How to Create Custom Animations with Tailwind CSS in ReactJS
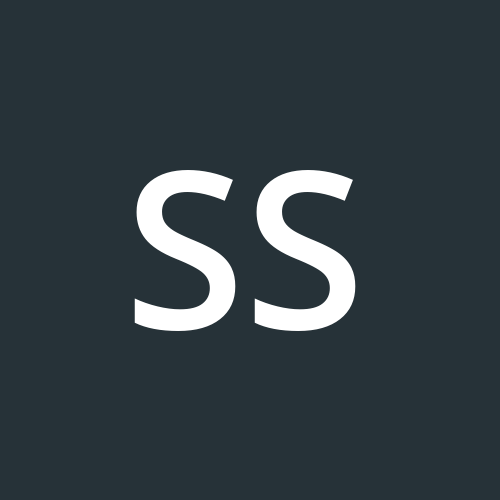
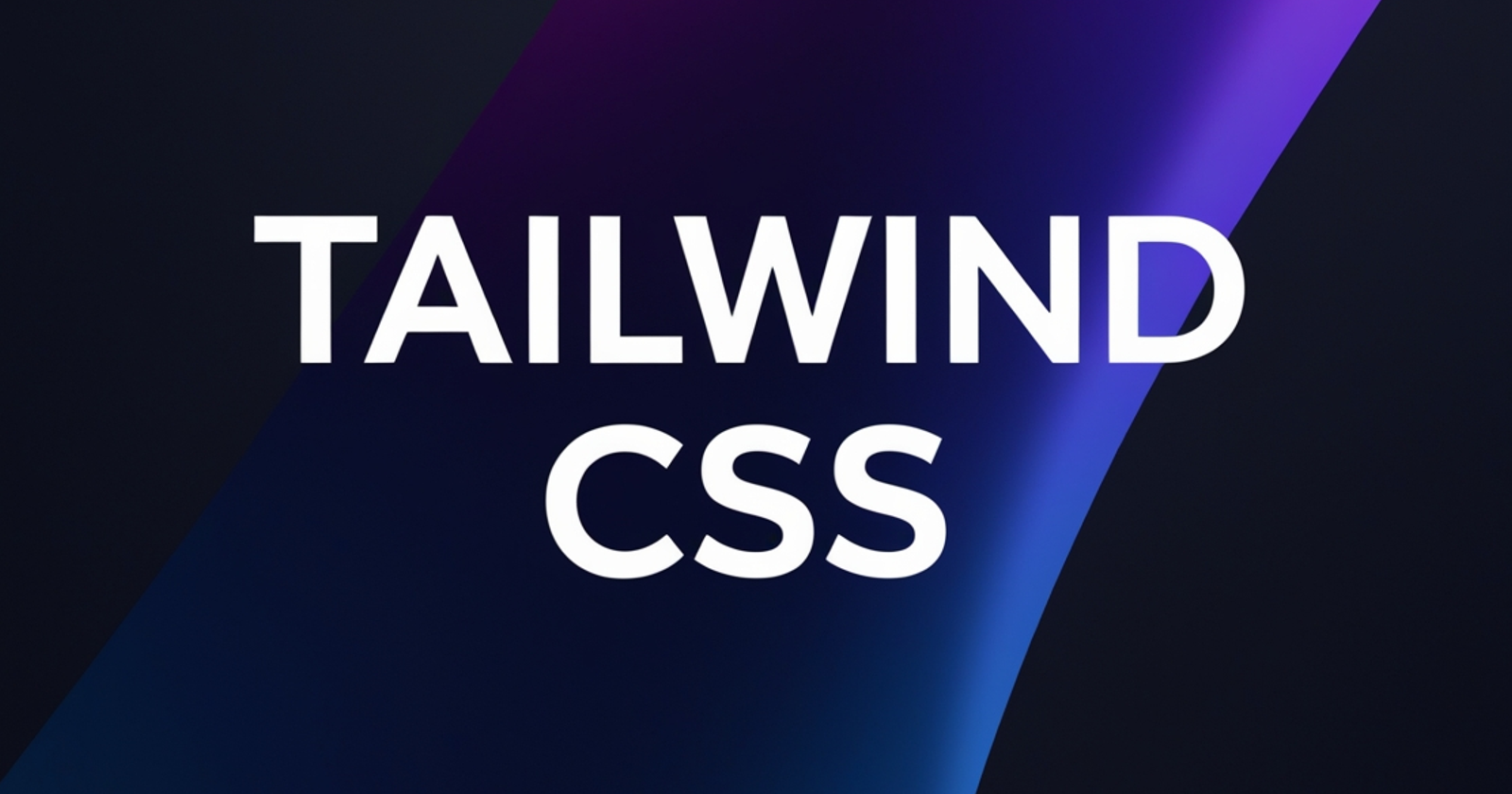
Sometimes, the animation properties that Tailwind provides are not enough, and we need a custom animation. For instance, if you want to display a fade-in effect when your page loads, you need to add some configuration for this animation. This is a one-time animation, but since Tailwind doesn't include it by default, we need to create a new class. By default, Tailwind only provides Spin, Ping, Pulse, and Bounce animations. Let's create a fade-in animation that we can use throughout our projects.
