Implementing Rate Limiting and Throttling in ASP.NET Core Web APIs
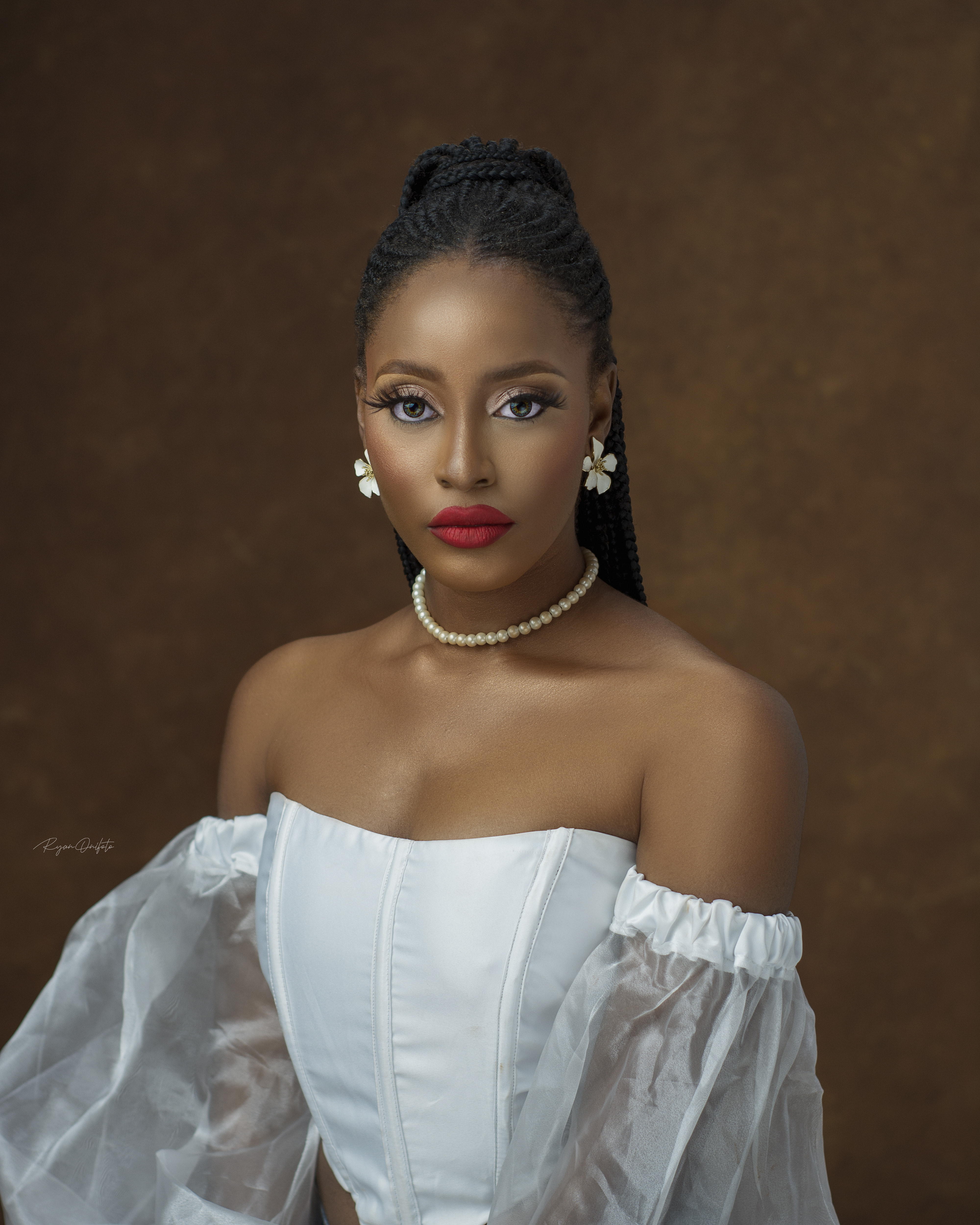
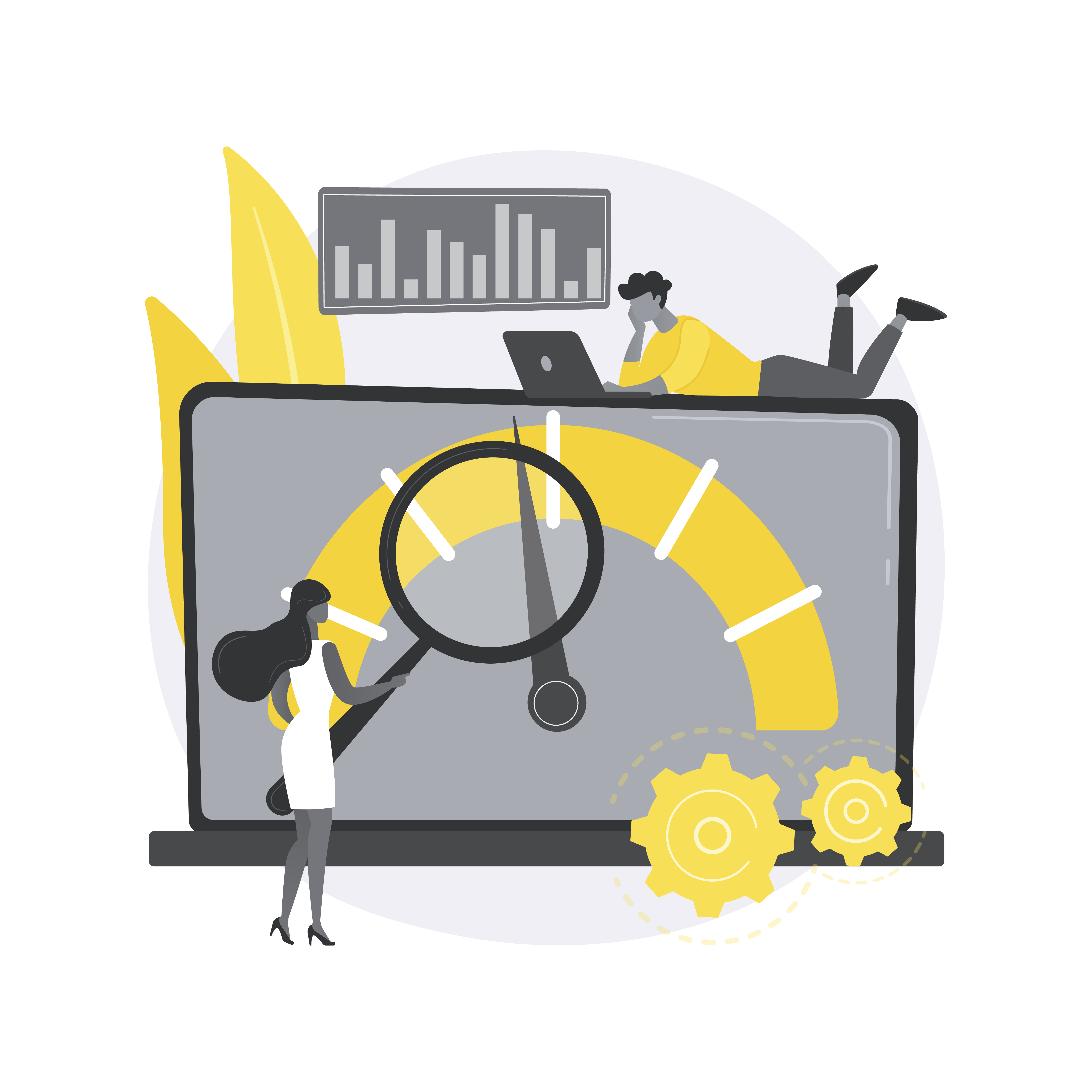
Today's installment is a guide to using rate limiting and throttling in ASP.NET Core Web APIs. When building web APIs, controlling how many requests a client can make in a given time is crucial.
This is where Rate Limiting and Throttling come into play. Rate Limiting is like setting a speed limit on a highway. It limits the number of requests a client can make to your API within a specific time frame. Throttling is a bit like traffic management. If a client is sending too many requests too quickly, throttling slows them down.
Pre-requisites
To fully benefit from this article, readers should have the following prerequisites:
Basic Knowledge of C# and ASP.NET Core
Understanding of C# syntax and concepts like classes, methods, and interfaces.
Familiarity with the ASP.NET Core framework, including how to create and run a Web API project.
Experience with Visual Studio or Visual Studio Code
- Ability to navigate and use an Integrated Development Environment (IDE) like Visual Studio or Visual Studio Code for developing ASP.NET Core applications.
