Kubernetes Demystified: A Practical Approach to Container Orchestration and Management

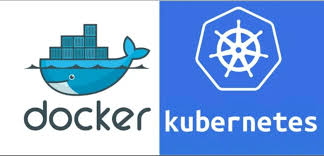
Containerization has revolutionized software development, with Docker leading the charge in making applications more portable and consistent across environments. However, as deployments grow in scale, Docker alone may not be sufficient to manage complex applications efficiently.
This is where Kubernetes steps in, offering powerful tools to orchestrate and manage containers at scale. In this blog, we'll explore how Kubernetes addresses the limitations of Docker, dive into its architecture, and walk through practical examples of deploying and managing containers.
Docker vs. Kubernetes
Challenges with Docker
Docker made containerization accessible and efficient, but it has some notable limitations:
Single Host Issue: Docker operates on a single host. If one container begins to consume excessive resources, it can affect the performance of other containers on the same host, potentially leading to service disruptions.
No Auto-Healing Mechanism: If a Docker container fails, it doesn't automatically restart, leaving applications unavailable until manual intervention occurs.
No Auto-Scaling: Docker doesn't inherently support auto-scaling. While there are third-party tools that can help, Docker lacks a built-in solution to scale containers based on demand.
Lack of Enterprise-Level Support: Docker is minimalistic and lacks the comprehensive support needed for enterprise-level deployments, making it less ideal for complex, large-scale applications.
How Kubernetes Solves These Problems
Kubernetes (K8s) was designed to address these limitations:
Multi-Node Architecture: Kubernetes operates as a cluster, consisting of multiple nodes. If one node is overloaded, Kubernetes can redistribute containers to other nodes, ensuring balanced resource utilization.
Auto-Healing: Kubernetes automatically detects and replaces failed containers, maintaining the desired state and ensuring continuous availability.
Auto-Scaling: Kubernetes can automatically scale containers up or down based on demand, using features like ReplicaSets and Horizontal Pod Autoscalers.
Enterprise-Level Support: Kubernetes is built for scale, providing robust features like role-based access control (RBAC), security policies, and integration with various cloud providers, making it suitable for enterprise deployments.
Kubernetes Architecture
Kubernetes, often abbreviated as K8s (with 8 representing the eight letters between 'K' and 's'), is designed with a modular architecture that consists of two main components:
1. Control Plane
The control plane manages the Kubernetes cluster and consists of the following components:
API Server: The API server is the core component that exposes Kubernetes' functionalities to the outside world. It processes RESTful requests and updates the cluster's state.
etcd: A distributed key-value store that holds the entire configuration and state of the cluster. It stores all cluster-related data securely.
Controller Manager: Manages various controllers that regulate the state of the cluster, such as the ReplicaSet controller, which ensures that the correct number of pods are running.
Scheduler: Allocates resources to different containers by scheduling pods to run on specific nodes based on resource availability and constraints.
Cloud Controller Manager: Interfaces with cloud providers (e.g., AWS, GCP, Azure) to manage resources like load balancers and storage volumes.
2. Data Plane
The data plane executes the operations defined by the control plane and consists of:
Kubelet: A node agent that ensures containers are running in a pod. It monitors the health of the pods and reports back to the control plane.
Container Runtime: The software that runs containers. While Docker is commonly used, Kubernetes also supports other runtimes like containerd and CRI-O.
Kube-proxy: Handles networking within the cluster by managing the routing of traffic between containers and ensuring proper load balancing.
Components in Kubernetes
Kubernetes introduces several key components that make it a robust platform for container orchestration:
Pods
Pods are the smallest deployable units in Kubernetes. A pod encapsulates one or more containers that share the same network and storage resources. Each pod is assigned a unique Cluster IP address, allowing containers within the pod to communicate seamlessly.
Example pod.yaml
File:
apiVersion: v1
kind: Pod
metadata:
name: my-pod
spec:
containers:
- name: my-container
image: nginx:latest
ports:
- containerPort: 80
This pod.yaml
file defines a pod named my-pod
, running a single container based on the nginx:latest
image, which listens on port 80.
ReplicaSets
A ReplicaSet ensures that a specified number of identical pods are running at any time. If a pod fails, the ReplicaSet automatically creates a new one to maintain the desired state.
Deployments
Deployments manage ReplicaSets and offer declarative updates to applications. They allow for easy rollouts and rollbacks of application versions, ensuring that changes can be tested and reverted if necessary.
Example deploy.yaml
File:
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-deployment
spec:
replicas: 3
selector:
matchLabels:
app: my-app
template:
metadata:
labels:
app: my-app
spec:
containers:
- name: my-container
image: nginx:latest
ports:
- containerPort: 80
This deploy.yaml
file defines a deployment named my-deployment
that manages three replicas of the nginx
container. The deployment ensures that at least three instances of the container are always running.
