Writing Your First Code-A Simple Project to Kickstart Your Learning
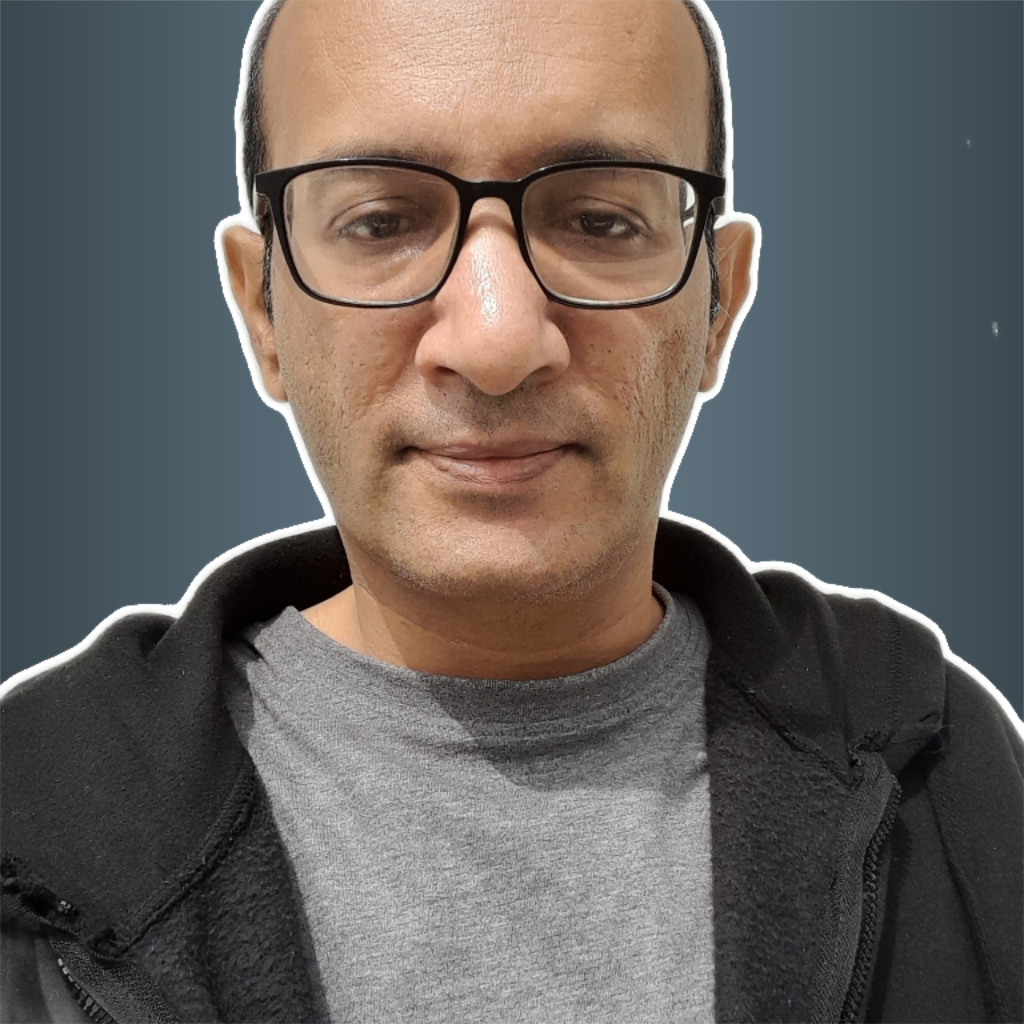
Table of contents
- Why Start with a Simple Project?
