Why React Hooks Matter: A Hands-On Guide with useState (Video 5th of react.js series)
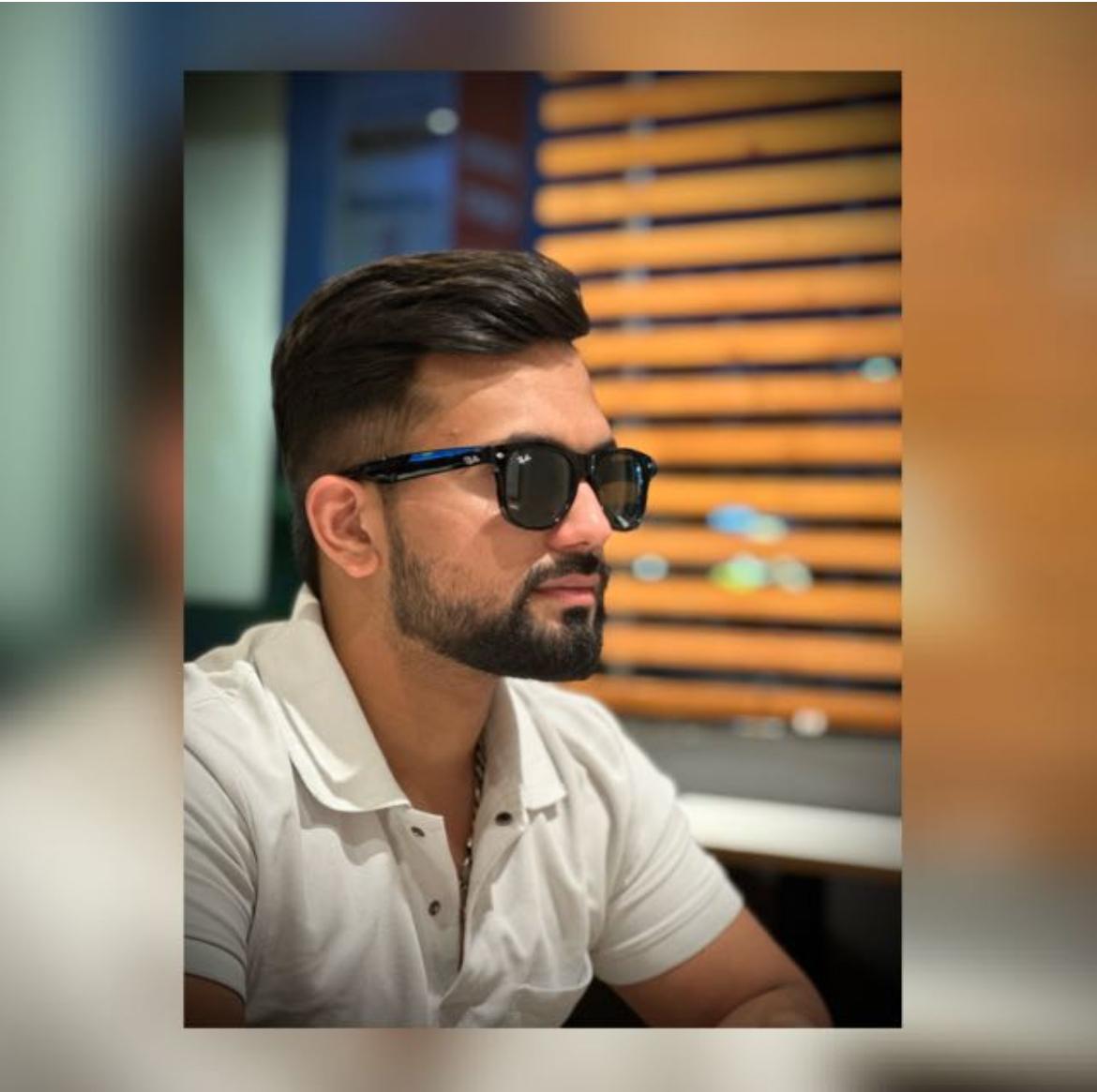
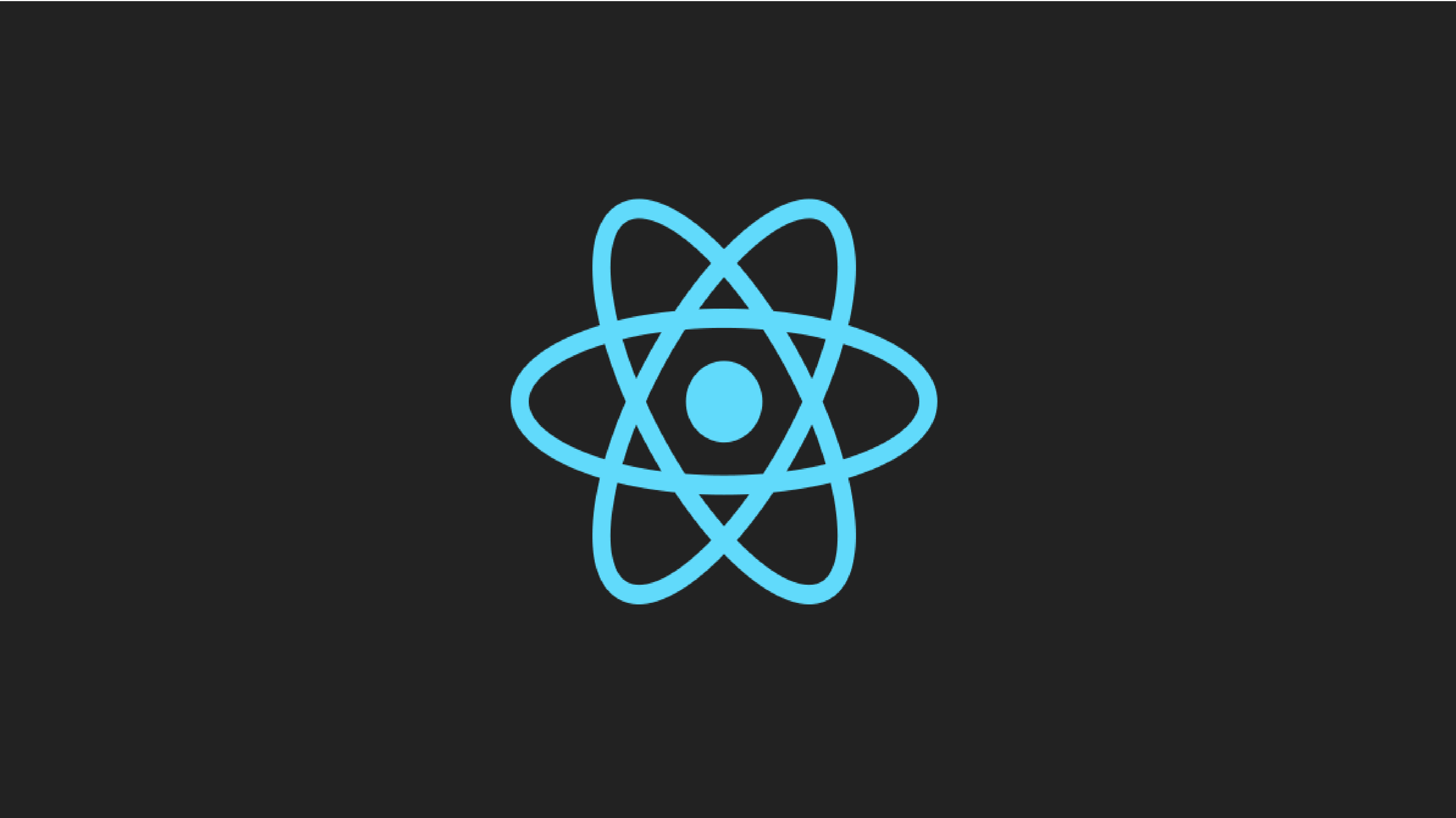
Understanding Hooks in React: My Journey with useState
As I continued my journey into React.js, I reached a crucial point in the learning curve—understanding the need for hooks and where they come into play. In this post, I'll walk you through my experience of learning hooks, specifically the useState
hook, by building a simple project.
The Need for Hooks
While working on a React project, I initially felt confident using the basic tools I had learned. However, as I delved deeper, I encountered a challenge that made me realize why hooks are essential. I decided to create a small project to explore this further.
The Project: A Simple Counter
To better understand hooks, I built a basic counter app. The app has two buttons: one to add value and another to remove value, with the count displayed on the webpage.
Steps I Took:
Creating the Buttons: I added two buttons—one labeled "Add Value" and the other "Remove Value."
Adding Functions: I created two functions:
addValue
andremoveValue
. TheaddValue
function would increment the count, while theremoveValue
function would decrement it.Handling Click Events: I attached the
onClick
attribute to both buttons and passed the corresponding functions (addValue
for the "Add Value" button andremoveValue
for the "Remove Value" button).
The Problem
Once I implemented the basic structure, I noticed that while the count updated in the console, it didn't reflect on the webpage. No matter how many times I clicked the buttons, the displayed count remained static.
The Solution: Using useState
This is when I understood the purpose of the useState
hook. The problem occurred because React wasn't aware that the state had changed. Without the useState
hook, React wouldn’t re-render the component to reflect the updated count.
By using the useState
hook, I could manage the state of the count effectively, ensuring that every time I clicked the buttons, the updated count would be displayed on the webpage.
