In-Depth Guide to Video Streaming: Monetization, Ad Insertion, DRM, and Dynamic Handling of .m3u8 Files

Table of contents
- Mastering Video Streaming: Unveiling the Secrets Behind Monetization, Ad Insertion, and DRM
- 1. Understanding Monetization in Video Streaming
- Behind the Scenes of the video.js Player Request
- Summary
- 2. Technical Aspects of Ad Insertion
- 3. On-the-Fly Modification of .m3u8 Files
- 4. Digital Rights Management (DRM) and Content Protection
- 5. Conclusion
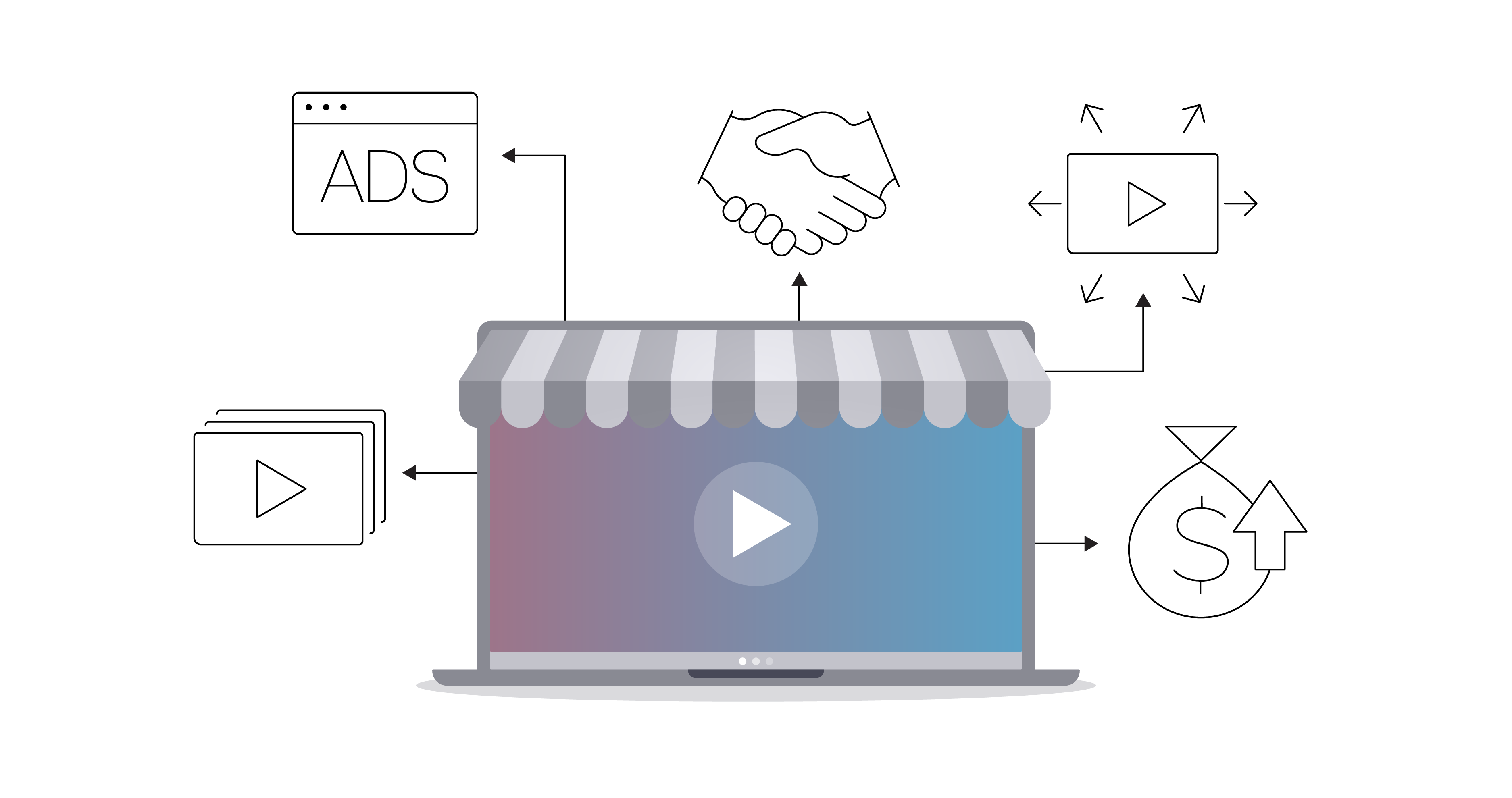
Mastering Video Streaming: Unveiling the Secrets Behind Monetization, Ad Insertion, and DRM
In our journey through the world of video streaming, we've explored many aspects of how content is delivered, processed, and secured. As we approach the final chapters, let's dive deep into some of the most crucial elements of a streaming platform: monetization, ad insertion, and Digital Rights Management (DRM). These are the technologies that ensure the sustainability and protection of streaming services.
