Handy GoLang Date Time Snippets
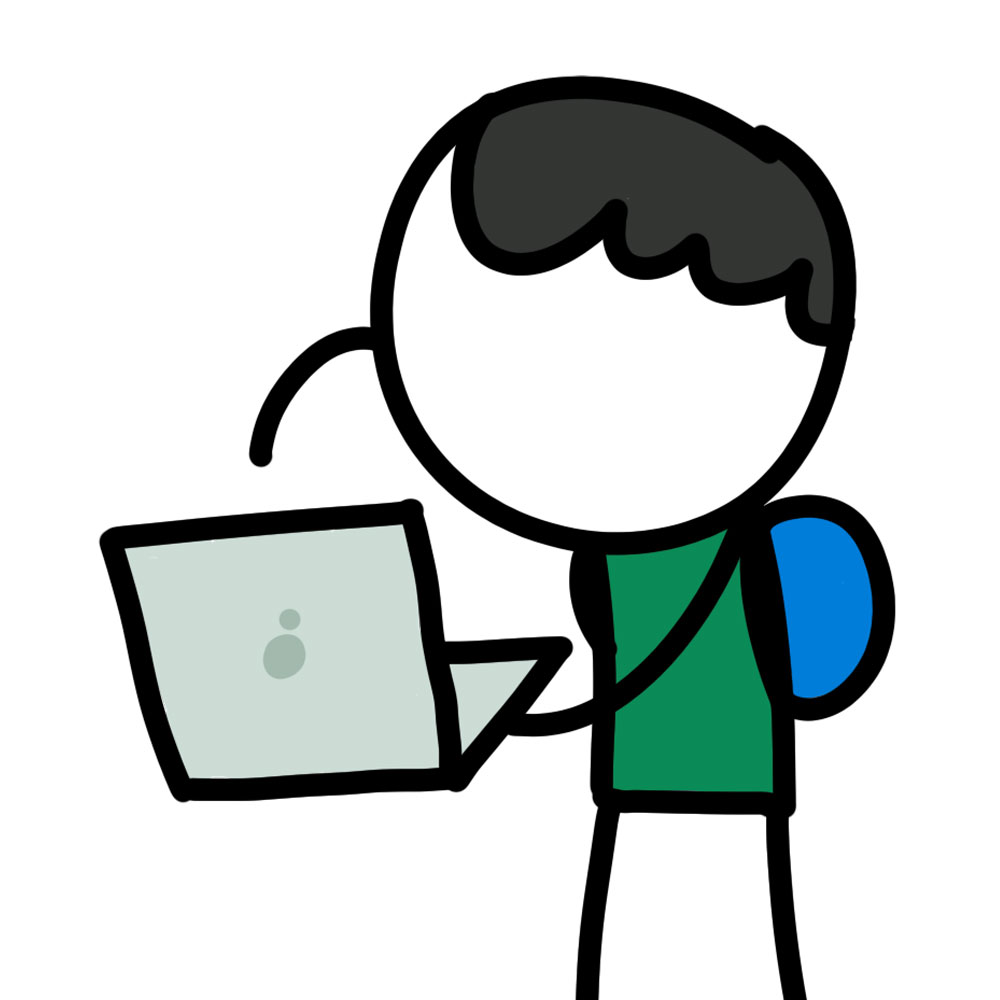
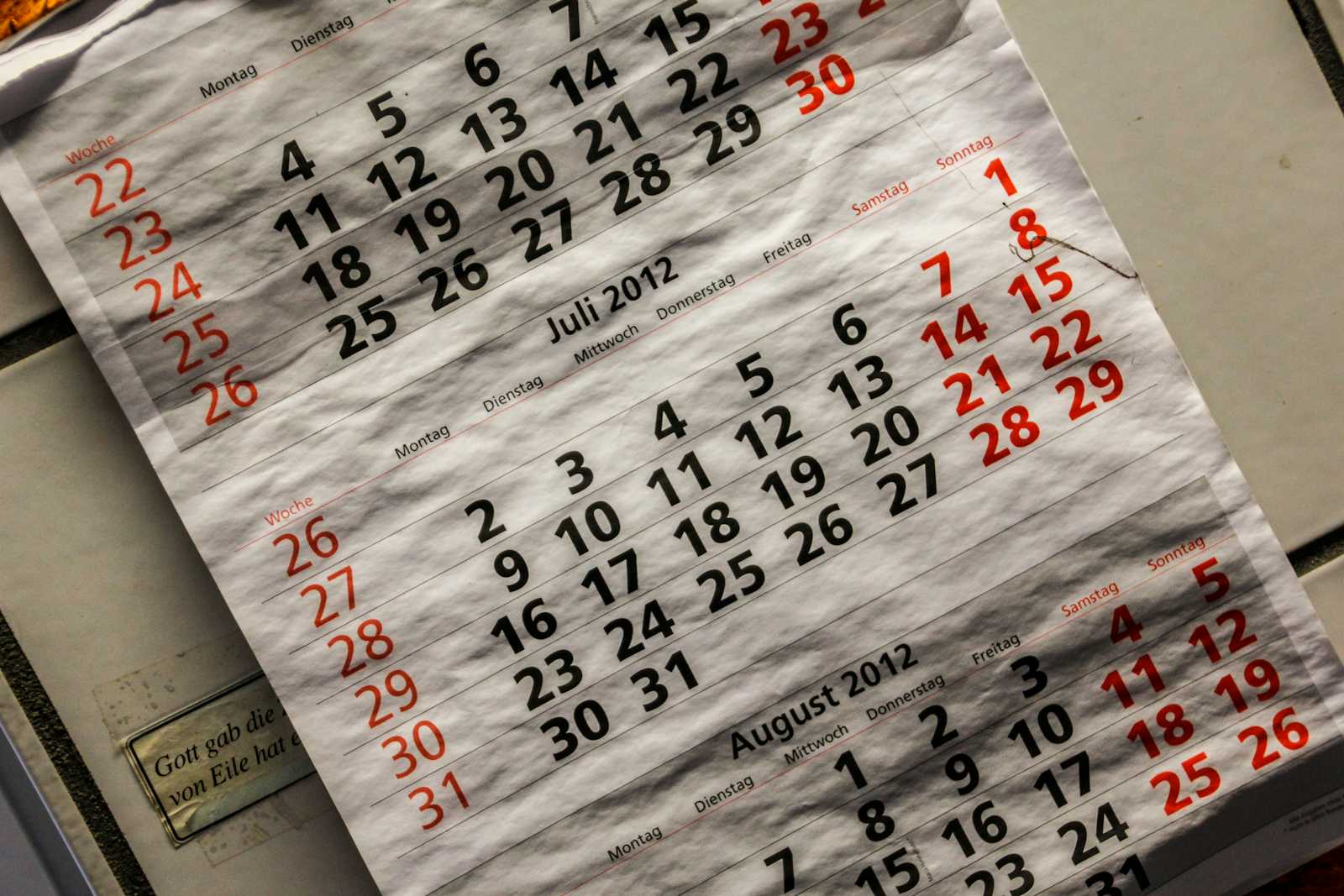
1. Getting the Current Date and Time
currentTime := time.Now()
fmt.Println("Current Time:", currentTime)
// Output: Current Time: 2024-08-10 14:30:05.123456789 +0000 UTC m=+0.000000001
Explanation: Retrieves the current date and time including nanoseconds, in the local time zone. The output includes the year, month, day, hour, minute, second, and nanosecond precision, along with the time zone information.
2. Formatting Date and Time
formattedTime := time.Now().Format("2006-01-02 15:04:05")
fmt.Println("Formatted Time:", formattedTime)
// Output: Formatted Time: 2024-08-10 14:30:05
Explanation: Converts the current date and time into a string using a specific layout. The layout 2006-01-02 15:04:05
is the reference time in Go (January 2, 2006 at 15:04:05), which defines the format.
3. Parsing a Date String into Time
timeStr := "2024-08-10 12:00:00"
parsedTime, err := time.Parse("2006-01-02 15:04:05", timeStr)
if err != nil {
fmt.Println("Error parsing time:", err)
}
fmt.Println("Parsed Time:", parsedTime)
// Output: Parsed Time: 2024-08-10 12:00:00 +0000 UTC
Explanation: Converts a date and time string into a time.Time
object using a specified format layout. The layout must match the format of the input string. Here, "2024-08-10 12:00:00"
is parsed using the layout "2006-01-02 15:04:05"
.
Note that in layout
, the first argument to time.Parse
function, the reference time is predefined and explained in this quote:
Format returns a textual representation of the time value formatted according to layout, which defines the format by showing how the reference time, defined to be Mon Jan 2 15:04:05 -0700 MST 2006 would be displayed if it were the value; it serves as an example of the desired output. The same display rules will then be applied to the time value.
