Step-by-Step Guide to Amazing Subarrays on Leetcode

Table of contents
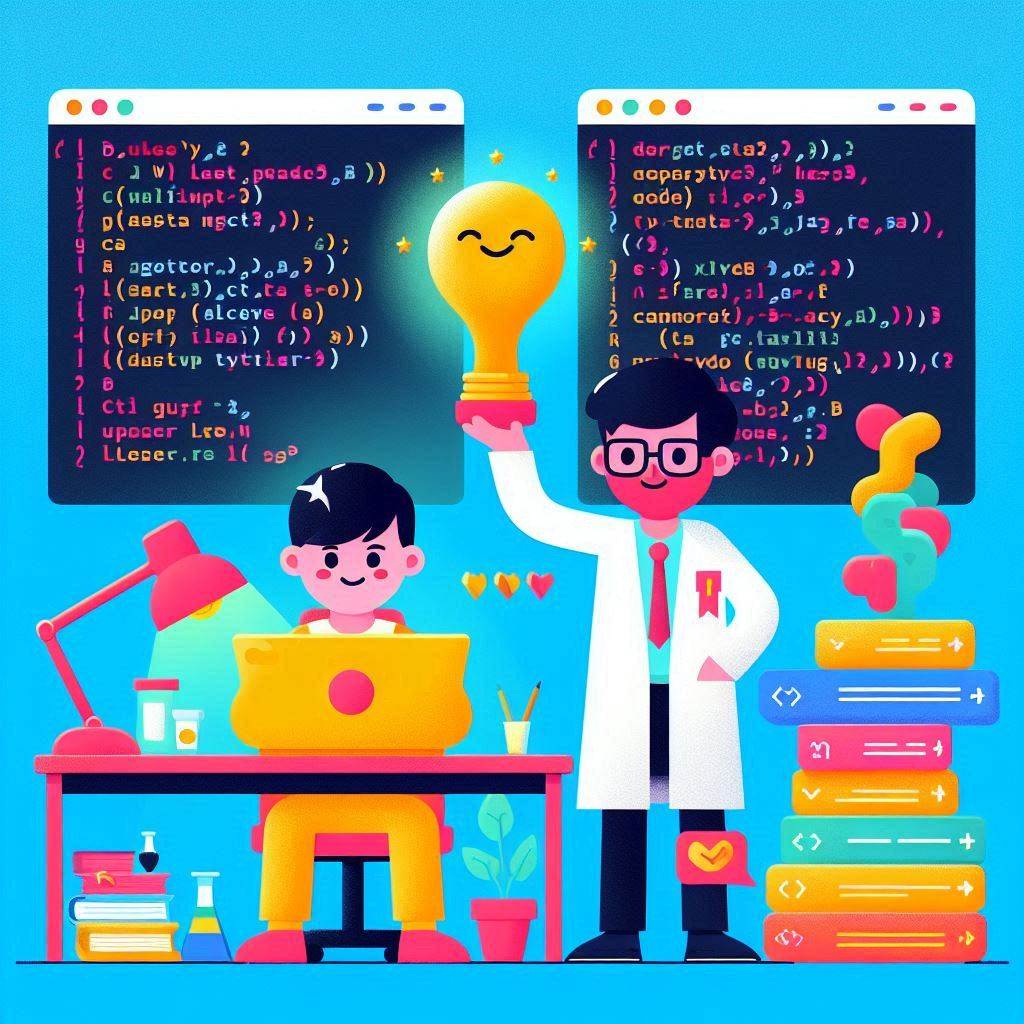
You are given a string S, and your task is to find all the amazing substrings within S.
An amazing substring is defined as any substring that begins with a vowel. The vowels considered for this task include both lowercase and uppercase letters: a, e, i, o, u, A, E, I, O, U.
To elaborate, a substring is any sequence of consecutive characters within the string S. For example, if S is "abc", then the substrings are "a", "b", "c", "ab", "bc", and "abc". Among these, only those starting with a vowel are considered amazing substrings. So, if S is "abc", the amazing substrings would be "a" because it starts with the vowel 'a'.
Your goal is to identify and list all such amazing substrings that start with any of the specified vowels.
Input
Only argument given is string S.
Output
Return a single integer X mod 10003, here X is the number of
Amazing Substrings in given the string.
Constraints
1 <= length(S) <= 1e6
S can have special characters
Example
Input
ABEC
Output
6
Explanation
Amazing substrings of given string are :
1. A
2. AB
3. ABE
4. ABEC
5. E
6. EC
here number of substrings are 6 and 6 % 10003 = 6.
Approach:
public class Solution {
public int solve(String s) {
int totalSubString=0;
int totalLength = s.length();
for(int i=0;i<totalLength;i++){
if(Solution.isVowel(s.charAt(i))){
totalSubString = totalSubString + (totalLength-i);
totalSubString = totalSubString%10003;
}
}
return totalSubString;
}
public static boolean isVowel(char c){
if(c == 'a' || c == 'A' || c == 'e' || c == 'E'
|| c == 'i' || c == 'I' || c == 'o' || c == 'O'
|| c == 'u' || c == 'U'){
return true;
}else{
return false;
}
}
}
Explanation:
int totalSubString = 0;
: This sets up a variable to count the total lengths of substrings that start with a vowel.int totalLength = s.length();
: This gets the total length of the strings
.A
for
loop goes through each character in the strings
:if(Solution.isVowel(s.charAt(i))){
: This checks if the character at the current indexi
is a vowel using theisVowel
method.If the character is a vowel, the code counts the number of substrings that can start with this vowel:
totalSubString = totalSubString + (totalLength - i);
: This adds the number of substrings that start with the current vowel tototalSubString
. The number of substrings starting from a vowel at indexi
is(totalLength - i)
.totalSubString = totalSubString % 10003;
: This takes the modulo 10003 oftotalSubString
to keep the result within bounds.
Finally, the method returns the value of
totalSubString
, which is the desired sum modulo 10003.
This article explains how to find all "amazing substrings" in a given string, where an amazing substring is defined as any substring starting with a vowel (a, e, i, o, u, A, E, I, O, U). The solution involves iterating through the string, identifying vowels, counting possible substrings starting from each vowel, and returning the result modulo 10003. The code and explanation are provided to illustrate the approach.
Subscribe to my newsletter
Read articles from Vishad Patel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
