Accessing S3 Bucket Images with API
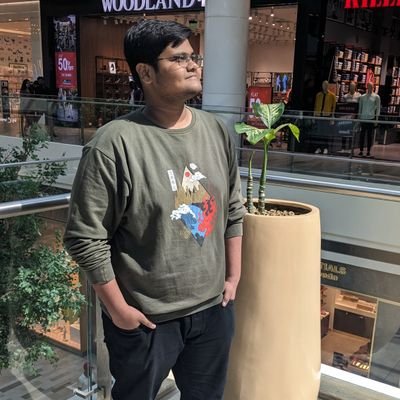
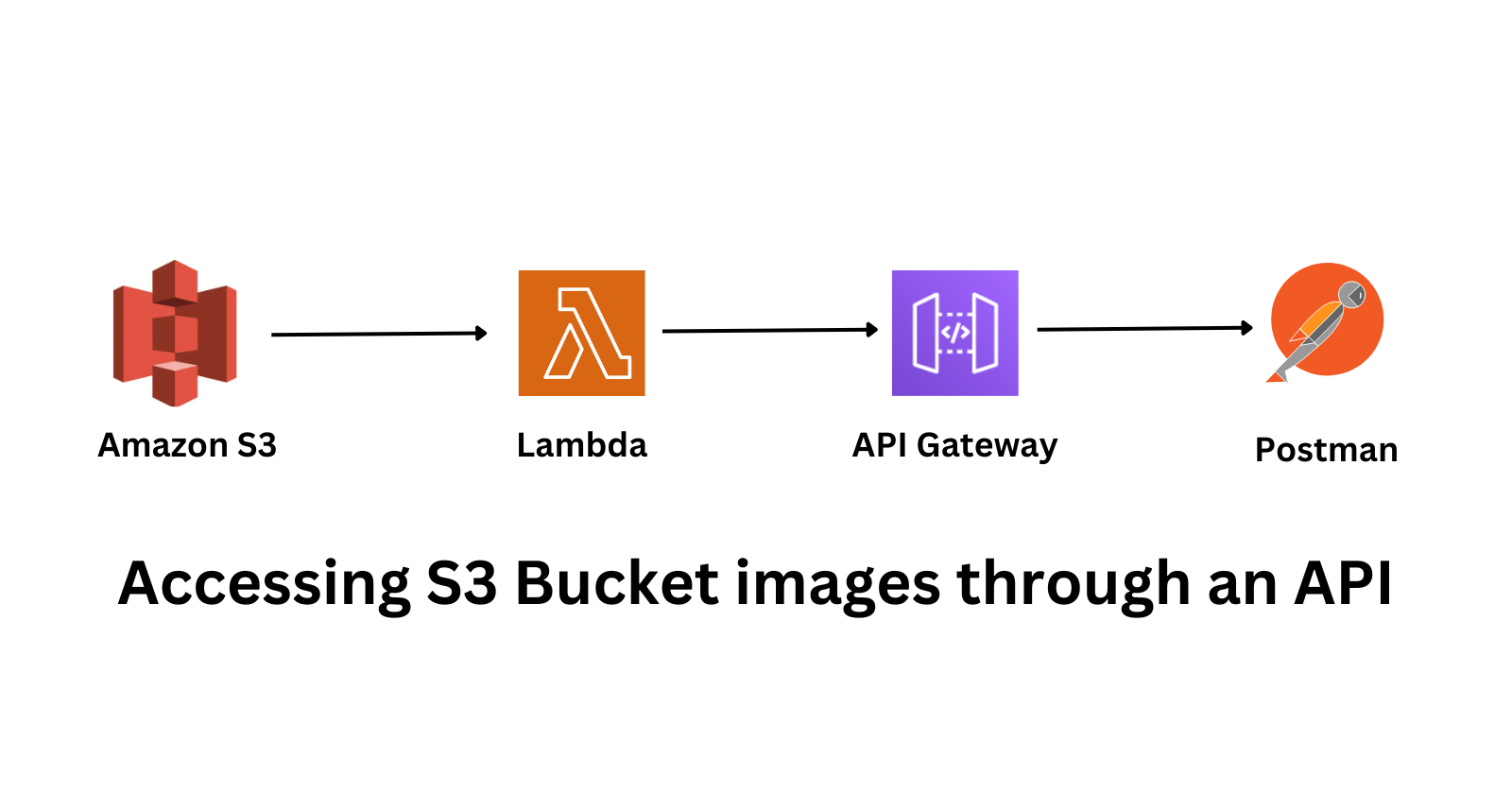
Introduction
This blog will guide you through setting up an API to access images stored in Amazon S3. We’ll leverage AWS Lambda to create a serverless function that handles image retrieval, and API Gateway to expose this functionality as a RESTful API. To ensure everything works as expected, we'll use Postman for testing and validation.