4. Adding Days to a Date
currentTime := time.Now()
futureTime := currentTime.AddDate(0, 0, 5) // Adds 5 days
fmt.Println("Future Time:", futureTime)
// Output: Future Time: 2024-08-15 14:30:05.123456789 +0000 UTC m=+432000.000000001
Explanation: Adds 5 days to the current date and time using AddDate(years, months, days)
. Here, 0, 0, 5
indicates adding 0 years, 0 months, and 5 days to the current date. (Hint: To subtract days, use a negative value like -5
.)
5. Adding Hours to a Date
currentTime := time.Now()
futureTime := currentTime.Add(10 * time.Hour)
fmt.Println("Future Time:", futureTime)
// Output: Future Time: 2024-08-10 24:30:05.123456789 +0000 UTC m=+36000.000000001
Explanation: Adds 10 hours to the current time using the Add
method. The time.Hour
constant represents one hour, so 10 * time.Hour
adds 10 hours. (Hint: To subtract hours, use a negative value like -5 * time.Hour
.)
6. Getting the Difference Between Two Dates
// time.Date(year, month, day, hour, minute, second, nanosecond, location)
time1 := time.Date(2024, 8, 10, 12, 0, 0, 0, time.UTC)
time2 := time.Date(2024, 8, 15, 12, 0, 0, 0, time.UTC)
duration := time2.Sub(time1)
fmt.Println("Difference in Days:", duration.Hours()/24)
// Output: Difference in Days: 5
Explanation: Calculates the difference between two dates using the Sub
method, which returns a Duration
. The difference is then converted to days by dividing the duration in hours by 24.
7. Comparing Dates: Before and After
time1 := time.Date(2024, 8, 10, 12, 0, 0, 0, time.UTC)
time2 := time.Date(2024, 8, 15, 12, 0, 0, 0, time.UTC)
isBefore := time1.Before(time2)
isAfter := time2.After(time1)
fmt.Println("Is time1 before time2?", isBefore)
fmt.Println("Is time2 after time1?", isAfter)
// Output:
// Is time1 before time2? true
// Is time2 after time1? true
Explanation: Checks if one date is before or after another date using the Before
and After
methods, both of which return boolean values.
8. Creating a Date from Year, Month, Day
date := time.Date(2024, time.August, 10, 0, 0, 0, 0, time.UTC)
fmt.Println("Created Date:", date)
// Output: Created Date: 2024-08-10 00:00:00 +0000 UTC
Explanation: Creates a specific date by specifying the year, month, day, hour, minute, second, nanosecond, and time zone. Here, the date is set to August 10, 2024, at midnight UTC.
9. Extracting the Year, Month, Day from a Date
year, month, day := time.Now().Date()
fmt.Printf("Year: %d, Month: %s, Day: %d\n", year, month, day)
// Output: Year: 2024, Month: August, Day: 10
Explanation: Extracts the year, month, and day components from the current date using the Date
method.
10. Extracting the Hour, Minute, Second from a Time
hour, minute, second := time.Now().Clock()
fmt.Printf("Hour: %d, Minute: %d, Second: %d\n", hour, minute, second)
// Output: Hour: 14, Minute: 30, Second: 5
Explanation: Extracts the hour, minute, and second components from the current time using the Clock
method.
11. Getting the Current Time in UTC
utcTime := time.Now().UTC()
fmt.Println("Current Time in UTC:", utcTime)
// Output: Current Time in UTC: 2024-08-10 14:30:05.123456789 +0000 UTC
Explanation: Converts the current local time to UTC (Coordinated Universal Time) using the UTC
method.
12. Converting UTC Time to Local Time
utcTime := time.Now().UTC()
localTime := utcTime.Local()
fmt.Println("Local Time:", localTime)
// Output: Local Time: 2024-08-10 10:30:05.123456789 -0400 EDT
Explanation: Converts a UTC time to the local time zone using the Local
method, adjusting the time according to the local time zone offset.
13. Parsing Time in a Different Time Zone
timeStr := "2024-08-10 12:00:00"
location, _ := time.LoadLocation("America/New_York")
parsedTime, err := time.ParseInLocation("2006-01-02 15:04:05", timeStr, location)
fmt.Println("Parsed Time in New York:", parsedTime)
// Output: Parsed Time in New York: 2024-08-10 12:00:00 -0400 EDT
Explanation: Parses a date string in a specified time zone using ParseInLocation
, which requires the time format, the time string, and the location. This example converts the time string into the time in New York.
