BUILDING YOUR VERY OWN BLOCKCHAIN SERVICE USING CRYPTO.js
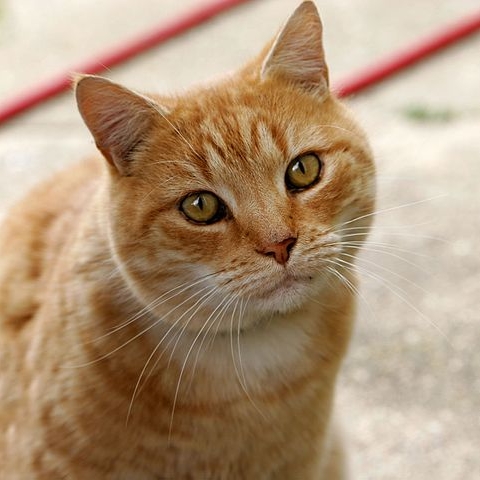
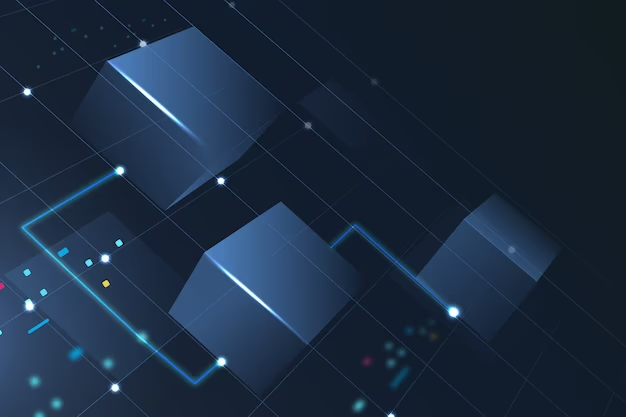
That's why they say not to believe everything you see on internet 🤭
here i can provide a beginner's guide on building your very own blockchain program AND I LL SHOW YOU HOW TO DEPLOY THIS ON API USING EXPRESS.JS (See i don't lie 😓)
Before we start, let's have a quick overview what we r gonna confront with
Introduction to Blockchain and Crypto.js (YOU CAN SKIP)
Setting Up Your Development Environment (YOU CAN SKIP)
Creating the Blockchain Class
Implementing Cryptographic Functions with Crypto.js
Adding and Validating Blocks
Deploying Your Blockchain Service
Conclusion (YOU CAN SKIP)
1. Introduction to Blockchain and Crypto.js
What is a Blockchain?
A blockchain is a decentralized, distributed ledger that stores data in a series of linked blocks. Each block contains a cryptographic hash of the previous block, ensuring the integrity and immutability of the entire chain.
What is Crypto.js?
Crypto.js is a powerful JavaScript library that provides a wide range of cryptographic functions, such as hashing, encryption, and decryption. It's a perfect fit for building blockchain applications where security and cryptography are essential.
2. Setting Up Your Development Environment
To get started, you’ll need Node.js and npm (Node Package Manager) installed on your system.
You know this step already, you will need to create a new project directory first:
mkdir blockchain-service
cd blockchain-service
Initialize a new Node.js project:
npm init -y
Next, install Crypto.js by running:
npm install crypto-js
Now, create a new JavaScript file to start coding your blockchain:
touch blockchain.js
3. Creating the Blockchain Class
In your blockchain.js
file, start by creating a Blockchain
class. This class will manage the blocks and handle the basic functionality of your blockchain service.
const CryptoJS = require('crypto-js');
// creating the blockchain chain class to manage blocks
class Blockchain {
constructor() {
this.chain = [];
this.createGenesisBlock();
}
createGenesisBlock() {
const genesisBlock = this.createBlock("0");
this.chain.push(genesisBlock);
}
createBlock(previousHash) {
const block = {
index: this.chain.length + 1,
timestamp: new Date().toISOString(),
data: "This is a new block",
previousHash: previousHash,
hash: ""
};
block.hash = this.hashBlock(block);
return block;
}
hashBlock(block) {
const blockString = JSON.stringify(block);
return CryptoJS.SHA256(blockString).toString();
}
getLastBlock() {
return this.chain[this.chain.length - 1];
}
addBlock(newBlock) {
this.chain.push(newBlock);
}
}
module.exports = Blockchain;
4. Implementing Cryptographic Functions with Crypto.js
Now that we have a basic structure, let's explore how Crypto.js enhances our blockchain with cryptographic security.
