Automate Tenant and SAML Provider Setup on Google Identity Platform
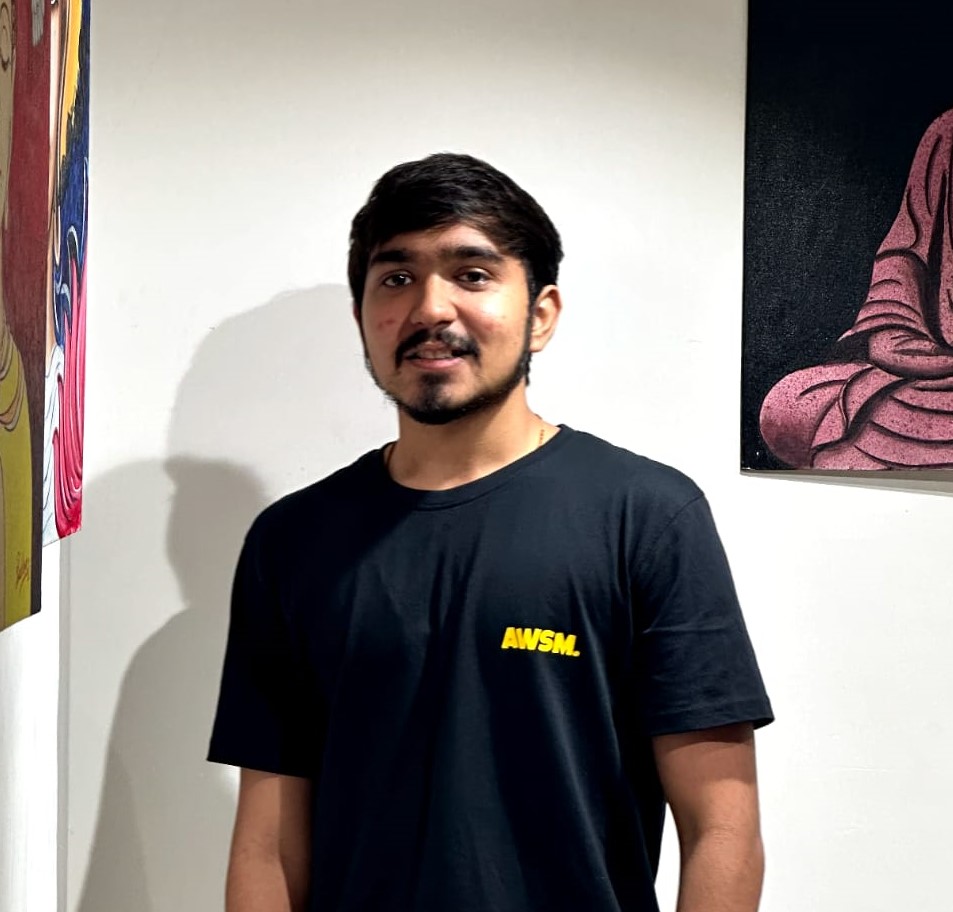
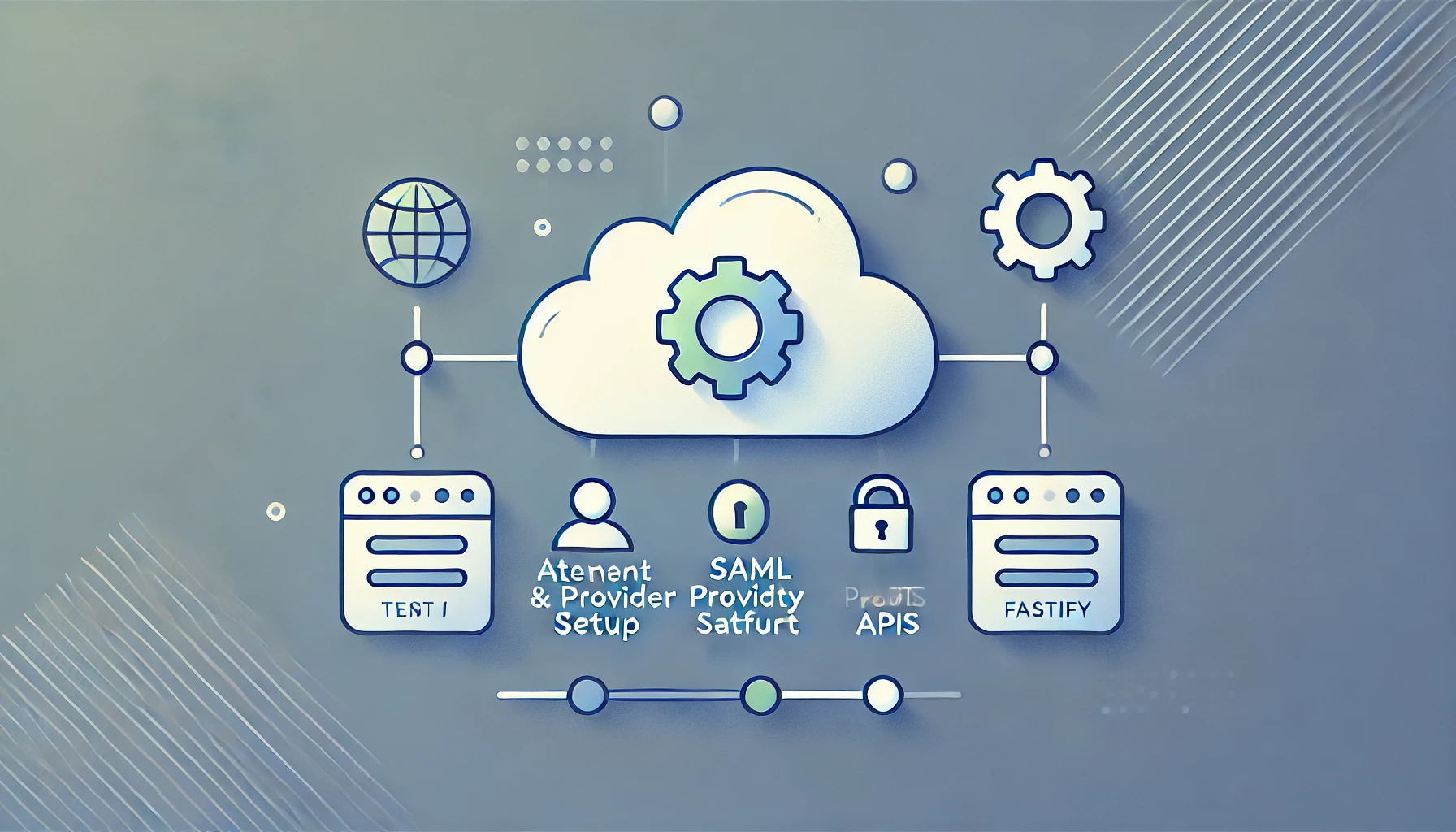
Automating the creation of tenants and SAML providers is a powerful way to streamline user identity management, especially when dealing with multiple clients or tenants in a cloud environment. This blog will guide you through the process of automating tenant creation and SAML provider setup on Google Identity Platform using REST APIs with Node.js and Fastify.
What is SAML and Tenant?
- SAML (Security Assertion Markup Language): A protocol that allows for secure, single sign-on (SSO) authentication by exchanging authentication data between
parties, typically a service provider (SP) and an identity provider (IdP).
- Tenant: A logical grouping in a cloud environment that isolates user data and configurations for different customers or clients, allowing for multi-tenancy.
Why Automate Tenant and SAML Provider Creation?
Automation saves time and reduces the risk of manual errors, especially when dealing with complex identity management tasks. By automating tenant and SAML provider setup, you ensure consistency across all tenants, enhance security, and simplify user management.
Step 1: Setting Up the Environment
Before diving into the code, ensure that your environment is ready:
Node.js and Fastify: Node.js is a popular runtime environment for building scalable server-side applications, while Fastify is a fast, low-overhead web framework for Node.js.
Google Cloud Project: Make sure you have a Google Cloud project with Identity Platform enabled and necessary APIs activated.