14. Calculating the Beginning of the Week
currentTime := time.Now()
weekday := currentTime.Weekday()
startOfWeek := currentTime.AddDate(0, 0, -int(weekday))
fmt.Println("Start of the Week:", startOfWeek)
// Output: Start of the Week: 2024-08-04 14:30:05.123456789 +0000 UTC
Explanation: Calculates the start of the current week (Sunday) by subtracting the number of days since the beginning of the week from the current date.
15. Calculating the Time Until a Specific Date
futureTime := time.Date(2024, 12, 31, 0, 0, 0, 0, time.UTC)
duration := time.Until(futureTime)
fmt.Printf("Time until New Year: %v\n", duration)
// Output: Time until New Year: 5094h29m54.876543211s
Explanation: Calculates the duration until a specific future date using the Until
function, which returns a Duration
representing the time remaining.
16. Checking if a Year is a Leap Year
year := 2024
isLeapYear := time.Date(year, 2, 29, 0, 0, 0, 0, time.UTC).Day() == 29
fmt.Printf("Is %d a leap year? %v\n", year, isLeapYear)
// Output: Is 2024 a leap year? true
Certainly! Let's continue from where we left off and add snippets for converting to and from Unix time, including examples for seconds, microseconds, and nanoseconds.
17. Converting Time to Unix Timestamp (Seconds)
currentTime := time.Now()
unixTimestamp := currentTime.Unix()
fmt.Println("Unix Timestamp (Seconds):", unixTimestamp)
// Output: Unix Timestamp (Seconds): 1723319405
Explanation: Converts the current time to a Unix timestamp, represented as the number of seconds that have elapsed since January 1, 1970 (the Unix epoch).
18. Converting Unix Timestamp (Seconds) to Time
unixTimestamp := int64(1723319405)
timeFromUnix := time.Unix(unixTimestamp, 0)
fmt.Println("Time from Unix Timestamp:", timeFromUnix)
// Output: Time from Unix Timestamp: 2024-08-10 14:30:05 +0000 UTC
Explanation: Converts a Unix timestamp in seconds back to a time.Time
object. The second argument (0
) is the nanosecond part, which is not used here.
19. Converting Time to Unix Timestamp (Microseconds)
currentTime := time.Now()
unixMicroseconds := currentTime.UnixMicro()
fmt.Println("Unix Timestamp (Microseconds):", unixMicroseconds)
// Output: Unix Timestamp (Microseconds): 1723319405123456
Explanation: Converts the current time to a Unix timestamp in microseconds. This provides higher precision than seconds but less than nanoseconds.
20. Converting Unix Timestamp (Microseconds) to Time
unixMicroseconds := int64(1723319405123456)
timeFromUnixMicro := time.Unix(0, unixMicroseconds*1000)
fmt.Println("Time from Unix Microseconds:", timeFromUnixMicro)
// Output: Time from Unix Microseconds: 2024-08-10 14:30:05.123456 +0000 UTC
Explanation: Converts a Unix timestamp in microseconds back to a time.Time
object. The first argument is the seconds part (0
here), and the second argument is the nanoseconds, obtained by multiplying microseconds by 1000.
21. Converting Time to Unix Timestamp (Nanoseconds)
currentTime := time.Now()
unixNanoseconds := currentTime.UnixNano()
fmt.Println("Unix Timestamp (Nanoseconds):", unixNanoseconds)
// Output: Unix Timestamp (Nanoseconds): 1723319405123456789
Explanation: Converts the current time to a Unix timestamp in nanoseconds. This provides the highest precision for representing time.
22. Converting Unix Timestamp (Nanoseconds) to Time
unixNanoseconds := int64(1723319405123456789)
timeFromUnixNano := time.Unix(0, unixNanoseconds)
fmt.Println("Time from Unix Nanoseconds:", timeFromUnixNano)
// Output: Time from Unix Nanoseconds: 2024-08-10 14:30:05.123456789 +0000 UTC
Explanation: Converts a Unix timestamp in nanoseconds back to a time.Time
object. The first argument is the seconds part (0
here), and the second argument is the nanoseconds directly.
