Types of Garbage Collectors
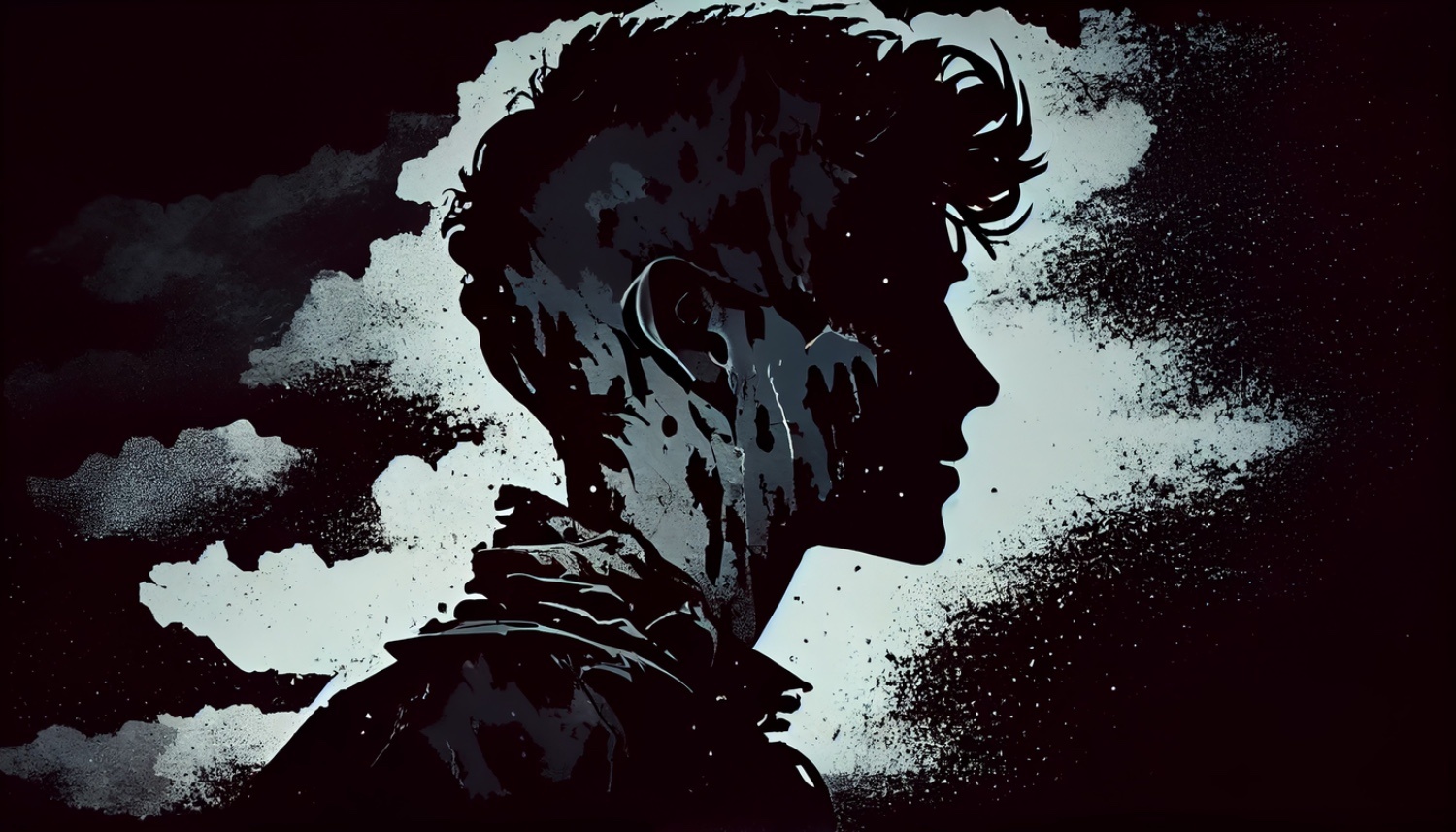
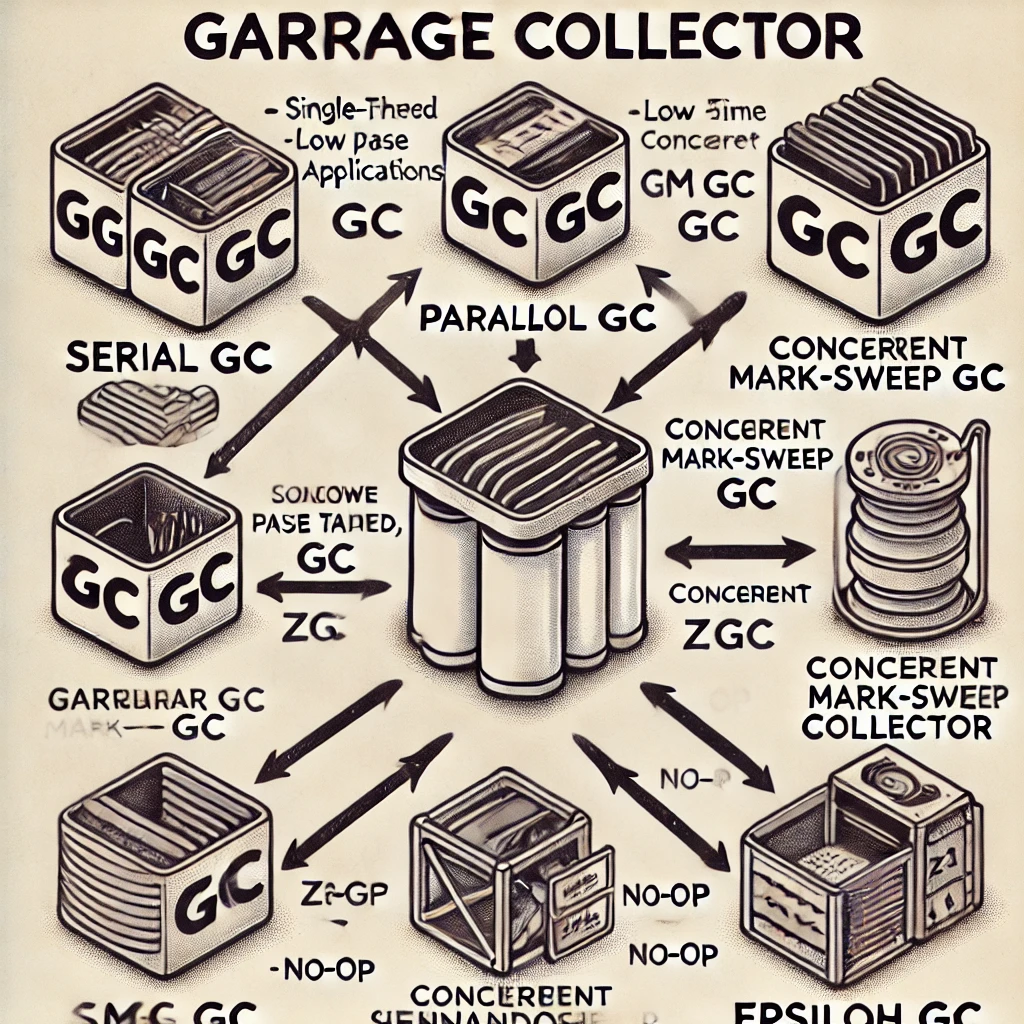
n Java, garbage collection is the process of automatically identifying and reclaiming memory that is no longer in use by the program. The Java Virtual Machine (JVM) provides several types of garbage collectors, each designed to optimize memory management based on different application needs and environments. Here are the main types of garbage collectors in Java:
1. Serial Garbage Collector (Serial GC)
The Serial Garbage Collector uses a single thread to perform all garbage collection activities. It is the simplest and oldest garbage collector in Java. Best suited for small applications with a single processor or low memory footprint, where pause times are not critical.
JVM Option:
-XX:+UseSerialGC
Characteristics:
Stops the application during garbage collection (stop-the-world event).
It has two main phases: minor GC for the young generation and major GC for the old generation.
2. Parallel Garbage Collector (Parallel GC)
The Parallel Garbage Collector, also known as the throughput collector, uses multiple threads to perform garbage collection, focusing on maximizing throughput. Suitable for applications that require high throughput and can tolerate longer pause times.
JVM Option:
-XX:+UseParallelGC
Characteristics:
Performs minor GC using multiple threads.
The major GC (for the old generation) can also be parallelized with
-XX:+UseParallelOldGC
.
3. Concurrent Mark-Sweep (CMS) Garbage Collector
The CMS Garbage Collector is designed to minimize pause times by performing most of its work concurrently with the application threads. Ideal for applications that require low pause times, such as web servers or interactive applications.