Step 2: Authenticating with Google Identity Platform
To interact with Google Cloud services programmatically, you need to authenticate your API requests. Google provides the google-auth-library
for this purpose, which you can integrate into your Node.js application.
const { google } = require('googleapis');
const fastify = require('fastify')({ logger: true });
const auth = new google.auth.GoogleAuth({
scopes: ['https://www.googleapis.com/auth/identitytoolkit'],
});
const client = await auth.getClient();
const identityToolkit = google.identitytoolkit({
version: 'v2',
auth: client,
});
This setup allows your application to securely communicate with Google services.
Step 3: Creating a Tenant
To create a new tenant, you'll use the Identity Toolkit API provided by Google. Here’s how you can create an API that handles tenant creation:
const createTenant = async (customerId) => {
const tenantResponse = await identityToolkit.projects.tenants.create({
parent: `projects/${projectId}`,
requestBody: {
displayName: `${customerId}`,
},
});
return tenantResponse.data;
};
fastify.post('/create-tenant', async (request, reply) => {
const { customerId } = request.body;
try {
const tenantData = await createTenant(customerId);
return { message: 'Tenant created successfully!', tenantId: tenantData.name };
} catch (error) {
return { error: 'Failed to create tenant', details: error.message };
}
});
Step 4: Setting Up the SAML Provider
After the tenant is created, you need to set up a SAML provider for that tenant. Here’s an example of how to achieve this:
const createSAMLProvider = async (tenantId, entityId, ssoUrl, x509Certificate, spEntityId, callbackUri) => {
const samlResponse = await identityToolkit.projects.tenants.inboundSamlConfigs.create({
parent: `projects/${projectId}/tenants/${tenantId}`,
inboundSamlConfigId: `saml.${tenantId}`,
requestBody: {
displayName: `saml.${tenantId}`,
idpConfig: {
idpEntityId: entityId,
ssoUrl: ssoUrl,
idpCertificates: [
{
x509Certificate: x509Certificate,
}
],
signRequest: true,
},
spConfig: {
spEntityId: spEntityId,
callbackUri: callbackUri,
},
enabled: true,
},
});
return samlResponse.data;
};
fastify.post('/create-saml-provider', async (request, reply) => {
const { tenantId, entityId, ssoUrl, x509Certificate, spEntityId, callbackUri } = request.body;
try {
const samlConfig = await createSAMLProvider(tenantId, entityId, ssoUrl, x509Certificate, spEntityId, callbackUri);
return { message: 'SAML Provider created successfully!', samlConfigId: samlConfig.name };
} catch (error) {
return { error: 'Failed to create SAML provider', details: error.message };
}
});
This code sets up a SAML provider based on the details provided by the user.
Step 5: Updating SAML Provider
You may also need to update the SAML provider configurations. Here's how to do that:
const updateSAMLProvider = async (tenantId, configId, entityId, ssoUrl, x509Certificate, spEntityId, callbackUri, spCertificates, displayName, enabled) => {
const samlResponse = await identityToolkit.projects.tenants.inboundSamlConfigs.patch({
name: `projects/${projectId}/tenants/${tenantId}/inboundSamlConfigs/${configId}`,
updateMask: 'idpConfig,spConfig,displayName,enabled',
requestBody: {
name: `projects/${projectId}/tenants/${tenantId}/inboundSamlConfigs/${configId}`,
idpConfig: {
idpEntityId: entityId,
ssoUrl: ssoUrl,
idpCertificates: [
{
x509Certificate: x509Certificate,
}
],
signRequest: true,
},
spConfig: {
spEntityId: spEntityId,
callbackUri: callbackUri,
},
displayName: displayName,
enabled: enabled,
},
});
return samlResponse.data;
};
fastify.put('/update-saml-provider', async (request, reply) => {
const { tenantId, configId, entityId, ssoUrl, x509Certificate, spEntityId, callbackUri, spCertificates, displayName, enabled } = request.body;
try {
const updatedConfig = await updateSAMLProvider(tenantId, configId, entityId, ssoUrl, x509Certificate, spEntityId, callbackUri, spCertificates, displayName, enabled);
return { message: 'SAML Provider updated successfully!', updatedConfigId: updatedConfig.name };
} catch (error) {
return { error: 'Failed to update SAML provider', details: error.message };
}
});
Conclusion
By following these steps, you can automate the creation and management of tenants and SAML providers on Google Identity Platform. This approach not only enhances efficiency but also ensures that your identity management processes are scalable and secure. The use of REST APIs combined with Node.js and Fastify makes the automation process streamlined and easy to integrate into your existing infrastructure.
