Send Email with dynamic body using X++

3 min read
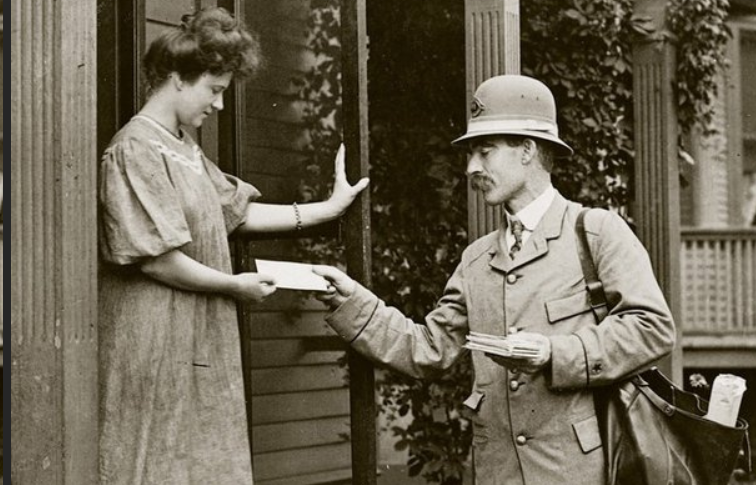
Hello, Coder.
Today, I will show you how to send an email with a dynamic body using an email template in Dynamics 365.
First, we need to set up the Email template in d365.
Path: System administration >> Setup >> Email >> System email templates
In Email message, you can upload your .HTML file for the email body.
HTML code example:
<!DOCTYPE html>
<html lang="en">
<body>
<p>Dear Valued Customer
<br><br>
Please find attached your invoice.
<br><br>
We will appriciate your prompt payment within terms.
<br><br>
Please quote the invoice number for our reference.
<br><br>
Thank you
<br><br>
<b>%1</b>
<br>
Accounts and Finance
<br><br>
ABC Group PVT LTD.
<br>
abc@gmail.com</p>
</body>
</html>
Email Code Example:
/// <summary>
/// This class will use to Send the Email to Customer
/// </summary>
internal final class SendEmail_SalesInvoicePost
{
CustInvoiceJour custInvoiceJour, custInvoiceJourLoc;
SysEmailMessageSystemTable emailMessageSystemTable;
SysEmailSystemTable emailSystemTable;
CustTable custTable;
LogisticsElectronicAddress electronicAddress;
DirPartyTable partyTable;
SysMailerMessageBuilder messageBuilder;
System.IO.MemoryStream fileStream;
str toEmailString,fromEmailString, subjectString, descriptionString,ccString, fileName;
str fromEmail, toEmail, ccEmail, subject, emailBody, errorLog;
/// <summary>
/// Use for fatch Custinvoicejour Table.
/// </summary>
/// <param name = "_custInvoiceJour">_custInvoiceJour</param>
/// <returns>custInvoiceJour</returns>
public CustInvoiceJour parmCustInvoiceJour(CustInvoiceJour _custInvoiceJour)
{
custInvoiceJour = _custInvoiceJour;
return custInvoiceJour;
}
/// <summary>
/// Using this method we can fatch the EmailMessageSystemTable
/// </summary>
/// <returns>emailMessageSystemTable</returns>
public SysEmailMessageSystemTable emailMessageSystemTable()
{
select firstonly emailMessageSystemTable
where emailMessageSystemTable.EmailId == 'Sales_Inv1';
return emailMessageSystemTable;
}
/// <summary>
/// Using this method we can fatch the EmailSystm
/// </summary>
/// <returns>emailSystemTable</returns>
public SysEmailSystemTable emailSystemTable()
{
select firstonly emailSystemTable
where emailSystemTable.EmailId == 'Sales_Inv1';
return emailSystemTable;
}
/// <summary>
/// This method will use to Create a Mail Body
/// </summary>
/// <returns>emailBody</returns>
public str mailBody()
{
//this.emailMessageSystemTable();
descriptionString = strFmt(emailMessageSystemTable.mail,emailSystemTable.SenderName);
emailBody = descriptionString;
return emailBody;
}
/// <summary>
/// This method will use to Fatch ToEmail.