But how do these things actually work? How does a platform like YouTube insert ads into video streams, and what really happens behind the scenes when content is protected by DRM? Let's break it all down.
1. Understanding Monetization in Video Streaming
Question: How do streaming platforms generate revenue?
Streaming platforms typically rely on three primary models for monetization:
Advertising: Showing ads during video playback.
Subscriptions: Offering premium content or ad-free experiences for a fee.
Transactional: Charging users per view or for specific content.
Among these, advertising is one of the most technically intricate models. Platforms like YouTube insert ads into video streams, but how do they do this, especially at scale?
When a video player like video.js makes a request to your server for an .m3u8
playlist file, several things happen behind the scenes. Understanding this process will give you insights into how you can modify the .m3u8
file dynamically to insert video ads. Here's a detailed breakdown of what occurs:
Behind the Scenes of the video.js
Player Request
1. Player Initialization
When the HTML page loads, the
<video>
element with thevideo.js
player is initialized.The
video.js
player reads thesource
element'ssrc
attribute to determine the URL of the.m3u8
playlist file.
2. Requesting the .m3u8
File
- The browser makes an HTTP GET request to the server to fetch the
.m3u8
file from the URL provided in thesrc
attribute (http://yourserver.com/yourplaylist.m3u8
).
3. Server Receives the Request
The server receives the request for the
.m3u8
file at the specified endpoint. This is where you can intercept the request and modify the playlist file before sending it back to the client.Example Server Code Flow (e.g., using Node.js, Python, or any backend framework):
Routing: The server routes the request to the appropriate handler function.
Processing: The handler function reads the original
.m3u8
file, processes it (e.g., inserts ad chunk URLs), and prepares the modified playlist.
4. Modifying the .m3u8
File
In the server's handler function, you can perform operations like:
Reading the Original Playlist: Load the original
.m3u8
file from the server or cloud storage.Parsing the Playlist: Split the playlist file into individual lines or entries to identify where you want to insert the ad chunk URLs.
Inserting Ad Chunks: Modify the playlist by inserting URLs for the ad chunks at the desired positions.
Rebuilding the Playlist: Reconstruct the playlist by combining the modified entries into a valid
.m3u8
format.
Example:
app.get('/yourplaylist.m3u8', (req, res) => {
fs.readFile('/path/to/original.m3u8', 'utf8', (err, data) => {
if (err) throw err;
let lines = data.split('\n');
let adChunk = '#EXTINF:10.0,\nhttp://example.com/ad/ad1.ts\n';
lines.splice(4, 0, adChunk);
let modifiedPlaylist = lines.join('\n');
res.setHeader('Content-Type', 'application/vnd.apple.mpegurl');
res.send(modifiedPlaylist);
});
});
5. Sending the Modified .m3u8
File
After processing the
.m3u8
file, the server sends the modified version back to the client as the HTTP response.The content type is set to
application/
vnd.apple
.mpegurl
to indicate that the file is an HLS playlist.