Implementing useState
:
I imported
useState
from React.Initialized the count state using
useState(0)
.Updated the
addValue
andremoveValue
functions to set the new count using the state setter function.
import { useState } from 'react'
import reactLogo from './assets/react.svg'
import viteLogo from '/vite.svg'
import './App.css'
function App() {
const [counter, setCounter] = useState(0)
const addValue = function(){
if (counter < 20) {
setCounter(counter + 1)
}
}
const removeValue = function(){
if (counter > 0) {
setCounter(counter - 1)
}
}
return (
<>
<h1>Prateek's Counter</h1>
<h2>count : {counter} </h2>
<button
onClick={addValue}>Add value</button>
<br />
<br />
<button
onClick={removeValue}>Remove value</button>
<p>Footer: {counter}</p>
</>
)
}
export default App
Conclusion
This project was a simple yet effective way to grasp the importance of hooks in React. The useState
hook not only helped me manage the state but also made me appreciate how React handles component re-renders efficiently. If you're new to hooks, I highly recommend experimenting with small projects like this—it’s a great way to solidify your understanding.
Subscribe to my newsletter
Read articles from Prateek directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
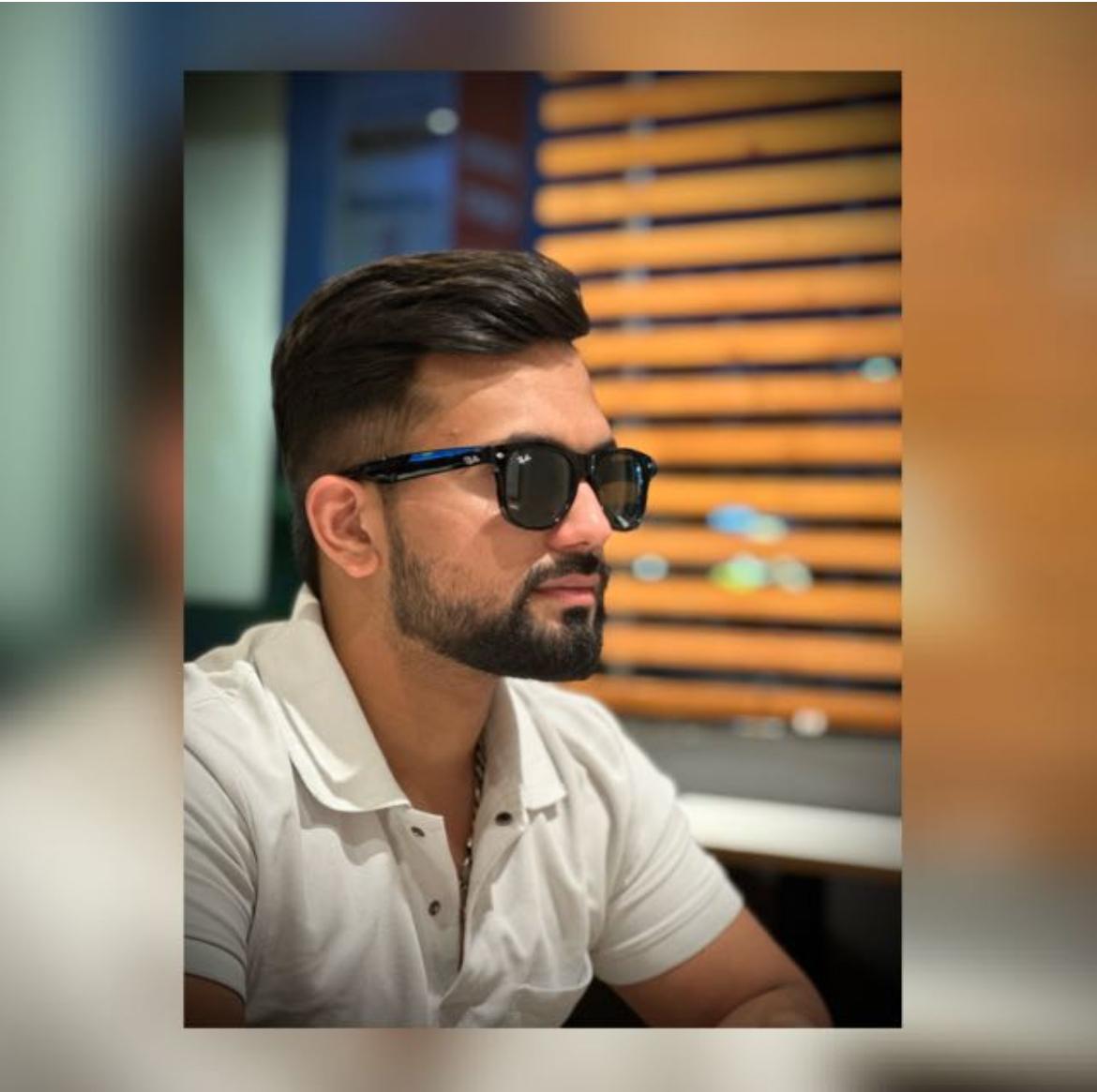