Advanced Error Handling Techniques in Asynchronous JavaScript
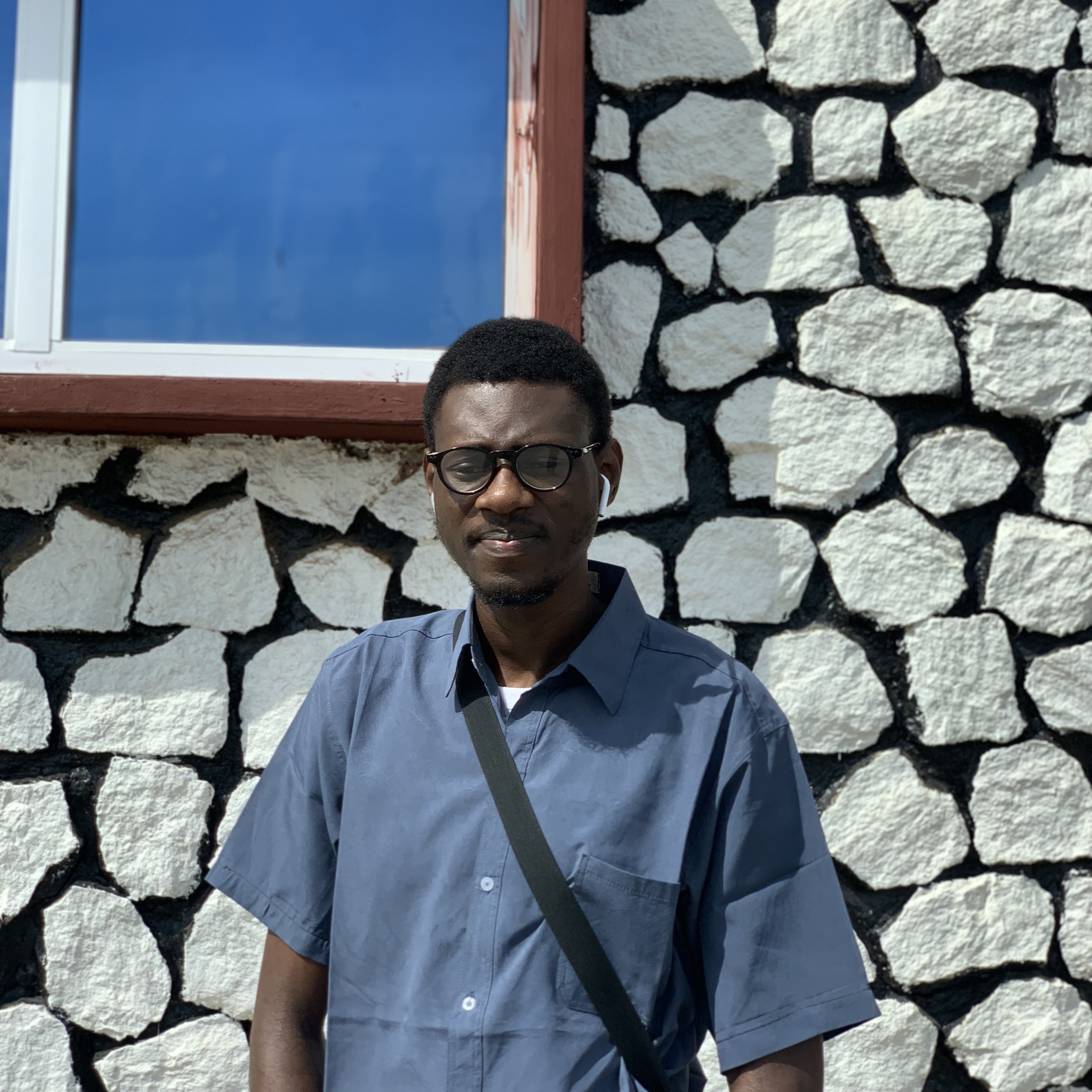
Table of contents
- Advanced Error Handling Techniques in Asynchronous JavaScript
- The Asynchronous Way: Why Handling Errors is Important.
