Comprehensive Guide to Spring Security
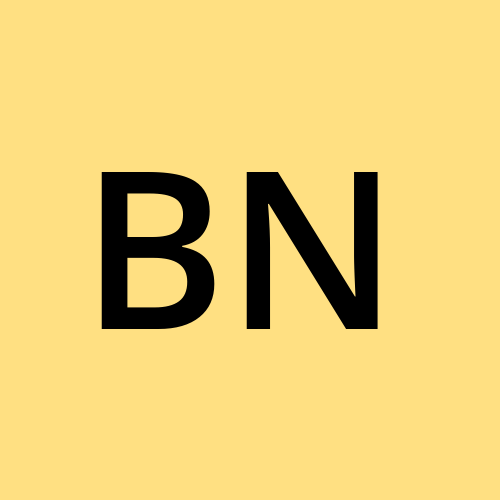
Table of contents
- Introduction to Spring Security
- Getting Started with Spring Security
- Authentication
- What is Authentication?
- 2. In-Memory Authentication
- 3. JDBC Authentication
- 4. LDAP Authentication
- 5. Custom Authentication Providers
- 1. What is a Custom Authentication Provider?
- 2. Why Use a Custom Authentication Provider?
- 3. How Does a Custom Authentication Provider Work?
- 4. Steps to Implement a Custom Authentication Provider
- 5. Flow of a Custom Authentication Provider
- 6. Advantages of Custom Authentication Providers
- 7. Real-World Use Cases
- Authorisation
- Form-Based Login
- HTTP Basic Authentication
- Token-Based Authentication
- OAuth2 and OpenID Connect
- Spring Security and RESTful APIs
- Method Security
- Password Management
- Session Management
- Security Context and Principal
- Remember-Me Authentication
- Exception Handling in Spring Security
- Best Practices and Advanced Topics
- Spring Security Extensions
- Conclusion
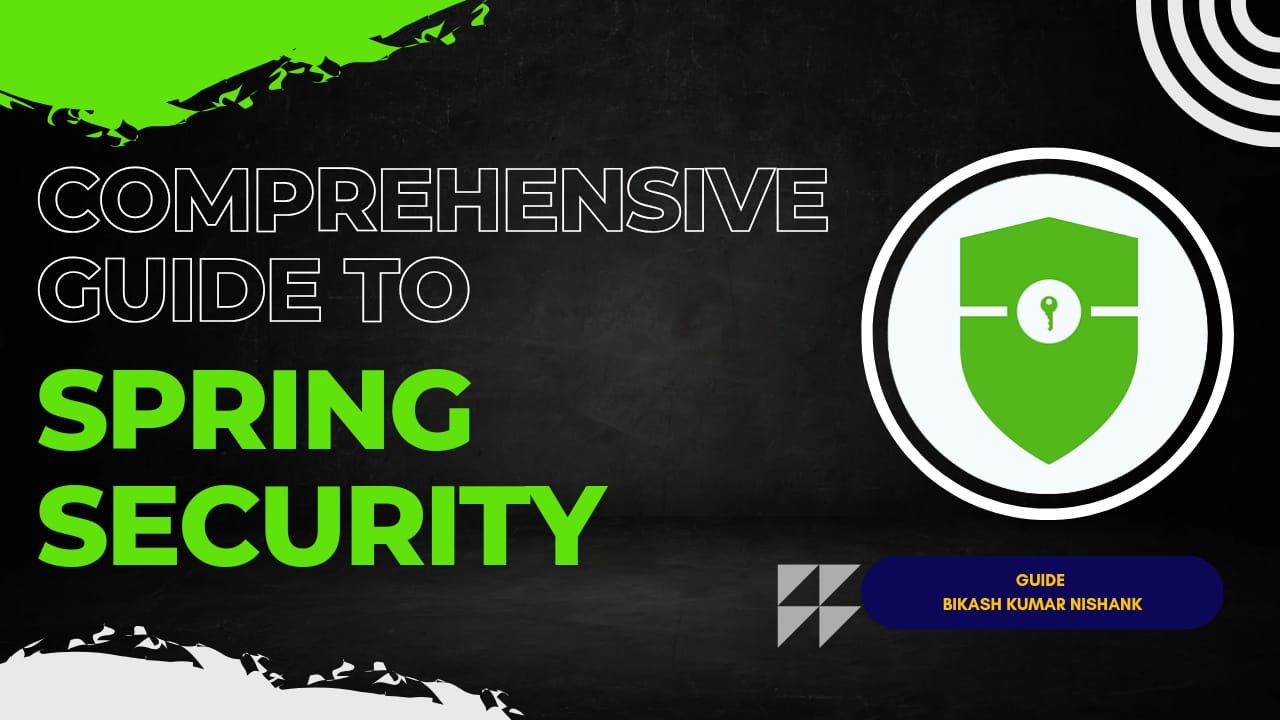
Introduction to Spring Security
What is Spring Security?
Spring Security is a powerful and highly customisable authentication and access control framework for Java applications. It is part of the larger Spring Framework ecosystem and provides comprehensive security features to protect applications from various security threats.
Benefits of Using Spring Security
Comprehensive Security:
Provides robust security mechanisms for authentication, authorization, and more.
Protects against common vulnerabilities like CSRF, XSS, and more.
Highly Customisable:
Flexible configuration options to suit various security requirements.
Supports custom authentication and authorization logic.
Integration with Other Spring Projects:
Seamlessly integrates with other Spring components like Spring Boot, Spring Data, and Spring MVC.
Simplifies the development of secure applications within the Spring ecosystem.
Ease of Use:
Offers sensible defaults and a straightforward API for common security tasks.
Reduces the amount of boilerplate code required for security configurations.
Extensibility:
Allows for extending and customizing almost every aspect of security.
Supports integration with various authentication mechanisms, such as LDAP, OAuth2, and more.
Overview of Spring Security Architecture
Spring Security's architecture is designed to be modular and flexible, making it easy to integrate security into Java applications. Key components include:
Security Filters:
Filter Chain: A sequence of servlet filters that process security logic before requests reach the application.
DelegatingFilterProxy: A standard servlet filter that delegates to a Spring-managed bean, enabling Spring Security to integrate into the servlet filter chain.
Authentication:
AuthenticationManager: The central interface responsible for processing authentication requests, delegating to one or more
AuthenticationProvider
instances.AuthenticationProvider: Components that perform the actual authentication logic (e.g.,
DaoAuthenticationProvider
for database authentication).UserDetailsService: An interface used to load user-specific data, typically from a database.
UserDetails: An interface representing the user information stored and managed by Spring Security.
Authorization:
AccessDecisionManager: A component that makes authorization decisions based on access control rules.
AccessDecisionVoter: Components that vote on whether access should be granted, such as
RoleVoter
andAuthenticatedVoter
.SecurityContext: Holds the security information for the current execution thread, including the
Authentication
object representing the authenticated user.SecurityContextHolder: A helper class that provides access to the
SecurityContext
.
Security Interceptors:
FilterSecurityInterceptor: The primary security interceptor for securing web requests, applying security logic defined by the
AccessDecisionManager
andSecurityMetadataSource
.MethodSecurityInterceptor: Secures method invocations using annotations like
@PreAuthorize
and@Secured
.
Security Configurations:
HttpSecurity: A fluent API for configuring web-based security at a high level, allowing the definition of security policies.
WebSecurityConfigurerAdapter: A base class providing default security configurations, extendable to customise security settings.
Example Configuration
Here is a basic example of a Spring Security configuration that secures all endpoints, uses form-based login, and sets up an in-memory user store:
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.authentication.builders.AuthenticationManagerBuilder;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication()
.withUser("user")
.password("{noop}password") // {noop} indicates no password encoding
.roles("USER")
.and()
.withUser("admin")
.password("{noop}admin")
.roles("ADMIN");
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.anyRequest().authenticated() // All requests require authentication
.and()
.formLogin()
.loginPage("/login") // Custom login page
.permitAll() // Allow everyone to see the login page
.and()
.logout()
.permitAll(); // Allow everyone to log out
}
}
This configuration secures all HTTP requests, requires authentication for every request, and uses a custom login page. It also sets up in-memory authentication with two users.
Getting Started with Spring Security
Adding Spring Security to a Spring Boot Application
To start using Spring Security in your Spring Boot application, you need to add the Spring Security dependency to your project. If you're using Maven, you can do this by adding the following dependency to your pom.xml
file:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
For Gradle users, add this line to your build.gradle
file:
implementation 'org.springframework.boot:spring-boot-starter-security'
Basic Configuration
After adding the dependency, Spring Security will automatically secure your application with some default configurations. By default, Spring Security:
Secures all endpoints.
Provides a default login page.
Requires a default user with a generated password (displayed in the console during application startup).
To customize these settings, you can create a security configuration class. This class should extend WebSecurityConfigurerAdapter
and override the necessary methods to define your custom security rules. Here's a basic example:
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.authentication.builders.AuthenticationManagerBuilder;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication()
.withUser("user")
.password("{noop}password") // {noop} indicates no password encoding
.roles("USER")
.and()
.withUser("admin")
.password("{noop}admin")
.roles("ADMIN");
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN") // Only ADMIN can access /admin/** URLs
.antMatchers("/user/**").hasRole("USER") // Only USER can access /user/** URLs
.antMatchers("/", "/home").permitAll() // Everyone can access the home page
.anyRequest().authenticated() // All other requests require authentication
.and()
.formLogin()
.loginPage("/login") // Custom login page
.permitAll() // Allow everyone to see the login page
.and()
.logout()
.permitAll(); // Allow everyone to log out
}
}
In this example:
configure(AuthenticationManagerBuilder auth)
: Sets up in-memory authentication with two users, each with different roles.configure(HttpSecurity http)
: Defines URL-based authorization rules and specifies a custom login page.
Understanding Security Filters
Security filters are a crucial part of Spring Security. They process HTTP requests and apply security measures before the requests reach your application's endpoints. Here’s a look at some of the key filters in the Spring Security filter chain:
SecurityContextPersistenceFilter:
- Manages the
SecurityContext
lifecycle, loading the security context at the beginning of a request and storing it at the end.
- Manages the
UsernamePasswordAuthenticationFilter:
- Processes form-based login requests. It attempts to authenticate the user by validating the username and password.
BasicAuthenticationFilter:
- Processes HTTP Basic authentication requests. This filter handles the
Authorization
header for HTTP Basic authentication.
- Processes HTTP Basic authentication requests. This filter handles the
CsrfFilter:
- Applies Cross-Site Request Forgery (CSRF) protection. This filter ensures that state-changing requests are legitimate and intended by the authenticated user.
LogoutFilter:
- Processes logout requests. It invalidates the user session and clears the
SecurityContext
.
- Processes logout requests. It invalidates the user session and clears the
ExceptionTranslationFilter:
- Translates security exceptions into HTTP responses. For example, it can redirect to the login page on authentication failure or return a 403 Forbidden status for access denial.
FilterSecurityInterceptor:
- The last filter in the chain. It handles authorization checks based on the security rules defined in your configuration.
Example of Customising Security Filters
You can customise the filter chain by adding your own filters or modifying existing ones. Here’s an example of adding a custom filter:
import org.springframework.context.annotation.Bean;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
import org.springframework.security.web.authentication.UsernamePasswordAuthenticationFilter;
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Bean
public CustomFilter customFilter() {
return new CustomFilter();
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.addFilterBefore(customFilter(), UsernamePasswordAuthenticationFilter.class)
.authorizeRequests()
.anyRequest().authenticated()
.and()
.formLogin().permitAll();
}
}
In this example, CustomFilter
is added to the filter chain before the UsernamePasswordAuthenticationFilter
. You can implement custom logic in CustomFilter
as needed.
