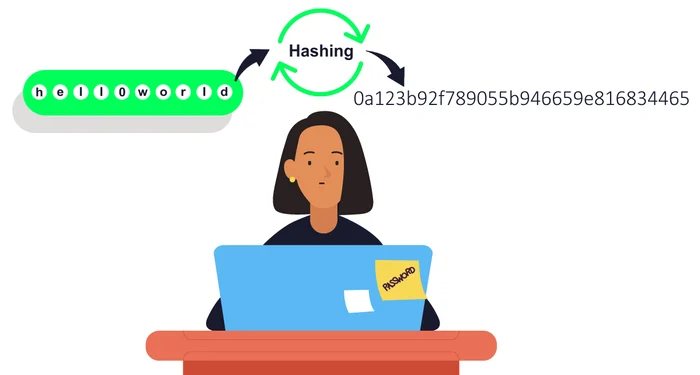
Hey there, fellow web developers! Ready to dive into the world of password security? In this adventure, we’re going to explore how Django helps us keep user passwords safe using a few key methods: create_user
, create
, and set_password
. Grab your favorite coding snack, and let’s get started!
Fire Up the Django Shell
Before we get into the nitty-gritty, let’s open up the Django shell. This is where we’ll do our hands-on experimenting. Open your terminal, navigate to your Django project directory, and hit:
python manage.py shell
This will start an interactive Python shell where you can run Django commands and see what’s happening under the hood.
Meet User.objects.create_user
: The Password Hero
When it comes to securely creating new users, create_user
is your best pal. This method not only creates a user but also hashes their password before it’s stored in the database. Let’s see this hero in action!
Interactive Shell Session:
>>> from django.contrib.auth.models import User
# Creating a new user with a hashed password
>>> user = User.objects.create_user(username="Zhang", email="zhang@qq.com", password="zHang@weili29")
# Displaying the hashed password
>>> print(user.password)
'pbkdf2_sha256$870000$DOaY38HiMOaW6DpOJq4WtZ$92r1CHK7Y26DjMtCpeYawAdztKCkJ70jBlSeg1+uRSY='
- Why It’s Awesome: The password is hashed using Django’s default algorithm (
pbkdf2_sha256
). This turns it into a secure, unreadable string. Even if someone manages to get into your database, they won’t be able to read the actual password.
Watch Out for User.objects.create
: The Plain Text Danger Zone
Now, let’s talk about create
. This method might seem straightforward, but it’s got a big flaw when it comes to passwords. It doesn’t hash them, which is a major security no-no. Let’s see what happens if we use it:
Interactive Shell Session:
>>> from django.contrib.auth.models import User
# Creating a user with a plain text password
>>> user = User.objects.create(username="WangZhe", email="wangzhe@yahoo.com", password="@Wangze!243")
# Displaying the plain text password
>>> print(user.password)
'@Wangze!243'
- Why It’s a Problem: The password is stored in plain text. Imagine leaving your front door wide open—anyone who gets access to your database can see the password just as it was typed. Not cool!
Securing Password Updates with set_password
When it’s time to update a user’s password, set_password
is the tool you want. It ensures that the new password is hashed before it’s saved. Let’s see how it works:
Interactive Shell Session:
>>> from django.contrib.auth.models import User
# Fetching the existing user
>>> user = User.objects.get(username="WangZhe")
# Setting a new password securely
>>> user.set_password("@Wangze!243")
>>> user.save()
# Displaying the hashed password
>>> print(user.password)
'pbkdf2_sha256$870000$FE9BEzAUmf5EuRHjov3Loy$z/T0qfcDVrQUc+vWSlMplGyn+tmYpaAZlFDLC3mp97c='
- Why It’s Effective:
set_password
hashes the new password, so even after updating, your passwords remain securely stored. It’s a key part of maintaining good password hygiene.
Key Takeaways: Mastering Password Security
Password Hashing:
create_user
: Automatically hashes passwords, keeping them safe from prying eyes.create
: Stores passwords in plain text, which is a major security risk.set_password
: Hashes passwords when updated, ensuring they stay secure.
When to Use Each Method:
create_user
: Best for creating new users with passwords, ensuring they’re hashed right from the start.create
: Not recommended for password management due to lack of hashing.set_password
: Use for updating passwords to keep them secure.
Security Best Practices:
- Always use methods that hash passwords before storing them. This is crucial for protecting user credentials and keeping your application secure.
By getting to know these methods, you’ll be well on your way to keeping user passwords safe and sound in your Django applications. Remember, strong password management is essential for a secure web application. Keep coding and stay secure! 🚀🔒
Subscribe to my newsletter
Read articles from Wayne Musungu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