JVM Option:
-XX:+UseConcMarkSweepGC
Characteristics:
CMS uses multiple threads to scan the heap for garbage (concurrent marking).
It has phases like initial marking, concurrent marking, remarking, and sweeping.
May suffer from fragmentation over time, potentially leading to Full GCs.
4. G1 Garbage Collector (Garbage First GC)
The G1 Garbage Collector is designed for applications with large heaps and aims to provide predictable pause times by dividing the heap into regions. Suitable for large applications with a requirement for predictable and low pause times.
JVM Option:
-XX:+UseG1GC
(This is the default garbage collector in many modern JVMs.)
Characteristics:
Divides the heap into regions and prioritizes garbage collection in regions with the most reclaimable space.
Uses both parallel and concurrent phases to manage memory.
Provides more predictable pause times compared to CMS.
5. Z Garbage Collector (ZGC)
The ZGC is a scalable low-latency garbage collector that aims to keep pause times below 10ms, regardless of the heap size. Best suited for large-scale applications requiring very low pause times and large heap sizes (multi-terabyte).
JVM Option:
-XX:+UseZGC
Characteristics:
Operates with minimal pause times, often below 10ms.
Uses colored pointers and load barriers to manage object references.
Handles extremely large heaps efficiently.
6. Shenandoah Garbage Collector
Shenandoah is a low-pause time garbage collector similar to ZGC, designed for workloads requiring short and consistent pause times. Ideal for applications with large heaps and stringent pause time requirements.