Authentication
What is Authentication?
Authentication is the process of verifying the identity of a user or entity. In web applications, it typically involves checking credentials (like a username and password) to ensure that the user is who they claim to be. Upon successful authentication, the user is granted access to the system.
General Flow of Authentication in a Web Application:
User Requests Access: The user tries to access a protected resource (e.g., a dashboard page).
Authentication Filter: An authentication filter intercepts the request to check if the user is authenticated.
Authentication Manager: If the user is not authenticated, the filter forwards the request to an
AuthenticationManager
, which is responsible for handling the authentication process.Authentication Provider: The
AuthenticationManager
delegates the authentication process to one or moreAuthenticationProvider
instances. These providers can authenticate using different strategies (e.g., in-memory, JDBC, LDAP).User Details Service: The provider may call a
UserDetailsService
to load user-specific data, such as username, password, and roles.Credentials Check: The provider checks the user’s credentials against stored data (e.g., database, memory).
Authentication Success/Failure: If the credentials match, the user is authenticated, and a
SecurityContext
is established. If not, an authentication failure is returned.Access Granted/Denied: Based on the result, the user is either granted access to the requested resource or denied.
2. In-Memory Authentication
How It Works:
In-Memory Authentication is used to store user credentials directly in the application’s configuration or code. This is useful for testing or simple applications.
Example:
@Configuration
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication()
.withUser("user1").password("{noop}password1").roles("USER")
.and()
.withUser("admin").password("{noop}adminpass").roles("ADMIN");
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/admin").hasRole("ADMIN")
.antMatchers("/user").hasRole("USER")
.anyRequest().authenticated()
.and()
.formLogin();
}
}
Flow of In-Memory Authentication:
Request: User tries to access
/admin
or/user
.Authentication Filter: The filter intercepts the request and checks if the user is authenticated.
In-Memory Authentication: The credentials provided by the user are compared against those stored in the configuration (
user1/password1
oradmin/adminpass
).Success/Failure: If the credentials match, the user is authenticated and can access the resource based on their role. If not, access is denied.
Explanation:
The
{noop}
prefix is used to indicate that no password encoding is applied (not recommended for production)..roles("USER")
assigns a role to the user. Roles help in authorization, determining what resources the user can access.
3. JDBC Authentication
How It Works:
JDBC Authentication uses a relational database to store user credentials and roles. The application queries the database to verify user credentials.
Database Setup: You would typically have two tables:
users: Stores usernames, passwords, and account status.
authorities: Stores roles associated with each user.
CREATE TABLE users (
username VARCHAR(50) NOT NULL PRIMARY KEY,
password VARCHAR(100) NOT NULL,
enabled BOOLEAN NOT NULL
);
CREATE TABLE authorities (
username VARCHAR(50) NOT NULL,
authority VARCHAR(50) NOT NULL,
FOREIGN KEY (username) REFERENCES users(username)
);
Example:
@Configuration
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private DataSource dataSource;
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.jdbcAuthentication()
.dataSource(dataSource)
.usersByUsernameQuery("select username, password, enabled from users where username=?")
.authoritiesByUsernameQuery("select username, authority from authorities where username=?");
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/admin").hasRole("ADMIN")
.antMatchers("/user").hasRole("USER")
.anyRequest().authenticated()
.and()
.formLogin();
}
}
Flow of JDBC Authentication:
Request: User tries to access
/admin
or/user
.Authentication Filter: The filter checks if the user is authenticated.
JDBC Authentication: The system queries the database to retrieve the user’s credentials using the
usersByUsernameQuery
.- Example query:
SELECT username, password, enabled FROM users WHERE username='user1'
.
- Example query:
Role Retrieval: The system retrieves the user’s roles using the
authoritiesByUsernameQuery
.- Example query:
SELECT username, authority FROM authorities WHERE username='user1'
.
- Example query:
Password Comparison: The password provided by the user is compared to the hashed password stored in the database.
Success/Failure: If credentials match and the account is enabled, the user is authenticated. If not, access is denied.
Explanation:
dataSource
: Provides the connection to the database.usersByUsernameQuery
: Queries theusers
table to fetch the user’s details.authoritiesByUsernameQuery
: Queries theauthorities
table to fetch the user’s roles.
4. LDAP Authentication
How It Works:
LDAP Authentication uses an LDAP directory service to manage user credentials and roles. LDAP is often used in enterprise environments where centralised user management is required.
LDAP Directory Structure: LDAP directories are structured hierarchically. Users are typically stored under organisational units (OUs). For example:
dc=example,dc=com
|
|-- ou=people
| |-- uid=user1
| |-- uid=admin
|
|-- ou=groups
|-- cn=admins
|-- cn=users
Example:
@Configuration
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.ldapAuthentication()
.userDnPatterns("uid={0},ou=people")
.groupSearchBase("ou=groups")
.contextSource()
.url("ldap://localhost:8389/dc=example,dc=com")
.and()
.passwordCompare()
.passwordEncoder(new LdapShaPasswordEncoder())
.passwordAttribute("userPassword");
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/admin").hasRole("ADMIN")
.antMatchers("/user").hasRole("USER")
.anyRequest().authenticated()
.and()
.formLogin();
}
}
Flow of LDAP Authentication:
Request: User tries to access
/admin
or/user
.Authentication Filter: The filter checks if the user is authenticated.
LDAP Authentication: The system connects to the LDAP server using the
contextSource
.User Search: The system searches for the user in the LDAP directory using
userDnPatterns
.- Example DN:
uid=user1,ou=people,dc=example,dc=com
.
- Example DN:
Password Comparison: The password provided by the user is compared to the hashed password stored in the LDAP directory using
passwordCompare
.Group Search: The system searches for the user’s roles in the
ou=groups
organizational unit usinggroupSearchBase
.Success/Failure: If the credentials match and the user exists in the LDAP directory, the user is authenticated. If not, access is denied.
Explanation:
userDnPatterns
: Defines the pattern to locate the user in the LDAP directory.groupSearchBase
: Specifies where to search for user roles in the LDAP directory.contextSource
: Configures the connection to the LDAP server.passwordCompare
: Compares the provided password with the stored password using a specified encoder (LdapShaPasswordEncoder
in this case).
5. Custom Authentication Providers
In Spring Security, an AuthenticationProvider is an interface that provides a mechanism to authenticate a user. While Spring Security comes with built-in authentication providers (e.g., In-Memory, JDBC, LDAP), there are scenarios where you might need to implement your own custom authentication logic. This is where Custom Authentication Providers come into play.
1. What is a Custom Authentication Provider?
A Custom Authentication Provider allows you to implement your own authentication logic, tailored to the specific needs of your application. For instance, you might need to authenticate users against a legacy system, a third-party service, or even a custom API.
2. Why Use a Custom Authentication Provider?
You might need a custom provider when:
Integrating with Legacy Systems: Your user credentials are stored in a non-standard format or system.
Third-Party Services: You need to authenticate against a service that isn't supported out-of-the-box (e.g., a custom OAuth provider).
Complex Authentication Logic: Your application requires more complex rules than what the standard providers can handle.
Multiple Authentication Mechanisms: You need to combine multiple authentication mechanisms in a single application.
3. How Does a Custom Authentication Provider Work?
A Custom Authentication Provider implements the AuthenticationProvider
interface and provides its own logic for authentication. The main method to implement is authenticate()
, which processes the authentication request and returns an Authentication
object if successful.
4. Steps to Implement a Custom Authentication Provider
Step 1: Implement the AuthenticationProvider
Interface
Here's a simple example where we authenticate users against a custom system.
import org.springframework.security.authentication.AuthenticationProvider;
import org.springframework.security.core.Authentication;
import org.springframework.security.core.AuthenticationException;
import org.springframework.security.core.userdetails.User;
import org.springframework.security.core.userdetails.UserDetails;
import org.springframework.security.core.userdetails.UserDetailsService;
import org.springframework.security.core.userdetails.UsernameNotFoundException;
import org.springframework.security.authentication.UsernamePasswordAuthenticationToken;
import org.springframework.security.core.authority.SimpleGrantedAuthority;
import org.springframework.stereotype.Component;
import java.util.Collections;
@Component
public class CustomAuthenticationProvider implements AuthenticationProvider {
private final UserDetailsService userDetailsService;
public CustomAuthenticationProvider(UserDetailsService userDetailsService) {
this.userDetailsService = userDetailsService;
}
@Override
public Authentication authenticate(Authentication authentication) throws AuthenticationException {
String username = authentication.getName();
String password = authentication.getCredentials().toString();
// Custom logic to authenticate the user
UserDetails user = userDetailsService.loadUserByUsername(username);
if (user == null) {
throw new UsernameNotFoundException("User not found");
}
if (!"customPassword".equals(password)) {
throw new AuthenticationException("Invalid password") {};
}
return new UsernamePasswordAuthenticationToken(
username, password, Collections.singletonList(new SimpleGrantedAuthority("ROLE_USER")));
}
@Override
public boolean supports(Class<?> authentication) {
return authentication.equals(UsernamePasswordAuthenticationToken.class);
}
}
Explanation:
authenticate()
Method: This method contains the custom logic to authenticate the user. In the example, we first retrieve the user details using aUserDetailsService
. We then compare the provided password with a hardcoded value"customPassword"
. If the password matches, we create and return anAuthentication
object; otherwise, we throw an exception.supports()
Method: This method tells Spring Security which type of authentication this provider supports. In this case, it supportsUsernamePasswordAuthenticationToken
.
Step 2: Register the Custom Authentication Provider
You need to register your custom authentication provider in the security configuration.
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.authentication.builders.AuthenticationManagerBuilder;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
private final CustomAuthenticationProvider customAuthenticationProvider;
public SecurityConfig(CustomAuthenticationProvider customAuthenticationProvider) {
this.customAuthenticationProvider = customAuthenticationProvider;
}
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.authenticationProvider(customAuthenticationProvider);
}
}
Explanation:
configure(AuthenticationManagerBuilder auth)
: In this method, we register the custom authentication provider usingauth.authenticationProvider(customAuthenticationProvider)
.
5. Flow of a Custom Authentication Provider
User Request: A user tries to log in with their credentials.
Authentication Filter: The authentication filter intercepts the request and passes it to the
AuthenticationManager
.Authentication Manager Delegation: The
AuthenticationManager
delegates the request to the registeredAuthenticationProvider
.Custom Authentication Logic: The
CustomAuthenticationProvider
processes the authentication logic, checking the credentials against the custom rules or external systems.Success/Failure:
On Success: If the credentials are valid, the
CustomAuthenticationProvider
returns anAuthentication
object, establishing the user's identity and roles.On Failure: If the credentials are invalid, an exception is thrown, and the user is denied access.
6. Advantages of Custom Authentication Providers
Flexibility: Allows integration with virtually any authentication system or service.
Control: Provides complete control over the authentication process, enabling the implementation of complex business rules.
Extensibility: Can be extended to support multiple authentication mechanisms or to add additional checks, such as multi-factor authentication.
7. Real-World Use Cases
Legacy Systems: Authenticating against a legacy system where credentials are stored in a custom format or require specific processing.
Third-Party Services: Authenticating against third-party APIs or services that require custom headers, tokens, or communication protocols.
Hybrid Authentication: Combining multiple authentication methods, such as integrating with an external OAuth provider while also checking internal credentials.
Authorisation
What is Authorisation?
Definition: Authorisation is the process that determines what a user is allowed to do after they have been authenticated. It involves checking permissions and roles to ensure users can only access resources or perform actions they are permitted to. Unlike authentication, which verifies a user’s identity, authorisation ensures that the authenticated user has the right permissions.
Example: After logging into an e-commerce website, a customer might be authorised to browse products and place orders but not to access the admin dashboard or change site settings.
