Lambda: The Single-line Function

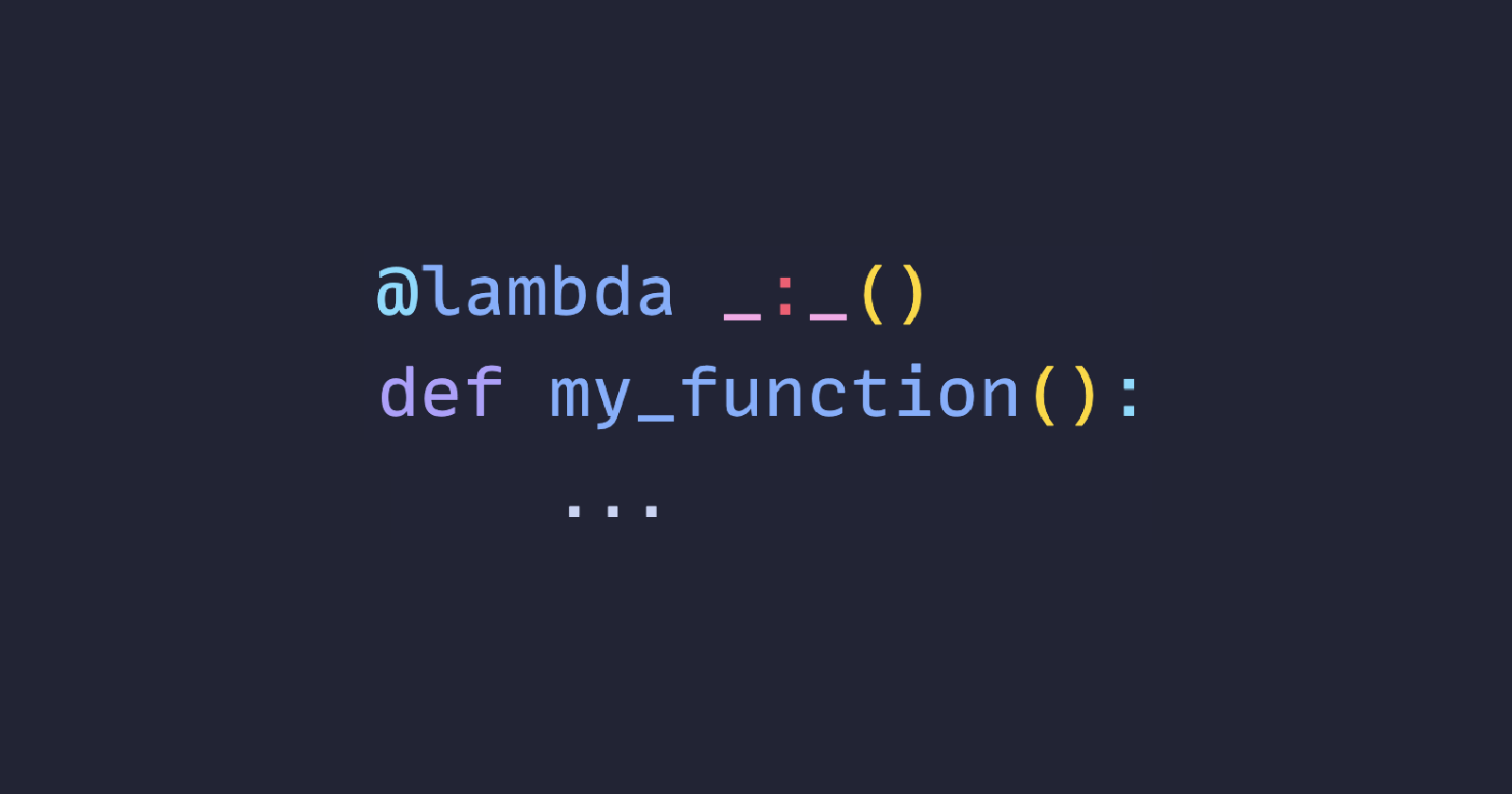
Lambda functions are an essential feature in Python, allowing developers to create small, anonymous functions without formally defining them using the def
keyword. These functions are typically used in situations where a small, throwaway function is needed for a short period.
What is a Lambda Function?
In Python, a lambda function is a small function defined using the lambda
keyword. The syntax for a lambda function is straightforward:
lambda arguments: expression
Arguments: The input parameters to the function.
Expression: The single expression that is evaluated and returned by the function.
A simple example is:
add = lambda x, y: x + y
print(add(2, 3)) # Output: 5
Here, lambda x, y: x + y
creates a function that takes two arguments, x
and y
, and returns their sum.