- Choosing Your First Project: A Basic Calculator
- Step 1: Setting Up Your Development Environment
- Step 2: Writing Your First Lines of Code
- Step 3: Taking User Input
- Step 4: Performing the Calculation
- Step 5: Running Your Code
- Expanding Your Project
- Conclusion: The Power of Starting Small
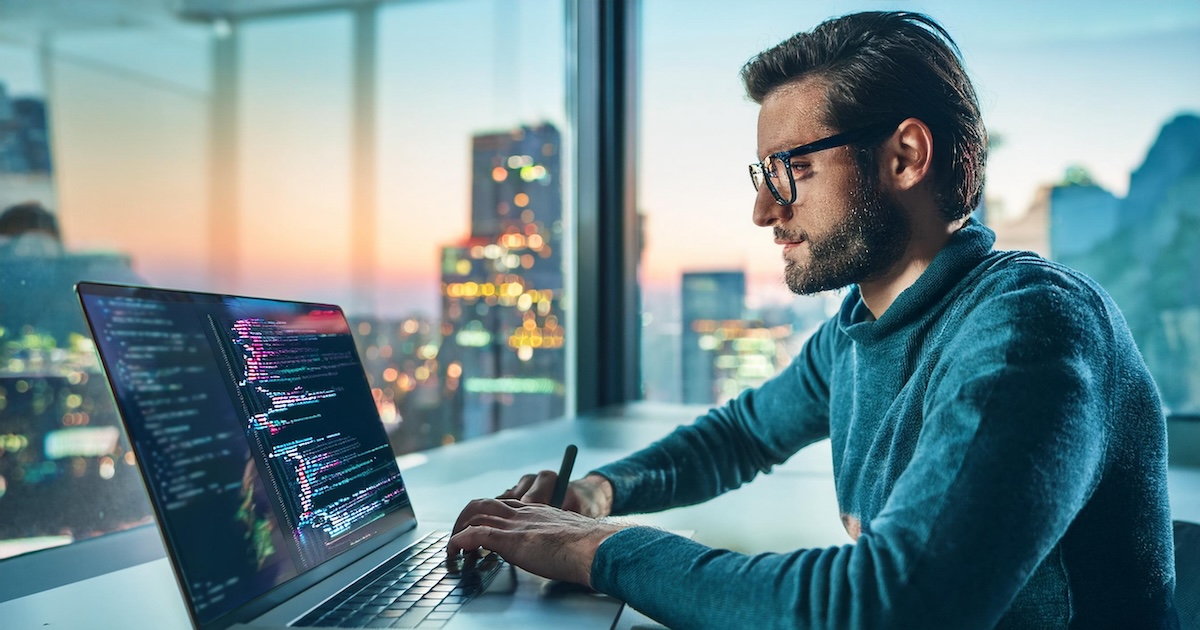
Embarking on your coding journey is both thrilling and challenging. Whether you're aiming to become a software engineer, a web developer, or simply want to learn how to code, the best way to start is by diving right in and writing your first code. In this post, we’ll guide you through a simple project that will help you kickstart your learning, giving you a hands-on experience that lays a solid foundation for your future coding endeavors.
Why Start with a Simple Project?
Starting with a simple project offers several advantages:
Hands-On Learning: Writing code from scratch helps you understand programming concepts better than just reading about them.
Immediate Feedback: You can see the results of your code instantly, allowing you to learn through trial and error.
Build Confidence: Completing a project, no matter how small, boosts your confidence and motivates you to tackle more complex challenges.
Foundation for Growth: A simple project provides a base from which you can expand your skills, adding complexity and new features as you learn more.
Choosing Your First Project: A Basic Calculator
One of the best beginner projects is creating a basic calculator. It’s simple enough for a first project but covers essential programming concepts like variables, functions, user input, and conditional statements. Plus, a calculator is something you can immediately use and test.
Step 1: Setting Up Your Development Environment
Before writing any code, you need to set up your development environment. Here’s what you’ll need:
A Code Editor: We recommend using Visual Studio Code (VS Code) because it’s free, beginner-friendly, and packed with useful features.
A Programming Language: For this project, we’ll use Python, a popular language known for its readability and ease of use.
Installing Python:
Download and install Python from the official website (python.org).
Ensure Python is added to your system’s PATH during installation, so you can run Python scripts from your command line.
Step 2: Writing Your First Lines of Code
With your environment ready, it’s time to write some code! Open your code editor and create a new file called calculator.py
. This will be the script where you write your calculator program.
Starting Your Script:
# Simple Calculator in Python
def add(x, y):
return x + y
def subtract(x, y):
return x - y
def multiply(x, y):
return x * y
def divide(x, y):
if y != 0:
return x / y
else:
return "Error: Division by zero is not allowed."
In this code, we’ve defined four basic arithmetic functions: add
, subtract
, multiply
, and divide
. Each function takes two arguments (x
and y
) and returns the result of the respective operation.
Step 3: Taking User Input
Next, let’s allow users to input numbers and choose an operation. This is where you’ll use Python’s built-in input()
function.
Adding User Input:
print("Select operation:")
print("1. Add")
print("2. Subtract")
print("3. Multiply")
print("4. Divide")
choice = input("Enter choice(1/2/3/4): ")
num1 = float(input("Enter first number: "))
num2 = float(input("Enter second number: "))
Here, the user can choose the operation they want to perform and input the numbers they want to calculate.
Step 4: Performing the Calculation
Finally, we’ll use conditional statements to perform the chosen operation and display the result.
Finalizing the Calculator:
if choice == '1':
print(f"The result is: {add(num1, num2)}")
elif choice == '2':
print(f"The result is: {subtract(num1, num2)}")
elif choice == '3':
print(f"The result is: {multiply(num1, num2)}")
elif choice == '4':
print(f"The result is: {divide(num1, num2)}")
else:
print("Invalid input")
This code checks the user’s choice and calls the corresponding function to perform the calculation. The result is then printed out.
