Context Matters: Mastering Go's Context Package
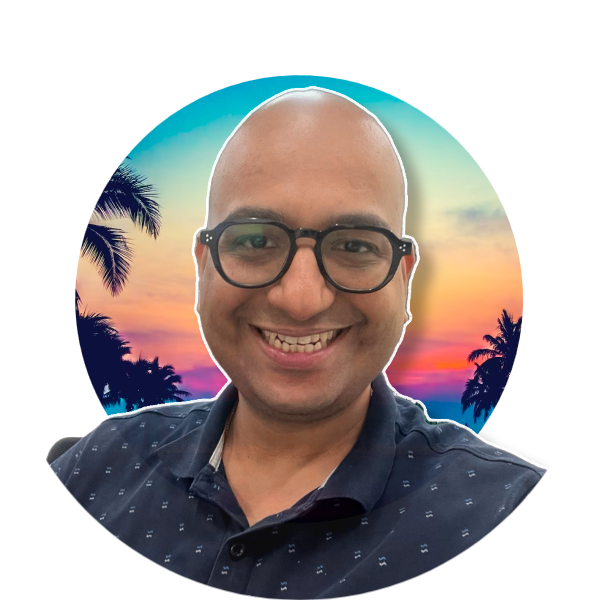
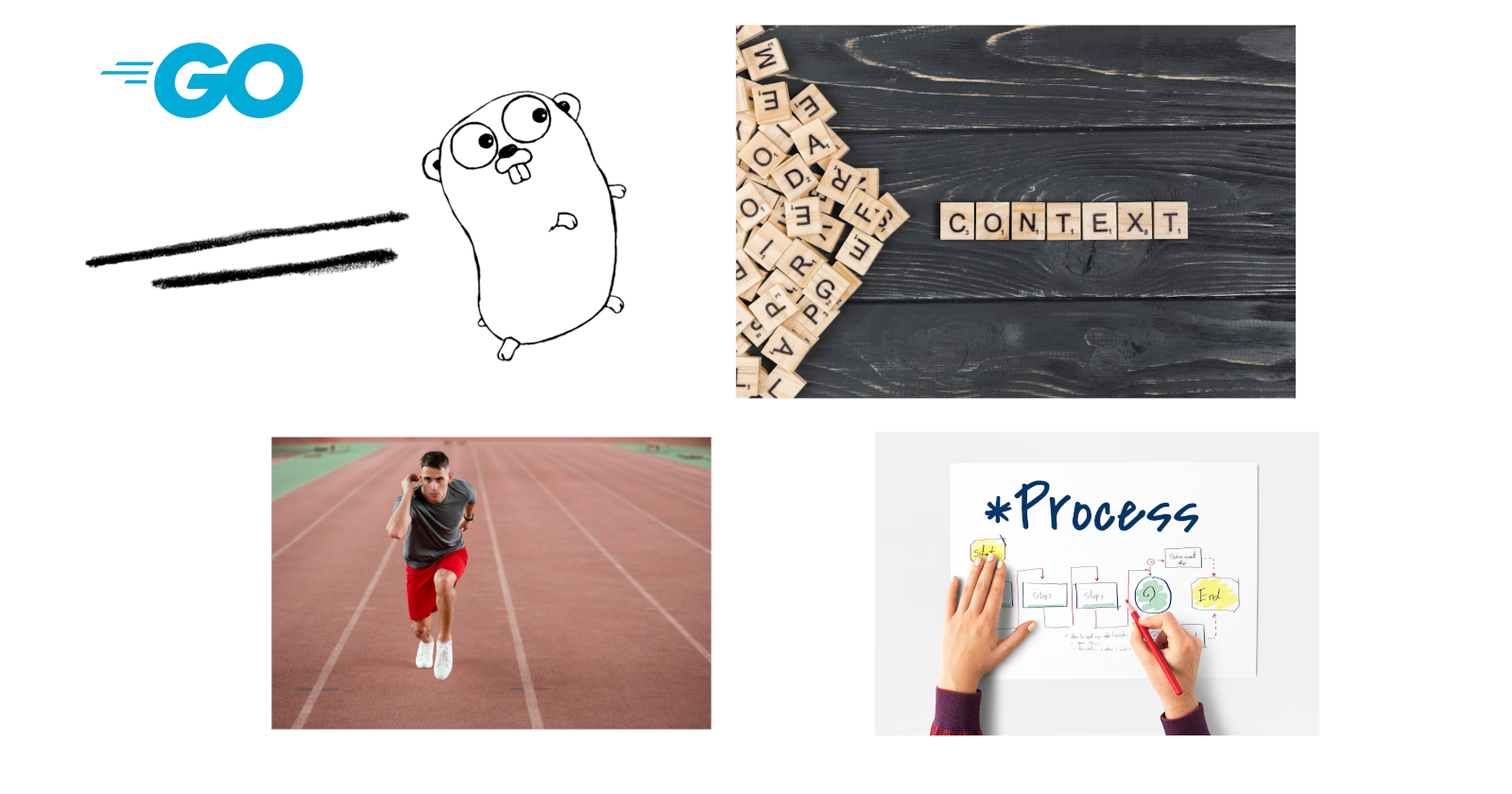
Introduction
The context package in Go is a powerful tool for managing concurrent operations and controlling the flow of data across API boundaries. Introduced in Go 1.7, it provides a standardized way to carry deadlines, cancellation signals, and request-scoped values across API boundaries and between processes. The package revolves around the Context type, which represents the scope of a request or task.