This simple lambda function is equivalent to the following def
function in Python:
def add(x, y):
return x + y
When to Use Lambda Functions?
Lambda functions are best used in scenarios where a small, simple function is required temporarily, especially when passing functions as arguments to higher-order functions like map()
and filter()
.
Common Use Cases
Using Lambda with
map()
:numbers = [1, 2, 3, 4] squared = list(map(lambda x: x ** 2, numbers)) print(squared) # Output: [1, 4, 9, 16]
Using Lambda with
filter()
:numbers = [1, 2, 3, 4, 5, 6] evens = list(filter(lambda x: x % 2 == 0, numbers)) print(evens) # Output: [2, 4, 6]
Using Lambda with
sorted()
:points = [(2, 3), (1, 2), (3, 1)] sorted_points = sorted(points, key=lambda point: point[1]) print(sorted_points) # Output: [(3, 1), (1, 2), (2, 3)]
Best Practices for Using Lambda Functions
While lambda functions can be a powerful tool, they are not without their limitations and potential pitfalls. Following best practices can help you avoid common issues.
Keep Lambda Functions Simple: Lambda functions should be simple and concise. If the function requires more than one expression or is complex, it's better to use a standard function defined with
def
.# Good increment = lambda x: x + 1 # Bad complex_lambda = lambda x: (x + 1, x ** 2) # Better as a regular function
Use Descriptive Names: When assigning lambda functions to variables, use descriptive names to indicate the purpose of the function. Just like a normal function.
multiply_by_two = lambda x: x * 2
Avoid Overusing Lambda Functions: While lambda functions can make code more concise, overuse can lead to code that is difficult to read and maintain. Use them judiciously and prefer regular functions when the logic is complex.
Use Lambdas in Context: Lambda functions shine when used in context, such as within
map()
,filter()
, orsorted()
. If the lambda is being assigned to a variable and used multiple times, consider defining a proper function.
Worst Practices with Lambda Functions
Complex Lambda Expressions: A lambda function should only contain a single expression. Using complex logic within a lambda function can make the code unreadable.
# Bad practice func = lambda x: x if x > 10 else x + 10 if x > 5 else x + 5
Ignoring Readability: Lambda functions can sometimes obscure the meaning of the code, especially if overused or if they contain nested lambdas.
# Hard to read result = (lambda x: (lambda y: x + y)(10))(5)
Using Lambdas Where
def
is Clearer: If a lambda function spans multiple lines or performs multiple operations, using a standard function definition is more appropriate.# Better as a regular function def add_and_square(x, y): return (x + y) ** 2
Advanced Usage of Lambda Functions
While lambda functions are typically used for simple tasks, there are some advanced use cases where they can be applied creatively and effectively.
Lambda with Higher-Order Functions: Lambda functions are often used in conjunction with higher-order functions that take other functions as arguments. It's quite similar to decorators, isn't it?!
def apply_function(func, value): return func(value) result = apply_function(lambda x: x ** 2, 4) print(result) # Output: 16
Lambda with
reduce()
: Thereduce()
function applies a lambda function cumulatively to the items of a sequence.from functools import reduce numbers = [1, 2, 3, 4] product = reduce(lambda x, y: x * y, numbers) print(product) # Output: 24
Lambda in Key Functions: Lambdas are often used as the key argument in functions like
sorted()
ormin()
to determine the sorting or selection criteria.names = ['Alice', 'Bob', 'Charlie'] longest_name = max(names, key=lambda name: len(name)) print(longest_name) # Output: Charlie
Currying with Lambda Functions: Lambda functions can be used to create curried functions, where a function with multiple arguments is transformed into a series of functions each with a single argument.
def multiply(x): return lambda y: x * y double = multiply(2) print(double(5)) # Output: 10
Using to Define Immediately Invoked Functions (IIFs): Some functions get invoked as they get defined. They are so popular in Javascript. In Python, there is a chance to define these types of functions using lambda functions.
@lambda _:_() def my_iif(): print("Don't call me. I'm already invoked!")
Conclusion
Lambda functions are a versatile and powerful feature in Python, ideal for situations where you need a small, one-off function. While they can lead to more concise code, using them wisely is essential to maintain readability and avoid complexity. Understanding when and how to use lambda functions effectively can enhance your Python programming skills and make your code more expressive and functional.
Subscribe to my newsletter
Read articles from Sadra Yahyapour directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Sadra Yahyapour
Sadra Yahyapour
A passionate software developer who enjoys sharing his thoughts with others and learning from them vise versa!