Understanding Key Features of DOM, Shadow DOM, Virtual DOM
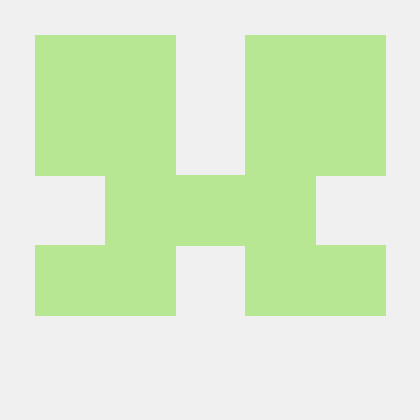
In the ever-evolving world of web development, the Document Object Model (DOM), Shadow DOM, and Virtual DOM are important concepts that shape how we interact with web pages and build dynamic applications. These terms are frequently used in front-end development.
This article breaks down these concepts, exploring their details, use cases, and practical applications. Whether you are a beginner or an experienced developer seeking deeper insights, this article will give you the knowledge you need to use the DOM, Shadow DOM, and Virtual DOM effectively.
WHAT IS DOM?
You will agree it is important to understand the meaning of the DOM and how it works in order to grasp the meaning of its other forms. Document Object Model is the hierarchy of elements in the browser. DOM is the foundation of web development. It represents the structure of an HTML document and allows developers to interact with its elements.
<!DOCTYPE html>
<html>
<head>
<title>DOM example</title>
</head>
<body>
<h1>Hello, DOM!</h1>
<p>This is a paragraph.</p>
</body>
</html>
In this example, the DOM tree has the HTML elements as its root node, with child nodes for the head and body and further child nodes for the title,h1, and p elements. Developers can access and manipulate these elements using JavaScript based on this hierarchical structure in DOM.
Structure and Hierarchy of DOM:
HTML document is the root node in the organized tree structure in DOM. Elements, attributes, and text content are represented as nodes within this tree, and their relationships are defined by their parent-child associations.
Manipulating DOM with Javascript:
One interesting aspect of DOM is the ability to manipulate it dynamically using JavaScript. Developers can access elements, modify their attributes, change their content, and respond to user interactions.
<button id="clickButton">Click me!</button>
<p id="message">Hello, DOM!</p>
Javascript can be used here to change the text of the paragraph when the button is clicked:
const button = document.getElementById("clickButton");
const message =document.getElementById("message");
button.addEventListener("click", () => {
message.textContent = "Button Clicked!";
});
In the code above, we retrieve references to the button and paragraph using "getElementById" and then add an event listener to the button. Clicking the button will update the text content in the paragraph, reflecting dynamic changes to the DOM.
Shadow DOM:
Shadow DOM is a web standard that brings encapsulation and isolation to web components. It allows developers to create self-contained, reusable components with their own scoped DOM tree and styles. This encapsulation prevents conflicts between different parts of a web page, ensuring that styles and functionality within a component do not inadvertently affect other parts of the page.
This is a custom video player component created using Shadow DOM:
<!DOCTYPE html>
<html>
<head>
<title>This is a Shadow DOM</title>
</head>
<body>
<video control width="600">
<source src="https://www.freepik.com/free-video/misty-forest-mountain_29617#fromView=search&page=1&position=0&uuid=f78a023e-fe02-4bae-9359-b3a54d208365">
</video>
</body>
</html>
In your browser , the button components on the video player will be encapsulated, such that changes made on the page won't affect the button component.
Creating and Using Shadow DOM: The `attachShadow` method attached to an element is a typical way to create a shadow DOM. You can manipulate the Shadow DOM just like you would with regular DOM. Shadow DOM can contain its elements, styles, and event listeners, making it a powerful tool for building web components. As a continuation of the previous example, we can add event listeners to the custom video player within Shadow DOM:
const video = shadowRoot.querySelector("video");
video.addEventListener("play", () => {
console.log("video is playing!");
});
video.addEventListener("pause", () => {
console.log("video is paused.");
});
Here, we add event listeners directly to the video
element within the Shadow DOM, encapsulating the behavior of the custom video player.
Shadow DOM vs. Regular DOM:
One key difference between Shadow DOM and regular DOM is encapsulation. The regular DOM allows styles and functionality to affect the entire page. Still, Shadow DOM provides a sandboxed environment where the styles and functionality are limited to the component, ensuring no unintended side effects on other page elements.
Benefits and Use Cases of Shadow DOM:
For a developer, Shadow DOM is a very useful concept when building web components and widgets. It allows programmers to create components with encapsulated styles and behavior , which are reusable and less prone to conflicts with other parts of a web page. Common use cases include custom form controls, media players, and UI widgets.
What is Virtual DOM?
If you're familiar with React, you've likely encountered the term "Virtual DOM" frequently. It is not limited to React, frameworks like Vue.js, and Svelte also use virtual DOM but what does it actually mean? The browser maintains a memory structure that represents the actual DOM, and this virtual representation is known as the Virtual DOM.
When changes are made to a web application's state, they are first reflected in the Virtual DOM before being applied to the real DOM. This process is similar to receiving a notification that a bank transaction was successful, even though your account hasn't been debited yet. The immediate alert you receive in your banking app is like the Virtual DOM—it shows a change that hasn't yet been finalized in the real system.
This approach is designed to optimize rendering performance and reduce unnecessary DOM manipulations.