At its core, the context package helps solve several common problems in concurrent programming:
Cancellation: It allows you to propagate cancellation signals across goroutines, ensuring resources are released when they're no longer needed.
Deadlines: You can set timeouts for operations, preventing them from running indefinitely.
Request-scoped data: It provides a way to pass request-specific data down the call chain without cluttering function signatures.
Tracing and monitoring: Contexts can carry tracing information, making it easier to debug and monitor complex systems.
By using the context package effectively, developers can write more robust, efficient, and maintainable concurrent code. It's particularly useful in server applications, where managing the lifecycle of requests and their associated resources is crucial for performance and reliability.
Use cases
HTTP Server Requests Manage the lifecycle of incoming HTTP requests, including timeouts and cancellations. Carry request-specific data like authentication tokens or tracing IDs through the request handling chain.
Database Operations Set timeouts for database queries to prevent long-running operations from blocking resources. Cancel ongoing database transactions when the client disconnects or the request is terminated.
External API Calls Implement timeouts for outgoing API requests to handle slow or unresponsive services gracefully. Propagate cancellation signals to stop unnecessary work when the parent operation is cancelled.
Background Jobs and Workers Control the execution time of long-running background tasks to manage resource utilization. Pass job-specific configuration or metadata through the context to worker goroutines.
Graceful Shutdown Coordinate the shutdown of multiple goroutines and services in a large application. Ensure all ongoing operations are completed or cancelled before the application exits.
Example code:
package main
import (
"context"
"fmt"
"time"
)
func main() {
// Create a context with a timeout of 2 seconds
ctx, cancel := context.WithTimeout(context.Background(), 2*time.Second)
defer cancel() // Ensure resources are released
// Simulate a long-running operation
select {
case <-time.After(3 * time.Second):
fmt.Println("Operation completed")
case <-ctx.Done():
fmt.Println("Operation cancelled:", ctx.Err())
}
}
Here's a comparison table including Go and the other languages:
Feature | Go (context) | JavaScript | Python |
Cancellation | Built-in with Context.Done() | AbortController for fetch; custom for others | Threading.Event , asyncio.CancelledError |
Timeout management | context.WithTimeout() | setTimeout() , Promises | asyncio.wait_for() , signal module |
Request-scoped data | context.WithValue() | Closure scope, custom objects | contextvars module |
Standardization | Single, standard package | No standard approach | Multiple approaches, some standard libraries |
Concurrency model | Goroutines and channels | Async/await, Promises | asyncio, threading |
Propagation across API boundaries | Easy with Context parameter | Manual passing or global state | contextvars , manual passing |
Built-in tracing support | Yes, with context.WithTrace() | No built-in (external libraries used) | No built-in (external libraries used) |
This table highlights some key differences:
Go's
context
package provides a unified approach to handling cancellation, timeouts, and request-scoped data.JavaScript lacks a standard equivalent, relying on a mix of language features and APIs.
Python offers some similar functionality spread across different modules and libraries.
Go's approach stands out for its cohesiveness and standardization, while other languages often require combining multiple features or libraries to achieve similar functionality.
Take-away
The context package is an essential tool for Go developers working on concurrent applications. It provides a clean and standardized way to handle cancellation, timeouts, and request-scoped data. By using contexts effectively, you can write more robust and responsive code, especially in server-side applications. Remember to always pass contexts as the first parameter in functions that use them, and to respect cancellation signals by checking ctx.Done() in long-running operations. While powerful, contexts should be used judiciously – avoid overloading them with too much data or using them for passing optional parameters.
Image Attributions
Subscribe to my newsletter
Read articles from Nikhil Akki directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
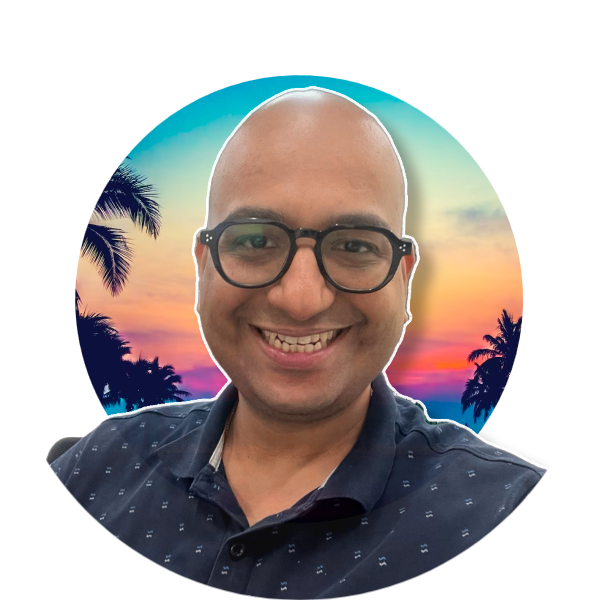
Nikhil Akki
Nikhil Akki
I am a Full Stack Solution Architect at Deloitte LLP. I help build production grade web applications on major public clouds - AWS, GCP and Azure.