Make Your Python Code Faster with Dictionary Lookups
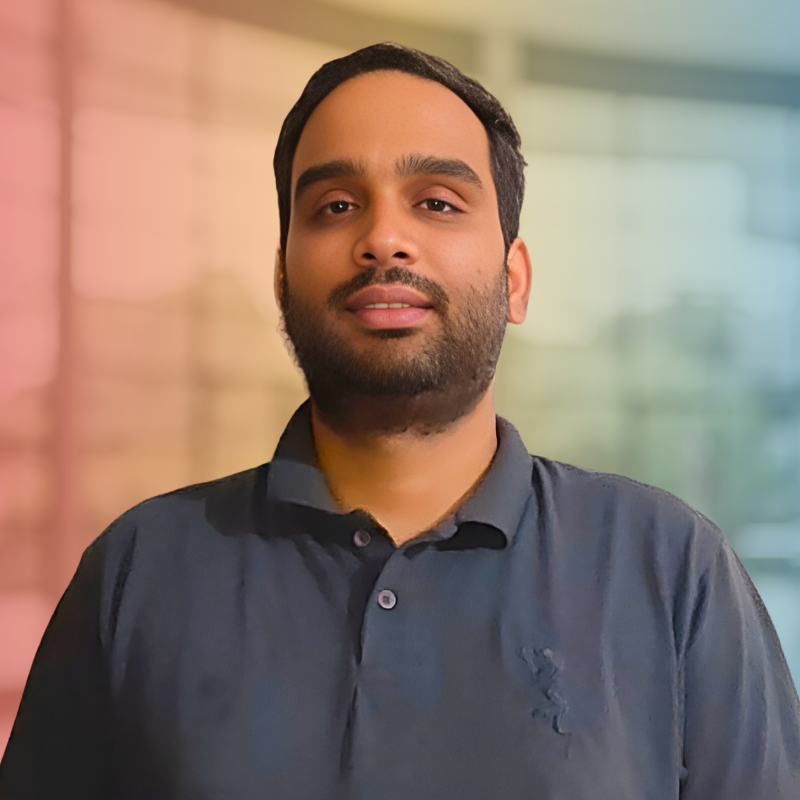
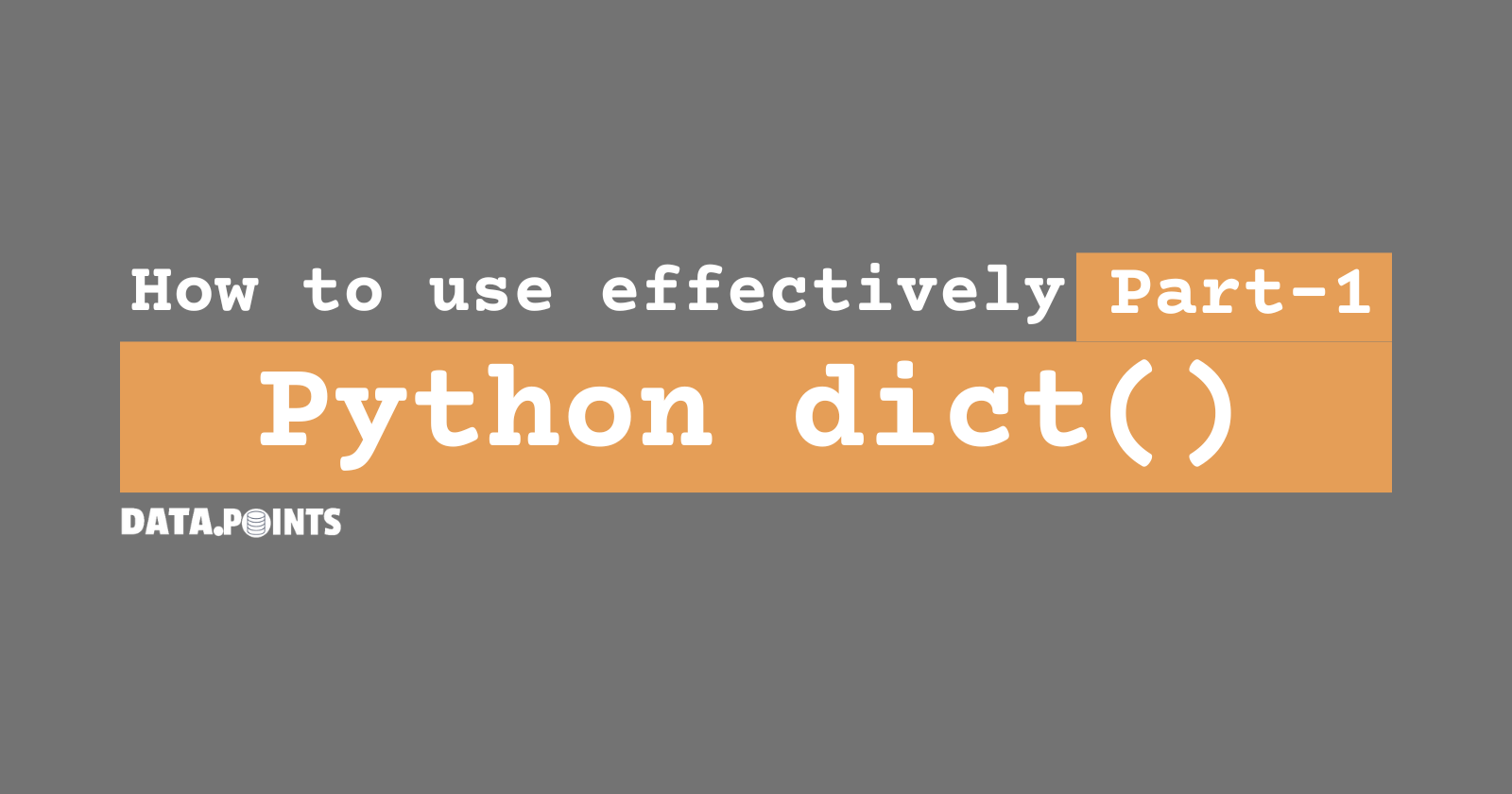
In the world of programming, efficiency is key. Whether you're a beginner or a seasoned developer, finding ways to optimize your code can make a significant difference, especially when working with large datasets. One powerful tool in Python that often goes underutilized is the dictionary lookup. In this post, we'll explore how dictionary lookups work, why they're so efficient, and how you can leverage them to enhance your Python programs.
What is a Dictionary Lookup?
In Python, a dictionary is a collection of key-value pairs, where each key is unique, and each key maps to a specific value. This data structure is incredibly versatile and allows for fast access to data. A dictionary lookup refers to the process of retrieving a value associated with a specific key in a dictionary.
Here is an example:
# Creating a dictionary
fruit_colors = {
"apple": "red",
"banana": "yellow",
"cherry": "red",
"orange": "orange"
}
# Performing a dictionary lookup
color_of_apple = fruit_colors["apple"]
print(color_of_apple) # Output: red
In this example, the key "apple"
is used to quickly access the value "red"
. This operation is extremely fast, and that speed is one of the primary reasons why dictionary lookups are so valuable.
Why Are Dictionary Lookups So Fast?
The speed of dictionary lookups is primarily due to the underlying data structure: hash tables. When you create a dictionary in Python, each key is hashed using a hash function, which generates a unique identifier for that key. This hash is then used to determine where the corresponding value is stored in memory.
