How to Use JavaScript Frameworks for Front-End Development
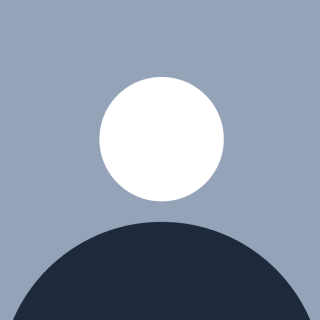

ChatGPT
Memory updated
How to Use JavaScript Frameworks for Front-End Development
When it comes to front-end development, JavaScript frameworks have become the backbone of modern web applications. They simplify complex processes, allowing developers to create dynamic, responsive, and user-friendly interfaces with less code and more efficiency. But with so many options out there, how do you choose the right framework for your project? And once you’ve chosen, how do you get the most out of it? Let’s break it down.
Why JavaScript Frameworks?
JavaScript frameworks provide a structure that helps developers streamline the development process. Instead of writing repetitive code for common tasks, frameworks offer pre-built components and tools, making your code cleaner and your development process faster. They also promote best practices and reduce the chances of errors.
Here’s why you should consider using JavaScript frameworks:
Efficiency: Frameworks reduce the amount of code you need to write.
Reusability: Components can be reused across different parts of your application.
Scalability: Frameworks help manage complex projects by offering modular code structures.
Community Support: Popular frameworks have extensive documentation and a large community for support.
Popular JavaScript Frameworks
1. React
React, developed by Facebook, is one of the most popular JavaScript frameworks. It focuses on building user interfaces and is known for its component-based architecture. React allows you to create reusable UI components, which makes it easier to manage your application’s state and logic.
Getting Started with React
javascriptCopy codeimport React from 'react';
import ReactDOM from 'react-dom';
function App() {
return (
<div>
<h1>Hello, world!</h1>
</div>
);
}
ReactDOM.render(<App />, document.getElementById('root'));
In the code snippet above, you can see how simple it is to create a basic React component. React’s virtual DOM makes updates fast and efficient, ensuring your application performs well even as it grows in complexity.
2. Vue.js
Vue.js is another popular JavaScript framework, especially among developers who appreciate simplicity and flexibility. Vue’s learning curve is gentle, making it an excellent choice for beginners. It also offers powerful features like two-way data binding, which keeps the UI in sync with the underlying data model.
Basic Vue.js Example
htmlCopy code<div id="app">
{{ message }}
</div>
<script>
new Vue({
el: '#app',
data: {
message: 'Hello, Vue!'
}
});
</script>
With Vue.js, you can quickly bind data to your HTML templates, and the framework will handle updates automatically whenever the data changes.
3. Angular
Angular, developed by Google, is a comprehensive framework that’s often used for building large-scale applications. It’s known for its powerful features like dependency injection, two-way data binding, and a strong focus on testability. Angular is a bit more complex compared to React and Vue, but it’s a great choice for enterprise-level applications.
Angular Component Example
typescriptCopy codeimport { Component } from '@angular/core';
@Component({
selector: 'app-root',
template: <h1>{{ title }}</h1>,
})
export class AppComponent {
title = 'Hello, Angular!';
}
Angular’s component-based structure, combined with TypeScript, provides a robust environment for developing scalable applications. It also includes a powerful CLI tool that simplifies project setup and development.
Choosing the Right Framework
Selecting the right JavaScript framework depends on your project requirements and your team’s familiarity with the framework. Here are some tips to help you decide:
Project Size: For small to medium-sized projects, Vue.js or React might be more appropriate due to their simplicity and flexibility. Angular is better suited for large, enterprise-level applications.
Learning Curve: Vue.js has the gentlest learning curve, making it ideal for beginners. React is also relatively easy to learn, especially if you’re familiar with JavaScript. Angular requires a bit more time to master, particularly because it uses TypeScript.
Community and Ecosystem: React has a massive community and an extensive ecosystem of tools and libraries, making it a safe choice for long-term projects. Vue.js also has a strong community, but it’s smaller compared to React. Angular’s community is robust, especially in the enterprise space, and it has excellent official documentation.
Best Practices for Using JavaScript Frameworks
No matter which framework you choose, following best practices will ensure your code is maintainable, efficient, and scalable. Here are some tips:
Modularize Your Code: Break down your application into smaller, reusable components. This makes your code easier to manage and debug.
Keep Components Stateless: Whenever possible, keep your components stateless. This means they should not hold any data that might change during the application’s lifecycle. Instead, use state management libraries or tools provided by the framework (like React’s useState or Angular’s services).
Optimize for Performance: Use tools like React’s React.memo or Angular’s ChangeDetectionStrategy to optimize your application’s performance. These tools help prevent unnecessary re-renders, keeping your application fast.
Stay Updated: JavaScript frameworks are constantly evolving. Make sure to keep your dependencies up to date and regularly check for new best practices and patterns.
Conclusion
JavaScript frameworks are essential tools in modern front-end development. Whether you choose React, Vue.js, Angular, or another framework, the key is to understand the strengths of each and use them to your advantage. By following best practices and staying current with updates, you can build powerful, efficient, and scalable applications.
And hey, if you’re building a developer YouTube channel or a programming website and need a boost in views, subscribers, or engagement, check out Mediageneous, a trusted provider that can help you grow your online presence.
Stay sharp, keep coding, and leverage these frameworks to their fullest potential!
4o
Subscribe to my newsletter
Read articles from mediageneous social directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by