3 Effective Ways I Use ChatGPT While Coding (and Maybe You Should Too)
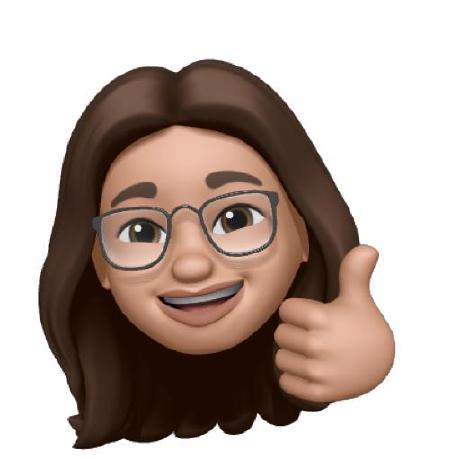
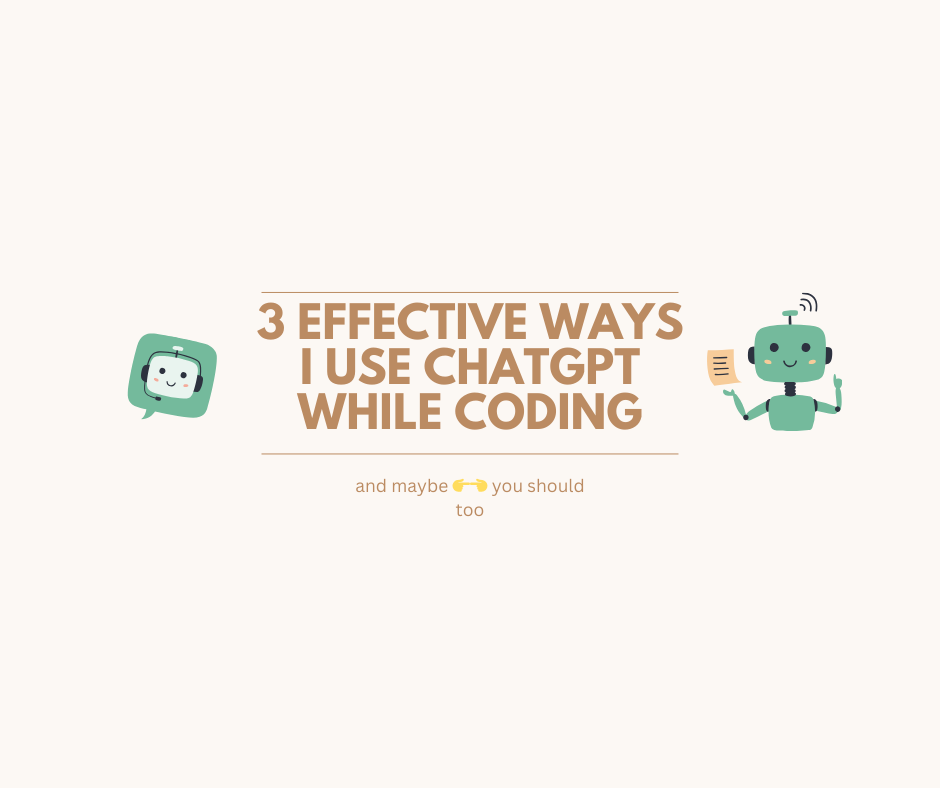
In the age of LLMs being upgraded every day, they're prepared to potentially take over your jobs. However, you can leverage them to become better at software development first.
ChatGPT is one such tool that I and many other developers use daily. It has become an invaluable assistant in my coding journey. Whether you're a seasoned developer or just starting out, here are some of the ways I use ChatGPT when I'm learning something on my own, which might be helpful for you too.
1. Acting as a Senior Developer
When you're learning a language on your own or building a project, you obviously cannot get the kind of feedback and guidance a senior developer might provide. This is where ChatGPT can step in.
How I Use It:
Scenario: You're working on a new project and want to ensure you're using all the right coding practices or learning a language and want to write it efficiently from the get-go.
Ask ChatGPT: "Act like a senior developer and review my code. What would you do differently?"
