Testing Node.js Applications

Table of contents
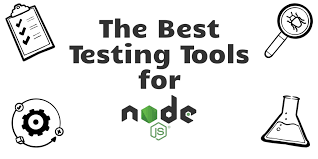
Welcome to Our Day 19 of our Node.js Zero to 1! Blog Series ๐๐
Testing is a critical part of the software development lifecycle, ensuring that your application functions as expected and helps catch bugs before they reach production. In this session, weโll explore testing frameworks for Node.js, writing unit tests, testing asynchronous code, and setting up continuous integration for automated testing.
Introduction to Testing Frameworks
Several testing frameworks are popular in the Node.js ecosystem. We'll look at Mocha, Chai, and Jest, which are among the most widely used.
Mocha
Mocha is a flexible and feature-rich JavaScript test framework running on Node.js and in the browser, making asynchronous testing simple. It provides a variety of interfaces for writing tests, making it versatile for different coding styles.
Installing Mocha:
npm install --save-dev mocha
Basic Mocha Test:
Create a test file, e.g., test.js
:
const assert = require('assert');
describe('Array', () => {
describe('#indexOf()', () => {
it('should return -1 when the value is not present', () => {
assert.strictEqual([1, 2, 3].indexOf(4), -1);
});
});
});
Run the test with:
npx mocha test.js
Chai
Chai is an assertion library often used with Mocha. It provides a variety of assertion styles, including BDD (Behavior-Driven Development) and TDD (Test-Driven Development).
Installing Chai:
npm install --save-dev chai
Using Chai with Mocha:
const { expect } = require('chai');
describe('Array', () => {
describe('#indexOf()', () => {
it('should return -1 when the value is not present', () => {
expect([1, 2, 3].indexOf(4)).to.equal(-1);
});
});
});
Jest
Jest is a comprehensive testing framework developed by Facebook. It includes features like snapshot testing, a powerful mocking library, and a test runner with a built-in code coverage tool.
Installing Jest:
npm install --save-dev jest
Basic Jest Test:
Create a test file, e.g., sum.test.js
:
const sum = (a, b) => a + b;
test('adds 1 + 2 to equal 3', () => {
expect(sum(1, 2)).toBe(3);
});
Run the test with:
npx jest
Writing Unit Tests for Node.js Applications
Unit tests focus on testing individual units of code, such as functions or methods, in isolation from the rest of the application.
Example: Unit Testing a Function
Suppose you have a simple function in math.js
:
// math.js
function add(a, b) {
return a + b;
}
module.exports = add;
Writing a Unit Test:
Using Mocha and Chai:
// test/math.test.js
const { expect } = require('chai');
const add = require('../math');
describe('add', () => {
it('should add two numbers correctly', () => {
expect(add(1, 2)).to.equal(3);
});
it('should return a number', () => {
expect(add(1, 2)).to.be.a('number');
});
});
Testing Asynchronous Code
Asynchronous code is common in Node.js applications, and testing it requires special handling to ensure that tests wait for asynchronous operations to complete.
Example: Testing Asynchronous Functions
Suppose you have an asynchronous function in user.js
:
// user.js
const fetchUser = (id) => {
return new Promise((resolve) => {
setTimeout(() => {
resolve({ id, name: 'John Doe' });
}, 100);
});
};
module.exports = fetchUser;
Writing a Test for Asynchronous Code:
Using Mocha and Chai:
// test/user.test.js
const { expect } = require('chai');
const fetchUser = require('../user');
describe('fetchUser', () => {
it('should fetch a user by ID', async () => {
const user = await fetchUser(1);
expect(user).to.deep.equal({ id: 1, name: 'John Doe' });
});
});
Using Jest:
// user.test.js
const fetchUser = require('../user');
test('fetches a user by ID', async () => {
const user = await fetchUser(1);
expect(user).toEqual({ id: 1, name: 'John Doe' });
});
Setting Up Continuous Integration for Automated Testing
Continuous Integration (CI) ensures that your tests are run automatically whenever changes are made to your codebase. Popular CI services include GitHub Actions, Travis CI, and CircleCI.
Example: Setting Up GitHub Actions
- Create a GitHub Actions Workflow:
Create a .github/workflows/ci.yml
file in your repository:
name: Node.js CI
on:
push:
branches: [main]
pull_request:
branches: [main]
jobs:
build:
runs-on: ubuntu-latest
strategy:
matrix:
node-version: [14, 16]
steps:
- uses: actions/checkout@v2
- name: Use Node.js ${{ matrix.node-version }}
uses: actions/setup-node@v2
with:
node-version: ${{ matrix.node-version }}
- run: npm install
- run: npm test
Explanation:
The workflow runs on pushes and pull requests to the
main
branch.It tests against multiple Node.js versions (14 and 16).
The
actions/checkout
action checks out your repository.The
actions/setup-node
action sets up the specified Node.js versions.The workflow installs dependencies and runs the tests.
Example: Setting Up Travis CI
- Create a
.travis.yml
File:
language: node_js
node_js:
- "14"
- "16"
script:
- npm install
- npm test
Explanation:
The
.travis.yml
file specifies the Node.js versions to test against.The
script
section installs dependencies and runs the tests.
Best Practices for Testing Node.js Applications
Write Isolated Tests: Each test should be independent and not rely on the state left by other tests.
Use Mocks and Stubs: Mock external dependencies and services to test your code in isolation. Libraries like Sinon.js are useful for creating mocks and stubs.
Test Coverage: Aim for high test coverage to ensure that most of your codebase is tested. Use tools like Istanbul (integrated with Jest) to measure test coverage.
Automate Testing: Use CI/CD pipelines to run tests automatically on every commit and pull request. This ensures that issues are caught early.
Handle Edge Cases: Write tests for edge cases and invalid inputs to ensure your application handles them gracefully.
Performance Testing: For critical parts of your application, consider performance testing to ensure they meet the required performance criteria.
Keep Tests Up-to-Date: Regularly update tests to reflect changes in the codebase. Outdated tests can give a false sense of security.
Conclusion
Testing is an essential part of developing reliable and maintainable Node.js applications. By leveraging testing frameworks like Mocha, Chai, and Jest, you can write robust unit tests, handle asynchronous code, and set up continuous integration for automated testing. Following best practices for testing ensures that your application remains stable and performs well under various conditions.
In the next post, we'll explore Working with Streams in Node.js . Stay tuned for more insights!
Subscribe to my newsletter
Read articles from Anuj Kumar Upadhyay directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Anuj Kumar Upadhyay
Anuj Kumar Upadhyay
I am a developer from India. I am passionate to contribute to the tech community through my writing. Currently i am in my Graduation in Computer Application.