Hashing Blocks
The hashBlock
method in the Blockchain
class uses Crypto.js to create a SHA-256 hash of each block. This ensures that any change to the block’s content will result in a completely different hash, making the blockchain tamper-proof.
hashBlock(block) {
const blockString = JSON.stringify(block);
return CryptoJS.SHA256(blockString).toString();
}
This line uses CryptoJS.SHA256()
to hash the block data, providing a unique hash string for each block.
5. Adding and Validating Blocks
Next, we'll implement functions to add new blocks and validate the blockchain.
Adding New Blocks
To add a block, you first need to get the hash of the last block and then create a new block linked to it:
const Blockchain = require('./blockchain');
const blockchain = new Blockchain();
const lastBlock = blockchain.getLastBlock();
const newBlock = blockchain.createBlock(lastBlock.hash);
blockchain.addBlock(newBlock);
console.log("New block added: ", newBlock);
Validating the Blockchain
To ensure that the blockchain is valid, we need to check two things:
Each block's
previousHash
matches thehash
of the preceding block.The
hash
of each block is still valid and corresponds to the block's data.
Add the following validation method to the Blockchain
class:
isChainValid() {
for (let i = 1; i < this.chain.length; i++) {
const currentBlock = this.chain[i];
const previousBlock = this.chain[i - 1];
if (currentBlock.previousHash !== previousBlock.hash) {
return false;
}
if (currentBlock.hash !== this.hashBlock(currentBlock)) {
return false;
}
}
return true;
}
This method will ensure the integrity of the blockchain, making it resistant to tampering.
6. Deploying Your Blockchain Service
Once your blockchain is functional, the next step is to deploy it as a service. You can use a framework like Express.js to create a simple API for interacting with your blockchain.
Here's a basic example:
const express = require('express');
const Blockchain = require('./blockchain');
const app = express();
const blockchain = new Blockchain();
app.get('/blockchain', (req, res) => {
res.json(blockchain.chain);
});
app.post('/mine', (req, res) => {
const lastBlock = blockchain.getLastBlock();
const newBlock = blockchain.createBlock(lastBlock.hash);
blockchain.addBlock(newBlock);
res.json(newBlock);
});
app.listen(3000, () => {
console.log('Blockchain service running on port 3000');
});
7. Conclusion
Congratulations! You've successfully built and deployed a simple blockchain service using JavaScript and Crypto.js. This project introduced you to the fundamentals of blockchain technology and the power of cryptographic functions provided by Crypto.js.
You can expand this service by adding features such as:
Smart Contracts: Implementing programmable contracts within your blockchain.
Consensus Algorithms: Ensuring decentralized decision-making.
Proof of Work: Adding mining and difficulty adjustments to your blockchain.
If you find it helpful, follow me on Hashnode for more guides on blockchain, JavaScript, and other exciting technologies.
Subscribe to my newsletter
Read articles from Subhradip Sinha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
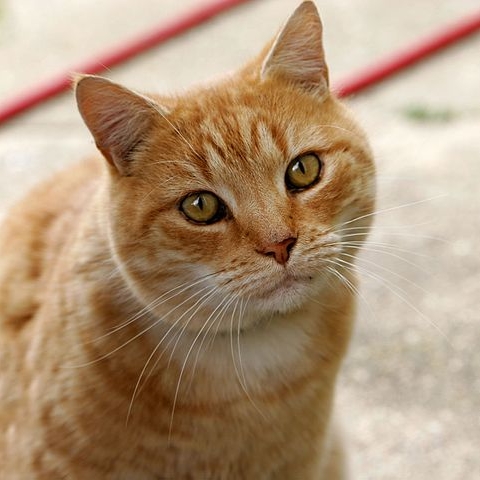