Services
In Kubernetes, a Service is an abstraction that defines a logical set of pods and a policy for accessing them. Services provide a stable network endpoint (IP address and DNS name) that external clients can use to communicate with the pods, even as the set of pods changes over time due to scaling or updates.
Types of Services
Kubernetes offers different types of services based on how they expose the application:
ClusterIP: The default type that exposes the service on an internal IP within the cluster. This service type is accessible only within the cluster and is ideal for communication between different microservices.
NodePort: Exposes the service on each node's IP at a static port. This type of service makes the application accessible outside the cluster using
<NodeIP>:<NodePort>
.LoadBalancer: Exposes the service externally using a cloud provider's load balancer. It automatically provisions a load balancer that distributes traffic to the backend pods.
ExternalName: Maps the service to a DNS name instead of a specific IP. It can be used to reference external services via DNS.
Example service.yaml
File:
Below is an example of a service.yaml
file for a ClusterIP
service:
apiVersion: v1
kind: Service
metadata:
name: my-service
spec:
selector:
app: my-app
ports:
- protocol: TCP
port: 80
targetPort: 8080
type: ClusterIP
This service.yaml
file defines a service named my-service
:
Selector: It targets pods that have the label
app: my-app
. The selector ensures that the service routes traffic to the correct pods.Ports: The service listens on port 80 (
port: 80
) and forwards traffic to port 8080 (targetPort: 8080
) on the selected pods.Type: The service is of type
ClusterIP
, meaning it will only be accessible within the Kubernetes cluster.
How Services Work
When you create a service, Kubernetes assigns it a unique ClusterIP address. Pods that match the service's selector are automatically associated with this service. Even if the underlying pods are replaced, the service's ClusterIP remains the same, providing a consistent interface for clients.
For example, if you have multiple instances of a web application running in different pods, you can create a service that balances traffic between these pods. Clients inside the cluster can then access the service via its ClusterIP, without needing to know the details of the individual pods.
Ingress Controllers
Ingress is an API object that manages external access to services within a Kubernetes cluster, typically HTTP/HTTPS traffic. Ingress allows you to define rules for routing external traffic to your services, enabling features like load balancing, SSL termination, and name-based virtual hosting.
Ingress Controllers are responsible for fulfilling the Ingress API's rules. They watch the Ingress resources and handle the traffic routing as specified in the Ingress objects. Popular Ingress Controllers include NGINX, HAProxy, and Traefik.
Example ingress.yaml
File:
apiVersion: networking.k8s.io/v1
kind: Ingress
metadata:
name: my-ingress
spec:
rules:
- host: myapp.example.com
http:
paths:
- path: /
pathType: Prefix
backend:
service:
name: my-service
port:
number: 80
This ingress.yaml
file defines an Ingress resource named my-ingress
that routes traffic to the service my-service
on port 80. The host
field specifies that this rule applies to requests sent to myapp.example.com
.
Kubernetes Commands Explained
Here are some essential Kubernetes commands and their purposes:
kubectl get pods
: Lists all pods in the current namespace.kubectl get all
: Lists all resources in the current namespace, including pods, services, and deployments.kubectl get pods -w
: Continuously watches and displays updates to pods in real-time.kubectl get pods -o wide
: Displays detailed information about pods, including node placement and IP addresses.kubectl delete pod <pod-name>
: Deletes the specified pod.kubectl describe pod <pod-name>
: Displays detailed information about the specified pod.kubectl get deploy
: Lists all deployments in the current namespace.kubectl get rs
: Lists all ReplicaSets in the current namespace.
Conclusion
Kubernetes has transformed the way we manage and deploy containerized applications. By addressing the limitations of Docker and offering advanced features like auto-scaling, auto-healing, and seamless integration with cloud providers, Kubernetes has become the go-to solution for enterprises looking to scale their applications efficiently.
Whether you're deploying a small microservice or a complex multi-tier application, Kubernetes provides the tools and flexibility needed to confidently manage your infrastructure.
Subscribe to my newsletter
Read articles from Snigdha Chaudhari directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Snigdha Chaudhari
Snigdha Chaudhari
Hi there! 👋 I’m a passionate Cloud Enthusiast and AWS Certified Solutions Architect – Associate, exploring and sharing the limitless possibilities of cloud computing. With hands-on experience in tools like Docker, Kubernetes, Terraform, and Python, I love simplifying complex cloud concepts for developers, tech enthusiasts, and businesses alike. On this blog, you’ll find: 🌟 Insights and tutorials on cloud technologies 🌟 Tips to optimize your DevOps practices 🌟 Stories of overcoming real-world tech challenges When I’m not diving into the cloud, you’ll find me exploring new tools, sharing tech tips, or brainstorming ways to build scalable, efficient infrastructure. Let’s connect, learn, and grow together in this ever-evolving tech space! 🚀