Use Case: In a hospital management system, doctors might be authorised to access patient records but not to modify hospital settings or manage administrative tasks.
Role-Based Access Control (RBAC)
Definition: Role-Based Access Control (RBAC) assigns permissions based on user roles. Each role has a set of permissions, and users are assigned roles according to their job functions. This approach simplifies the management of permissions by grouping them into roles rather than managing them individually.
Example Configuration:
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication()
.withUser("john")
.password("{noop}password")
.roles("USER")
.and()
.withUser("admin")
.password("{noop}admin")
.roles("ADMIN");
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN") // Admins only
.antMatchers("/user/**").hasRole("USER") // Users only
.and()
.formLogin().permitAll()
.and()
.logout().permitAll();
}
}
Explanation:
hasRole("ADMIN")
: Restricts access to paths under/admin/**
to users with the "ADMIN" role.hasRole("USER")
: Restricts access to paths under/user/**
to users with the "USER" role.
Real-World Use Case:
- Corporate Applications: In a company, employees might be assigned roles like "Manager" or "Staff". Each role has different access levels, such as managers having access to sensitive reports and staff having access to general information.
Authority-Based Access Control
Definition: Authority-Based Access Control (ABAC) is a more granular approach compared to RBAC. It focuses on permissions or authorities that a user has, which can be fine-tuned beyond roles. Users are granted specific authorities that determine their access levels.
Example Configuration:
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication()
.withUser("alice")
.password("{noop}password")
.authorities("READ_PRIVILEGES", "WRITE_PRIVILEGES")
.and()
.withUser("bob")
.password("{noop}password")
.authorities("READ_PRIVILEGES");
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/edit/**").hasAuthority("WRITE_PRIVILEGES") // Requires WRITE_PRIVILEGES
.antMatchers("/view/**").hasAuthority("READ_PRIVILEGES") // Requires READ_PRIVILEGES
.and()
.formLogin().permitAll()
.and()
.logout().permitAll();
}
}
Explanation:
hasAuthority("WRITE_PRIVILEGES")
: Restricts access to paths under/edit/**
to users with "WRITE_PRIVILEGES".hasAuthority("READ_PRIVILEGES")
: Restricts access to paths under/view/**
to users with "READ_PRIVILEGES".
Real-World Use Case:
- Content Management Systems: A CMS might have different authorities for actions like "publish", "edit", and "view". This allows detailed control over what each user can do, beyond just having a role.
Method Security
Definition: Method security allows you to secure specific methods within your service classes using annotations. It provides a way to enforce security constraints directly at the method level, based on roles, permissions, or other criteria.
Example Configuration:
import org.springframework.security.access.annotation.Secured;
import org.springframework.security.access.prepost.PreAuthorize;
import org.springframework.stereotype.Service;
@Service
public class DocumentService {
@Secured("ROLE_ADMIN")
public void deleteDocument(Long documentId) {
// Only users with ROLE_ADMIN can delete documents
}
@PreAuthorize("hasAuthority('READ_PRIVILEGES')")
public void viewDocument(Long documentId) {
// Only users with READ_PRIVILEGES can view documents
}
}
Explanation:
@Secured("ROLE_ADMIN")
: Ensures that only users with the "ADMIN" role can access thedeleteDocument
method.@PreAuthorize("hasAuthority('READ_PRIVILEGES')")
: Restricts access to theviewDocument
method to users with "READ_PRIVILEGES".
Real-World Use Case:
- Financial Services: In a banking application, you might have methods to transfer funds that should only be accessible to authorized users. Method security ensures that these methods are protected according to the user’s permissions.
Domain Object Security
Definition: Domain object security involves securing access to specific instances of domain objects based on user permissions. It ensures that users can only access or manipulate objects they are permitted to, often by checking ownership or other attributes.
Example Configuration:
import org.springframework.security.access.prepost.PostAuthorize;
import org.springframework.stereotype.Service;
@Service
public class AccountService {
@PostAuthorize("returnObject.owner == authentication.name")
public Account getAccountById(Long accountId) {
// Returns an Account object; user can only access if they own the account
return accountRepository.findById(accountId).orElse(null);
}
}
Explanation:
@PostAuthorize("returnObject.owner ==
authentication.name
")
: After retrieving theAccount
object, this annotation ensures that the user can only access it if they are the owner of the account.
Real-World Use Case:
- Personal Account Management: In a banking application, users should only be able to view or modify their own account details. Domain object security enforces this by checking the ownership of the account object.
Form-Based Login
Form-based login is one of the most common methods of authentication in web applications. It involves a user submitting a login form with a username and password, which the server then authenticates.
Workflow of Form-Based Login
User Requests a Protected Resource:
The user tries to access a URL that requires authentication.
If the user is not authenticated, they are redirected to a login page.
User Submits Login Form:
The user fills out a login form with their username and password and submits it.
The form data is sent as a POST request to a specific URL (e.g.,
/login
).
Authentication Process:
Spring Security intercepts the request and uses an
AuthenticationManager
to validate the credentials.The
AuthenticationManager
typically uses anAuthenticationProvider
to authenticate the user (e.g., In-Memory, JDBC, or Custom Authentication Provider).
Success or Failure:
If authentication is successful, the user is redirected to the originally requested URL or a default URL.
If authentication fails, the user is redirected back to the login page with an error message.
Post-Authentication:
A
SecurityContext
is created to store the user's authentication information.The user can now access protected resources based on their roles and permissions.
Configuring Form-Based Login
Form-based login can be configured in Spring Security with just a few lines of code.
Basic Configuration
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/login", "/public/**").permitAll()
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login") // URL of the custom login page
.loginProcessingUrl("/perform_login") // URL to submit the username and password to
.defaultSuccessUrl("/homepage", true) // URL to redirect to after successful login
.failureUrl("/login?error=true") // URL to redirect to if login fails
.and()
.logout()
.logoutUrl("/perform_logout")
.deleteCookies("JSESSIONID")
.logoutSuccessUrl("/login?logout=true");
}
}
Explanation:
authorizeRequests()
: Configures which URLs are accessible to everyone and which require authentication.formLogin()
: Enables form-based authentication.loginPage()
: Specifies the URL for the custom login page.loginProcessingUrl()
: The URL where the login form POSTs data for processing (typically/login
).defaultSuccessUrl()
: The URL the user is redirected to after successful login.failureUrl()
: The URL the user is redirected to if login fails.
Custom Login Page
By default, Spring Security provides a basic login page. However, you can easily create a custom login page to match your application’s design.
Creating a Custom Login Page
HTML Example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Login Page</title>
</head>
<body>
<h2>Login</h2>
<form method="POST" action="/perform_login">
<div>
<label for="username">Username:</label>
<input type="text" id="username" name="username" required>
</div>
<div>
<label for="password">Password:</label>
<input type="password" id="password" name="password" required>
</div>
<div>
<button type="submit">Login</button>
</div>
<div>
<span style="color:red;">
<!-- Error message placeholder -->
<sec:authorize access="hasAuthority('ROLE_ANONYMOUS') and #request.getParameter('error') == 'true'">
Invalid username or password.
</sec:authorize>
</span>
</div>
</form>
</body>
</html>
Explanation:
The form’s
action
attribute is set to/perform_login
, matching theloginProcessingUrl()
in the security configuration.The
sec:authorize
tag (part of Spring Security's tag library) can be used to show or hide elements based on the user's authentication state or roles.
Configuring the Custom Login Page in Spring Security
As shown in the previous configuration, you can use .loginPage("/login")
to specify the custom login page.
.formLogin()
.loginPage("/login") // Specify the custom login page URL
.loginProcessingUrl("/perform_login")
.defaultSuccessUrl("/homepage", true)
.failureUrl("/login?error=true")
Handling Login Errors
Handling login errors gracefully is important to provide a good user experience. Spring Security allows you to specify what should happen when authentication fails.
Redirecting to the Login Page with an Error Message
In the configuration, you can set the failureUrl()
to redirect to the login page with an error message.
.formLogin()
.loginPage("/login")
.loginProcessingUrl("/perform_login")
.defaultSuccessUrl("/homepage", true)
.failureUrl("/login?error=true")
Displaying the Error Message on the Login Page
You can modify your custom login page to display an error message based on the presence of a request parameter.
Modified Login Page:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Login Page</title>
</head>
<body>
<h2>Login</h2>
<form method="POST" action="/perform_login">
<div>
<label for="username">Username:</label>
<input type="text" id="username" name="username" required>
</div>
<div>
<label for="password">Password:</label>
<input type="password" id="password" name="password" required>
</div>
<div>
<button type="submit">Login</button>
</div>
<div>
<span style="color:red;">
<!-- Display error message if login failed -->
<sec:authorize access="isAnonymous()">
<sec:authentication property="details.sessionId" var="sessionId"/>
<c:if test="${not empty param.error}">
Invalid username or password.
</c:if>
</sec:authorize>
</span>
</div>
</form>
</body>
</html>
Explanation:
param.error
: Checks if theerror
parameter is present in the request (set by thefailureUrl("/login?error=true")
).sec:authorize
andsec:authentication
: These are part of Spring Security's JSP tag library, used to display content based on the user's authentication status.
HTTP Basic Authentication
HTTP Basic Authentication is a simple authentication scheme built into the HTTP protocol. It requires the client to send the Authorization
header with the username and password encoded in Base64 on each request. Spring Security provides easy integration for HTTP Basic Authentication.
How HTTP Basic Authentication Works
Client Request: The client requests a protected resource from the server.
Server Response: If the request is unauthenticated, the server responds with a
401 Unauthorized
status and includes aWWW-Authenticate
header, indicating that basic authentication is required.Client Re-sends Request: The client resends the request with an
Authorization
header containing the credentials (username and password) encoded in Base64.Server Authentication: The server decodes the credentials and authenticates the user.
Access Granted or Denied:
If the credentials are valid, the server grants access to the requested resource.
If invalid, the server responds with a
401 Unauthorized
status again.
Configuring HTTP Basic Authentication in Spring Security
Spring Security makes it straightforward to set up HTTP Basic Authentication.
Basic Configuration
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/public/**").permitAll()
.anyRequest().authenticated()
.and()
.httpBasic(); // Enables HTTP Basic Authentication
}
}
Explanation:
authorizeRequests()
: Configures which URLs are accessible without authentication (e.g.,/public/**
) and which require authentication.httpBasic()
: This method enables HTTP Basic Authentication. When this is set, Spring Security will intercept requests and require anAuthorization
header containing a valid Base64-encoded username and password.
Customizing HTTP Basic Authentication
You can further customize the HTTP Basic Authentication behavior by specifying entry points and authentication details.
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
import org.springframework.security.web.AuthenticationEntryPoint;
import org.springframework.security.web.authentication.www.BasicAuthenticationEntryPoint;
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/public/**").permitAll()
.anyRequest().authenticated()
.and()
.httpBasic()
.authenticationEntryPoint(authenticationEntryPoint()); // Custom Entry Point
}
@Bean
public AuthenticationEntryPoint authenticationEntryPoint() {
BasicAuthenticationEntryPoint entryPoint = new BasicAuthenticationEntryPoint();
entryPoint.setRealmName("my-app-realm");
return entryPoint;
}
}
Explanation:
authenticationEntryPoint()
: Defines a customBasicAuthenticationEntryPoint
that can be used to set a realm name for the authentication prompt. The realm name appears in the authentication dialog box shown to the user.setRealmName("my-app-realm")
: Sets the realm name to "my-app-realm". This is part of the challenge sent back to the client and can be customized to match your application's branding or security requirements.
Advantages of HTTP Basic Authentication
Simplicity:
Easy to implement and requires minimal configuration.
Built into the HTTP protocol, so it is supported by all modern browsers and HTTP clients.
