How HikariCP Connection Pools Improve Spring Boot Microservices
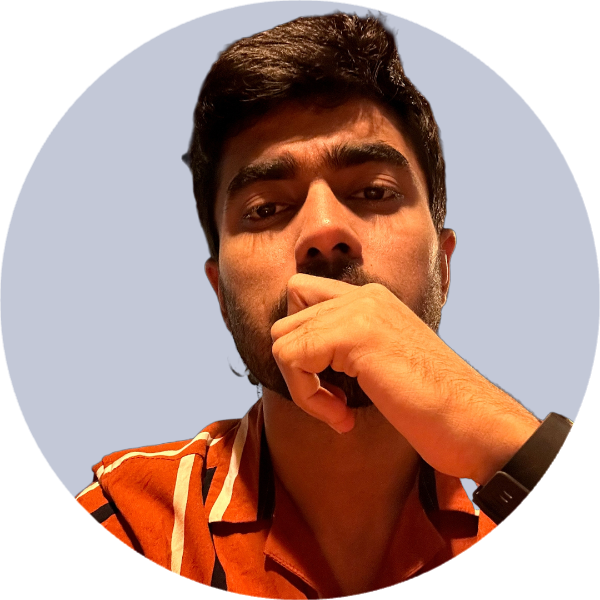
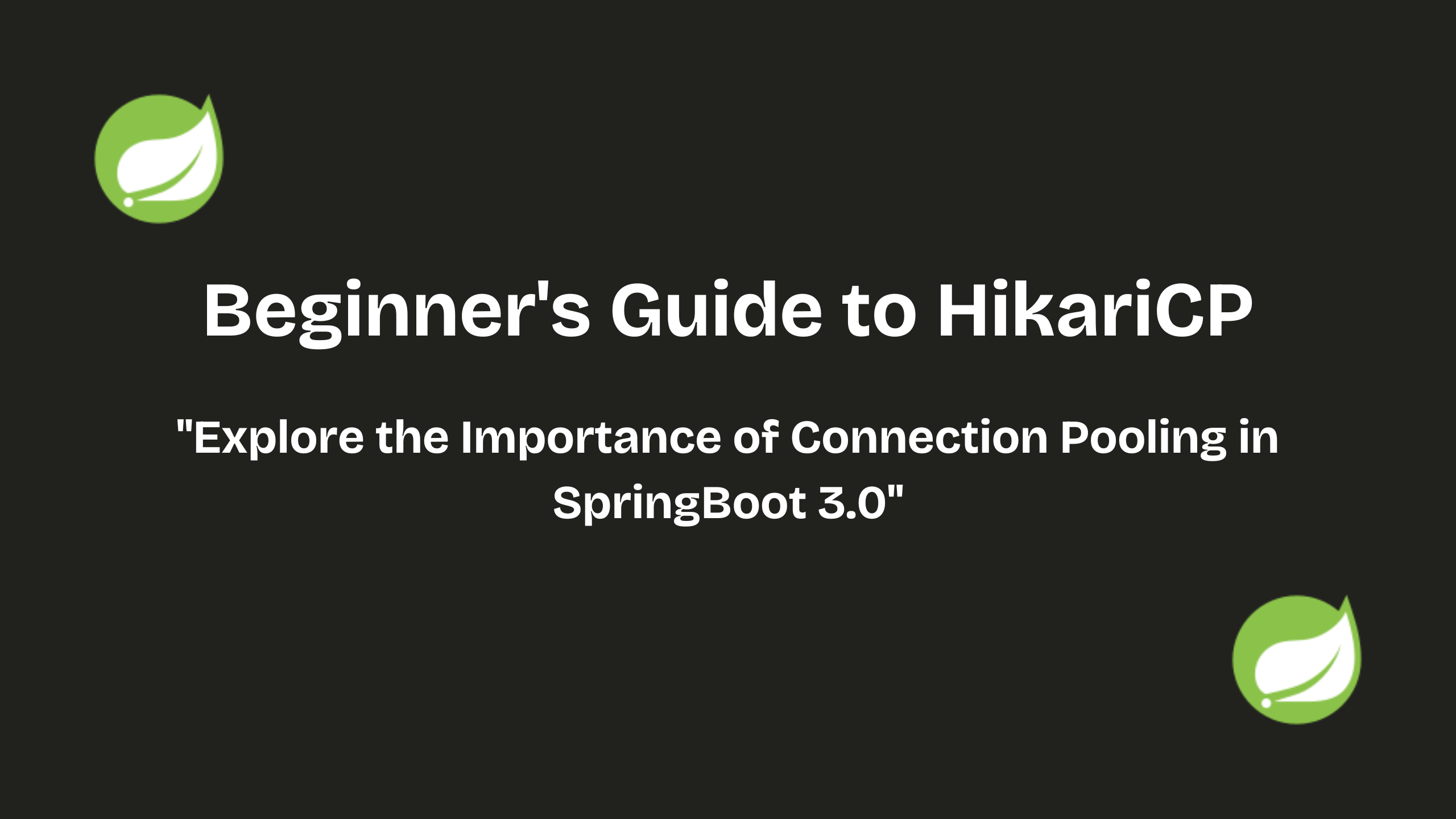
Introduction
In the world of microservices, where efficiency, scalability, and performance are paramount, managing database connections effectively is crucial. HikariCP, a robust connection pool, plays a vital role in achieving optimal connection pooling. But why is a connection pool so important? Let’s explore this by diving into a real-world scenario.