Because the hash function allows for direct access to the location of the value, retrieving a value from a dictionary typically takes O(1) time, meaning it’s constant time, regardless of the size of the dictionary. This is in stark contrast to other data structures, such as lists, where searching for a value can take O(n) time (linear time) because you might need to check each element.
When Should You Use Dictionary Lookups?
Dictionary lookups are particularly useful in situations where you need to frequently access data based on a unique identifier. Here are a few scenarios where dictionary lookups can greatly enhance your code:
- Data Mapping: When you have a set of unique keys that map to specific values, such as user IDs mapping to user information.
user_data = {
"user123": {"name": "Alice", "age": 30},
"user456": {"name": "Bob", "age": 25},
# more users...
}
Caching Results: If you’re performing an expensive computation or accessing a slow resource (like a database or API), you can store the results in a dictionary and reuse them to avoid repeated operations.
expensive_computation_cache = {} def expensive_computation(x): if x in expensive_computation_cache: return expensive_computation_cache[x] # Perform the computation result = x * x expensive_computation_cache[x] = result return result
Fast Membership Testing: If you need to frequently check if an item exists in a collection, using a dictionary (or a set, which also uses hashing) allows for O(1) membership tests.
blacklisted_emails = {"spam@example.com", "junk@example.com"} if email in blacklisted_emails: print("This email is blacklisted.")
Building Lookup Tables from Lists
In some cases, you might start with a list of dictionaries or tuples and need to frequently search for items based on a specific attribute. Instead of iterating through the list every time, you can convert it into a dictionary (often referred to as a lookup table) to speed up your searches.
