Understanding Memory Management in Python

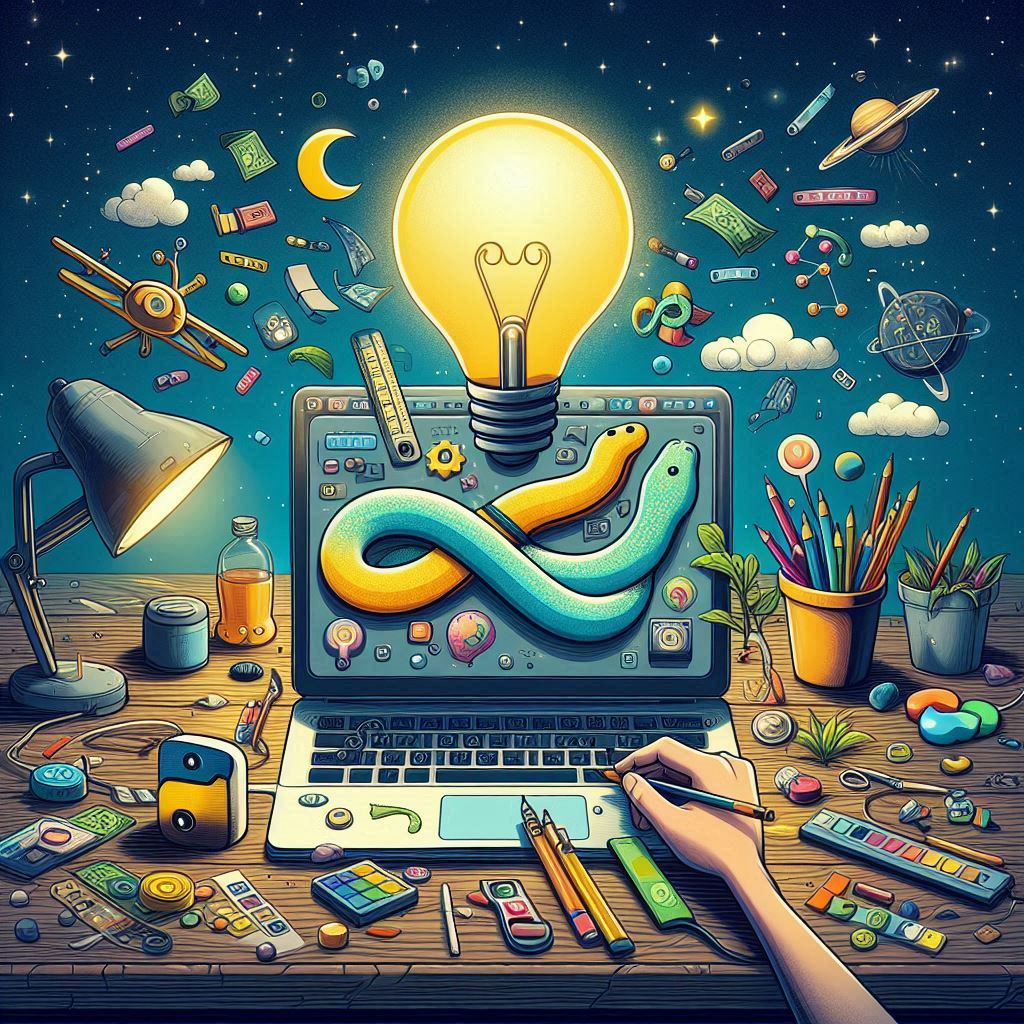
Memory Management in Python
Memory management is a crucial aspect of any programming language, and Python is no exception. Understanding how Python handles memory allocation and deallocation will help you write efficient and optimized code.
1. Detailed Explanation
Python’s Memory Management Model
Python uses a private heap space to manage memory. All Python objects and data structures are stored in this heap, which is maintained by the Python memory manager. The memory manager is responsible for allocating memory for objects and deallocating it when they are no longer needed.
Python abstracts memory management, meaning you don’t need to manually allocate or free memory. However, understanding how it works under the hood can help you optimize performance.
Garbage Collection
Python uses garbage collection (GC) to automatically reclaim memory. The garbage collector identifies objects that are no longer in use and deletes them to free up memory. Python's garbage collector is implemented using reference counting and a cyclic garbage collector.
Reference Counting: Each object in Python has a reference count, which tracks how many references point to the object. When an object’s reference count drops to zero, the memory occupied by the object is deallocated.
Cyclic Garbage Collection: Python's cyclic garbage collector detects and cleans up reference cycles—objects that reference each other, preventing their reference counts from dropping to zero.
Memory Leaks
A memory leak occurs when memory is allocated but not released after it's no longer needed. In Python, memory leaks are less common due to its automatic garbage collection, but they can still happen, particularly with reference cycles that the garbage collector cannot clean up.
2. Internal Details
The Python Memory Manager
Python's memory manager is responsible for allocating and deallocating heap space. It has several components:
Object-specific Allocators: Python has specialized allocators for different types of objects like integers, floats, and strings. These allocators manage memory more efficiently by reusing and pooling memory.
The Global Interpreter Lock (GIL): Python’s memory manager is thread-safe due to the GIL, which ensures that only one thread executes Python bytecode at a time. This prevents memory corruption in multithreaded programs.
How Python Handles Large Objects
Python has a memory allocation mechanism for large objects (objects larger than 512 bytes) called "arenas". Arenas are large chunks of memory allocated from the operating system, and they are subdivided into smaller blocks for storing large objects.