Stateless:
- Since credentials are sent with every request, HTTP Basic Authentication is stateless, making it suitable for RESTful APIs where session management is not desirable.
Wide Compatibility:
- Supported by various HTTP clients, tools, and libraries out of the box.
No Session Management Required:
- No need for session handling or cookies, as credentials are sent with each request.
Disadvantages of HTTP Basic Authentication
Security Concerns:
Plaintext Transmission: Although credentials are Base64 encoded, this does not provide any encryption. Without HTTPS, the credentials can easily be intercepted and decoded by an attacker.
Replay Attacks: Since the credentials are the same for every request, they are susceptible to replay attacks if intercepted.
User Experience:
Browser Prompts: Browsers typically show a modal dialog for HTTP Basic Authentication, which can be jarring and difficult to customize.
Credential Re-entry: Users may need to enter credentials repeatedly if the session is interrupted or cookies are cleared.
Not Suitable for Modern Web Applications:
- Modern web applications often require more sophisticated authentication mechanisms (e.g., OAuth, JWT) that provide better security and user experience.
Limited Customization:
- Customizing the login experience or adding multi-factor authentication is difficult with HTTP Basic Authentication.
Best Practices for Using HTTP Basic Authentication
Always Use HTTPS:
- To mitigate the risk of credentials being intercepted, always use HTTPS to encrypt the communication channel.
Limit Usage to APIs:
- HTTP Basic Authentication is more suitable for APIs rather than web applications. For web applications, consider using more secure and user-friendly authentication methods like form-based login or OAuth.
Token-Based Alternatives:
- Consider using token-based authentication (e.g., JWT) for better security and scalability, especially in distributed systems.
Regularly Rotate Credentials:
- Implement policies for regular password rotation to minimise the risk if credentials are compromised.
Combine with IP Whitelisting:
- To add an extra layer of security, you can restrict access to certain IP addresses or networks.
Token-Based Authentication
Token-based authentication is a method where, after the initial login, the server issues a token to the client. This token is then sent with each request to verify the user’s identity. This approach is particularly useful for stateless authentication, where the server does not store any session information.
1. Introduction to JWT (JSON Web Tokens)
JWT (JSON Web Token) is a compact and self-contained way of representing claims between two parties. The JWT consists of three parts:
Header: Contains metadata about the token, such as the signing algorithm.
Payload: Contains the claims or the information being transmitted.
Signature: Ensures the token hasn’t been tampered with.
Example JWT:
eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJzdWIiOiIxMjM0NTY3ODkwIiwibmFtZSI6IkpvaG4gRG9lIiwiaWF0IjoxNTE2MjM5MDIyfQ.SflKxwRJSMeKKF2QT4fwpMeJf36POk6yJV_adQssw5c
2. Creating and Validating JWTs
Let’s see how to create and validate JWTs using Java and the io.jsonwebtoken
library.
Step 1: Add Dependency
Include the jjwt
dependency in your pom.xml
if using Maven:
<dependency>
<groupId>io.jsonwebtoken</groupId>
<artifactId>jjwt</artifactId>
<version>0.9.1</version>
</dependency>
Step 2: Creating a JWT
Here’s a Java example to create a JWT:
import io.jsonwebtoken.Jwts;
import io.jsonwebtoken.SignatureAlgorithm;
import java.util.Date;
public class JwtUtil {
// Secret key used for signing the JWT.
// In production, ensure this is securely stored.
private static final String SECRET_KEY = "mySecretKey";
// Method to create a JWT
public static String createJWT(String id, String subject, long ttlMillis) {
long nowMillis = System.currentTimeMillis(); // Current time in milliseconds
Date now = new Date(nowMillis);
// Create and sign the JWT, then serialize it to a compact, URL-safe string
return Jwts.builder()
.setId(id) // Unique identifier for the JWT
.setSubject(subject) // The subject of the JWT, usually user information
.setIssuedAt(now) // Timestamp of when the JWT was issued
.setExpiration(new Date(nowMillis + ttlMillis)) // Expiration time of the JWT
.signWith(SignatureAlgorithm.HS256, SECRET_KEY) // Sign the JWT using HS256 algorithm and secret key
.compact(); // Serialize the JWT to a URL-safe string
}
public static void main(String[] args) {
// Generate a JWT with a unique ID, subject, and validity of 10 minutes
String jwt = createJWT("1", "user@example.com", 600000);
System.out.println("Generated JWT: " + jwt);
}
}
Explanation:
setId
: Adds a unique identifier to the JWT.setSubject
: Sets the subject (e.g., user ID or email).setIssuedAt
: Sets the token issuance timestamp.setExpiration
: Sets the expiration timestamp.signWith
: Signs the token using the HS256 algorithm and the provided secret key.
Step 3: Validating a JWT
To validate a JWT, use the following Java code:
import io.jsonwebtoken.Claims;
import io.jsonwebtoken.Jwts;
import io.jsonwebtoken.SignatureException;
public class JwtUtil {
private static final String SECRET_KEY = "mySecretKey";
// Method to validate a JWT and extract claims
public static Claims validateJWT(String jwt) {
try {
// Parse the JWT and validate its signature
return Jwts.parser()
.setSigningKey(SECRET_KEY)
.parseClaimsJws(jwt)
.getBody(); // Return the claims if the JWT is valid
} catch (SignatureException e) {
// Handle invalid JWT signature
System.out.println("Invalid JWT signature");
return null;
}
}
public static void main(String[] args) {
// Example JWT to validate
String jwt = "your.jwt.here";
Claims claims = validateJWT(jwt);
if (claims != null) {
System.out.println("Valid JWT. Subject: " + claims.getSubject());
} else {
System.out.println("Invalid JWT");
}
}
}
Explanation:
parseClaimsJws
: Parses and validates the JWT. If the token is invalid, aSignatureException
is thrown.getBody
: Retrieves the payload (claims) from the JWT if it is valid.
3. Refresh Tokens
Refresh Tokens allow obtaining new access tokens without re-authenticating the user.
Creating Refresh Tokens
Here’s how to create and validate refresh tokens:
import java.util.Date;
import io.jsonwebtoken.Jwts;
import io.jsonwebtoken.SignatureAlgorithm;
public class JwtUtil {
private static final String SECRET_KEY = "mySecretKey";
// Method to create a refresh token
public static String createRefreshToken(String userId) {
long nowMillis = System.currentTimeMillis();
Date now = new Date(nowMillis);
// Refresh tokens are typically valid for a longer period, e.g., 7 days
long refreshTokenValidity = 604800000L; // 7 days
return Jwts.builder()
.setId(userId) // Unique identifier for the user
.setIssuedAt(now) // Timestamp of when the refresh token was issued
.setExpiration(new Date(nowMillis + refreshTokenValidity)) // Expiration time
.signWith(SignatureAlgorithm.HS256, SECRET_KEY) // Sign the refresh token
.compact(); // Serialize the refresh token
}
// Method to validate the refresh token
public static Claims validateRefreshToken(String refreshToken) {
try {
return Jwts.parser()
.setSigningKey(SECRET_KEY)
.parseClaimsJws(refreshToken)
.getBody();
} catch (SignatureException e) {
System.out.println("Invalid Refresh Token");
return null;
}
}
public static void main(String[] args) {
// Generate a refresh token
String refreshToken = createRefreshToken("1");
System.out.println("Generated Refresh Token: " + refreshToken);
// Validate the refresh token
Claims claims = validateRefreshToken(refreshToken);
if (claims != null) {
System.out.println("Valid Refresh Token. User ID: " + claims.getId());
} else {
System.out.println("Invalid Refresh Token");
}
}
}
Explanation:
createRefreshToken
: Generates a refresh token with a longer validity period.validateRefreshToken
: Validates the refresh token and extracts claims if it is valid.
4. Stateless Authentication
In stateless authentication, the server does not keep any state about the user between requests. Each request includes a token that contains all the necessary information.
Example Code for Stateless Authentication
Here’s how you might use JWT for stateless authentication in a Spring Boot application:
import javax.servlet.FilterChain;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import org.springframework.security.authentication.UsernamePasswordAuthenticationToken;
import org.springframework.security.core.context.SecurityContextHolder;
import org.springframework.security.web.authentication.AbstractAuthenticationProcessingFilter;
import io.jsonwebtoken.Claims;
import io.jsonwebtoken.Jwts;
public class JwtAuthorizationFilter extends AbstractAuthenticationProcessingFilter {
private static final String SECRET_KEY = "mySecretKey";
public JwtAuthorizationFilter() {
super("/api/**"); // Filter applies to all requests under /api/
}
@Override
public Authentication attemptAuthentication(HttpServletRequest request, HttpServletResponse response) throws IOException, ServletException {
// This method is not used for JWT, as JWT is handled in doFilterInternal()
return null;
}
@Override
protected void successfulAuthentication(HttpServletRequest request, HttpServletResponse response, FilterChain chain, Authentication authResult) throws IOException, ServletException {
// Continue with the request if the authentication is successful
chain.doFilter(request, response);
}
@Override
protected void doFilterInternal(HttpServletRequest request, HttpServletResponse response, FilterChain chain)
throws IOException, ServletException {
// Get the Authorization header from the request
String header = request.getHeader("Authorization");
// Check if the header contains a Bearer token
if (header != null && header.startsWith("Bearer ")) {
// Extract the token from the header
String token = header.substring(7);
try {
// Parse and validate the token
Claims claims = Jwts.parser()
.setSigningKey(SECRET_KEY)
.parseClaimsJws(token)
.getBody();
// Create an authentication token with the claims
UsernamePasswordAuthenticationToken authentication = new UsernamePasswordAuthenticationToken(
claims.getSubject(), null, new ArrayList<>());
// Set the authentication in the Security Context
SecurityContextHolder.getContext().setAuthentication(authentication);
} catch (Exception e) {
// Handle invalid token
System.out.println("Invalid Token");
}
}
// Continue with the request
chain.doFilter(request, response);
}
}
Explanation:
attemptAuthentication
: Not used in this case, as JWT is validated indoFilterInternal()
.doFilterInternal
: Extracts and validates the JWT from the Authorization header. Sets the authentication context if the token is valid.
OAuth2 and OpenID Connect
OAuth2 and OpenID Connect are essential protocols for authorization and authentication. Let’s break them down:
1. Introduction to OAuth2
OAuth2 is an authorization framework that allows third-party applications to obtain limited access to a user’s resources without sharing their credentials.
Key Components:
Resource Owner: The user who owns the data.
Resource Server: The server hosting the user’s data.
Client: The application requesting access to the user's data.
Authorisation Server: The server that issues access tokens to the client.
Basic OAuth2 Flow:
Authorisation Request: The client requests authorisation from the resource owner.
Authorisation Grant: The resource owner provides an authorisation grant (e.g., authorisation code) to the client.
Access Token Request: The client exchanges the authorisation grant for an access token from the authorisation server.
Access Token: The client uses the access token to access resources from the resource server.
Example: An application wants to access your Google Calendar. OAuth2 allows this without exposing your Google password.
2. OAuth2 Grant Types
OAuth2 defines several grant types, each suited for different scenarios:
Authorisation Code Grant:
Use Case: Web applications and mobile apps.
Flow:
User is redirected to the authorisation server.
User grants permission.
Authorisation server redirects back with an authorization code.
Client exchanges the code for an access token.
Example:
// Redirect the user to the authorization server
String authorizationUrl = "https://authserver.com/authorize?response_type=code&client_id=client_id&redirect_uri=http://yourapp.com/callback";
Implicit Grant:
Use Case: Single-page apps (SPA) where the client-side is trusted.
Flow:
User is redirected to the authorisation server.
User grants permission.
Authorisation server redirects back with an access token.