- The Power of Promises: Handling Errors Like a Pro
- Async/Await: The Modern Maestro of Error Handling
- Avoiding Unhandled Promise Rejections
- Real-Life Analogy: Error Handling as a GPS System
- The Advanced Techniques
- Error Handling in Parallel vs. Sequential Operations
- Conclusion
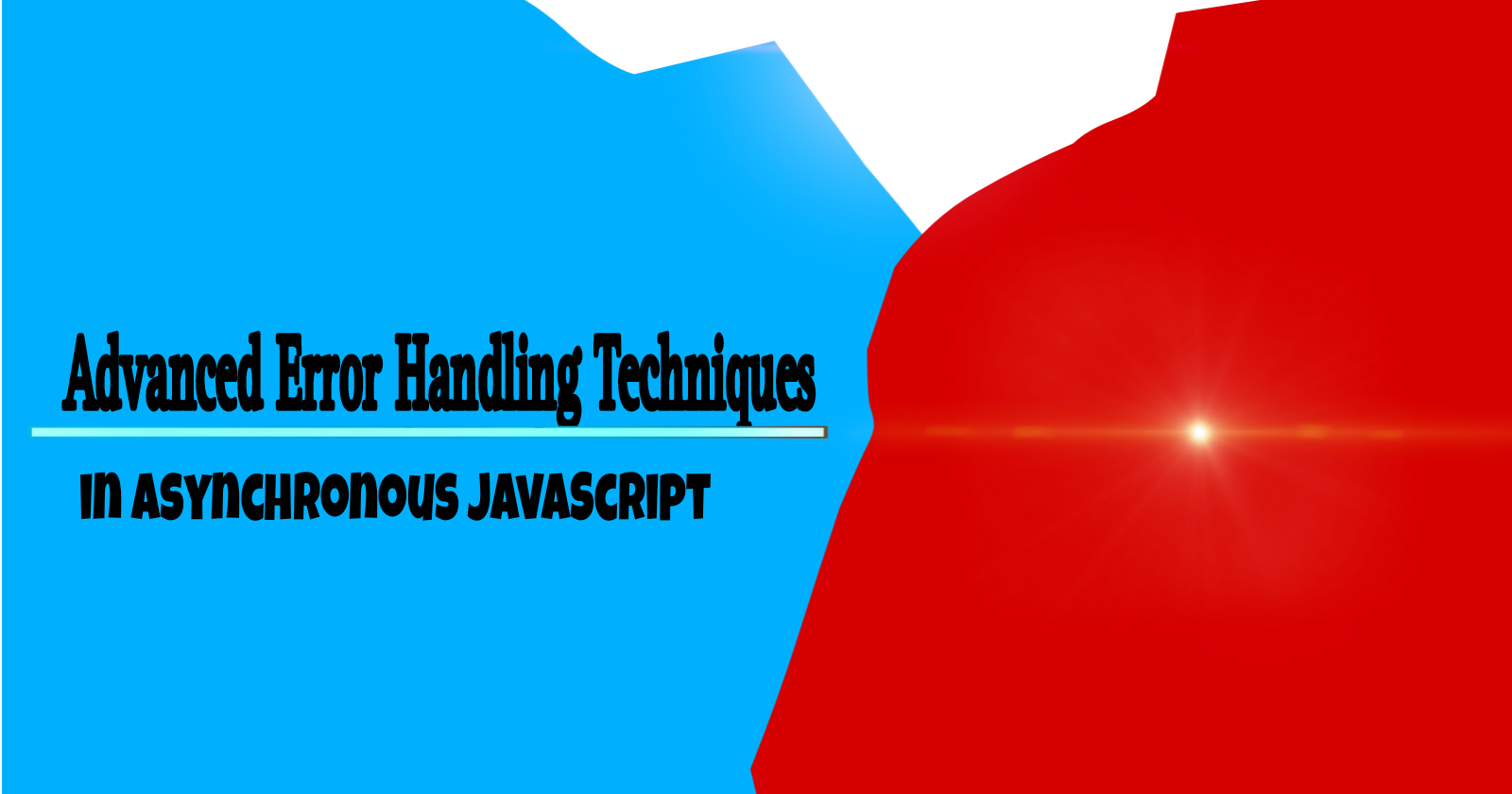
Advanced Error Handling Techniques in Asynchronous JavaScript
Asynchronous JavaScript, with its elegant promises and crafty async/await syntax, feels like the playground of the modern developer. It offers power, efficiency, and a path from the dreaded callback hell. But this power isn't without its pitfalls - errors. Picture this: you're steering a high-speed car on a winding road when suddenly, you hit an unexpected bump; yes, that's the error. If your reaction is delayed or unrefined, it could derail the entire journey. Error handling is the art of navigating these bumps, ensuring your application reaches its destination smoothly.