6. Client Receives and Parses the .m3u8
File
The video.js player receives the modified
.m3u8
file from the server.The player parses the playlist to get the URLs of the video chunks and the ad chunks.
The player then requests each chunk in sequence, downloading and playing them as specified in the playlist.
7. Playing the Video and Ads
As the player processes the playlist, it requests each video chunk and ad chunk sequentially.
The player handles the playback, seamlessly integrating the ad chunks with the video content, as specified in the modified
.m3u8
file.
Summary
The sequence of events is as follows:
Request for
.m3u8
: The player makes a request to your server.Server Processing: The server intercepts the request, reads the original
.m3u8
file, modifies it to include ad chunks, and sends it back.Player Execution: The player receives the modified playlist, requests the chunks, and plays them in sequence.
This flow allows you to dynamically inject ads into your HLS streams, providing a customized and monetized viewing experience.
2. Technical Aspects of Ad Insertion
Question: How are ads inserted into video streams? Can we modify the video playlist to add ads dynamically?
Server-Side Ad Insertion (SSAI)
In Server-Side Ad Insertion (SSAI), ads are stitched into the video stream on the server side before being delivered to the user. This method makes the ads nearly impossible to block and ensures a seamless experience.
Here's a high-level overview of how SSAI works:
Content Preparation: The video is transcoded and chunked into segments (e.g., stored on AWS S3).
Ad Decision: An ad decision server (ADS) selects ads based on various criteria like user profile, content type, etc.
Playlist Modification: The
.m3u8
playlist file, which contains the URLs of the video chunks, is modified to include URLs for ad chunks.Content Delivery: The modified playlist is served to the client, and the video player fetches and plays the content, including the ads.
Example: Modifying the .m3u8
Playlist
Imagine you have a video player using HLS and video.js
for playback. The player requests an .m3u8
file that lists the URLs of the video chunks. By modifying this file on the server, you can insert ads into the stream.
Example Code (Node.js):
app.get('/yourplaylist.m3u8', (req, res) => {
fs.readFile('/path/to/original.m3u8', 'utf8', (err, data) => {
if (err) throw err;
let lines = data.split('\n');
let adChunk = '#EXTINF:10.0,\nhttp://example.com/ad/ad1.ts\n';
lines.splice(4, 0, adChunk); // Insert ad after the first chunk
let modifiedPlaylist = lines.join('\n');
res.setHeader('Content-Type', 'application/vnd.apple.mpegurl');
res.send(modifiedPlaylist);
});
});
Handling Dynamic Ad Insertion for Advertisers
Question: How can we integrate ads provided by an advertiser into our video stream?
Let’s say an advertiser comes to you with a request to run ads for a particular video. Here's how you can achieve that:
Ad Selection: First, based on the advertiser’s request, you identify the ad to be inserted. This could be based on criteria like the user’s location, the content type, or the ad campaign’s target audience.
Fetch the Advertiser's
.m3u8
File: The ad itself may be provided in the form of its own.m3u8
playlist file, containing URLs to the ad chunks.Modify the Original Playlist: When the original video playlist is requested, the server fetches this file from the database (or another source), modifies it to include the ad chunks from the advertiser's
.m3u8
file, and then sends the updated playlist to the client.
Example Code (Node.js):
app.get('/yourplaylist.m3u8', async (req, res) => {
// Fetch the original playlist
let originalPlaylist = await fetchOriginalPlaylistFromDB(req.params.id);
// Fetch the advertiser's ad playlist
let adPlaylist = await fetchAdPlaylist(req.query.adId); // Assume adId is passed as a query parameter
// Merge the ad chunks into the original playlist
let mergedPlaylist = mergePlaylists(originalPlaylist, adPlaylist);
res.setHeader('Content-Type', 'application/vnd.apple.mpegurl');
res.send(mergedPlaylist);
});
function mergePlaylists(videoPlaylist, adPlaylist) {
let videoLines = videoPlaylist.split('\n');
let adLines = adPlaylist.split('\n');
// Insert ad after the first chunk of video (adjust based on logic)
videoLines.splice(4, 0, ...adLines);
return videoLines.join('\n');
}
This dynamic insertion allows the platform to tailor ads based on real-time decisions, ensuring that the right ad reaches the right user.