Example:
// Redirect to the authorization server
window.location.href = "https://authserver.com/authorize?response_type=token&client_id=client_id&redirect_uri=http://yourapp.com/callback";
Resource Owner Password Credentials Grant:
Use Case: Trusted applications where the user’s credentials are known.
Flow:
User provides username and password directly to the client.
Client sends these credentials to the authorisation server to get an access token.
Example:
// Request an access token using user credentials
HttpPost post = new HttpPost("https://authserver.com/token");
List<NameValuePair> params = new ArrayList<>();
params.add(new BasicNameValuePair("grant_type", "password"));
params.add(new BasicNameValuePair("username", "user"));
params.add(new BasicNameValuePair("password", "password"));
post.setEntity(new UrlEncodedFormEntity(params));
Client Credentials Grant:
Use Case: Machine-to-machine communication.
Flow:
Client sends its credentials to the authorization server.
Authorization server returns an access token.
Example:
// Request an access token using client credentials
HttpPost post = new HttpPost("https://authserver.com/token");
List<NameValuePair> params = new ArrayList<>();
params.add(new BasicNameValuePair("grant_type", "client_credentials"));
params.add(new BasicNameValuePair("client_id", "client_id"));
params.add(new BasicNameValuePair("client_secret", "client_secret"));
post.setEntity(new UrlEncodedFormEntity(params));
3. Integrating with OAuth2 Providers (Google, Facebook, etc.)
Integrating with OAuth2 providers involves configuring your application to interact with their authorisation servers. Here's how to set up Google OAuth2 integration with a Spring Boot application.
Ste**p 1: Set Up Google OAuth2**
Go to the Google API Console.
Create a new project.
Configure OAuth2 credentials (client ID and client secret).
Set up redirect URIs (e.g.,
http://localhost:8080/login/oauth2/code/google
).
Step 2: Configure Sp**ring Boot Applicat**ion
Add the required dependency to po``m.xml
:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-oauth2-client</artifactId>
</dependency>
Conf**iguration in** appli``cation.yml
:
spring:
security:
oauth2:
client:
registration:
google:
client-id: your-client-id
client-secret: your-client-secret
scope: profile, email
redirect-uri: "{baseUrl}/login/oauth2/code/{registrationId}"
authorization-grant-type: authorization_code
provider:
google:
authorization-uri: https://accounts.google.com/o/oauth2/auth
token-uri: https://oauth2.googleapis.com/token
user-info-uri: https://www.googleapis.com/oauth2/v3/userinfo
Step 3: Configure Security
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.anyRequest().authenticated() // Require authentication for all requests
.and()
.oauth2Login(); // Enable OAuth2 login
}
}
Step 4: Handle OAuth2 Login
Create a controller to handle user information after login:
import org.springframework.security.core.annotation.AuthenticationPrincipal;
import org.springframework.security.oauth2.core.user.OAuth2User;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class UserController {
@GetMapping("/user")
public String user(@AuthenticationPrincipal OAuth2User principal) {
// Access user information
return "User name: " + principal.getAttribute("name");
}
}
4. OpenID Connect Basics
OpenID Connect (OIDC) is an identity layer on top of OAuth2 that adds authentication. It provides a standardised way to authenticate users and obtain their identity information.
Ke**y Component**s:
ID Token: A JWT that contains identity information about the user.
UserInfo Endpoint: An endpoint where additional user information can be retrieved.
Basic Flow:
Authorisa**tion Request**: The client requests authorisation from the user, including the
openid
scope.Authorisation Response: The authorisation server responds with an authorisation code.
Token Exchange: The client exchanges the authorisation code for an ID token and access token.
ID Token: The client verifies the ID token to authenticate the user.
Example Integration with OpenID Connect (Google):
Step 1: Configure OpenID Connect in applic``ation.yml
:
spring:
security:
oauth2:
client:
registration:
google:
client-id: your-client-id
client-secret: your-client-secret
scope: openid, profile, email
redirect-uri: "{baseUrl}/login/oauth2/code/{registrationId}"
authorization-grant-type: authorization_code
client-authentication-method: basic
provider:
google:
authorization-uri: https://accounts.google.com/o/oauth2/auth
token-uri: https://oauth2.googleapis.com/token
user-info-uri: https://www.googleapis.com/oauth2/v3/userinfo
jwk-set-uri: https://www.googleapis.com/oauth2/v3/certs
Step 2: Handle ID Token
You can access the ID token and user details in your Spring Boot application as shown previously. The oauth2Login()
method in SecurityConfig
handles the OpenID Connect login automatically.
Spring Security and RESTful APIs
1. Securing REST APIs
To secure REST APIs, you need to ensure that unauthorised users cannot access protected resources. This is achieved through proper configuration of Spring Security, including setting up authentication, authorization, and session management.
1.1. Setting Up Spring Security
Add Dependencies: Make sure you have the necessary dependencies in your pom.xml
for Spring Security and JWT:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>io.jsonwebtoken</groupId>
<artifactId>jjwt</artifactId>
<version>0.9.1</version>
</dependency>
1.2. Security Configuration
Create a configuration class SecurityConfig
to set up security settings:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.builders.AuthenticationManagerBuilder;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
import org.springframework.security.config.http.SessionCreationPolicy;
import org.springframework.security.web.authentication.UsernamePasswordAuthenticationFilter;
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.csrf().disable() // Disable CSRF for stateless APIs
.authorizeRequests()
.antMatchers("/public/**").permitAll() // Allow unauthenticated access to public endpoints
.anyRequest().authenticated() // Require authentication for all other endpoints
.and()
.sessionManagement().sessionCreationPolicy(SessionCreationPolicy.STATELESS) // Stateless session management
.and()
.addFilterBefore(new JwtAuthenticationFilter(), UsernamePasswordAuthenticationFilter.class); // Add JWT filter
}
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
// Configure in-memory authentication or other authentication mechanisms if needed
}
}
1.3. Implementing JWT Authentication
Create a JwtAuthenticationFilter
to validate JWT tokens in incoming requests:
import io.jsonwebtoken.Claims;
import io.jsonwebtoken.Jwts;
import org.springframework.security.core.context.SecurityContextHolder;
import org.springframework.security.core.Authentication;
import org.springframework.security.web.authentication.UsernamePasswordAuthenticationFilter;
import javax.servlet.FilterChain;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
public class JwtAuthenticationFilter extends UsernamePasswordAuthenticationFilter {
private final String secretKey = "your-secret-key"; // Secret key for JWT
@Override
protected void doFilterInternal(HttpServletRequest request, HttpServletResponse response, FilterChain chain) throws IOException, ServletException {
String header = request.getHeader("Authorization");
if (header != null && header.startsWith("Bearer ")) {
String token = header.substring(7); // Extract token from the header
try {
Claims claims = Jwts.parser()
.setSigningKey(secretKey)
.parseClaimsJws(token)
.getBody();
// Create authentication object
Authentication auth = new UsernamePasswordAuthenticationToken(claims.getSubject(), null, new ArrayList<>());
SecurityContextHolder.getContext().setAuthentication(auth);
} catch (Exception e) {
// Handle invalid token
System.out.println("Invalid Token");
}
}
chain.doFilter(request, response); // Continue filter chain
}
}
Explanation:
doFilterInternal
: Extracts and validates the JWT token. If valid, sets the authentication in theSecurityContext
.JwtAuthenticationFilter
: Runs beforeUsernamePasswordAuthenticationFilter
to ensure JWT validation.
2. CSRF Protection
2.1. Understanding CSRF: Cross-Site Request Forgery (CSRF) attacks trick a user into performing actions they did not intend. CSRF is a concern primarily for stateful applications where sessions are managed with cookies.
2.2. Disabling CSRF for REST APIs: For REST APIs, you typically use tokens for authentication rather than cookies, making CSRF protection less relevant. You can disable CSRF in your SecurityConfig
:
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.csrf().disable() // Disable CSRF for stateless APIs
// Other security configurations
}
Explanation:
- Disable CSRF: In stateless applications using tokens, disabling CSRF is often appropriate because tokens are sent via headers, not cookies.
2.3. When to Use CSRF: If your application uses cookies for authentication, you should enable CSRF protection to prevent unauthorized actions.
3. CORS Configuration
3.1. Understanding CORS: Cross-Origin Resource Sharing (CORS) is a security feature implemented by browsers to prevent web pages from making requests to a different domain than the one that served the web page.
3.2. Configuring CORS in Spring Boot:
Global CORS Configuration:
Create a configuration class to apply CORS settings globally:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.CorsRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
@Configuration
public class WebConfig implements WebMvcConfigurer {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/**") // Apply CORS to all endpoints
.allowedOrigins("http://allowed-origin.com") // Specify allowed origin(s)
.allowedMethods("GET", "POST", "PUT", "DELETE") // Allowed methods
.allowedHeaders("*"); // Allowed headers
}
}
Controller-Level CORS Configuration:
You can also set CORS settings for specific controllers or endpoints:
import org.springframework.web.bind.annotation.CrossOrigin;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RequestMapping("/api")
public class ApiController {
@CrossOrigin(origins = "http://allowed-origin.com") // Allow CORS for this endpoint
@GetMapping("/data")
public String getData() {
return "Data";
}
}
Explanation:
allowedOrigins
: Specifies which domains can access the resources. Replace"
http://allowed-origin.com
"
with your actual domain.allowedMethods
: Defines which HTTP methods (GET, POST, etc.) are permitted.allowedHeaders
: Lists the headers that are allowed in requests.
Method Security
Method Security in Spring Security allows you to enforce security constraints at the method level. This provides a fine-grained control over who can access which methods, typically used for service or repository layer security. Here’s a detailed look at the key annotations used in method security:
Method Security Annotations
1. @Secured
Annotation
Introduction: The @Secured
annotation provides a way to specify the roles or authorities required to access a particular method. It's a simple way to implement role-based access control.
Usage: To use the @Secured
annotation, you need to enable global method security in your configuration class.
Example:
1.1. Enable Method Security:
In your configuration class, enable method security:
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.method.configuration.EnableGlobalMethodSecurity;
@Configuration
@EnableGlobalMethodSecurity(securedEnabled = true) // Enable @Secured annotation
public class MethodSecurityConfig {
}
1.2. Use @Secured
Annotation:
In your service class, use @Secured
to protect methods:
import org.springframework.security.access.annotation.Secured;
import org.springframework.stereotype.Service;
@Service
public class UserService {
@Secured("ROLE_ADMIN") // Only users with ROLE_ADMIN can access this method
public void deleteUser(Long userId) {
// Delete user logic
}
@Secured({"ROLE_USER", "ROLE_ADMIN"}) // Users with ROLE_USER or ROLE_ADMIN can access
public void updateUser(Long userId) {
// Update user logic
}
}
Explanation:
@Secured("ROLE_ADMIN")
: Only users with theROLE_ADMIN
authority can invoke thedeleteUser
method.@Secured({"ROLE_USER", "ROLE_ADMIN"})
: Users with eitherROLE_USER
orROLE_ADMIN
can invoke theupdateUser
method.
2. @PreAuthorize
and @PostAuthorize
Annotations
Introduction: @PreAuthorize
and @PostAuthorize
provide more advanced security options compared to @Secured
. They allow for method-level security based on expressions that evaluate the authentication or method arguments.
Usage: These annotations allow for dynamic access control based on method parameters, user attributes, and other conditions.