Installing tailwind in your project
You can follow this guide for your specific framework.
Link to Tailwind CSS configuration for your project: https://tailwindcss.com/docs/installation/framework-guides
npm install -D tailwindcss postcss autoprefixer
npx tailwindcss init -p
The commands above install Tailwind CSS and create the tailwind.config.js
file for our project.
Add the configuration for tailwind
/** @type {import('tailwindcss').Config} */
export default {
content: [
"./index.html",
"./src/**/*.{js,ts,jsx,tsx}",
],
theme: {
extend: {},
},
plugins: [],
}
If you installed Tailwind correctly, your tailwind.config.js
should look like the example above.
@tailwind base;
@tailwind components;
@tailwind utilities;
Add the Tailwind directives to your index.css
as shown above.
Adding the Animation
Now that your Tailwind setup is complete, let's add the animation. We will focus on the extend property inside the tailwind.config.js
file. Let's create a keyframe named fade
. This keyframe will be used in the animation property to create the animation.
/** @type {import('tailwindcss').Config} */
export default {
content: [],
theme: {
extend: {
keyframes: {
fade: {
"0%": { opacity: 0 },
"100%": { opacity: 1 },
},
}
},
},
plugins: [],
};
Inside the keyframes, add another property called animation and specify the keyword you want to use. This keyword will be used as a property.
/** @type {import('tailwindcss').Config} */
export default {
content: [],
theme: {
extend: {
keyframes: {
fade: {
"0%": { opacity: 0 },
"100%": { opacity: 1 },
},
},
animation: {
"fade-in": "fade 1s linear", // Add this...
},
},
},
plugins: [],
};
That's it! You can now use animate-fade-in
for a one-time animation when your page loads. You can add other animations in a similar way.
// Usage Example:
function App() {
return <h1 className="animate-fade-in">Hello!</h1>;
}
export default App;
Thank you for reading this! I hope it helps you with your projects.
Subscribe to my newsletter
Read articles from Shiwanshu Shubham directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
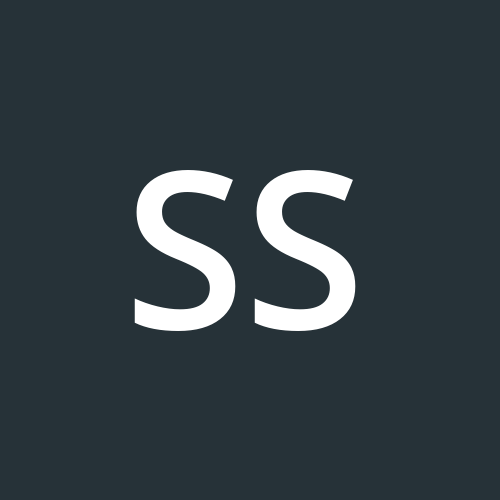
Shiwanshu Shubham
Shiwanshu Shubham
Hey there, I'm Shiwanshu, a passionate frontend developer and designer hailing from India. With proficiency in NextJS, ReactJS, CSS3, HTML5, Figma, UI Design, and Typescript, I've embarked on a journey into the tech space. This blog is a documentation of my progress as I delve deeper into the world of technology.