The Problem: Handling Multiple Users Without a Connection Pool
Real-World Example: A Basic E-Commerce Application
Consider an e-commerce application where users can browse products, add items to their cart, and complete purchases. Each user action triggers a database query. Without a connection pool, every request would establish a new connection to the database. Let’s break down what happens in such a scenario:
High Overhead: Each time a user interacts with the application, a new database connection is created and later closed. This process is resource-intensive and time-consuming.
Limited Connections: Databases have a limited number of concurrent connections they can handle. As more users access the application, the database may quickly reach its connection limit and you can might see this kind of errors:
Connection Storms: During peak traffic, the application might overload the database with connection requests, leading to delays, timeouts, and potential failures.
In this scenario, users might experience slow response times or even receive errors when trying to perform actions like checking out their cart. This could result in lost sales and a poor user experience.
The Solution: Implementing HikariCP Connection Pool
To address these issues, we can implement a connection pool, and HikariCP is one of the most popular choices in the Java ecosystem. HikariCP is known for its performance, reliability, and ease of integration with Spring Boot. HikariCP is the default DataSource implementation in Spring Boot 3, as stated in the reference manual.
How HikariCP Works :
HikariCP manages a pool of database connections that can be reused across multiple requests. Instead of creating a new connection for each user request, the application borrows a connection from the pool, executes the query, and returns the connection to the pool. This approach offers several key benefits:
Reduced Overhead: Connections are reused, reducing the time and resources needed to establish new connections.
Efficient Resource Utilization: By limiting the number of connections in the pool, the application ensures that the database is not overloaded by excessive connection requests.
Improved Performance: With connections readily available in the pool, user requests can be processed faster, leading to a smoother and more responsive application.
Example: Configuring HikariCP in Spring Boot
Let's walk through the steps to configure HikariCP in a Spring Boot application.
Add HikariCP Dependency
If you’re using Spring Boot 3, HikariCP is included by default. However, if you need to add it manually, include the following dependency in your
pom.xml
:Configure HikariCP in
application.properties
Here's a basic configuration:
Explanation of Key Properties:
maximum-pool-size
What it does: This sets the maximum number of database connections that can be open at the same time.
Why it matters: If too many connections are allowed, the database can become overloaded. If too few are allowed, the application might slow down when lots of users are active. It’s about finding the right balance.
minimum-idle
What it does: This specifies the minimum number of connections that should always be ready and waiting in the pool.
Why it matters: It ensures that when a new request comes in, there’s likely to be a connection ready to go, making the app faster.
idle-timeout
What it does: This is the maximum time a connection can sit in the pool without being used before it gets removed.
Why it matters: This helps free up unused connections, ensuring that the pool isn’t holding onto connections that aren’t needed.
connection-timeout
What it does: This sets how long the application will wait to get a connection from the pool before giving up.
Why it matters: If the application has to wait too long for a connection, it could slow down or even fail. Setting a reasonable timeout helps avoid long waits.
max-lifetime
What it does: This is the maximum time a connection can live before it’s replaced with a new one.
Why it matters: Over time, connections can become less efficient or even cause problems. Replacing them regularly keeps things running smoothly.
Fine-Tuning and Monitoring HikariCP
Connection Leak Detection: Enable leak detection by setting
spring.datasource.hikari.leak-detection-threshold=2000
. This helps in identifying connections that aren't closed properly within the specified time (in milliseconds).Metrics and Monitoring: HikariCP integrates well with monitoring tools like Prometheus and Micrometer. By configuring metrics, you can keep an eye on the pool's performance and make adjustments as needed.
Conclusion
By implementing HikariCP in your Spring Boot application, you can significantly enhance performance and ensure efficient database connection management. The properties we've discussed allow you to fine-tune the connection pool to suit your application's needs, leading to a more reliable and scalable system. Properly configured, HikariCP can be the key to handling large volumes of database requests without compromising on speed or user experience.
Subscribe to my newsletter
Read articles from Sudarshan Doiphode directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
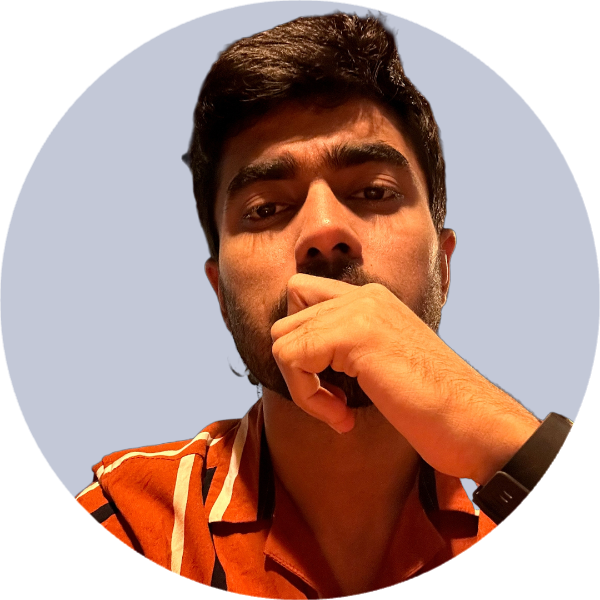
Sudarshan Doiphode
Sudarshan Doiphode
👋 Hey there! I'm Sudarshan, a tech enthusiast with a focus on: • 🚀 Spring Boot • 🌼 Spring • 🔒 Spring Security • 🌱 Hibernate • ☕ Java 8 & Java • 🚀 Apache Kafka • 🛢️ Apache Maven • 🐳 Docker • 🔗 Git • 🏗️ Design Patterns • And more tech adventures! Follow for insights and join the journey. 🌟👨💻 #TechEnthusiast