The Asynchronous Way: Why Handling Errors is Important.
JavaScript, with its non-blocking, event-driven nature, excels in multitasking as in running multiple operations simultaneously without waiting for one to finish before starting another. However, this creates a problem: how do you catch and manage errors when the execution flow isn’t linear? The traditional try-catch blocks won’t always be helpful.
What does this mean? In synchronous code, when an error occurs, it’s like hitting a brick wall; everything stops until you handle the situation. In asynchronous code, mistakes can happen in the background, and if you don’t manage them properly, they might quietly sabotage your entire application; I am sure you wouldn't want that.
The Power of Promises: Handling Errors Like a Pro
Promises represent the eventual completion (or failure) of an asynchronous operation. They offer a chainable, structured way to manage asynchronous operations and, more importantly, handle errors effectively.
const fetch data = () => {
return new Promise((resolve, reject) => {
// Simulating async operation
setTimeout(() => {
const success = Math.random() > 0.5;
if (success) {
resolve("Data fetched successfully!");
} else {
reject("Error fetching data");
}
}, 1000);
});
};
fetchData()
.then(data => console.log(data))
.catch(err => console.error(err));
In the code above, .catch()
captures any error in the promise chain. This method is efficient for handling errors at any stage in the chain.
Dealing with Multiple Promises:
Consider a scenario where multiple asynchronous operations are executed in parallel, and you want to handle errors collectively. Enter Promise. All
.
const promise1 = Promise.resolve('First Promise');
const promise2 = Promise.reject('Second Promise Failed');
const promise3 = Promise.resolve('Third Promise');
Promise.all([promise1, promise2, promise3])
.then(results => console.log(results))
.catch(error => console.error('Error in one of the promises:', error));
Promise.all
will be rejected once one of the promises fails, providing a consolidated way to catch and handle errors.
Async/Await: The Modern Maestro of Error Handling
With async/await,
managing asynchronous code becomes more intuitive, almost resembling synchronous code. Yet, error handling needs the right touch.
async function fetchUserData() {
try {
const data = await fetch data();
console.log(data);
} catch (error) {
console.error('Caught by try-catch:', error);
}
}
fetchUserData();
Here, the traditional try-catch
block reemerges as the knight in shining armor. It wraps the await
call, catching any errors thrown by the promise it awaits.
Avoiding Unhandled Promise Rejections
A common pitfall in asynchronous JavaScript is unhandled promise rejections. They can creep up unexpectedly, causing runtime issues that are hard to trace.
Pro Tip: Always attach a .catch()
to promises or ensure async
functions are wrapped in try-catch
blocks.
// Example of Unhandled Promise Rejection
fetch data()
.then(data => console.log(data));
// Attach a .catch to prevent unhandled rejection
fetch data()
.then(data => console.log(data))
.catch(err => console.error('Handled error:', err));
This precaution prevents silent failures, ensuring you’re always in control of your asynchronous flow.