For example, let's say you have a list of products and you want to quickly find a product by its ID:
products = [
{"id": 101, "name": "Laptop", "price": 799},
{"id": 102, "name": "Tablet", "price": 499},
{"id": 103, "name": "Smartphone", "price": 699},
]
# Build a lookup table for products by ID
product_lookup = {product["id"]: product for product in products}
# Now you can quickly find a product by its ID
product = product_lookup.get(102)
print(product) # Output: {'id': 102, 'name': 'Tablet', 'price': 499}
By transforming your list into a dictionary, you transform your search operation from O(n) to O(1), greatly improving efficiency.
Pitfalls to Watch Out For
While dictionary lookups are powerful, there are a few things to be aware of:
Memory Usage: Dictionaries are fast, but they can use more memory than lists due to the overhead of storing hash tables. If memory is a constraint, consider this trade-off.
Mutable Keys: In Python, dictionary keys must be immutable (e.g., strings, numbers, tuples). If you try to use a mutable type (like a list) as a key, you’ll encounter an error.
Collisions: Although rare, hash collisions can occur, where two different keys produce the same hash value. Python handles this internally, but it’s something to be aware of if you’re working with a large set of keys.
Conclusion
Dictionary lookups are a fundamental tool in Python programming that offers significant performance benefits. By understanding how they work and when to use them, you can write more efficient, scalable, and maintainable code. Whether you're dealing with large datasets, building a cache, or simply trying to speed up your searches, dictionary lookups should be one of your go-to techniques.
So, next time you're faced with a problem that involves frequent data retrieval, consider reaching for a dictionary—you'll be amazed at how much faster your code can run!
#Python #Programming #DataScience #CodingEfficiency #TechTips #Optimization
Subscribe to my newsletter
Read articles from Shantanu Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
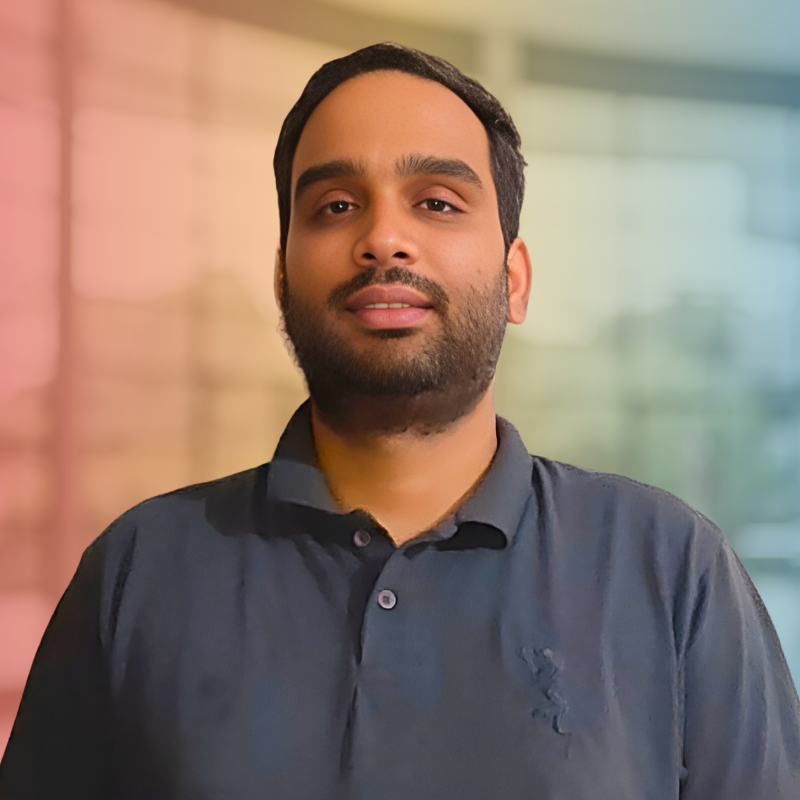
Shantanu Sharma
Shantanu Sharma
I am Data Scientist from India, working as Associate Data Scientist at KDataScience, with responibilities for data warehousing, data preprocessing for PBI reports, and interacting with business stakeholders to implement business logic. Building PBI reports and adding features in reports as per business requirements. Currently, I am Part of the 1ViewETL project in building and integrating ETL services for 1ViewETL. 1ViewETL is about providing data center services more accessible way from all popular sources to any destination.