Debugging 101-How to Find and Fix Your First Bugs
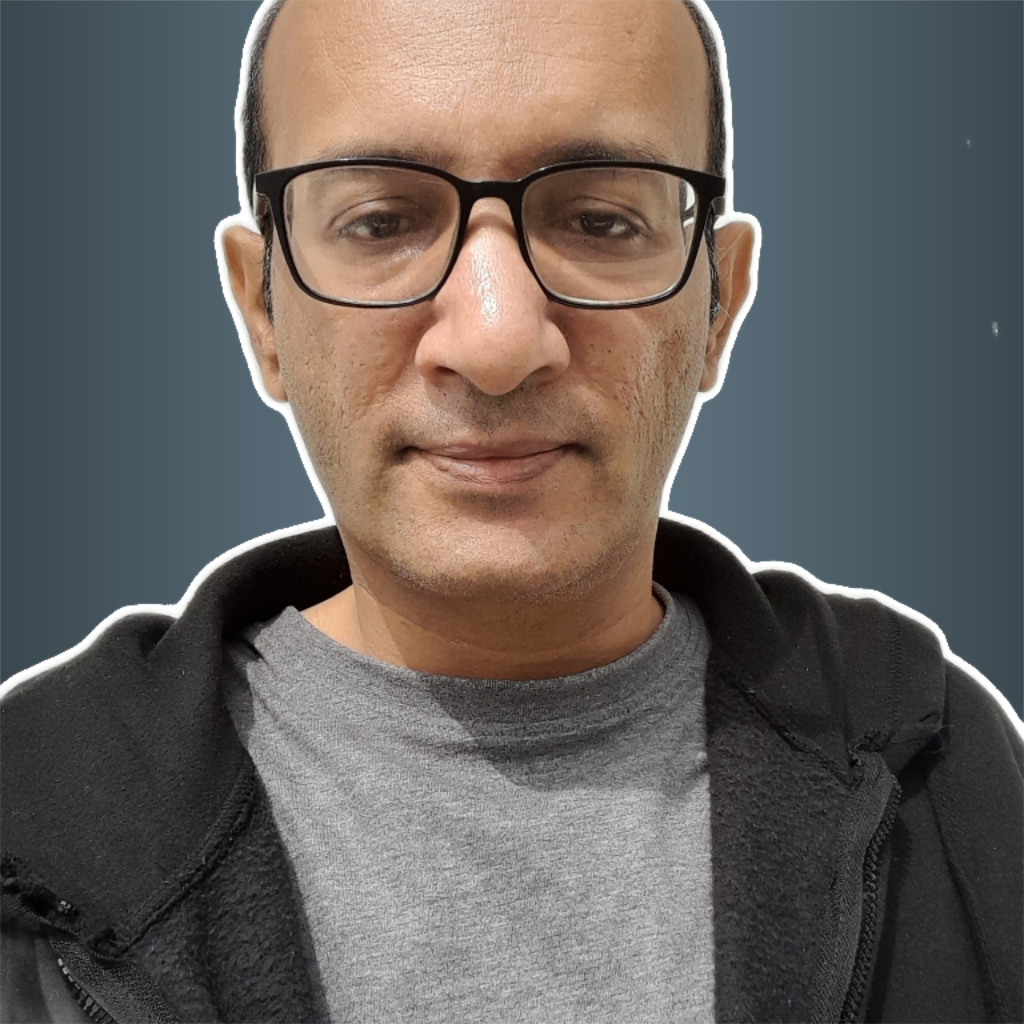
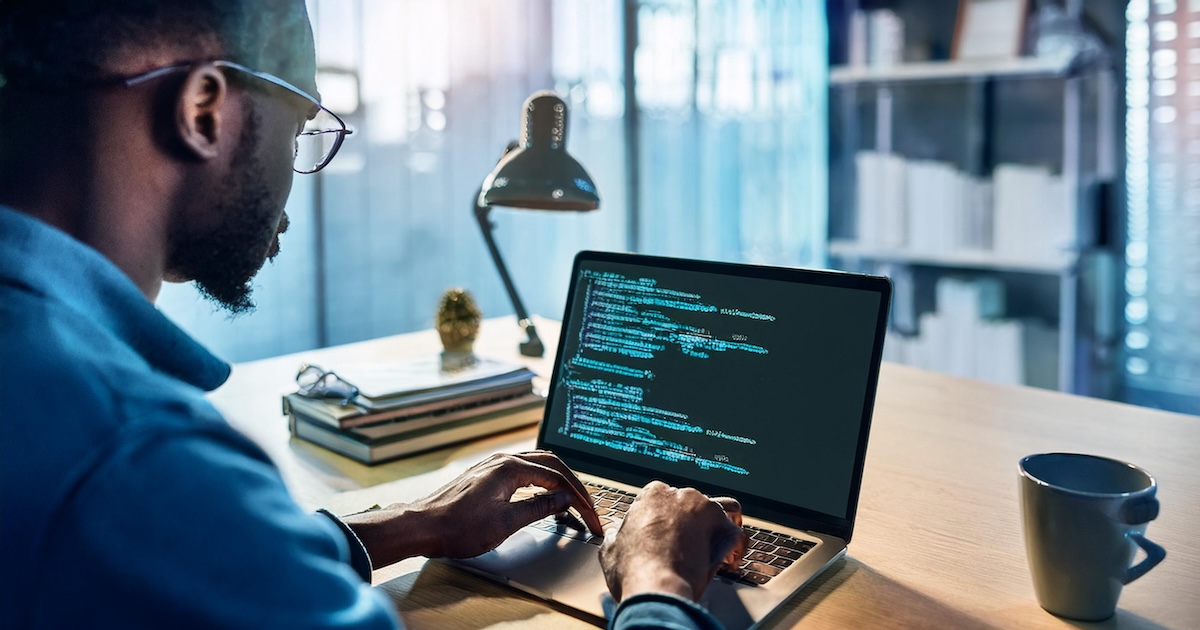
Debugging is an essential skill for any programmer, whether you're a beginner or an experienced developer. It’s the process of identifying, analyzing, and fixing bugs—errors or issues in your code that cause it to behave unexpectedly. In this post, we'll cover the basics of debugging, provide tips for finding and fixing your first bugs, and help you develop the mindset needed to troubleshoot effectively.
What is Debugging?
Debugging involves diagnosing and resolving issues in your code. Bugs can range from syntax errors that prevent your code from running to logical errors that cause incorrect output. Learning to debug effectively is crucial for improving your coding skills and ensuring your programs run smoothly.
Common Types of Bugs
Before diving into debugging techniques, it’s helpful to understand the different types of bugs you might encounter:
Syntax Errors: These occur when you write code that doesn’t follow the rules of the programming language. Common syntax errors include missing semicolons, unmatched parentheses, and incorrect indentation.
Runtime Errors: These errors occur while your program is running. They can be caused by issues like dividing by zero, accessing an undefined variable, or trying to open a file that doesn’t exist.
Logical Errors: These are the trickiest to catch because they don’t prevent your code from running but cause it to produce incorrect results. Logical errors often stem from mistakes in your algorithm or flawed reasoning in your code.
Semantic Errors: These errors occur when the code executes correctly but does not produce the intended outcome, often due to misunderstandings of the problem requirements.
Step-by-Step Guide to Debugging
Now that you know the types of bugs, let's walk through a systematic approach to debugging:
1. Reproduce the Bug
The first step in debugging is to reproduce the bug. This means running your program in a way that consistently triggers the error. By reproducing the bug, you can better understand its nature and identify where it occurs in your code.
Tip: Reproduce the bug with different inputs to see if it’s an isolated issue or a recurring problem.
2. Read the Error Message
