Part 1 - Understanding Inheritance in Python: A Comprehensive Guide

Table of contents
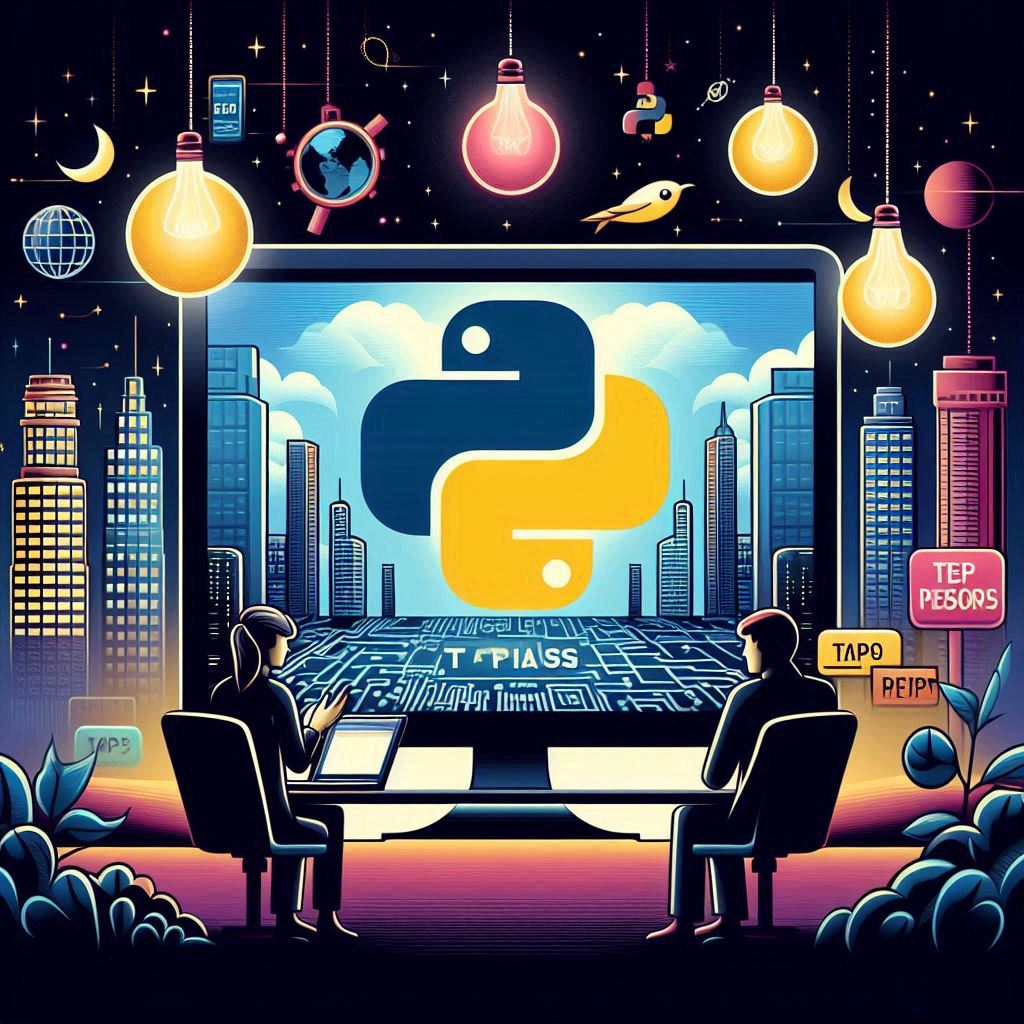
Inheritance is a fundamental concept in object-oriented programming that allows a class to inherit attributes and methods from another class. This promotes code reuse and establishes a natural hierarchy between classes. In this guide, we will explore inheritance in Python, provide detailed explanations and examples, and present coding interview questions to test your understanding.
What is Inheritance?
Inheritance enables a new class (known as a subclass or derived class) to inherit attributes and methods from an existing class (known as a superclass or base class). The primary purpose of inheritance is to promote code reuse and establish a natural hierarchy between classes.
Basic Example of Inheritance
Let's start with a simple example of inheritance:
class Animal:
def speak(self):
return "Some generic sound"
class Dog(Animal):
def speak(self):
return "Bark"
class Cat(Animal):
def speak(self):
return "Meow"
# Test
dog = Dog()
cat = Cat()
print(dog.speak()) # Output: Bark
print(cat.speak()) # Output: Meow
In this example, Dog
and Cat
classes inherit from the Animal
class. They override the speak
method to provide specific sounds.
Detailed Explanation of Inheritance
How Inheritance Works
When you create a subclass, it inherits all methods and attributes from the parent class. This allows you to use and extend the functionalities of the parent class without rewriting code.
Method Resolution Order (MRO)
Python uses the Method Resolution Order (MRO) to determine the sequence in which classes are searched when calling a method. The MRO ensures a consistent order for method resolution, especially in multiple inheritance scenarios. Python uses the C3 linearization algorithm for this purpose.
To view the MRO of a class, you can use the __mro__
attribute or the mro()
method:
class A:
pass
class B(A):
pass
class C(A):
pass
class D(B, C):
pass
print(D.__mro__)
# Output: (<class '__main__.D'>, <class '__main__.B'>, <class '__main__.C'>, <class '__main__.A'>, <class 'object'>)
The super()
Function
The super()
function is used to call a method from the parent class. This is useful when extending or modifying the behavior of inherited methods:
class A:
def __init__(self):
print("A's __init__")
class B(A):
def __init__(self):
super().__init__()
print("B's __init__")
b = B()
# Output:
# A's __init__
# B's __init__
Overriding Methods
In a subclass, you can override methods of the parent class to provide a specific implementation:
class Animal:
def speak(self):
return "Generic sound"
class Dog(Animal):
def speak(self):
return "Bark"
dog = Dog()
print(dog.speak()) # Output: Bark
Examples of Inheritance
Single Inheritance
When a subclass inherits from a single parent class:
class A:
pass
class B(A):
pass
Multiple Inheritance
When a subclass inherits from multiple parent classes:
class A:
pass
class B:
pass
class C(A, B):
pass
Multilevel Inheritance
When a subclass inherits from a class that is itself a subclass of another class:
class A:
pass
class B(A):
pass
class C(B):
pass
Hierarchical Inheritance
When multiple subclasses inherit from a single parent class:
class A:
pass
class B(A):
pass
class C(A):
pass
Conclusion
Inheritance in Python provides a powerful way to create a new class that builds upon an existing class. It supports code reuse and helps in organizing code hierarchically. Understanding how to use inheritance effectively is crucial for designing scalable and maintainable object-oriented systems.
In the second part of this article, we'll explore common interview questions related to inheritance. These questions will test your understanding of how inheritance works in Python, including practical coding problems. We’ll provide sample solutions and explanations to help you master this crucial aspect of object-oriented programming.
Subscribe to my newsletter
Read articles from Tarun Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Tarun Sharma
Tarun Sharma
Hi there! I’m Tarun, a Senior Software Engineer with a passion for technology and coding. With experience in Python, Java, and various backend development practices, I’ve spent years honing my skills and working on exciting projects. On this blog, you’ll find insights, tips, and tutorials on topics ranging from object-oriented programming to tech trends and interview prep. My goal is to share valuable knowledge and practical advice to help fellow developers grow and succeed. When I’m not coding, you can find me exploring new tech trends, working on personal projects, or enjoying a good cup of coffee. Thanks for stopping by, and I hope you find my content helpful!