Step 5: Running Your Code
Save your file and run the script to see your calculator in action. You can run the script from the terminal by navigating to the folder where your calculator.py
file is located and typing:
python calculator.py
Follow the on-screen prompts to select an operation and input numbers. Your calculator should output the correct result.
Expanding Your Project
Once you’ve successfully created your basic calculator, you can expand the project to add more features:
Add more operations: Include operations like exponentiation, square root, or modulus.
Improve the user interface: Create a graphical user interface (GUI) using libraries like Tkinter.
Error handling: Enhance the script to handle invalid inputs or unexpected errors more gracefully.
Conclusion: The Power of Starting Small
Writing your first code with a simple project like a calculator is a great way to start your coding journey. It allows you to apply basic programming concepts in a practical way, giving you the confidence and skills needed to take on more complex projects. Remember, every coding journey begins with a single line of code—so don’t hesitate to dive in and start building!
By following this guide, you’ve not only learned how to write your first code but also laid the groundwork for future success in programming. Keep experimenting, keep learning, and most importantly, keep coding!
Subscribe to my newsletter
Read articles from Azhar Hussain directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
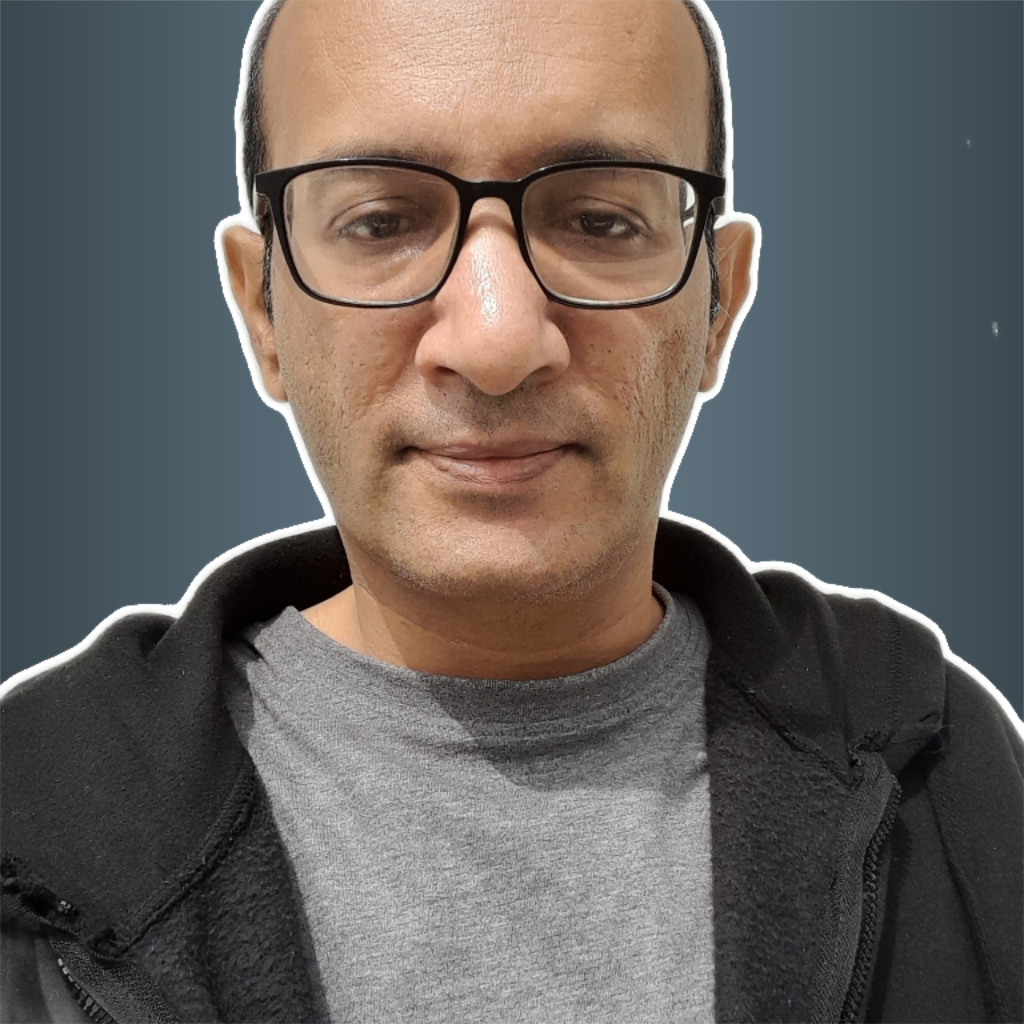
Azhar Hussain
Azhar Hussain
Over 2 decades of software engineering experience, including over a decade in building, scaling and leading engineering teams.