Understanding of HTTP Protocol
Basic knowledge of HTTP requests and responses.
Awareness of concepts like HTTP methods (GET, POST, PUT, DELETE), status codes, and headers.
Familiarity with Middleware in ASP.NET Core
- Understanding of what middleware is and how it works in the ASP.NET Core request pipeline.
Basic Understanding of API Security Concepts
- Awareness of common API security practices, such as authentication and authorization.
Experience with NuGet Package Manager
- Familiarity with installing and managing packages using NuGet in ASP.NET Core projects.
Basic Knowledge of Networking Concepts (Optional)
- Understanding of IP addresses, whitelisting, and blacklisting may be helpful but not strictly necessary.
Table of Contents
Introduction to Rate Limiting and Throttling
Understanding the Basics
Setting Up Your ASP.NET Core Project
Implementing Rate Limiting in ASP.NET Core
Advanced Rate Limiting Techniques
Testing Your Rate Limiting Setup
Fine-Tuning Rate Limiting Policies
Monitoring and Scaling
Best Practices and Common Pitfalls
Conclusion and Further Reading
Introduction to Rate Limiting and Throttling
When building web APIs, it’s crucial to control how many requests a client can make in a given time period. This is where Rate Limiting and Throttling come into play.
What is Rate Limiting?
Rate Limiting is like setting a speed limit on a highway. It limits the number of requests a client can make to your API within a specific time frame. For example, you might allow only 100 requests per minute. If someone tries to exceed this limit, the extra requests will be blocked or delayed.
What is Throttling?
Throttling is a bit like traffic management. If a client is sending too many requests too quickly, throttling slows them down. Instead of blocking the extra requests completely, it might queue them or process them at a slower pace.
Why are Rate Limiting and Throttling Important?
Rate Limiting and Throttling help protect your API from being overwhelmed by too many requests at once. They ensure that your service remains stable, secure, and fair for all users. By controlling the flow of traffic, you prevent abuse, avoid server crashes, and maintain a smooth experience for everyone.
Understanding the Basics
Common Scenarios for Rate Limiting
Preventing Overloading:
Rate limiting helps protect your API from being overwhelmed by too many requests in a short period. For example, if too many users try to access your service at once, rate limiting ensures the API remains responsive by controlling the flow of requests.Security and Abuse Prevention:
It helps to block malicious users from spamming your API with excessive requests, which could lead to denial-of-service attacks or other security issues.Fair Usage:
Rate limiting ensures all users have equal access to your service, preventing any single user from hogging the resources.
Key Concepts: Requests, Limits, and Quotas
Requests:
These are the individual calls made to your API by users or other services. Every time someone interacts with your API, a request is made.Limits:
This is the maximum number of requests allowed within a specific timeframe. For example, you might allow 100 requests per minute.Quotas:
Quotas are similar to limits but typically apply over a longer period, like daily or monthly usage caps. For example, a user might be allowed 10,000 requests per day.
Types of Rate Limiting Strategies
Fixed Window:
This strategy limits requests based on a set time window, like 100 requests per minute. If a user hits the limit, they must wait until the next minute to send more requests.Sliding Window:
Unlike fixed windows, the sliding window strategy recalculates limits based on when the requests were made, providing a more flexible approach.Token Bucket:
This method allows requests to be processed as long as tokens are available in the bucket. Tokens are added over time, and if the bucket is empty, new requests are limited until more tokens are available. This strategy is useful for handling bursts of requests.
These concepts form the foundation of understanding how to implement rate limiting in your ASP.NET Core Web APIs.
Setting Up Your ASP.NET Core Project
Creating a New ASP.NET Core Web API Project
Start by opening your preferred development environment, like Visual Studio or Visual Studio Code.
Create a new project and choose "ASP.NET Core Web API" as the project template. This will set up the basic structure you need for building your web API.
Give your project a name and choose the framework version (preferably the latest version for the best features and security).
Once done, your new project will be ready with a default setup, including some sample code and folders.
Installing Necessary Packages for Rate Limiting
To implement rate limiting in your API, you need to install specific packages that provide the tools and functionalities.
Open the NuGet Package Manager in Visual Studio or use the terminal in Visual Studio Code.