For more detailed information, refer to the official Google Identity Platform documentation:https://cloud.google.com/identity-platform/docs/reference/rest/v2/projects.tenants.inboundSamlConfigs
By implementing this automated setup, you'll be well-equipped to manage user identities across multiple tenants with ease, setting a solid foundation for scalable and secure identity management in your application.
Subscribe to my newsletter
Read articles from VanshSutariya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
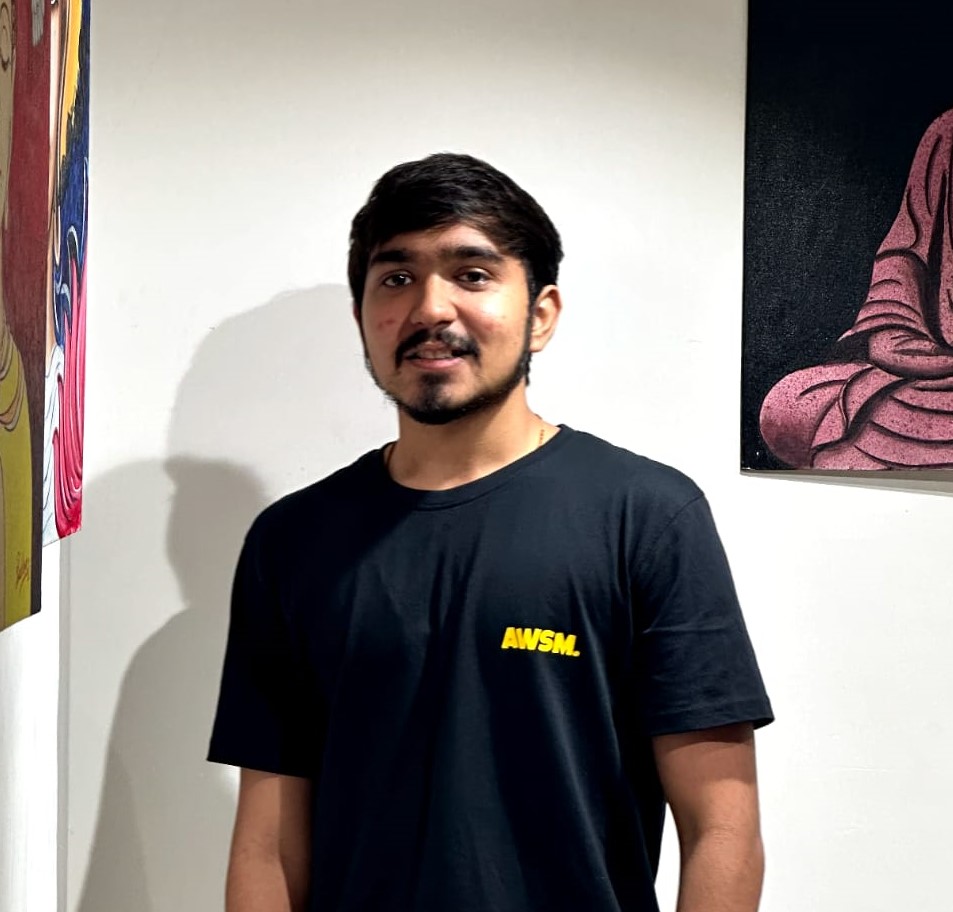
VanshSutariya
VanshSutariya
Full-Stack Developer | Angular, Node.js, Nextjs, NestJS Expert | Crafting Scalable Web Solutions | Firebase & Google Cloud Enthusiast