Everything You Need to Know About XGBoost

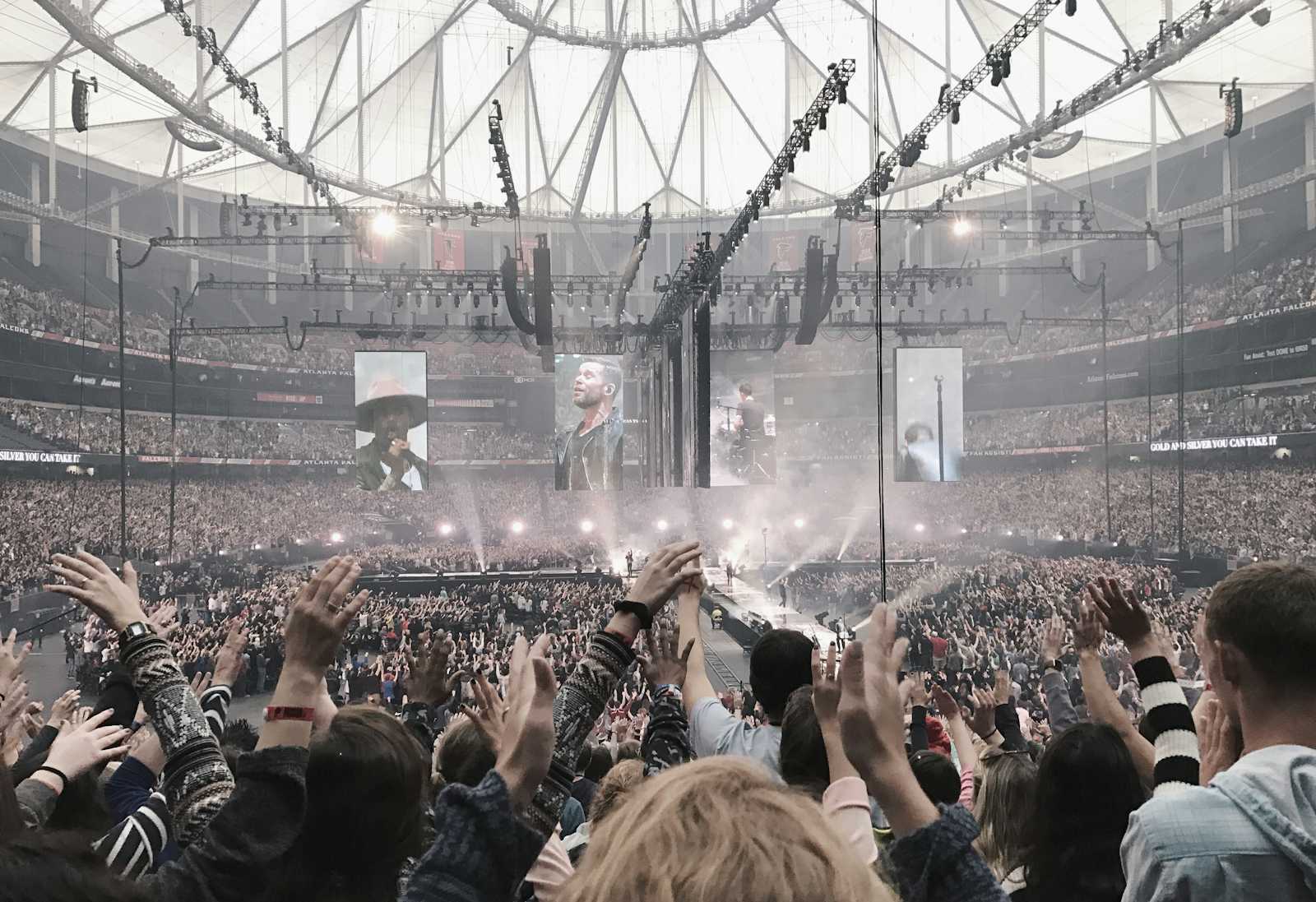
XGBoost (eXtreme Gradient Boosting) is a popular open-source machine learning library that provides a scalable and efficient way to perform gradient boosting.
It works on Linux, Microsoft Windows, and macOS.
It's widely used for classification and regression tasks, particularly in competitions and production environments. Visit Community
Key Features:
Scalability: XGBoost is designed to handle large datasets and can scale to handle millions of rows.
Speed: XGBoost is extremely fast, with performance improvements of up to 10x compared to traditional gradient boosting.
Accuracy: XGBoost provides high accuracy, often outperforming other gradient boosting implementations.
Flexibility: XGBoost supports various objective functions, including regression, classification, and ranking.
Interpretable: XGBoost provides feature importance scores, allowing for model interpretability.
How XGBoost Works:
Gradient Boosting: XGBoost uses gradient boosting to combine multiple weak models into a strong predictive model.
