Exploring the Basics of Python Modules and Packages

Table of contents
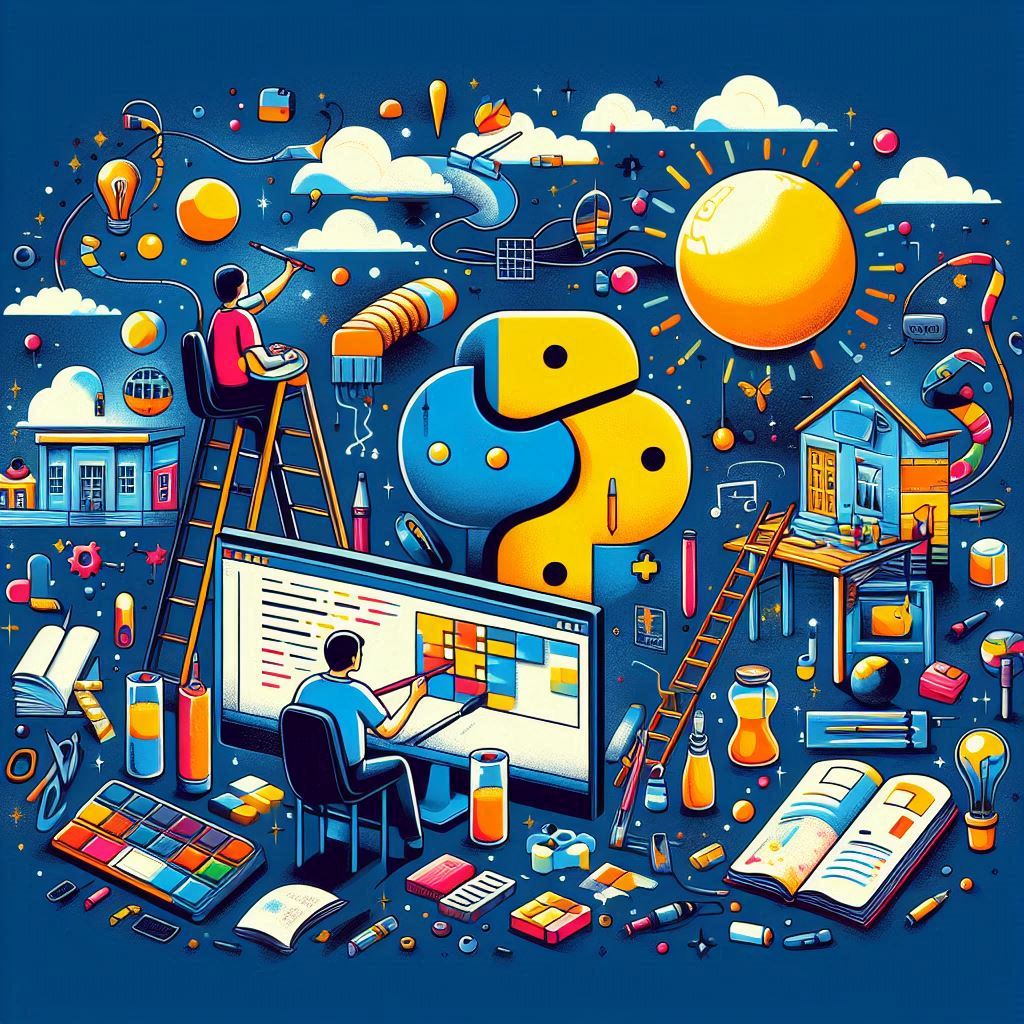
What Are Modules?
A module is simply a file with Python code. It can contain functions, classes, and variables. Modules help organize code into manageable chunks.
Creating a Module
Let’s say you have a file named math_
utils.py
:
# math_utils.py
def add(x, y):
return x + y
def subtract(x, y):
return x - y
PI = 3.14159
In this file, we have two functions (add
and subtract
) and a variable (PI
).
Using a Module
To use the code from math_
utils.py
in another file, you need to import it. For example, in a file named main.py
:
# main.py
import math_utils
result = math_utils.add(5, 3)
print(result) # Output: 8
print(math_utils.PI) # Output: 3.14159
Importing Specific Elements
You can also import only what you need:
from math_utils import add, PI
result = add(5, 3)
print(result) # Output: 8
print(PI) # Output: 3.14159
Aliasing Modules
If the module name is long, you can give it a shorter alias:
import math_utils as mu
result = mu.add(5, 3)
print(result) # Output: 8
What Are Packages?
A package is a collection of modules organized in a directory. Each directory in a package must have an __init__.py
file (which can be empty) to be recognized as a package.
Creating a Package
Let’s say you have a directory structure like this:
mypackage/
__init__.py
math/
__init__.py
operations.py
string/
__init__.py
manipulations.py
In mypackage/math/
operations.py
:
# mypackage/math/operations.py
def add(x, y):
return x + y
In mypackage/string/
manipulations.py
:
# mypackage/string/manipulations.py
def reverse(s):
return s[::-1]
Using a Package
To use the modules in mypackage
, you can import them like this:
from mypackage.math.operations import add
from mypackage.string.manipulations import reverse
print(add(5, 3)) # Output: 8
print(reverse("hello")) # Output: "olleh"
Interview Questions and Answers
What is the difference between a module and a package in Python?
- Answer: A module is a single file with Python code, while a package is a directory containing multiple modules and sub-packages. Packages help organize modules into a hierarchy.
How do you create and use a module in Python?
- Answer: Create a module by saving Python code in a
.py
file. To use the module, import it using theimport
statement. For example,import module_name
orfrom module_name import function_name
.
- Answer: Create a module by saving Python code in a
What is the purpose of the
__init__.py
file in a package?- Answer: The
__init__.py
file tells Python that a directory should be treated as a package. It can be empty or contain initialization code for the package.
- Answer: The
How can you import a specific function from a module?
- Answer: Use the
from module_name import function_name
syntax. For example,from math_utils import add
.
- Answer: Use the
What is module aliasing, and why is it used?
- Answer: Module aliasing means giving a module a different name when importing, which can make the code easier to read and write. For example,
import math_utils as mu
.
- Answer: Module aliasing means giving a module a different name when importing, which can make the code easier to read and write. For example,
Subscribe to my newsletter
Read articles from Tarun Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Tarun Sharma
Tarun Sharma
Hi there! I’m Tarun, a Senior Software Engineer with a passion for technology and coding. With experience in Python, Java, and various backend development practices, I’ve spent years honing my skills and working on exciting projects. On this blog, you’ll find insights, tips, and tutorials on topics ranging from object-oriented programming to tech trends and interview prep. My goal is to share valuable knowledge and practical advice to help fellow developers grow and succeed. When I’m not coding, you can find me exploring new tech trends, working on personal projects, or enjoying a good cup of coffee. Thanks for stopping by, and I hope you find my content helpful!