Install the
AspNetCoreRateLimit
package by running the following command:dotnet add package AspNetCoreRateLimit
This package gives you built-in tools to easily set up and manage rate limiting in your ASP.NET Core Web API.
After installation, the package will be added to your project, ready to be configured for rate limiting.
Implementing Rate Limiting in ASP.NET Core
Adding Rate Limiting Middleware
Step 1:
Start by installing a NuGet package that provides rate limiting functionality. For example, you can use theAspNetCoreRateLimit
package like the above.Step 2:
Add the rate limiting middleware to your ASP.NET Core application in theStartup.cs
orProgram.cs
file. This middleware will automatically monitor and control the number of requests each user can make.
Configuring Rate Limiting Options
Step 1:
Define the rate limiting rules in yourappsettings.json
file. You can specify options like the maximum number of requests allowed and the time period (e.g., 100 requests per 1 minute).Step 2:
These rules can be applied globally or to specific endpoints in your API. This configuration helps to prevent users from overwhelming your API with too many requests in a short time.
Setting Up Basic Policies (e.g., Per-User, Per-IP)
Per-User Policy:
Limit requests based on the user making the request. For example, each user can only make a certain number of requests per minute.Per-IP Policy:
Limit requests based on the IP address making the request. This prevents any single IP address from flooding your API with requests.How to Set Up:
You can easily define these policies in theappsettings.json
file by specifying the key (e.g.,User
orIP
) and the corresponding limits.
Advanced Rate Limiting Techniques
Customizing Rate Limiting Rules
What It Means:
Instead of using default settings, you can create custom rules to control how often users can make requests.Example:
You might allow users to make 100 requests per minute, but only 10 requests per second to prevent sudden bursts of traffic.
Implementing IP Whitelisting/Blacklisting
What It Means:
You can allow or block specific IP addresses from making requests to your API.Example:
If you trust certain users or systems, you can whitelist their IP addresses, giving them more lenient rate limits. Conversely, you can block suspicious IPs entirely by blacklisting them.
Handling Rate Limiting Responses and Error Messages
What It Means:
When users hit the rate limit, you need to let them know with clear messages.Example:
If a user exceeds their limit, you can send a response that says, “Too many requests, please try again in 30 seconds,” along with the appropriate HTTP status code (like 429 Too Many Requests).
Rate Limiting in ASP.NET Core
What It Means:
This involves implementing rate limiting within an ASP.NET Core application, using available tools and libraries.Example:
You can use middleware in ASP.NET Core to apply rate limiting rules to different parts of your API, ensuring users don’t overwhelm your system.
Testing Your Rate Limiting Setup
Simulating API Requests to Test Limits
To ensure that your rate limiting setup works correctly, you can simulate multiple API requests in a short period. This helps you verify that your API is correctly enforcing the limits you’ve set.
Use Postman or CURL:
Tools like Postman or CURL allow you to send a large number of requests quickly. Try sending more requests than your rate limit allows to see if the extra requests are blocked or receive a "Too Many Requests" response (usually a 429 HTTP status code).Automate with a Script:
You can also write a simple script in C# or another language to send multiple requests in a loop. This helps you see how your API behaves when it receives a burst of traffic.
Monitoring and Logging Rate-Limited Requests
Once you've set up rate limiting, it's important to monitor and log requests that hit the limit so you can track usage patterns and identify potential issues.
Enable Logging:
Make sure logging is enabled in your ASP.NET Core application. You can log information like the time of the request, the user who made it, and the reason it was rate-limited.Review Logs:
Regularly check your logs to see how often users hit the rate limit. This helps you understand if your limits are set appropriately and if any users are being blocked too frequently.Set Up Alerts (Optional):
If your application is in production, consider setting up alerts to notify you when rate limits are exceeded often. This can help you respond quickly to unexpected spikes in traffic.
Testing and monitoring are key to ensuring that your rate limiting setup is effective and doesn't disrupt legitimate users.
Fine-Tuning Rate Limiting Policies
Adjusting Rate Limits for Different Endpoints:
Not all parts of your API are the same; some are more critical or resource-intensive than others. For example, you might want stricter limits on endpoints that perform heavy database operations or access sensitive data.
You can set different rate limits for each endpoint to ensure that your system remains responsive and that no single part of your API becomes a bottleneck.
Balancing Performance and Security:
Rate limiting helps protect your API from misuse and overload, but if the limits are too strict, legitimate users might be affected.