2.1. @PreAuthorize
Annotation:
Example:
2.1.1. Enable Method Security:
Make sure method security is enabled in your configuration class:
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.method.configuration.EnableGlobalMethodSecurity;
@Configuration
@EnableGlobalMethodSecurity(prePostEnabled = true) // Enable @PreAuthorize and @PostAuthorize annotations
public class MethodSecurityConfig {
}
2.1.2. Use @PreAuthorize
Annotation:
In your service class, use @PreAuthorize
for pre-invocation security checks:
import org.springframework.security.access.prepost.PreAuthorize;
import org.springframework.stereotype.Service;
@Service
public class UserService {
@PreAuthorize("hasRole('ROLE_ADMIN')") // Only users with ROLE_ADMIN can access
public void deleteUser(Long userId) {
// Delete user logic
}
@PreAuthorize("#userId == authentication.principal.id or hasRole('ROLE_ADMIN')") // Allow access if userId matches current user or user has ROLE_ADMIN
public void updateUser(Long userId) {
// Update user logic
}
}
Explanation:
@PreAuthorize("hasRole('ROLE_ADMIN')")
: Checks if the user hasROLE_ADMIN
before executing the method.@PreAuthorize("#userId ==
authentication.principal.id
or hasRole('ROLE_ADMIN')")
: Allows access ifuserId
matches the ID of the currently authenticated user or if the user hasROLE_ADMIN
.
2.2. @PostAuthorize
Annotation:
Usage: @PostAuthorize
is used to perform authorization checks after the method execution based on the returned value.
Example:
2.2.1. Use @PostAuthorize
Annotation:
import org.springframework.security.access.prepost.PostAuthorize;
import org.springframework.stereotype.Service;
@Service
public class UserService {
@PostAuthorize("returnObject.userId == authentication.principal.id or hasRole('ROLE_ADMIN')") // Check after method execution
public User getUser(Long userId) {
// Retrieve user logic
return userRepository.findById(userId).orElseThrow(() -> new RuntimeException("User not found"));
}
}
Explanation:
@PostAuthorize("returnObject.userId ==
authentication.principal.id
or hasRole('ROLE_ADMIN')")
: Checks if theuserId
of the returnedUser
object matches the ID of the currently authenticated user or if the user hasROLE_ADMIN
before allowing access to the returned object.
3. Pre and Post Filter Annotations
Introduction: Spring Security provides @PreFilter
and @PostFilter
annotations to filter method arguments and return values based on security constraints.
Usage: These annotations are useful for filtering collections or arrays based on user permissions.
3.1. @PreFilter
Annotation:
Example:
import org.springframework.security.access.prepost.PreFilter;
import org.springframework.stereotype.Service;
@Service
public class UserService {
@PreFilter("filterObject == authentication.principal.id or hasRole('ROLE_ADMIN')") // Filter method arguments
public void updateUsers(List<Long> userIds) {
// Update users logic
}
}
Explanation:
@PreFilter("filterObject ==
authentication.principal.id
or hasRole('ROLE_ADMIN')")
: Filters theuserIds
list before passing it to the method. Only IDs that match the current user's ID or if the user hasROLE_ADMIN
will be included.
3.2. @PostFilter
Annotation:
Example:
import org.springframework.security.access.prepost.PostFilter;
import org.springframework.stereotype.Service;
@Service
public class UserService {
@PostFilter("filterObject.userId == authentication.principal.id or hasRole('ROLE_ADMIN')") // Filter method return value
public List<User> getAllUsers() {
// Retrieve all users
return userRepository.findAll();
}
}
Explanation:
@PostFilter("filterObject.userId ==
authentication.principal.id
or hasRole('ROLE_ADMIN')")
: Filters the list ofUser
objects returned by the method. Only users whoseuserId
matches the current user's ID or if the user hasROLE_ADMIN
will be included in the result.
Password Management
Password management is a critical aspect of application security, ensuring that user credentials are handled securely. It encompasses various practices such as encoding passwords, enforcing password policies, and implementing "Forgot Password" functionality. Here's a detailed explanation of each aspect:
1. Password Encoding
Introduction: Password encoding (or hashing) is the process of transforming a plain-text password into a secure format that is not easily reversible. This is essential to protect passwords from being compromised if a database is breached.
1.1. Password Encoding Strategies
1.1.1. BCrypt
BCrypt is a widely used hashing function that incorporates a salt to prevent rainbow table attacks and allows for adjustable computational cost.
Example:
Add Dependency:
Ensure you have the spring-boot-starter-security
dependency which includes BCrypt:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
Configure Password Encoding:
In your security configuration class, set up a BCryptPasswordEncoder
:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder;
import org.springframework.security.crypto.password.PasswordEncoder;
@Configuration
public class SecurityConfig {
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder(); // Use BCrypt for password encoding
}
}
Usage Example:
Hash a password before saving it to the database and verify it during authentication:
import org.springframework.security.crypto.password.PasswordEncoder;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class UserService {
@Autowired
private PasswordEncoder passwordEncoder;
public void registerUser(String username, String rawPassword) {
String encodedPassword = passwordEncoder.encode(rawPassword); // Encode the password
// Save encodedPassword and username to the database
}
public boolean authenticate(String rawPassword, String encodedPassword) {
return passwordEncoder.matches(rawPassword, encodedPassword); // Verify the password
}
}
Explanation:
passwordEncoder.encode(rawPassword)
: Encodes the plain-text password.passwordEncoder.matches(rawPassword, encodedPassword)
: Compares a raw password with an encoded one.
1.1.2. PBKDF2
PBKDF2 (Password-Based Key Derivation Function 2) is another strong hashing algorithm, also using a salt and allowing for adjustable iterations.
Usage Example:
Similar to BCrypt, you would configure PBKDF2 in Spring Security, though it's less commonly used than BCrypt in modern applications.
2. Password Policies
Introduction: Password policies enforce rules and constraints to ensure passwords are strong and secure. Policies often include requirements for length, complexity, and expiration.
2.1. Implementing Password Policies
2.1.1. Custom Password Validator:
Create a custom validator to enforce password policies:
import org.springframework.security.core.userdetails.UserDetails;
import org.springframework.stereotype.Service;
@Service
public class PasswordValidator {
public boolean validatePassword(String password) {
// Check length
if (password.length() < 8) {
return false;
}
// Check for complexity: at least one uppercase letter, one lowercase letter, one number, and one special character
return password.matches("(?=.*[a-z])(?=.*[A-Z])(?=.*\\d)(?=.*[@$!%*?&])[A-Za-z\\d@$!%*?&]{8,}");
}
}
Explanation:
password.length() < 8
: Checks minimum length requirement.password.matches("regex")
: Uses a regular expression to enforce complexity requirements.
2.1.2. Enforcing Password Expiration:
You can enforce password expiration by storing a last password change date and prompting users to update their password periodically.
Example:
Add a field to store the last password update date:
import java.time.LocalDateTime;
@Entity
public class User {
// Other fields
private LocalDateTime lastPasswordChangeDate;
// Getter and setter for lastPasswordChangeDate
}
Check the password expiration in your authentication logic:
import java.time.LocalDateTime;
import java.time.temporal.ChronoUnit;
@Service
public class UserService {
private static final long PASSWORD_EXPIRATION_DAYS = 90;
public boolean isPasswordExpired(User user) {
return user.getLastPasswordChangeDate().plus(PASSWORD_EXPIRATION_DAYS, ChronoUnit.DAYS).isBefore(LocalDateTime.now());
}
}
Explanation:
lastPasswordChangeDate.plus
(PASSWORD_EXPIRATION_DAYS, ChronoUnit.DAYS)
: Calculates the expiration date.isBefore(
LocalDateTime.now
())
: Checks if the password has expired.
3. Implementing "Forgot Password" Functionality
Introduction: The "Forgot Password" feature allows users to reset their passwords if they forget them. This typically involves sending a password reset link to the user's email.
3.1. Implementation Steps
3.1.1. Generate Password Reset Token:
Generate a secure token when a user requests a password reset:
import java.util.UUID;
import org.springframework.stereotype.Service;
@Service
public class PasswordResetService {
public String generateResetToken() {
return UUID.randomUUID().toString(); // Generate a unique token
}
}
3.1.2. Send Password Reset Email:
Send an email containing the password reset link with the token:
import org.springframework.mail.SimpleMailMessage;
import org.springframework.mail.javamail.JavaMailSender;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class EmailService {
@Autowired
private JavaMailSender mailSender;
public void sendPasswordResetEmail(String email, String resetToken) {
String resetUrl = "http://yourapp.com/reset-password?token=" + resetToken;
SimpleMailMessage message = new SimpleMailMessage();
message.setTo(email);
message.setSubject("Password Reset Request");
message.setText("To reset your password, click the link below:\n" + resetUrl);
mailSender.send(message);
}
}
Explanation:
UUID.randomUUID().toString()
: Creates a unique reset token.mailSender.send(message)
: Sends the email containing the reset link.
3.1.3. Reset Password Endpoint:
Provide an endpoint for users to reset their password using the token:
import org.springframework.web.bind.annotation.*;
import org.springframework.security.crypto.password.PasswordEncoder;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
@Controller
public class PasswordResetController {
@Autowired
private PasswordResetService passwordResetService;
@Autowired
private PasswordEncoder passwordEncoder;
@PostMapping("/reset-password")
public String resetPassword(@RequestParam("token") String token, @RequestParam("newPassword") String newPassword) {
// Validate the token and update the password
String encodedPassword = passwordEncoder.encode(newPassword);
// Update user password logic
return "Password reset successful";
}
}
Explanation:
@RequestParam("token")
: Extracts the reset token from the request.passwordEncoder.encode(newPassword)
: Encodes the new password before updating it.
Session Management
Session management is crucial for maintaining security and a consistent user experience in web applications. It involves handling user sessions, protecting against session fixation attacks, and managing concurrent sessions. Here’s a detailed explanation of these concepts:
1. Managing Sessions
Introduction: Session management involves tracking and maintaining the state of user interactions with a web application. Sessions help to remember user-specific data, such as login status and user preferences.
1.1. Session Creation and Storage
In Spring Security, sessions are managed by the HTTP session mechanism. By default, Spring Security uses HTTP sessions to keep track of authenticated users.
Example:
Configure Session Management:
You can customize session management in your SecurityConfig
class:
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@Configuration
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.permitAll()
.and()
.sessionManagement()
.sessionCreationPolicy(SessionCreationPolicy.IF_REQUIRED); // Session management policy
}
}
Explanation:
SessionCreationPolicy.IF_REQUIRED
: Creates a session if needed, otherwise, it does not create a session.
1.2. Session Attributes
You can store attributes in the session for user-specific data:
import javax.servlet.http.HttpSession;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestParam;
@Controller
public class UserController {
@Autowired
private HttpSession session;
@GetMapping("/setSessionAttribute")
public String setSessionAttribute(@RequestParam("name") String name) {
session.setAttribute("username", name); // Store data in session
return "attributeSet";
}
@GetMapping("/getSessionAttribute")
public String getSessionAttribute() {
String username = (String) session.getAttribute("username"); // Retrieve data from session
return "welcome";
}
}
Explanation:
session.setAttribute("username", name)
: Stores the username in the session.session.getAttribute("username")
: Retrieves the username from the session.
2. Session Fixation Protection
Introduction: Session fixation attacks occur when an attacker sets a user's session ID to a known value, thereby gaining access to the user's session. Spring Security provides protection against these attacks by ensuring that the session ID is changed upon authentication.
2.1. Session Fixation Protection Mechanism
Spring Security automatically protects against session fixation by default. It changes the session ID upon successful authentication.
Example:
Customizing Session Fixation Protection:
You can customize session fixation protection in your SecurityConfig
class:
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
import org.springframework.security.web.authentication.session.SessionAuthenticationStrategy;
import org.springframework.security.web.authentication.session.HttpSessionStrategy;
@Configuration
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.permitAll()
.and()
.sessionManagement()
.sessionFixation().newSession() // Change session ID on authentication
.sessionCreationPolicy(SessionCreationPolicy.IF_REQUIRED);
}
}
Explanation:
.sessionFixation().newSession()
: Creates a new session and invalidates the old one upon authentication, protecting against session fixation attacks.
