🌟 Understanding POJO in Java: The Backbone of Simplicity

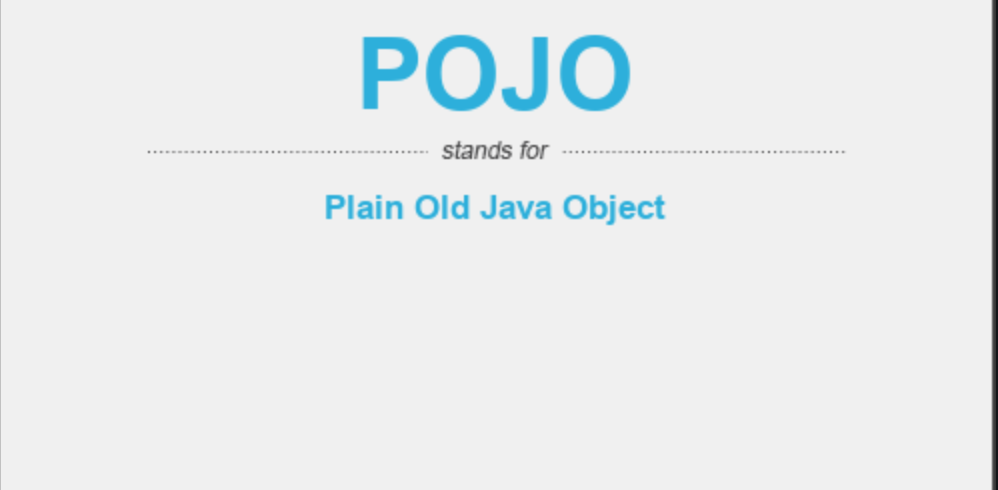
🚀 What is a POJO?
A POJO is a basic Java object that doesn't follow any special conventions or frameworks. It’s free from the clutches of EJBs, and it doesn’t implement any interfaces or extend any classes outside of the basic Java library. Think of it as the purest form of an object in Java, doing exactly what it’s supposed to—holding data.
✨ Key Characteristics of a POJO
No Dependencies: A POJO doesn’t need to implement any specific interface or extend any particular class.
Simple and Plain: It’s straightforward, with no special restrictions or rules imposed by Java EE or any other framework.
Private Fields: Fields are typically private, ensuring data encapsulation.
Public Getters and Setters: Access to these fields is provided through public getter and setter methods.
No Business Logic: A POJO is designed to be a container for data and should not contain any business logic.
🛠️ The POJO Contract
While the term POJO emphasizes simplicity, there are still a few best practices—often referred to as the POJO Contract—that you should follow:
🛑 No-Argument Constructor: Always provide a public no-argument constructor to allow easy instantiation.
🔄 Getters and Setters: Make sure all fields are private, with public getters and setters to control access.
💼 Serializable (Optional): If your POJO needs to be serialized (e.g., when sending it over a network or saving it to a file), implement the
Serializable
interface.🧩 Override
equals()
,hashCode()
, andtoString()
: These methods should be overridden to ensure correct behavior when comparing objects, storing them in collections, or printing them.
🧑💻 Example of a POJO in Action
javaCopy codepublic class Employee {
private String name;
private int id;
private String department;
// Public no-arg constructor
public Employee() {
}
// Getters and Setters
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getDepartment() {
return department;
}
public void setDepartment(String department) {
this.department = department;
}
// Override equals(), hashCode(), and toString()
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Employee employee = (Employee) o;
if (id != employee.id) return false;
if (!name.equals(employee.name)) return false;
return department.equals(employee.department);
}
@Override
public int hashCode() {
int result = name.hashCode();
result = 31 * result + id;
result = 31 * result + department.hashCode();
return result;
}
@Override
public String toString() {
return "Employee{" +
"name='" + name + '\'' +
", id=" + id +
", department='" + department + '\'' +
'}';
}
}
🎯 Why Use POJOs?
Flexibility: POJOs are not tied to any framework, so they can be easily reused across different layers of an application.
Testability: Their simplicity makes POJOs easy to test.
Maintainability: With no complex dependencies, POJOs are easy to maintain and extend.
Ease of Use: POJOs are straightforward, reducing the learning curve for new developers.
Subscribe to my newsletter
Read articles from Pathan Naheda Mohammed Khan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Pathan Naheda Mohammed Khan
Pathan Naheda Mohammed Khan
👋 Hey there! I'm Pathan Naheda Mohammed Khan, a tech enthusiast with a passion for Full Stack Java Development and a keen interest in mastering DevOps practices. 💻 As a Full Stack Java Developer, I immerse myself in the intricacies of software development, leveraging Java's versatility to build powerful and innovative solutions. 🚀 In my journey towards excellence, I'm actively exploring the realm of DevOps, fascinated by its ability to streamline the software development lifecycle and drive continuous improvement. 🔍 On my blog, you'll find a blend of Java coding insights, Full Stack development strategies, and DevOps best practices aimed at empowering fellow tech enthusiasts to unlock their full potential. 📝 Join me as we embark on this exciting adventure together, diving deep into the ever-evolving world of technology. Got a question or topic suggestion? Feel free to reach out—I'm always eager to connect and share knowledge!