The key is to find a balance where you provide a good user experience while still protecting your API from abuse. This might involve setting higher limits during peak hours or adjusting them based on user feedback.
Handling High Traffic Scenarios:
During times of high traffic, like a sale or an event, your API might receive a lot of requests. Proper rate limiting ensures your system can handle the load without crashing.
Consider implementing dynamic rate limits that can adjust automatically based on the current load, or temporarily relaxing limits during known high-traffic periods to ensure a smooth user experience.
This approach ensures that your API remains fast, secure, and reliable, even under varying conditions.
Monitoring and Scaling
Using Monitoring Tools to Track API Usage
Monitoring is all about keeping an eye on how your API is performing and how it's being used. This helps you ensure that everything is running smoothly and allows you to spot any potential issues early on.
Why Monitor? Monitoring lets you track how many requests your API is handling, how often rate limits are being hit, and if there are any errors. This information is crucial for maintaining a good user experience and ensuring your API performs well.
How to Monitor? Use monitoring tools like:
Application Insights: A tool provided by Microsoft that helps you track the performance and usage of your application. It can show you how often your API is being accessed, if there are any performance bottlenecks, and if users are hitting rate limits.
Grafana: A tool that visualizes data from various sources. You can use it to create dashboards that show how your API is being used over time.
Seq: A logging tool that helps you analyze log data from your API. It’s useful for finding issues and understanding how your API is being used.
Scaling Rate Limiting for High-Volume Applications
Scaling means adjusting your system to handle more traffic or more users. For high-volume applications where many users are making requests to your API, you might need to adjust your rate limiting to handle the increased load.
Why Scale? As your application grows and more users start using your API, the amount of traffic can increase. Proper scaling ensures your API remains responsive and doesn’t become overloaded.
How to Scale? Consider the following strategies:
Adjust Rate Limits:
Increase or decrease the number of allowed requests per user based on your API’s load. For example, during peak times, you might temporarily increase the limit to accommodate more traffic.Distribute Load:
Use multiple servers or instances to handle incoming requests. This helps to balance the load and prevent any single server from becoming a bottleneck.Cache Results:
Implement caching to reduce the number of requests hitting your API. For example, store frequently requested data temporarily so that repeated requests can be served quickly without hitting the API every time.
Best Practices and Common Pitfalls
Ensuring Fairness Across Users:
Use Consistent Rules:
Apply the same rate limiting rules to all users to ensure fairness. For example, if you limit API calls to 100 per hour, this should be the same for every user.Consider User Types:
If you have different types of users (e.g., free vs. paid), you might need different rate limits. Ensure your system handles these variations fairly.Monitor Usage:
Regularly check how your rate limiting is affecting different users. Make adjustments if some users are being unfairly restricted.
Avoiding Common Rate Limiting Mistakes:
Don’t Overcomplicate Limits:
Start with simple rate limits (e.g., 100 requests per hour) and avoid making the rules too complex. This helps in easy management and understanding.Handle Edge Cases:
Ensure your rate limiting logic can handle situations like bursts of traffic or system failures gracefully. For example, have a way to handle when limits are accidentally exceeded.Test Thoroughly:
Before deploying, test your rate limiting rules under different scenarios to make sure they work as expected.
Maintaining a Positive User Experience:
Provide Clear Error Messages:
If a user hits their rate limit, provide a clear and friendly message explaining why and when they can try again.Offer Rate Limit Exemptions:
Consider offering rate limit exemptions for critical users or requests if it makes sense for your application.Monitor and Adjust:
Regularly review the impact of rate limiting on user experience. Be ready to adjust limits to avoid negatively impacting your users.
By following these best practices and avoiding common pitfalls, you can implement rate limiting effectively while maintaining a positive experience for your users.
Conclusion and Further Reading
Summary of Key Takeaways
Rate Limiting and Throttling:
You’ve learned how to control the number of requests users can make to your API within a certain time frame, helping to prevent abuse and ensure fair usage.Implementing Middleware:
We covered how to add and configure rate limiting middleware in your ASP.NET Core application to automatically manage and enforce these limits.Fine-Tuning Policies:
You now know how to adjust your rate limiting settings to balance between user experience and protection, ensuring optimal performance and security.