3. Concurrency Control
Introduction: Concurrency control manages how multiple simultaneous sessions for a single user are handled. This ensures that a user cannot have multiple concurrent sessions that may lead to inconsistent application states.
3.1. Concurrency Control Mechanism
Spring Security provides the ability to control session concurrency by limiting the number of concurrent sessions a user can have.
Example:
Configure Concurrency Control:
Set up concurrency control in your SecurityConfig
class:
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@Configuration
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.permitAll()
.and()
.sessionManagement()
.maximumSessions(1) // Limit to one session per user
.expiredUrl("/login?expired"); // Redirect to login on session expiration
}
}
Explanation:
.maximumSessions(1)
: Limits the number of concurrent sessions to one per user..expiredUrl("/login?expired")
: Redirects the user to the login page with an "expired" parameter if their session expires.
Security Context and Principal
Introduction: The SecurityContext
is a central part of Spring Security that holds the authentication information of the current user. It stores the Authentication
object, which contains details like the principal (the user), credentials, roles, and other details.
1.1. How SecurityContext Works
The SecurityContext
is stored in the SecurityContextHolder
, which is a thread-local variable that ensures the security context is accessible during the lifecycle of a request.
Basic Structure:
SecurityContextHolder: Holds the
SecurityContext
.SecurityContext: Holds the
Authentication
object.Authentication: Contains the
Principal
, credentials, authorities (roles), and other details.
Example:
Accessing the SecurityContext
to retrieve the Authentication
object:
import org.springframework.security.core.Authentication;
import org.springframework.security.core.context.SecurityContextHolder;
public class SecurityService {
public void printCurrentUser() {
// Get the authentication object from the SecurityContext
Authentication authentication = SecurityContextHolder.getContext().getAuthentication();
// Get the principal (user) from the authentication object
Object principal = authentication.getPrincipal();
System.out.println("Current user: " + principal);
}
}
Explanation:
SecurityContextHolder.getContext().getAuthentication()
: Retrieves theAuthentication
object from theSecurityContext
.authentication.getPrincipal()
: Retrieves the currently authenticated user (principal).
2. Working with Principal
Introduction: The Principal
represents the currently authenticated user. In Spring Security, the Principal
is often the user’s username or an instance of UserDetails
.
2.1. Accessing Principal in Controllers
You can easily access the Principal
in your Spring MVC controllers using the Principal
or Authentication
parameters.
Example:
import org.springframework.security.core.Authentication;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import java.security.Principal;
@Controller
public class UserController {
@GetMapping("/user")
public String getUserPage(Principal principal) {
// Access the principal (user)
System.out.println("Logged in user: " + principal.getName());
return "userPage";
}
@GetMapping("/userDetails")
public String getUserDetails(Authentication authentication) {
// Access the authentication object directly
Object principal = authentication.getPrincipal();
System.out.println("Logged in user details: " + principal);
return "userDetailsPage";
}
}
Explanation:
Principal principal
: Injects the principal into the controller method, allowing you to access the username withprincipal.getName()
.Authentication authentication
: Injects the fullAuthentication
object, giving access to the principal, roles, and other details.
3. Custom Security Context
Introduction: In some cases, you may need to customize the SecurityContext
to suit specific application needs. This could involve setting a custom Authentication
object or modifying the SecurityContext
based on specific conditions.
3.1. Custom SecurityContext Implementation
You can create a custom SecurityContext
by extending the existing classes or implementing interfaces provided by Spring Security.
Example:
Suppose you want to add additional information to the SecurityContext
:
import org.springframework.security.core.Authentication;
import org.springframework.security.core.context.SecurityContext;
import org.springframework.security.core.context.SecurityContextHolderStrategy;
public class CustomSecurityContext implements SecurityContext {
private Authentication authentication;
private String customAttribute; // Custom attribute
@Override
public Authentication getAuthentication() {
return this.authentication;
}
@Override
public void setAuthentication(Authentication authentication) {
this.authentication = authentication;
}
public String getCustomAttribute() {
return customAttribute;
}
public void setCustomAttribute(String customAttribute) {
this.customAttribute = customAttribute;
}
}
Explanation:
CustomSecurityContext
: A custom implementation ofSecurityContext
that includes an additional attribute.getCustomAttribute()
: Retrieves the custom attribute added to the context.
3.2. Setting Custom SecurityContext
To set the custom SecurityContext
, you need to configure Spring Security to use it:
import org.springframework.security.core.context.SecurityContextHolder;
import org.springframework.security.core.context.SecurityContextHolderStrategy;
public class CustomSecurityContextHolderStrategy implements SecurityContextHolderStrategy {
private SecurityContext context = new CustomSecurityContext();
@Override
public void clearContext() {
this.context = null;
}
@Override
public SecurityContext getContext() {
return (this.context == null) ? new CustomSecurityContext() : this.context;
}
@Override
public void setContext(SecurityContext context) {
this.context = context;
}
@Override
public SecurityContext createEmptyContext() {
return new CustomSecurityContext();
}
}
Then, you would set this strategy as the default:
SecurityContextHolder.setStrategyName(CustomSecurityContextHolderStrategy.class.getName());
Explanation:
CustomSecurityContextHolderStrategy
: A custom strategy for managing theSecurityContextHolder
.SecurityContextHolder.setStrategyName()
: Sets the custom strategy as the default for the application.
Remember-Me Authentication
Introduction: "Remember-Me" authentication is a feature in web applications that allows users to stay logged in across sessions, even after closing the browser. In Spring Security, this is achieved using cookies that store authentication information.
The "Remember-Me" feature is especially useful for applications where users do not want to log in every time they visit the site. It enhances user experience by maintaining their authentication state over time.
14.1. Configuring Remember-Me
To enable "Remember-Me" authentication in a Spring Boot application, you need to configure it in your SecurityConfig
class. Spring Security offers two types of "Remember-Me" services: Token-Based and Persistent Token-Based. We’ll start with the basic configuration.
1. Basic Remember-Me Configuration:
Here’s how you can configure basic "Remember-Me" functionality using a token-based approach:
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@Configuration
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.permitAll()
.and()
.rememberMe() // Enable Remember-Me authentication
.key("uniqueAndSecret") // A unique key for generating the Remember-Me token
.tokenValiditySeconds(86400); // Set the validity of the Remember-Me cookie (in seconds)
}
}
Explanation:
.rememberMe()
: Enables the "Remember-Me" feature..key("uniqueAndSecret")
: Sets a key used for generating the token. This key must be unique and secure..tokenValiditySeconds(86400)
: Sets the validity period of the "Remember-Me" cookie in seconds (e.g., 86400 seconds equals 1 day).
2. Customizing Remember-Me Services:
You can also customize the Remember-Me service by providing a custom RememberMeServices
bean:
import org.springframework.context.annotation.Bean;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
import org.springframework.security.web.authentication.rememberme.InMemoryTokenRepositoryImpl;
import org.springframework.security.web.authentication.rememberme.PersistentTokenBasedRememberMeServices;
import org.springframework.security.web.authentication.rememberme.PersistentTokenRepository;
@Configuration
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.permitAll()
.and()
.rememberMe()
.rememberMeServices(rememberMeServices());
}
@Bean
public PersistentTokenBasedRememberMeServices rememberMeServices() {
return new PersistentTokenBasedRememberMeServices("uniqueAndSecret", userDetailsService(), tokenRepository());
}
@Bean
public PersistentTokenRepository tokenRepository() {
return new InMemoryTokenRepositoryImpl(); // This stores tokens in memory, not persistent
}
}
Explanation:
PersistentTokenBasedRememberMeServices
: A more advanced "Remember-Me" service that can use persistent tokens.InMemoryTokenRepositoryImpl
: A simple in-memory token repository for storing Remember-Me tokens.
14.2. Persistent Tokens
Introduction: Persistent tokens provide a more secure and reliable way to implement "Remember-Me" functionality by storing tokens in a persistent storage (like a database) instead of just a simple cookie.
1. Persistent Token-Based Remember-Me Services:
When you use persistent tokens, the tokens are stored in a database and can survive application restarts. This makes it harder for attackers to predict or reuse tokens.
Example:
To implement persistent tokens using a database, you need to create a table to store the tokens:
1.1. Creating the Token Table:
CREATE TABLE persistent_logins (
username VARCHAR(64) NOT NULL,
series VARCHAR(64) PRIMARY KEY,
token VARCHAR(64) NOT NULL,
last_used TIMESTAMP NOT NULL
);
Explanation:
username
: The username associated with the token.series
: A unique identifier for the series of tokens issued to the user.token
: The actual token value.last_used
: The timestamp when the token was last used.
1.2. Configuring Persistent Token-Based Remember-Me:
Here’s how to configure the persistent token-based "Remember-Me" in your Spring Security configuration:
import org.springframework.context.annotation.Bean;
import org.springframework.jdbc.core.JdbcTemplate;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
import org.springframework.security.web.authentication.rememberme.JdbcTokenRepositoryImpl;
import org.springframework.security.web.authentication.rememberme.PersistentTokenBasedRememberMeServices;
import org.springframework.security.web.authentication.rememberme.PersistentTokenRepository;
import javax.sql.DataSource;
@Configuration
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.permitAll()
.and()
.rememberMe()
.rememberMeServices(persistentRememberMeServices())
.tokenValiditySeconds(1209600); // 14 days
}
@Bean
public PersistentTokenBasedRememberMeServices persistentRememberMeServices() {
return new PersistentTokenBasedRememberMeServices("uniqueAndSecret", userDetailsService(), tokenRepository());
}
@Bean
public PersistentTokenRepository tokenRepository() {
JdbcTokenRepositoryImpl jdbcTokenRepository = new JdbcTokenRepositoryImpl();
jdbcTokenRepository.setDataSource(dataSource());
return jdbcTokenRepository;
}
@Bean
public DataSource dataSource() {
// Configure and return your DataSource here
return new JdbcTemplate().getDataSource();
}
}
Explanation:
JdbcTokenRepositoryImpl
: This implementation stores tokens in a relational database using JDBC.persistentRememberMeServices()
: Configures the persistent token-based "Remember-Me" service with a customPersistentTokenRepository
.dataSource()
: Provides the database connection for storing tokens.
Exception Handling in Spring Security
Introduction: Exception handling in Spring Security is essential for managing errors that occur during authentication and authorization processes. It ensures that your application responds appropriately to various security-related issues, such as failed login attempts, access denied errors, and more. This includes configuring how these exceptions are handled and presenting custom error pages to the user.
1. Handling Authentication Exceptions
1.1. What Are Authentication Exceptions?
Authentication exceptions occur when there is a failure in the authentication process. Common scenarios include incorrect credentials, expired passwords, or users attempting to access restricted resources without proper authentication.
Spring Security provides a mechanism to handle these exceptions globally, allowing you to customize the response or redirect users to specific pages based on the type of exception.
1.2. Types of Authentication Exceptions
Here are some common authentication exceptions in Spring Security:
BadCredentialsException
: Thrown when the user provides incorrect credentials (e.g., wrong username or password).LockedException
: Thrown when a user account is locked.DisabledException
: Thrown when a user account is disabled.AccountExpiredException
: Thrown when a user account has expired.CredentialsExpiredException
: Thrown when a user’s credentials (e.g., password) have expired.
1.3. Configuring Exception Handling in Spring Security
Spring Security allows you to configure how these exceptions are handled using the exceptionHandling()
method in the HttpSecurity
configuration. You can specify what happens when a user encounters an authentication or authorization error.