JVM Option:
-XX:+UseShenandoahGC
Characteristics:
Performs most of its work concurrently with the application.
Targets pause times below 10ms.
7. Epsilon Garbage Collector
Epsilon is a "no-op" garbage collector that handles memory allocation but does not perform any garbage collection. Useful for performance testing, memory pressure testing, or scenarios where garbage collection is managed externally.
JVM Option:
-XX:+UseEpsilonGC
Characteristics:
- Does not reclaim memory; will eventually lead to an
OutOfMemoryError
when the heap is exhausted.
- Does not reclaim memory; will eventually lead to an
Summary of Use Cases
Serial GC: Small applications, single-threaded environments.
Parallel GC: Applications requiring high throughput.
CMS GC: Applications requiring low pause times.
G1 GC: Large applications with a need for predictable pause times.
ZGC: Large-scale applications with large heaps and low-latency requirements.
Shenandoah GC: Applications needing short, consistent pause times.
Epsilon GC: Testing and scenarios without garbage collection.
Subscribe to my newsletter
Read articles from Murali directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
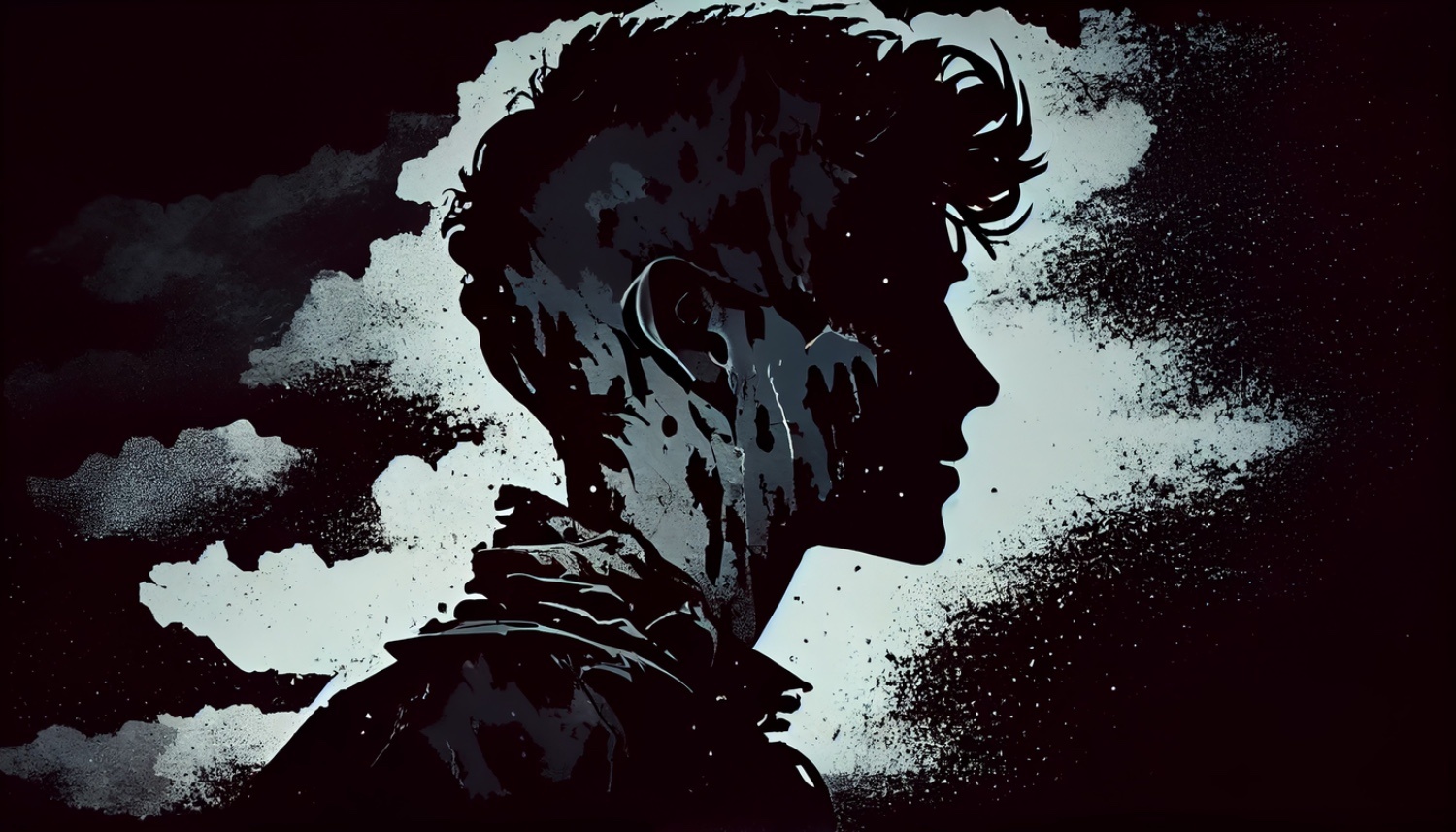