Additional Resource for Learning More
- Microsoft Documentation:
Explore official guides on ASP.NET Core Middleware to understand more about how middleware works in ASP.NET Core.
I hope you found this guide helpful and learned something new. Stay tuned for the next article in the "Mastering C#" series: Monitoring and Logging in ASP.NET Core Web APIs: Using Application Insights and ELK Stack
Happy coding!
Subscribe to my newsletter
Read articles from Opaluwa Emidowo-ojo directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
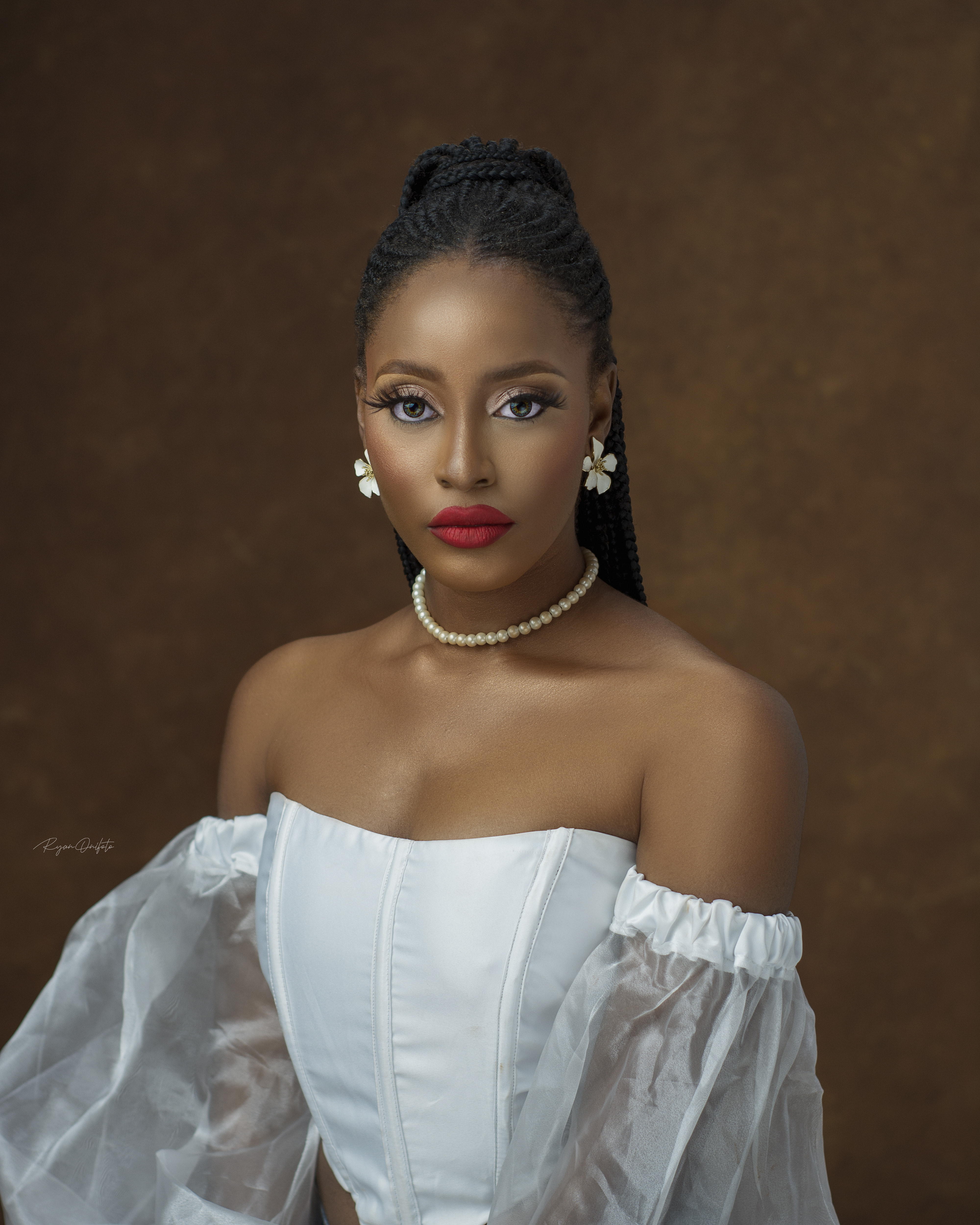