23. Profiling Execution Time
Snippet 1: Measuring Function Execution Time
start := time.Now() // Start timing
longRunningTask()
duration := time.Since(start) // Calculate duration
fmt.Printf("Execution time: %v\n", duration)
// Output: Execution time: 2.000123456s
Explanation: Captures the start time before executing a function (longRunningTask
). After the function completes, time.Since(start)
computes the duration elapsed since the start, providing the total execution time.
Snippet 2: Using defer
to Automatically Measure Execution Time
func measureExecutionTime(start time.Time) {
duration := time.Since(start)
fmt.Printf("Execution time: %v\n", duration)
}
func longRunningTask() {
defer measureExecutionTime(time.Now()) // Measure execution time
time.Sleep(2 * time.Second) // Simulates a long task
}
Explanation: Utilizes defer
to automatically call measureExecutionTime
when longRunningTask
finishes. time.Now()
captures the start time, and defer
ensures that measureExecutionTime
runs at the end of the function, measuring how long the task took to complete.
Subscribe to my newsletter
Read articles from Omkar Bhagat directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
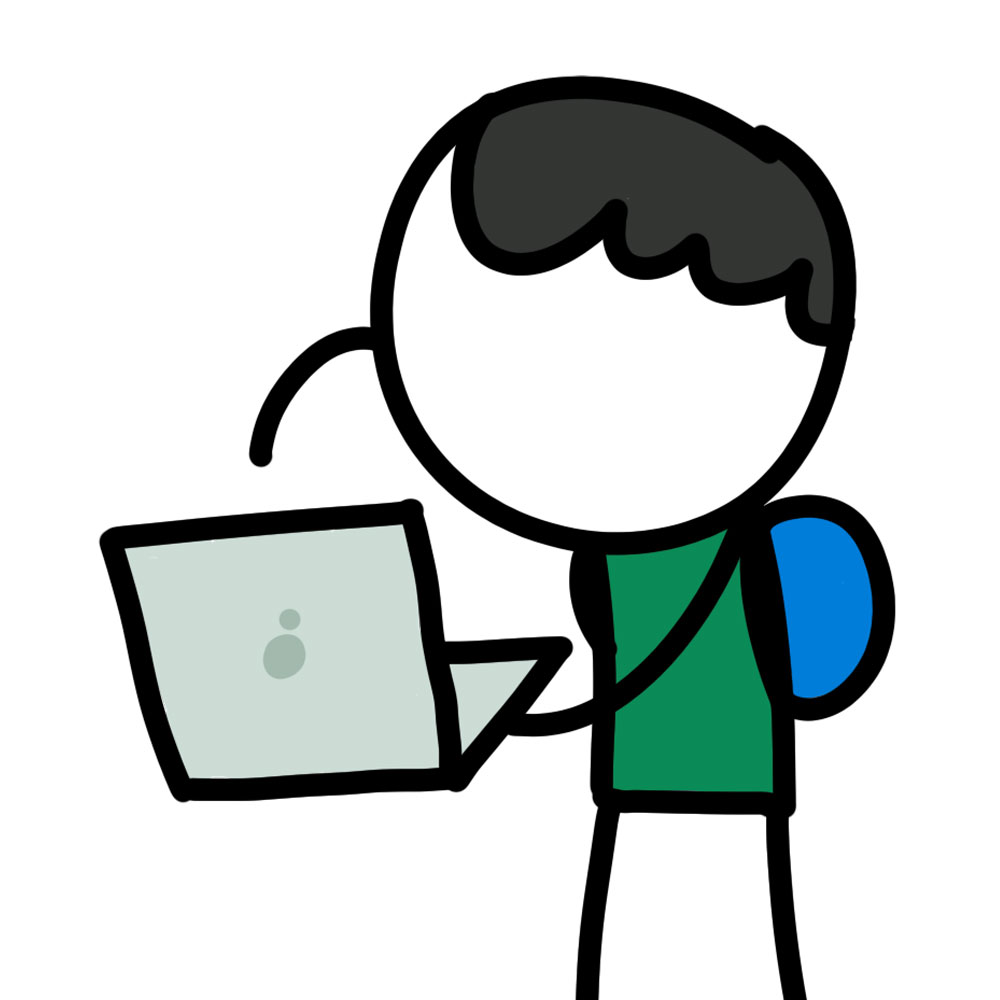
Omkar Bhagat
Omkar Bhagat
I am a Site Reliability Engineer at Google Ireland