Real-Life Analogy: Error Handling as a GPS System
This analogy will make you understand better the concept of error handling. Now, Imagine driving with a GPS that reroutes you at every wrong turn. That’s what error handling does in asynchronous JavaScript—it keeps you on track, preventing you from veering off the road into a buggy abyss. Just as you wouldn’t drive without a GPS in unfamiliar territory, you shouldn’t write asynchronous code without robust error handling.
The Advanced Techniques
When simple error handling isn’t enough, you can turn to more sophisticated methods:
Custom Error Classes: Craft your error types to handle specific cases more elegantly.
class CustomError extends Error { constructor(message) { super(message); this.name = 'CustomError'; } } async function risky operation() { try { throw new CustomError('Something went wrong in risky operation); } catch (error) { if (error instance of CustomError) { console.error('Caught a custom error:', error.message); } else { console.error('Caught an unexpected error:', error.message); } } } riskyOperation();
Retry Mechanism: Sometimes, errors are transient, like a temporary network glitch. Implement a retry mechanism to handle such scenarios gracefully.
async function retryFetchData(retries = 3) { for (let i = 0; i < retries; i++) { try { const data = await fetch data(); console.log('Data fetched:', data); return; } catch (error) { console.warn(`Attempt ${i + 1} failed. Retrying...`); } } console.error('All retry attempts failed.'); } retryFetchData();
Error Handling in Parallel vs. Sequential Operations
In asynchronous code, you might handle multiple operations in parallel or sequentially. Understanding the nuances of error handling in each case is crucial.
Parallel Handling:
When operations run in parallel, each error needs to be managed independently. Use Promise.allSettled
to handle resolved and rejected promises without short-circuiting.
const promises = [
Promise.resolve('Resolved'),
Promise.reject('Rejected'),
Promise.resolve('Also Resolved')
];
Promise.allSettled(promises)
.then(results => {
results.forEach((result, index) => {
if (result.status === 'fulfilled') {
console.log(`Promise ${index} fulfilled:`, result.value);
} else {
console.error(`Promise ${index} rejected:`, result.reason);
}
});
});
Sequential Handling:
In sequential execution, errors propagate through the chain. Here, try-catch
within async functions is your best ally.
async function executeTasksSequentially() {
try {
const result1 = await promise1();
console.log('Task 1:', result1);
const result2 = await promise2();
console.log('Task 2:', result2);
const result3 = await promise3();
console.log('Task 3:', result3);
} catch (error) {
console.error('Error during sequential execution:', error);
}
}
executeTasksSequentially();
Conclusion
Advanced error handling in asynchronous JavaScript is not just about catching errors - it's about anticipating them, gracefully recovering, and ensuring your code remains resilient. By mastering these techniques, you will be like a seasoned driver deftly maneuvering through obstacles.
As Grace Hopper once said, "A ship in port is safe, but that's not what ships are built for." Similarly, while writing synchronous code might feel safer, it doesn't unleash the full power of JavaScript. With advanced error-handling techniques, you can confidently set sail into the asynchronous seas, prepared for any challenges.