If your program throws an error, read the error message carefully. Most programming languages provide detailed error messages that include the type of error, the line number where it occurred, and a brief description. This information is your first clue in diagnosing the problem.
Tip: Don’t just skim the error message—read it thoroughly. Sometimes, the solution is hidden in the details.
3. Use Print Statements or Logging
One of the simplest debugging techniques is to insert print statements or logging into your code. By printing out variable values, function outputs, and checkpoints in your code, you can track the flow of execution and identify where things go wrong.
Example:
print("Starting the loop")
for i in range(10):
print(f"i is {i}")
if i == 5:
print("Found the bug!")
Tip: If you’re using a larger project, consider using a logging library to manage your debug output more effectively.
4. Leverage Debugging Tools
Modern code editors and IDEs (Integrated Development Environments) come with powerful debugging tools. These tools allow you to set breakpoints, step through your code line by line, and inspect the values of variables at different stages of execution.
Popular Debugging Tools:
Visual Studio Code: Offers a built-in debugger for languages like Python, JavaScript, and more.
PyCharm: A popular Python IDE with advanced debugging features.
Chrome DevTools: Great for debugging JavaScript in web applications.
Tip: Learn to use the debugging tools available in your development environment to speed up the debugging process.
5. Check Your Assumptions
Often, bugs arise from incorrect assumptions about how your code should work. If you’re stuck, take a step back and question your assumptions. Are you sure the input data is correct? Did you consider all edge cases? Are you certain about how a particular function behaves?
Tip: Writing tests can help validate your assumptions and catch bugs early.
6. Simplify the Problem
If the bug is elusive, try simplifying your code to isolate the problem. Remove or comment out parts of your code that aren’t directly related to the bug. By narrowing down the scope, you can focus on the section that’s causing the issue.
Tip: Work incrementally—test your code after every small change to see if the bug is resolved.
7. Seek Help When Needed
If you’ve tried everything and still can’t find the bug, don’t hesitate to seek help. Consult the documentation, search online forums like Stack Overflow, or ask a more experienced developer for guidance. Sometimes, a fresh set of eyes can spot the issue quickly.