/// </summary>
public void getToEmailId()
{
toEmail = 'yourtoemail@gmail.com';
}
/// <summary>
/// This Method will use to Set the Email Subject.
/// </summary>
public void getSubject()
{
//this.emailMessageSystemTable();
subject = emailMessageSystemTable.Subject;
}
/// <summary>
/// This is Message Builder Method
/// </summary>
public void initMessageBuilder()
{
messageBuilder = new SysMailerMessageBuilder();
}
/// <summary>
/// This Method will use to Get From Email Id.
/// </summary>
public void getFromEmailId()
{
fromEmail = SysEmailSystemTable.SenderAddr;
}
/// <summary>
/// This run method will use to Run Email.
/// </summary>
public void run()
{
try
{
this.initMessageBuilder();
this.getFromEmailId();
this.getToEmailId();
//this.uploadSalesInvoicePrint();
this.getSubject();
System.Exception exception;
if (this.validate())
{
emailBody = this.mailBody();
try
{
this.sendEmail();
}
catch
{
SysInfologEnumerator infologEnumerator;
str errorMessage;
infologEnumerator = SysInfologEnumerator::newData(infolog.infologData());
//looping the enumerator to get the value.
while (infologEnumerator.moveNext())
{
//sorting based on the exception type and adding my custom message
switch (infologEnumerator.currentException())
{
case Exception::Info:
Message::Add(MessageSeverity::Informational, infologEnumerator.currentMessage());
break;
case Exception::Warning:
Message::Add(MessageSeverity::Warning, infologEnumerator.currentMessage());
break;
case Exception::Error:
errorMessage = infologEnumerator.currentMessage();
Message::Add(MessageSeverity::Error, errorMessage);
break;
case Exception::CLRError:
errorMessage = infologEnumerator.currentMessage();
Message::Add(MessageSeverity::Error, errorMessage);
break;
}
}
}
info("@LabelFile:EmailSuccessfullySend");
}
}
catch (Exception::Error)
{
errorLog = con2Str(infolog.infologData());
throw Error(strFmt("@LabelFile:Error",infolog.infologData()));
}
}
/// <summary>
/// This method will Through the Error if validation return true.
/// </summary>
/// <returns>ret</returns>
public boolean validate()
{
boolean ret = true;
if (!fromEmail)
{
errorLog += "@LabelFile:FromEmailErrorLog" ;
ret = checkFailed("@LabelFile:FromEmailThrowError");
}
if (!toEmail)
{
errorLog += "@LabelFile:ToEmailErrorLog" ;
ret = checkFailed("@LabelFile:ToEmailThrowError");
}
if (!subject)
{
errorLog += "@LabelFile:SubjectErrorLog" ;
ret = checkFailed("@LabelFile:SubjectThrowError");
}
return ret;
}
/// <summary>
/// This method will send the email.
/// </summary>
public void sendEmail()
{
messageBuilder.addTo(toEmail).addCc(ccEmail).setSubject(subject).setBody(emailBody);
messageBuilder.setFrom(fromEmail);
messageBuilder.addAttachment(fileStream,fileName);
SysMailerFactory::getNonInteractiveMailer().sendNonInteractive(messageBuilder.getMessage());
}
}
Example for run this code:
public void processOperation(SalesInvoicePostSendEmailBatchContract _conract)
{
SendEmail_SalesInvoicePost sendEmail = new SendEmail_SalesInvoicePost();
CustInvoiceJour custInvoiceJour;
InvoiceId invoiceId = _conract.parmInvoiceId();
select custInvoiceJour
where custInvoiceJour.InvoiceId == invoiceId;
sendEmail.parmcustInvoiceJour(custInvoiceJour);
sendEmail.emailMessageSystemTable();
sendEmail.emailSystemTable();
sendEmail.run();
}
1
Subscribe to my newsletter
Read articles from Harin Patel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Harin Patel
Harin Patel
Dynamic D365 AX Developer