Example:
Let's say you're writing a function to filter out even numbers from a list. Here's a simple implementation:
function filterEvenNumbers(numbers) {
const evenNumbers = [];
for (const num of numbers) {
if (num % 2 === 0) {
evenNumbers.push(num);
}
}
return evenNumbers;
}
const numbers = [1, 2, 3, 4, 5, 6];
console.log(filterEvenNumbers(numbers)); // Output: [2, 4, 6]
ChatGPT's Suggestion:
function filterEvenNumbers(numbers) {
return numbers.filter(num => num % 2 === 0);
}
const numbers = [1, 2, 3, 4, 5, 6];
console.log(filterEvenNumbers(numbers)); // Output: [2, 4, 6]
As you can see, ChatGPT gave you a more efficient version of your code and introduced you to the filter function in JavaScript. Which, if you're veryyyy new to JavaScript, is very valuable.
2. As a Code Reviewer
Code reviews are an essential part of the development process, but when you're coding on your own and need a second opinion, ChatGPT can help with that too.
How I Use It:
Scenario: You've just gotten done with coding and are looking to optimize your code.
Ask ChatGPT: "How do I optimize this code?"
Example:
Consider this function that calculates the factorial of a number:
function factorial(n) {
if (n === 0) {
return 1;
} else {
return n * factorial(n - 1);
}
}
console.log(factorial(5)); // Output: 120
ChatGPT's Suggestion:
Using an iterative approach can be more efficient for larger numbers:
function factorial(n) {
let result = 1;
for (let i = 1; i <= n; i++) {
result *= i;
}
return result;
}
console.log(factorial(5)); // Output: 120
Always remember to read the suggestions and try them on your own. Never copy the whole thing without understanding it first.
3. Understanding a New Codebase
Contributing to open source is the newest and hottest thing in the tech industry right now. However, many people get overwhelmed when they see enormous codebases and give up on it. You don't have to. Let ChatGPT help you navigate and understand unfamiliar territories in the sea of open-source codebases.
How I Use It:
Scenario: You're exploring a new Laravel project and need to understand how a particular controller works.
Ask ChatGPT: "Explain the purpose and functionality of this code."
Example:
Suppose you're looking at a controller that handles user registration:
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Models\User;
use Illuminate\Support\Facades\Hash;
use Illuminate\Support\Facades\Validator;
class RegisterController extends Controller
{
public function register(Request $request)
{
$validator = Validator::make($request->all(), [
'name' => 'required|string|max:255',
'email' => 'required|string|email|max:255|unique:users',
'password' => 'required|string|min:8|confirmed',
]);
if ($validator->fails()) {
return response()->json(['errors' => $validator->errors()], 422);
}
$user = User::create([
'name' => $request->name,
'email' => $request->email,
'password' => Hash::make($request->password),
]);
return response()->json(['user' => $user], 201);
}
}
ChatGPT's Explanation:
The RegisterController
handles user registration by validating the request data for a new user using the Validator
facade. It checks for a required name, a unique email, and a confirmed password. If validation fails, it returns an error response. Upon successful validation, it creates a new user by hashing the password and returns the created user in a JSON response.
Conclusion
And there you have it: a few simple ways to leverage ChatGPT effectively. But always remember to try things on your own, learn about them, and play around with them. Whenever you get a solution from ChatGPT, take some time to understand why its optimizations were necessary.
Right now, ChatGPT isn't really smart, so it has only a specific type of answer to your questions. While it can't replace the touch of a senior developer or a code reviewer, it can help you get started.
Subscribe to my newsletter
Read articles from Aqsa Aqeel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
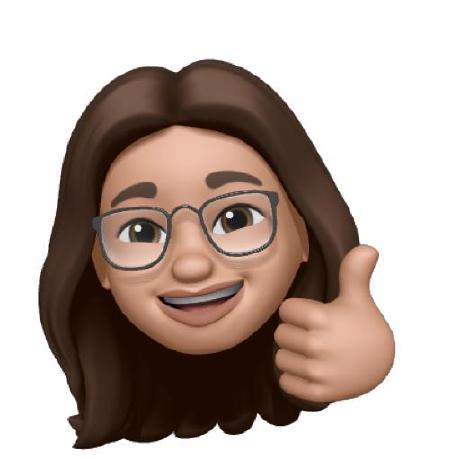
Aqsa Aqeel
Aqsa Aqeel
A front-end developer practicing her super power that is ReactJS.