Scenario: Inserting Ads in video.js
When the modified playlist is sent to the client, video.js
will play the ad chunk as if it were part of the original content. This seamless integration is what makes SSAI so effective.
3. On-the-Fly Modification of .m3u8
Files
Question: What happens when a video player requests an .m3u8
file? Can it be modified dynamically?
When the video player requests an .m3u8
file, the server can dynamically modify this file to include additional chunks (such as ads). This dynamic handling of the .m3u8
file allows for real-time personalization and ad insertion.
Example of Dynamic Handling:
app.get('/playlist/:id.m3u8', async (req, res) => {
const playlist = await getPlaylistFromDatabase(req.params.id); // Fetch playlist from DB
let modifiedPlaylist = insertAdsIntoPlaylist(playlist); // Insert ads or other modifications
res.setHeader('Content-Type', 'application/vnd.apple.mpegurl');
res.send(modifiedPlaylist);
});
In this approach, the server processes the .m3u8
request on-the-fly, ensuring that the playlist sent to the client is tailored to the user's context, which could include personalized ads or other content.
4. Digital Rights Management (DRM) and Content Protection
Question: How does DRM protect video content? What happens behind the scenes when DRM is applied?
DRM is a critical technology that prevents unauthorized access to video content. It ensures that only authorized users can view the content by encrypting the video chunks and requiring a decryption key that only the authorized player can obtain.
Key DRM Processes:
Encryption: Video chunks are encrypted using a secure encryption key. This ensures that even if someone downloads the chunks, they cannot view the content without the decryption key.
License Server: A license server is responsible for managing the decryption keys. When a user attempts to play a video, the video player sends a request to the license server to obtain the key. The server verifies the user's credentials and, if authorized, provides the decryption key.
Playback: The authorized video player uses the decryption key to decrypt the video chunks on-the-fly as they are being played. This ensures that the content remains protected throughout the streaming process.
Example: DRM with HLS
HLS supports DRM by allowing video chunks to be encrypted, with the decryption key provided via a secure URL in the .m3u8
playlist.
Example of Encrypted Playlist:
#EXTM3U
#EXT-X-VERSION:3
#EXT-X-TARGETDURATION:10
#EXT-X-KEY:METHOD=AES-128,URI="https://example.com/decrypt-key",IV=0x1234567890abcdef
#EXTINF:10.0,
http://example.com/encrypted_chunk1.ts
#EXTINF:10.0,
http://example.com/encrypted_chunk2.ts
Question: What happens if someone downloads encrypted chunks without the decryption key?
Without the decryption key, the downloaded chunks are useless. The encryption ensures that only authorized players, which have access to the key, can decrypt and play the video.
5. Conclusion
As we conclude this series, we’ve covered an extensive array of topics, from the basics of video streaming to the complexities of monetization, ad insertion, and DRM. Each of these components plays a vital role in delivering high-quality, secure, and profitable content to viewers across the globe.
By understanding these concepts, you're well on your way to mastering the intricate world of video streaming. Whether you're building your own platform or optimizing an existing one, these insights will serve as a solid foundation for your endeavors.
This version includes the necessary discussions on the on-the-fly modification of .m3u8
files, DRM processes, and how these technologies work together in a real-world context. The article is structured to provide a clear and comprehensive understanding, addressing the technical
Subscribe to my newsletter
Read articles from ritiksharmaaa directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

ritiksharmaaa
ritiksharmaaa
Hy this is me Ritik sharma . i am software developer