Example:
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@Configuration
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.failureUrl("/login?error=true") // Redirect to login page with error on failure
.and()
.exceptionHandling()
.authenticationEntryPoint((request, response, authException) -> {
// Custom logic for handling authentication exceptions
response.sendRedirect("/login?authError=true");
});
}
}
Explanation:
.failureUrl("/login?error=true")
: Redirects the user to the login page with an error message when authentication fails..authenticationEntryPoint()
: Customizes the response when an authentication exception occurs. In this example, it redirects the user to a custom URL with an error parameter.
1.4. Custom Authentication Failure Handler
To have more control over what happens when an authentication exception occurs, you can implement a custom AuthenticationFailureHandler
.
Example:
import org.springframework.security.web.authentication.AuthenticationFailureHandler;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
public class CustomAuthenticationFailureHandler implements AuthenticationFailureHandler {
@Override
public void onAuthenticationFailure(HttpServletRequest request, HttpServletResponse response, AuthenticationException exception) throws IOException, ServletException {
// Custom logic for handling authentication failure
if (exception instanceof BadCredentialsException) {
response.sendRedirect("/login?error=bad_credentials");
} else if (exception instanceof LockedException) {
response.sendRedirect("/login?error=locked");
} else {
response.sendRedirect("/login?error=true");
}
}
}
Explanation:
onAuthenticationFailure()
: Handles the specific type of authentication exception and redirects the user to an appropriate error page.
2. Custom Error Pages
2.1. What Are Custom Error Pages?
Custom error pages are user-friendly pages that display when an error occurs. In Spring Security, you can configure custom pages to show specific messages or actions to users when they encounter authentication or authorization errors.
2.2. Configuring Custom Error Pages
Spring Security allows you to define custom error pages for various exceptions. This is typically done in the HttpSecurity
configuration.
Example:
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@Configuration
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.failureUrl("/login?error=true")
.and()
.exceptionHandling()
.accessDeniedPage("/access-denied"); // Custom access denied page
}
}
Explanation:
.accessDeniedPage("/access-denied")
: Specifies a custom page to be shown when a user encounters an access denied error (i.e., a403 Forbidden
error).
2.3. Custom Access Denied Handler
For more advanced handling of access denied errors, you can implement a custom AccessDeniedHandler
.
Example:
import org.springframework.security.access.AccessDeniedException;
import org.springframework.security.web.access.AccessDeniedHandler;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
public class CustomAccessDeniedHandler implements AccessDeniedHandler {
@Override
public void handle(HttpServletRequest request, HttpServletResponse response, AccessDeniedException accessDeniedException) throws IOException, ServletException {
// Custom logic for handling access denied errors
response.sendRedirect("/access-denied?error=" + accessDeniedException.getMessage());
}
}
Explanation:
handle()
: Handles access denied errors and redirects the user to a custom page with a specific error message.
Best Practices and Advanced Topics
In advanced Spring Security implementations, it's crucial to consider best practices and tackle more complex scenarios, such as securing sensitive endpoints, managing multi-tenancy, and adhering to secure coding practices. Let’s explore these topics in detail.
17.1. Securing Sensitive Endpoints
Introduction: Sensitive endpoints in an application are those that require heightened security because they handle critical operations, such as financial transactions, user management, or access to confidential data. Securing these endpoints ensures that only authorized and authenticated users can access or manipulate these resources.
1.1. Defining Sensitive Endpoints
Sensitive endpoints might include:
Admin consoles: Interfaces where administrators manage users, settings, and configurations.
Financial transactions: Endpoints handling payments, transfers, or any financial operations.
Data access points: APIs that provide access to personal, health, or financial data.
1.2. Securing Sensitive Endpoints in Spring Security
To secure sensitive endpoints, you need to apply stringent access controls, often using roles and permissions.
Example: Securing an admin console.
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@Configuration
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN") // Only users with ADMIN role can access
.antMatchers("/api/transactions/**").hasAuthority("ROLE_FINANCE") // Finance role for transaction-related endpoints
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.permitAll()
.and()
.logout()
.permitAll();
}
}
Explanation:
antMatchers("/admin/**").hasRole("ADMIN")
: Ensures that only users with theADMIN
role can access endpoints under/admin/**
.antMatchers("/api/transactions/**").hasAuthority("ROLE_FINANCE")
: Restricts access to transaction-related endpoints to users with theROLE_FINANCE
authority.
1.3. Adding Multi-Factor Authentication (MFA)
For extremely sensitive endpoints, consider implementing Multi-Factor Authentication (MFA). MFA adds an additional layer of security by requiring users to provide more than one form of verification.
17.2. Multi-Tenancy Security
Introduction: Multi-tenancy refers to an architecture where a single application serves multiple tenants (e.g., different customers, organizations, or groups), each with its own data and configurations. Ensuring security in a multi-tenant environment is challenging but crucial to prevent data breaches and unauthorized access across tenants.
2.1. Approaches to Multi-Tenancy in Spring Security
There are several approaches to implementing multi-tenancy in Spring Security:
Database-per-tenant: Each tenant has its own separate database.
Schema-per-tenant: All tenants share the same database but have separate schemas.
Shared schema with tenant discrimination: All tenants share the same database and schema, with tenant data distinguished by a tenant identifier (e.g., a column in the database).
2.2. Implementing Multi-Tenancy Security
Example: Implementing tenant discrimination in a shared schema.
import org.springframework.security.core.Authentication;
import org.springframework.security.core.context.SecurityContextHolder;
import org.springframework.security.web.authentication.preauth.AbstractPreAuthenticatedProcessingFilter;
import javax.servlet.http.HttpServletRequest;
public class TenantIdentifierFilter extends AbstractPreAuthenticatedProcessingFilter {
@Override
protected Object getPreAuthenticatedPrincipal(HttpServletRequest request) {
// Extract tenant ID from the request (e.g., from a header or query parameter)
return request.getHeader("X-Tenant-ID");
}
@Override
protected Object getPreAuthenticatedCredentials(HttpServletRequest request) {
return "N/A"; // No credentials needed for this example
}
@Override
protected void successfulAuthentication(HttpServletRequest request, HttpServletResponse response, Authentication authResult) {
// Set the tenant ID in the security context
String tenantId = (String) authResult.getPrincipal();
TenantContext.setCurrentTenant(tenantId);
super.successfulAuthentication(request, response, authResult);
}
}
Explanation:
TenantIdentifierFilter
: A filter that extracts the tenant ID from the request and sets it in the security context.TenantContext.setCurrentTenant(tenantId)
: A context holder to store the current tenant ID for use in the application (e.g., for data access filtering).
2.3. Data Isolation
Ensure that each tenant’s data is isolated, either by using separate databases/schemas or by applying tenant-specific filtering in queries. This can be achieved using a combination of Spring Data JPA, Hibernate, and Spring Security.
Example: Implementing data filtering by tenant.
import javax.persistence.EntityManager;
import javax.persistence.PersistenceContext;
import javax.persistence.Query;
import org.hibernate.Session;
public class TenantAwareRepository {
@PersistenceContext
private EntityManager entityManager;
public List<MyEntity> findAllByTenantId(String tenantId) {
Session session = entityManager.unwrap(Session.class);
Query query = session.createQuery("FROM MyEntity WHERE tenantId = :tenantId");
query.setParameter("tenantId", tenantId);
return query.getResultList();
}
}
Explanation:
findAllByTenantId(String tenantId)
: Retrieves data specific to a tenant by filtering based on the tenant ID.
17.3. Secure Coding Practices
Introduction: Secure coding practices are guidelines and best practices that developers should follow to minimize security vulnerabilities in their code. Adhering to secure coding practices is essential to prevent common security issues such as SQL injection, Cross-Site Scripting (XSS), Cross-Site Request Forgery (CSRF), and more.
3.1. Input Validation
Ensure that all user input is validated and sanitized to prevent injection attacks.
Example: Using Spring’s @Validated
annotation for input validation.
import javax.validation.constraints.NotEmpty;
public class UserInput {
@NotEmpty(message = "Username cannot be empty")
private String username;
@NotEmpty(message = "Password cannot be empty")
private String password;
// Getters and setters
}
Explanation:
@NotEmpty
: Ensures that the input fields are not empty, preventing blank inputs.
3.2. Output Encoding
Use output encoding to prevent XSS attacks by ensuring that data displayed in the browser is properly encoded.
Example: Using Thymeleaf for safe HTML rendering.
<p th:text="${userInput.username}">Username</p>
Explanation:
th:text
: Automatically escapes HTML characters to prevent XSS.
3.3. Secure Authentication and Authorization
Implement strong authentication mechanisms (e.g., password hashing, MFA) and ensure proper role-based access control (RBAC).
Example: Using BCrypt for password encoding.
import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder;
public class UserService {
private BCryptPasswordEncoder passwordEncoder = new BCryptPasswordEncoder();
public void saveUser(User user) {
user.setPassword(passwordEncoder.encode(user.getPassword()));
// Save user to the database
}
}
Explanation:
BCryptPasswordEncoder
: Encodes passwords using the BCrypt hashing algorithm, which is resistant to brute-force attacks.
3.4. CSRF Protection
Ensure CSRF protection is enabled to prevent unauthorized actions from being performed on behalf of authenticated users.
Example: CSRF protection in Spring Security.
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@Configuration
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.csrf().csrfTokenRepository(CookieCsrfTokenRepository.withHttpOnlyFalse()) // Enable CSRF protection
.and()
.authorizeRequests()
.anyRequest().authenticated();
}
}
Explanation:
csrfTokenRepository(CookieCsrfTokenRepository.withHttpOnlyFalse())
: Configures CSRF token storage in cookies to protect against CSRF attacks.
3.5. Secure Error Handling
Ensure that error messages do not reveal sensitive information that could be exploited by attackers.
Example: Customizing error responses.
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.http.ResponseEntity;
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(Exception.class)
public ResponseEntity<String> handleException(Exception ex) {
// Log the error
return ResponseEntity.status(500).body("An error occurred. Please try again later.");
}
}
Explanation:
@ControllerAdvice
: Handles exceptions globally, ensuring that detailed error information is not exposed to users.
Spring Security Extensions
Spring Security OAuth2
Spring Security OAuth2 provides support for OAuth2 authorisation and resource servers. It simplifies integration with OAuth2 providers and enables building custom OAuth2 solutions.
Spring Security SAML
Spring Security SAML enables integration with SAML-based single sign-on (SSO) systems, allowing applications to authenticate users with SAML identity providers.
Spring Security Kerberos
Spring Security Kerberos provides support for Kerberos authentication in Spring applications, enabling secure single sign-on using Kerberos tickets.
Conclusion
Summary of Key Concepts
Spring Security provides robust authentication and authorisation mechanisms.
Authentication involves verifying user identity, while Authorisation determines access permissions.
Spring Security supports various authentication methods, including in-memory, JDBC, LDAP, and custom providers.
Advanced features include method security, token-based authentication, and integration with OAuth2 and OpenID Connect.
Subscribe to my newsletter
Read articles from Bikash Nishank directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
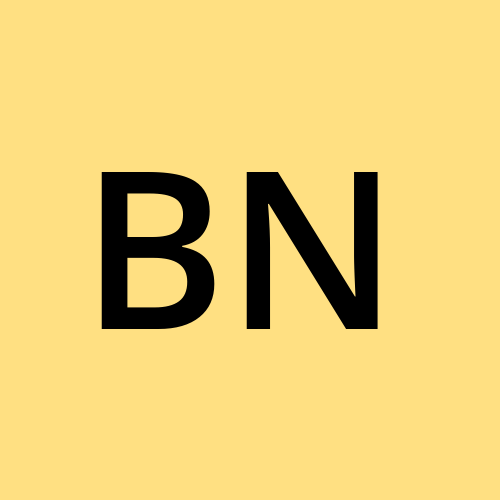