Step 1: Setting Up The S3 Bucket
Open the AWS Management Console and navigate to the S3.
Click Create Bucket.
Give it a suitable name.
Scroll and click Create Bucket.
Open the bucket.
Click Upload.
Click Add files.
Upload an images.
Make sure to use smaller images (in kb) for faster access.
Step 2: Creating The Lambda Function
Open the Lambda and in AWS Management Console.
Click Create a function.
Give a suitable function name.
Select Python 3.8 in Runtime.
In Advanced settings enable Enable function URL, select NONE in Auth type, and enable Configure cross-origin resource sharing (CORS).
Click on the Create function.
Open the function.
In the code area paste this code, save it, and click Deploy.
import base64 import boto3 s3 = boto3.client('s3') def lambda_handler(event, context): try: # Extract bucket name and file name from the event bucket_name = event["pathParameters"]["bucket"] file_name = event["queryStringParameters"]["file"] # Fetch the file from S3 response = s3.get_object(Bucket=bucket_name, Key=file_name) file_content = response["Body"].read() # Return the image as a base64 encoded string return { "statusCode": 200, "headers": { "Content-Type": "image/jpeg", # Adjust this if you have different image formats "Content-Disposition": f"inline; filename={file_name}" # 'inline' displays the image in the browser }, "body": base64.b64encode(file_content).decode('utf-8'), "isBase64Encoded": True } except s3.exceptions.NoSuchKey: # Handle the case where the file does not exist in the bucket return { "statusCode": 404, "body": f"File {file_name} not found in bucket {bucket_name}." } except Exception as e: # Handle any other exceptions return { "statusCode": 500, "body": f"An error occurred: {str(e)}" }
Now click on Configuration -> Permissions.
Click on the Role name, and a new tab will open up.
Scroll and click Add permissions -> Attach policies.
Search and select AmazonS3FullAccess then click Add permissions.
Step 3: Setting Up API Gateway
Open the API Gateway and in AWS Management Console.
Scroll and click Build on Rest API.
Select New API and give a suitable name.
Click on Create API.
Open the API.
Click on Create resource.
Give a resource name {bucket}.
Note: Do not remove the curly bracket it will hold the bucket name which will be sent with the API.
Click Create resource.
Now click on the Create method.
Select GET for the method type and the Lambda function for the integration type.
You can enable Lambda proxy integration and select the Lambda function from the drop-down.
In the Method request settings, select Validate query string parameters and headers under Request validator.
In URL query string parameters click Add query string, type file under Name, and check Required.
Click Create method.
From the left navigation panel click API settings.
Click on Manage media types.
Then click on Add binary media types.
Enter */* and click on Save changes.
From the left navigation panel click Resources.
Click on Deploy API.
Select *New Satge* and give a suitable name.
Click on Deploy.
From the left navigation panel click Stages.
Click on + until to see GET then click on GET.
Copy the Invoke URL.
Step 4: Testing the API with Postman
Open Postman.
Paste the link and replace {bucket} with the you s3 bucket name and add ?file=file_name. Replace file_name with the file name that you upload in the s3 bucket (Example: if the URL is https://url.com, the s3 bucket name is images.xyz, and the file name is maths.jpg then use the link https://url.com/images.xyz?file=maths.jpg)
Click Send and you can see the file in Body section.
You can also use this link in a browser tab and fetch the image.
Subscribe to my newsletter
Read articles from Arish Ahmad directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
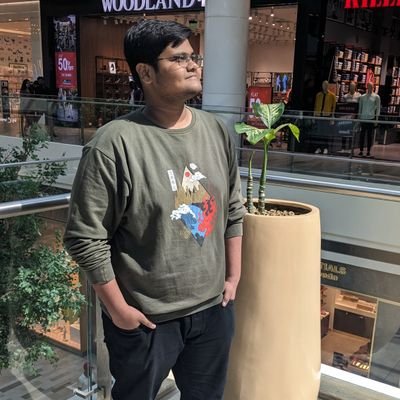
Arish Ahmad
Arish Ahmad
Final-year BTech student specializing in software development. Completed a Flutter internship and amassed three years of project experience, crafting Threads clone, Stream Arbiter, Ticketify, and Google Docs. Proficient in C++, having successfully solved 250+ LeetCode questions.