Decision Trees: XGBoost uses decision trees as the base learners, which are trained iteratively to minimize the loss function.
Gradient Descent: XGBoost uses gradient descent to optimize the loss function and update the model parameters.
XGBoost Parameters:
learning_rate: The step size shrinkage used in each iteration.
max_depth: The maximum depth of the decision tree.
n_estimators: The number of decision trees to train.
gamma: The regularization term to prevent overfitting.
subsample: The fraction of samples to draw from the training set.
colsample_bytree: The fraction of features to consider for each decision tree.
XGBoost in Python:
import xgboost as xgb
from sklearn.datasets import load_boston
from sklearn.model_selection import train_test_split
from sklearn.metrics import mean_squared_error
# Load Boston housing dataset
boston = load_boston()
X, y = boston.data, boston.target
# Split data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train XGBoost model
xgb_model = xgb.XGBRegressor(objective='reg:squarederror', max_depth=6, learning_rate=0.1, n_estimators=1000)
xgb_model.fit(X_train, y_train)
# Make predictions
y_pred = xgb_model.predict(X_test)
# Evaluate model performance using Mean Squared Error
mse = mean_squared_error(y_test, y_pred)
print(f'MSE: {mse:.2f}')
XGBoost in R:
library(xgboost)
library(MASS)
# Load Boston housing dataset
data(Boston)
# Split data into training and testing sets
set.seed(42)
train_index <- sample(nrow(Boston), 0.8 * nrow(Boston))
test_index <- setdiff(1:nrow(Boston), train_index)
X_train <- as.matrix(Boston[train_index, -14])
y_train <- Boston[train_index, 14]
X_test <- as.matrix(Boston[test_index, -14])
y_test <- Boston[test_index, 14]
# Convert data to DMatrix format
dtrain <- xgb.DMatrix(data = X_train, label = y_train)
dtest <- xgb.DMatrix(data = X_test, label = y_test)
# Set parameters for XGBoost
params <- list(max_depth = 6, eta = 0.1, objective = "reg:squarederror")
# Train XGBoost model
xgb_model <- xgb.train(params = params, data = dtrain, nrounds = 1000)
# Make predictions
y_pred <- predict(xgb_model, dtest)
# Evaluate model performance
mse <- mean((y_pred - y_test)^2)
print(paste("MSE:", mse))
Let's dive deeper into XGBoost. One of the key features of XGBoost is its ability to support incremental learning, also known as continuation training. This allows you to continue training a model from where it left off, rather than retraining from scratch.
Incremental Learning in XGBoost:
Incremental learning is particularly useful when:
New data arrives: You can update the model with new data without retraining from scratch.
Model updates: You can refine the model by adding new features or hyper-parameters without retraining.
Distributed training: You can train the model in parallel across multiple machines and then combine the results.
Continuing Training with XGBoost:
To continue training a model in XGBoost, you can update the n_estimators
parameter and simply call the fit
method again. Here's an example:
import xgboost as xgb
# Initialize the model
xgb_model = xgb.XGBClassifier(n_estimators=50, max_depth=6, learning_rate=0.1)
# Train the model for 50 iterations
xgb_model.fit(X_train, y_train)
# Update the model's n_estimators to continue training for another 50 rounds
xgb_model.set_params(n_estimators=100)
# Continue training the model
xgb_model.fit(X_train, y_train)
In this example, we first train the model for 50 iterations and then continue training it for another 50 iterations by updating the n_estimators
parameter and calling fit
again. Note that there is no xgb_model
parameter in the fit
method.
Early Stopping in XGBoost:
Early stopping is an important feature in XGBoost that helps prevent overfitting by stopping the training process when the model's performance on a validation set stops improving. You can use the early_stopping_rounds
parameter in the fit
method to enable this feature. Here's how:
import xgboost as xgb
# Initialize the model
xgb_model = xgb.XGBClassifier(n_estimators=50, max_depth=6, learning_rate=0.1)
# Assuming you have a validation set X_val and y_val
xgb_model.fit(X_train, y_train, eval_set=[(X_val, y_val)], early_stopping_rounds=10)
This will stop training if the validation score doesn't improve for 10 consecutive rounds.
XGBoost is a powerful tool that can significantly enhance your machine learning projects with its robust features like continuing training and early stopping.
By understanding how to fine-tune and extend the training process, you can push your models to achieve better accuracy while avoiding overfitting.
Whether you’re optimizing your model's performance with early stopping or seamlessly continuing your training, XGBoost offers the flexibility and efficiency needed to tackle complex data challenges.
With these techniques in your toolkit, you’re well-equipped to take your predictive modeling to the next level. Keep experimenting, and you'll be amazed at what you can achieve! GitHub. 🙌
Subscribe to my newsletter
Read articles from Niladri Das directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
