NestJS Made Easy: Essential Techniques for Developers

Table of contents
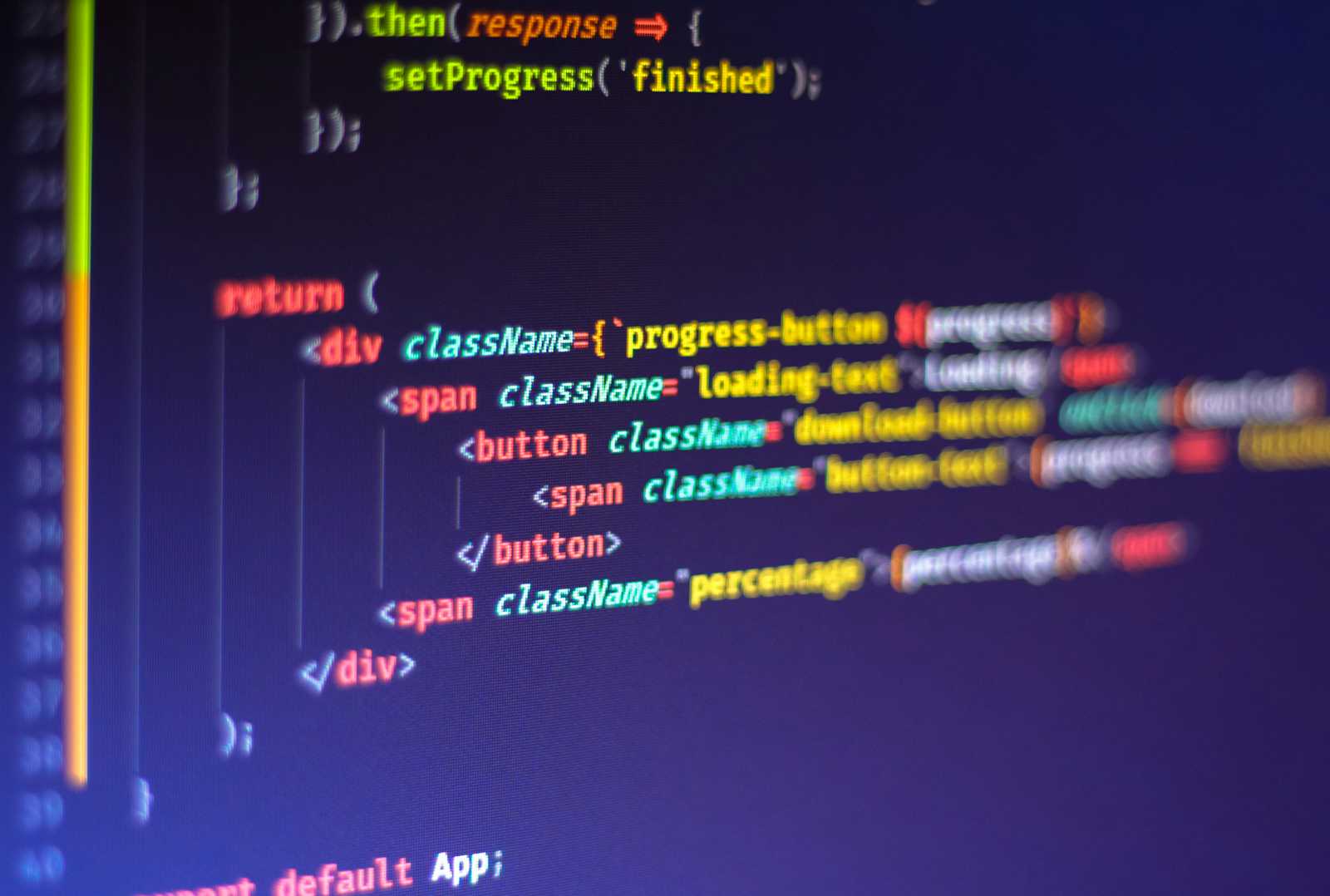
Introduction
I was working on a project comprising of Nestjs, typescript e.t.c, and I needed to extract data from a PDF document, and then the research started. I searched the npm platform for different packages, there I saw multiple packages that would seemingly work, but as I tested some of them errors were popping up, from types not being defined in the package to the package lacking constructive props(there about). Then I came across a certain package ‘pdf-parse’ and I decided to try it, and to my amazement it worked. So here, I’m going to give you a breakdown of the code and some results.
Code breakdown I guess you would already have a project ready, but if not here’s some snippets. For a regular
nodejs project:
npm init -y
For a Nestjs project:
nest new project-name
Then you install the following packages
npm i multer @types/multer pdf-parse
You’re supposed to have a module to perform the extraction, and within that a controller file to handle and direct requests, and a service file to hold the logic of said module.
In the service file :
import { Injectable, Logger } from '@nestjs/common';
import * as pdfParse from 'pdf-parse';
@Injectable()
export class PDFExtractService {
private readonly logger = new Logger(
PDFExtractService.name
);
async extractTextFromPdf(pdfBuffer: Buffer): Promise {
try { const data = await pdfParse(pdfBuffer);
return data.text; }
catch (error) {
this.logger.error('Error extracting text from PDF', error);
throw new Error('Error extracting text from PDF');
} } }
We import pdfparse from the pdfparse package we installed; Our function takes in pdfbuffer as a parameter, this is how we’ll pass the uploaded PDF from the controller. And then we return the text of the parsed pdf, we could return the entire object by using
return data.text;
And then if there’s an error we have the logger to display our caught errors.
In the controller file:
import { Controller, Post, UploadedFile, UseInterceptors, } from '@nestjs/common';
import { FileInterceptor } from '@nestjs/platform-express';
import { PDFExtractService } from './pdf-extract.service';
@Controller('pdf-extract')
export class PdfExtractController {
constructor(private readonly pdfExtractService: PDFExtractService) {}
@Post('extract-text') @UseInterceptors(FileInterceptor('file'))
async extractText(@UploadedFile() file: Express.Multer.File) {
const pdfBuffer = file.buffer; const text = await this.pdfExtractService.extractTextFromPdf(pdfBuffer);
return text; // return extracted text as a response } }
For those who aren’t used to this syntax, it’s nest js and you can read the documentation to get familiar with it if you find it’s use case. As soon as we call our API at route: “pdf-extract/extract-text” using the Post http method. We attach our pdf file while calling this route, it takes in the file using multer, and then passes the file buffer as a parameter to the service function, which in turn parses the buffer and return an object.
The image above is the result of implementing pdf-parser into my project.
As time goes on I’ll keep updating the blog on similar topics, code-based, theory-based and so on.
Subscribe to my newsletter
Read articles from Manuel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Manuel
Manuel
💻 Self-taught Fullstack Developer | 🚀 Passionate about building web solutions with NestJS | 💡 Critical thinker with a love for philosophy and technology |