Tip: When asking for help, provide as much context as possible, including the relevant code, error messages, and steps to reproduce the bug.
Best Practices for Preventing Bugs
While debugging is an essential skill, preventing bugs in the first place is even better. Here are some best practices to minimize the chances of introducing bugs into your code:
Write Clear and Concise Code: Simple, readable code is less prone to bugs and easier to debug.
Test Regularly: Write tests for your code and run them frequently to catch issues early.
Use Version Control: Tools like Git allow you to track changes and revert to previous versions if a bug is introduced.
Code Reviews: Having someone else review your code can catch potential bugs you might have missed.
Conclusion: Debugging is a Skill You Can Master
Debugging is an integral part of coding that every developer must master. By following the steps outlined in this guide—reproducing the bug, reading error messages, using print statements, leveraging debugging tools, and questioning your assumptions—you’ll become more efficient at finding and fixing bugs.
Remember, every bug is an opportunity to learn. As you debug more, you’ll develop a deeper understanding of how your code works and become a more confident programmer. Keep practicing, stay patient, and soon, debugging will become second nature to you.
Subscribe to my newsletter
Read articles from Azhar Hussain directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
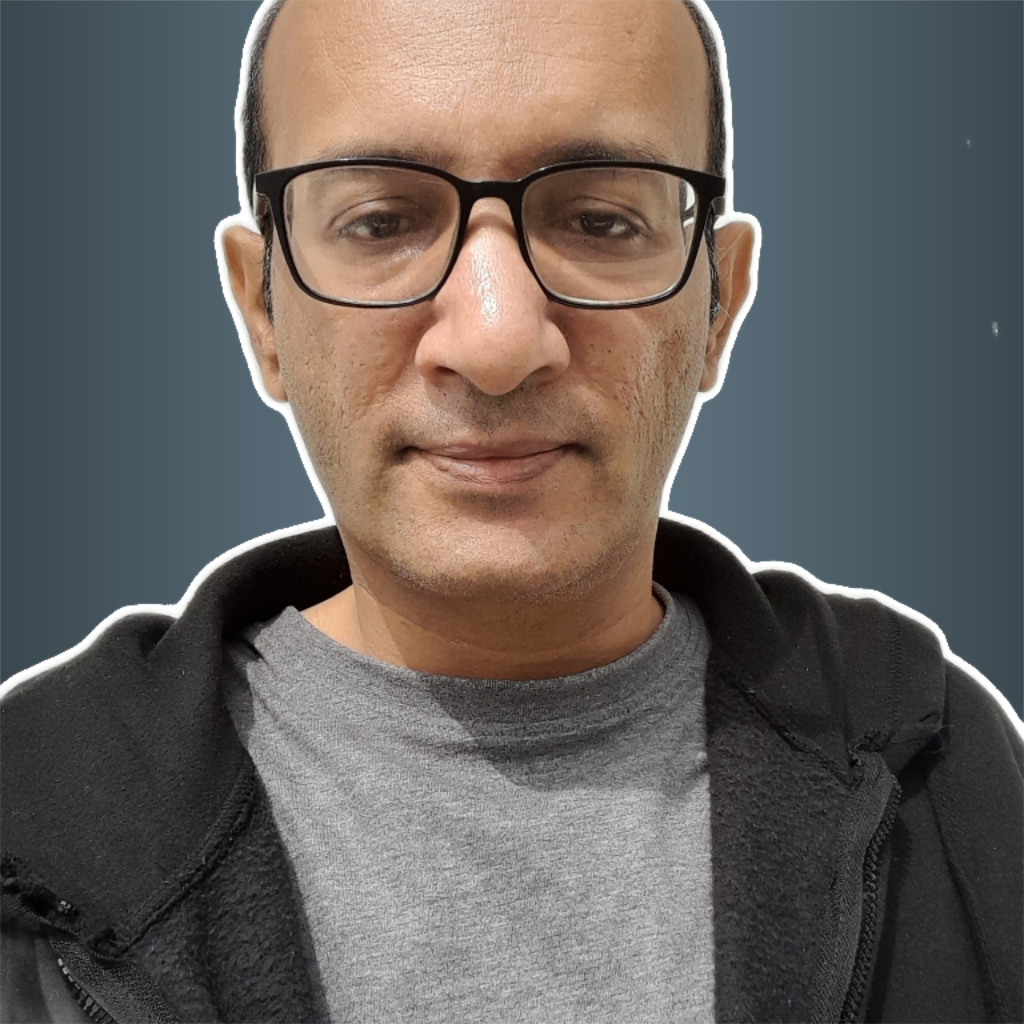
Azhar Hussain
Azhar Hussain
Over 2 decades of software engineering experience, including over a decade in building, scaling and leading engineering teams.