Building Your First Full-Stack Application
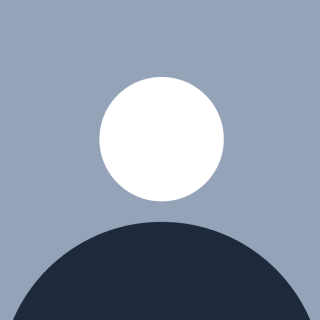
Table of contents

ChatGPT
Memory updated
Building Your First Full-Stack Application
So, you’ve decided to build your first full-stack application. Whether you're a beginner or have some coding experience, this journey is both challenging and rewarding. But, before we dive into the how-to, let’s clarify what a full-stack application is.
What is a Full-Stack Application?
A full-stack application is a web application that has both a front-end (client-side) and a back-end (server-side). The front-end is what users interact with, while the back-end processes requests, handles the database, and performs other essential operations. As a full-stack developer, you're responsible for building and maintaining both parts.
Why Build a Full-Stack Application?
Creating a full-stack application allows you to understand how the front-end and back-end work together. It’s a crucial skill for any developer, as it gives you the flexibility to work on both sides of web development.
Step 1: Setting Up Your Development Environment
Before you start coding, you need to set up your development environment. Here’s what you’ll need:
1. Code Editor
- VS Code is the most popular choice among developers due to its vast extensions and customization options. Download VS Code.
2. Version Control System
- Git helps you track changes and collaborate with others. It’s essential for any project. Install Git.
3. Package Manager
- npm (Node Package Manager) comes with Node.js and allows you to install libraries and frameworks quickly. Install Node.js to get npm.
Step 2: Choosing Your Tech Stack
A tech stack is the combination of technologies you use to build your application. Here’s a common stack you might consider:
Front-End: React.js
Back-End: Node.js with Express
Database: MongoDB
These technologies are popular for their ease of use and extensive documentation.
Step 3: Creating the Front-End
Let’s start by setting up the front-end using React.js.
1. Initialize Your React Project
Open your terminal and run:
bashCopy codenpx create-react-app my-fullstack-app
cd my-fullstack-app
This command will set up a basic React project for you.
2. Build Your Components
In React, everything is a component. Start by creating a simple component in src/App.js
:
javascriptCopy codeimport React from 'react';
function App() {
return (
<div className="App">
<h1>Hello, Full-Stack World!</h1>
</div>
);
}
export default App;
This will render a basic "Hello, Full-Stack World!" message in your browser.
3. Styling Your Components
You can style your components using CSS or a styling library like styled-components. For now, let’s stick with basic CSS.
cssCopy code/* src/App.css */
.App {
text-align: center;
font-family: Arial, sans-serif;
}
h1 {
color: #333;
}
Step 4: Building the Back-End
Now that you have a basic front-end, it’s time to build the back-end using Node.js and Express.
1. Set Up Express
Create a new directory for your back-end and initialize a new Node.js project:
bashCopy codemkdir backend
cd backend
npm init -y
npm install express
2. Create the Server
In your backend
directory, create a server.js
file:
javascriptCopy codeconst express = require('express');
const app = express();
const PORT = process.env.PORT || 5000;
app.get('/', (req, res) => {
res.send('Hello from the back-end!');
});
app.listen(PORT, () => {
console.log(Server is running on http://localhost:<span class="hljs-subst">${PORT}</span>);
});
Run the server using:
bashCopy codenode server.js
You should see "Hello from the back-end!" when you navigate to http://localhost:5000
.
Step 5: Connecting Front-End and Back-End
To connect your front-end with the back-end, you'll need to make HTTP requests from React to Express. Let’s use the axios
library for this.
1. Install Axios
In your React project, install axios
:
bashCopy codenpm install axios
2. Fetch Data from the Back-End
Update your App.js
to fetch data from the back-end:
javascriptCopy codeimport React, { useEffect, useState } from 'react';
import axios from 'axios';
function App() {
const [message, setMessage] = useState('');
useEffect(() => {
axios.get('http://localhost:5000/')
.then(response => {
setMessage(response.data);
})
.catch(error => {
console.error('Error fetching data:', error);
});
}, []);
return (
<div className="App">
<h1>{message}</h1>
</div>
);
}
export default App;
When you run your React application, it should now display the message from your back-end.
Step 6: Deploying Your Application
Once your application is working locally, it’s time to deploy it so others can use it. Here are some popular platforms:
Heroku: Great for quick and easy deployment of full-stack applications.
Netlify: Ideal for deploying static sites, but can also handle full-stack apps with serverless functions.
Vercel: Perfect for Next.js and React applications, with easy integration and automatic deployments.
Follow the documentation on these platforms to deploy your application.
Final Thoughts
Building your first full-stack application is a significant milestone. It not only helps you understand the different parts of web development but also equips you with the skills to build complex applications in the future. As you continue to develop your skills, remember that consistency and practice are key.
And if you're looking to boost the visibility of your developer content, whether it’s on YouTube or your website, consider getting professional help from Mediageneous. They provide trusted services to enhance your online presence, ensuring your hard work gets the attention it deserves.
Happy coding!
4o
Subscribe to my newsletter
Read articles from MediaGeneous directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by