Subscribe to my newsletter
Read articles from Modefoluwa Adeniyi-Samuel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
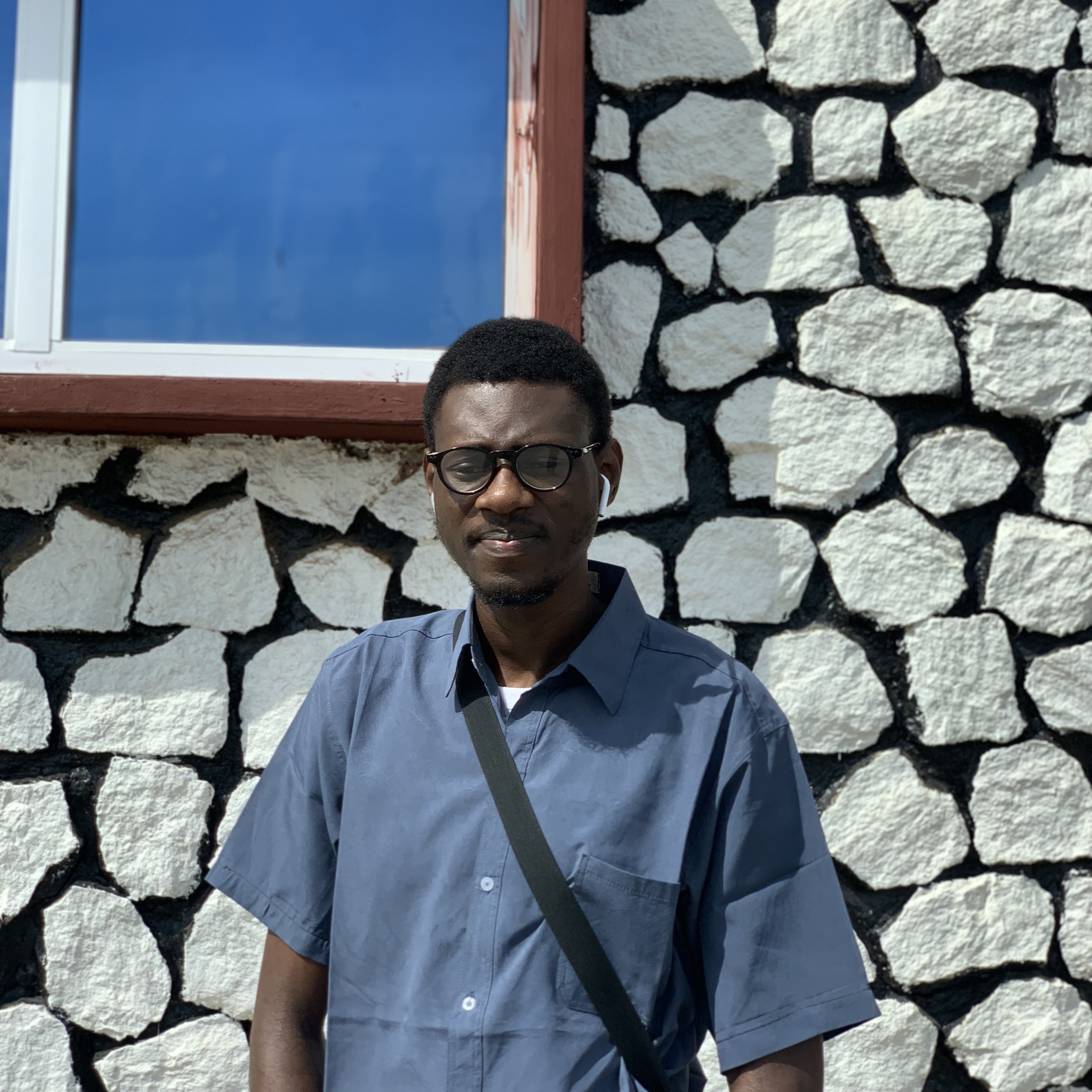
Modefoluwa Adeniyi-Samuel
Modefoluwa Adeniyi-Samuel
Hi there! I'm Modefoluwa, a committed technical writer, Web3 enthusiast, and a Computer Engineering student. With a knack for simplifying complex concepts, I specialize in creating tutorials, guides, and articles that empower developers and non-technical users alike. As a learning full-stack developer, I’m actively expanding my technical skill set to better understand the technologies I write about. My journey started with a love for storytelling, which evolved into crafting impactful content for the ever-evolving tech landscape. When I’m not writing or coding, you’ll find me exploring emerging technologies, brainstorming creative ideas, or engaging with communities that share my love for innovation. My goal? To bridge the gap between technology and its users through clear, concise, and engaging communication - cheers.