Memory Fragmentation
Memory fragmentation occurs when memory is allocated and deallocated in such a way that there are many small gaps (fragments) of unused memory. Python's memory manager works to minimize fragmentation by reusing memory blocks.
Using sys.getsizeof()
The sys.getsizeof()
function in Python can be used to get the size of an object in bytes. This is useful for understanding the memory footprint of your objects.
import sys
x = [1, 2, 3, 4, 5]
print(sys.getsizeof(x)) # Output: size of the list object in bytes
3. Common Interview Questions
What is memory management in Python, and how does it differ from languages like C++ or Java?
- Memory management in Python is automatic, handled by the Python memory manager, unlike C++ where the programmer manually allocates and deallocates memory.
Explain the concept of garbage collection in Python.
- Garbage collection in Python is the process of automatically freeing up memory by deleting objects that are no longer in use, using reference counting and cyclic garbage collection.
What is the Global Interpreter Lock (GIL), and how does it affect memory management?
- The GIL ensures that only one thread executes Python bytecode at a time, which makes memory management thread-safe but can limit concurrency in CPU-bound programs.
How does Python handle memory leaks?
- Python's garbage collector handles most memory leaks by reclaiming unused memory. However, reference cycles can cause memory leaks if not properly managed.
What is
sys.getsizeof()
and how is it used?sys.getsizeof()
is a function that returns the size of an object in bytes. It’s used to understand the memory footprint of objects in Python.
4. Coding Questions and Solutions
Write a Python program to demonstrate how reference counting works.
import sys a = [] b = a c = a print(sys.getrefcount(a)) # Output: 4 (the function itself adds 1 reference) del b print(sys.getrefcount(a)) # Output: 3 del c print(sys.getrefcount(a)) # Output: 2
Demonstrate how cyclic references can lead to memory leaks in Python.
class Node: def __init__(self, value): self.value = value self.ref = None n1 = Node(1) n2 = Node(2) n1.ref = n2 n2.ref = n1 # Even though we delete the references, the objects are not freed immediately due to the cycle del n1 del n2
Use
gc
module to force garbage collection and show how it handles cyclic references.import gc gc.collect() # Forces garbage collection print(gc.garbage) # Lists objects that could not be freed
Write a function to calculate the memory usage of a Python program.
import sys def memory_usage(): import os, psutil process = psutil.Process(os.getpid()) return process.memory_info().rss print(f"Memory usage: {memory_usage()} bytes")
How would you minimize memory usage in a large Python application?
- Solution: Use generators instead of lists, avoid unnecessary object creation, release resources as soon as they are no longer needed, and make use of memory profiling tools.
What happens when an object’s reference count drops to zero?
- Solution: The memory occupied by the object is deallocated, and the object is destroyed.
Write a Python program that causes a memory leak and then fix it.
class Leak: def __init__(self): self.ref = self leak = Leak() del leak # Fix by breaking the cycle class NoLeak: def __init__(self): self.ref = None no_leak = NoLeak() del no_leak
Explain how Python's memory manager avoids memory fragmentation.
- Solution: Python’s memory manager pools and reuses memory blocks, and it uses arenas for managing large objects to avoid fragmentation.
How would you detect and debug a memory leak in a Python application?
- Solution: Use memory profiling tools like
objgraph
,memory_profiler
, andgc
module to track objects and detect leaks.
- Solution: Use memory profiling tools like
What is the role of the
gc
module in Python?- Solution: The
gc
module provides an interface to the garbage collector, allowing developers to manually manage and inspect the collection process.
- Solution: The
Can the Global Interpreter Lock (GIL) lead to memory-related issues?
- Solution: The GIL itself doesn't lead to memory issues, but it can cause performance bottlenecks in multi-threaded programs, which may indirectly affect memory usage.
How can you prevent Python's garbage collector from collecting an object?
- Solution: You can increase the reference count of the object by adding more references to it.
Write a Python script to manually trigger garbage collection.
import gc print("Garbage collection enabled:", gc.isenabled()) gc.collect()
What is an arena in Python's memory management?
- Solution: An arena is a large block of memory allocated by the Python memory manager to handle large objects (over 512 bytes). Arenas are subdivided into smaller blocks.
How does Python handle memory allocation for immutable objects like strings and tuples?
- Solution: Python uses internal optimization techniques like interning for small strings and tuples to reduce memory usage by reusing objects.
Conclusion and Further Reading
Understanding Python's memory management is crucial for writing efficient and bug-free code. By knowing how memory is allocated, managed, and deallocated, you can avoid common pitfalls like memory leaks and fragmentation. This knowledge is also essential for optimizing the performance of your Python applications.
For further reading, explore the following topics:
Python's garbage collection (
gc
) module.Memory profiling tools like
memory_profiler
andobjgraph
.Deep dive into the Global Interpreter Lock (GIL) and its impact on performance.
Subscribe to my newsletter
Read articles from Tarun Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Tarun Sharma
Tarun Sharma
Hi there! I’m Tarun, a Senior Software Engineer with a passion for technology and coding. With experience in Python, Java, and various backend development practices, I’ve spent years honing my skills and working on exciting projects. On this blog, you’ll find insights, tips, and tutorials on topics ranging from object-oriented programming to tech trends and interview prep. My goal is to share valuable knowledge and practical advice to help fellow developers grow and succeed. When I’m not coding, you can find me exploring new tech trends, working on personal projects, or enjoying a good cup of coffee. Thanks for stopping by, and I hope you find my content helpful!