To understand the concept of virtual DOM, consider a simple React component:
class Counter extends React.Component {
constructor(props) {
super(props);
this.state = {count: 0 };
}
incrementCount() {
this.setState({ count: this.state.count + 1});
}
render(){
return(
<div>
<p> Count : {this.state.count}</p>
<button onClick={() => this.incrementCount()}>Increment</button>
</div>
);
}
}
When you click the "increment" button in the example above, React updates the virtual DOM with the new count value, compares it with the previous Virtual DOM state, and efficiently updates the actual DOM with only the necessary changes. This reduces the number of direct DOM manipulations and enhances performance.
Overall, imagine in a shopping app that you have to add and remove items. Without Virtual DOM, each addition or removal would directly manipulate the DOM, potentially causing layout thrashing and poor performance. With Virtual DOM, these changes are batched and optimized, leading to a smoother user experience.
Comparing DOM, Shadow DOM, and Virtual DOM
Let us explore when and how to choose what to use between actual DOM, Shadow DOM, and Virtual DOM.
• DOM: Use the real DOM when you need to manipulate individual elements on a webpage or create dynamic interactions. It is essential for basic web development
• Shadow DOM: You can use this when building custom web components or widgets that should be encapsulated and reusable without affecting other parts of the page.
• Virtual DOM: Javascript frameworks like React, Vue.js, or Svelte uses Virtual DOM to optimize rendering performance in complex applications with frequent state update. To understand this better, let's say you are building a complex web application with multiple interactive components. You could use the regular DOM for simple interactions like showing/hiding elements or handling form submissions.
However, for more intricate user interface components, such as a custom date picker or an image carousel, Shadow DOM is employed to encapsulate their styles and behaviors. If your application involves frequent state changes and updates, a library like React with virtual DOM can efficiently manage the rendering and improve performance.
Performance Considerations:
• DOM: Direct DOM manipulation when making many changes can be less efficient. It may lead to layout thrashing and performance bottlenecks.
• Shadow DOM: The encapsulation employed in shadow DOM can improve performance by preventing unintended style conflicts, but it doesn't directly address rendering optimization.
• Virtual DOM: Virtual DOM excels in rendering optimization, significantly reducing the number of updates to the actual DOM, which can lead to substantial performance improvements in complex applications.
Combining them?
It is possible to combine this variant of DOM within a single web application. For example, you could use Shadow DOM for encapsulated components within a React-based application that employs Virtual DOM for overall rendering optimization. These approaches can complement each other, offering the benefits of both encapsulation and performance.
Common Mistakes when working with DOM:
• Excessive DOM Manipulation: Excessive DOM manipulation is one of the most common mistakes in actual DOM, especially within loops or animation. Each change to the DOM can trigger a reflow, impacting performance. Instead, it is best to batch DOM changes when possible.
• Not Using Event Delegation: Attaching event listeners to multiple individual elements can lead to inefficient code. Event delegation, where you attach an event listener to a common ancestor and check the target of the event, is often a better approach for handling multiple similar elements.
Challenges in Using Shadow DOM Effectively?
• Styling Isolation: Even though Shadow DOM provides style encapsulation, challenges can occur when you want to apply global styles to certain elements. You might need to use CSS variables or CSS custom properties to bridge this gap.
• Accessibility: Making Shadow DOM components accessible to screen readers and assistive technologies can be complex. It requires proper use of ARIA {what is ARIA?} attributes and careful consideration of keyboard navigation.
Potential Issues and Optimization with Virtual DOM:
• Over-Rendering: Virtual DOM can sometimes result in over-rendering, where components re-render unnecessarily. Implementing shouldComponenUpdate or using React's PureComponent can help resolve this issue.
• Memory Consumption: Maintaining a virtual representation of the DOM can consume memory, especially in applications with large component trees. Developers should be aware of memory optimization strategies, such as component unmounting and data cleanup.
• Complex Component Hierarchies: Performance bottlenecks may happen if updates propagate through many layers in deeply nested components. Developers should implement state management solutions like Redux to manage state efficiently.
• Key Prop Optimization: For a javascript library like React that implements virtual DOM, it's crucial to provide unique keys to each element. Without keys, React may re-render the entire list instead of just changed elements.
{todos.map((todo) => (
<TodoItem key={todo.Id} todo={todo} />
))}
Best Practices to Address Challenges:
• Performance Profiling: Use browser developer tools to profile your application's performance and identify bottlenecks. Tools like the Chrome DevTools performance tab can help you pinpoint performance issues related to DOM manipulation.
• Testing: Comprehensive testing, including unit testing and accessibility testing, is essential to catch issues early in development.
• Code Reviews: It is advisable to collaborate with peers or engage a mentor to conduct code reviews for feedback and ensure best practices are followed. In conclusion, our exploration of DOM, Shadow DOM, and Virtual DOM has underscored their pivotal roles in modern web development.
Conclusion
The Document Object Model (DOM) enables dynamic web experiences through JavaScript. Shadow DOM offers encapsulation for self-contained components, preventing styling conflicts. Virtual DOM, used in frameworks like React, optimizes performance by reducing direct DOM manipulations. Mastering these concepts boosts productivity and enhances user experiences. Embrace these technologies, engage with the developer community, and commit to lifelong learning to excel in web development.
Subscribe to my newsletter
Read articles from Lukman Badru directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
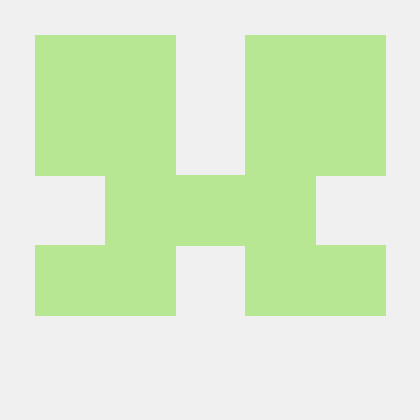