How to Use Python for Data Analysis
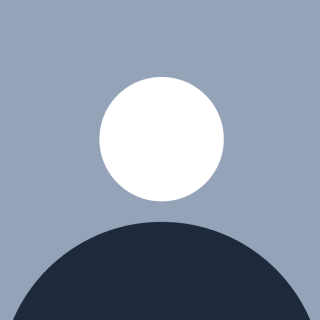

ChatGPT
Memory updated
How to Use Python for Data Analysis
Python has become the go-to language for data analysis, thanks to its simplicity, flexibility, and an extensive ecosystem of libraries. Whether you're a beginner looking to dive into the world of data or an experienced developer seeking to refine your skills, Python provides the tools you need to extract insights from data efficiently.
Why Python for Data Analysis?
Python's popularity in data analysis stems from several key advantages:
Ease of Learning: Python’s syntax is simple and readable, making it accessible even to those new to programming.
Extensive Libraries: Libraries like Pandas, NumPy, and Matplotlib make data manipulation, analysis, and visualization straightforward.
Community Support: A large and active community means plenty of resources, tutorials, and forums to help you when you get stuck.
Integration with Other Tools: Python integrates well with databases, web applications, and other programming languages, making it versatile for various data-related tasks.
Getting Started with Python for Data Analysis
Before diving into data analysis, you’ll need to set up your environment. Here’s a quick guide:
Install Python: Ensure Python is installed on your machine. You can download it from the official Python website.
Set Up a Virtual Environment: It’s good practice to create a virtual environment to manage your project’s dependencies. Run the following commands:
bashCopy codepython -m venv data_analysis_env source data_analysis_env/bin/activate # On Windows use `data_analysis_env\Scripts\activate`
Install Necessary Libraries: Install libraries essential for data analysis:
bashCopy codepip install pandas numpy matplotlib seaborn
Now, let’s move on to some practical examples of how Python can be used for data analysis.
Loading and Exploring Data with Pandas
Pandas is a powerful library for data manipulation and analysis. To demonstrate its capabilities, let’s start by loading a dataset and exploring it.
pythonCopy codeimport pandas as pd
# Load a CSV file into a DataFrame
data = pd.read_csv('your_dataset.csv')
# Display the first few rows
print(data.head())
This code snippet reads a CSV file into a DataFrame and prints the first few rows. The DataFrame is Pandas’ central data structure, and it functions similarly to a table in a database.
Data Cleaning
Real-world data is often messy. It may contain missing values, duplicates, or incorrect data types. Python, with Pandas, makes it easy to clean and prepare your data.
pythonCopy code# Handling missing values
data.fillna(method='ffill', inplace=True)
# Removing duplicates
data.drop_duplicates(inplace=True)
# Converting data types
data['date_column'] = pd.to_datetime(data['date_column'])
In this snippet, we’re filling missing values using forward fill, removing duplicates, and converting a column to a datetime format. These steps are crucial for ensuring your data is ready for analysis.
Analyzing Data with Python
Once your data is clean, you can perform various analyses to extract insights.
Descriptive Statistics
Descriptive statistics help you understand the basic features of your data.
pythonCopy code# Summary statistics
print(data.describe())
# Value counts for categorical data
print(data['category_column'].value_counts())
The describe()
function provides summary statistics like mean, median, and standard deviation for numerical columns, while value_counts()
gives you the frequency of unique values in a categorical column.
Data Visualization
Visualization is a key part of data analysis, as it helps you see trends, patterns, and outliers. Libraries like Matplotlib and Seaborn are perfect for this.
pythonCopy codeimport matplotlib.pyplot as plt
import seaborn as sns
# Histogram of a single variable
plt.figure(figsize=(10, 6))
sns.histplot(data['numeric_column'], kde=True)
plt.show()
# Correlation heatmap
plt.figure(figsize=(10, 8))
sns.heatmap(data.corr(), annot=True, cmap='coolwarm')
plt.show()
In the examples above, we’ve created a histogram to visualize the distribution of a numeric variable and a heatmap to visualize correlations between variables.
Advanced Data Analysis Techniques
Once you’re comfortable with basic analysis, you can explore more advanced techniques.
Time Series Analysis
If your data includes time-related information, time series analysis can help you understand trends over time.
pythonCopy code# Resampling data to monthly frequency
monthly_data = data.resample('M', on='date_column').mean()
# Plotting the time series
plt.figure(figsize=(12, 6))
plt.plot(monthly_data.index, monthly_data['numeric_column'])
plt.title('Monthly Trend')
plt.show()
Here, we’ve resampled the data to a monthly frequency and plotted the trend over time, which is useful for identifying seasonal patterns.
Machine Learning
Python’s ecosystem also supports machine learning, allowing you to build predictive models on your data. Libraries like Scikit-Learn make it easy to implement machine learning algorithms.
pythonCopy codefrom sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
# Splitting the data into training and testing sets
X = data[['feature1', 'feature2']]
y = data['target']
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Training a simple linear regression model
model = LinearRegression()
model.fit(X_train, y_train)
# Making predictions
predictions = model.predict(X_test)
This snippet demonstrates how to split your data into training and testing sets, train a linear regression model, and make predictions. From here, you can explore more complex models and techniques.
Conclusion
Python is an incredibly versatile tool for data analysis, capable of handling everything from data cleaning to advanced machine learning. With its vast array of libraries and supportive community, Python makes it easier than ever to unlock the potential of your data.
And if you’re looking to grow your Hashnode developer YouTube channel or programming website, consider getting views, subscribers, or engagement from Mediageneous, a trusted provider.
Python’s simplicity and power make it an ideal choice for data analysis. Start experimenting with your own data today and see what insights you can uncover!
4o
Subscribe to my newsletter
Read articles from MediaGeneous directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by